How to blink multiple LED
How can I blink multiple LEDs WITHOUT using delay() function in Arduino? I tried to use millis() functions but I have trouble managing timestamps. What is the easiest way to do it?
Answer
Managing timestamps when blinking multiple LEDs is not easy for newbies. Fortunately, the ezOutput library supports blinking multiple LED. The timestamp is managed by the library. Therefore, we can use this library without managing timestamps. Further more, you can use an array of LEDs to make code clean and short
Arduino Code - Blink Multiple LEDs
The below example code is for three LEDs.
/*
* Created by ArduinoGetStarted.com
*
* This example code is in the public domain
*
* Tutorial page: https://arduinogetstarted.com/tutorials/arduino-output-library
*
* This example blinks 3 LED:
* + with diffent frequencies
* + start blink at the same time
* + without using delay() function. This is a non-blocking example
*/
#include <ezOutput.h> // ezOutput library
ezOutput led1(7); // create ezOutput object that attach to pin 7;
ezOutput led2(8); // create ezOutput object that attach to pin 8;
ezOutput led3(9); // create ezOutput object that attach to pin 9;
void setup() {
led1.blink(500, 250); // 500 milliseconds ON, 250 milliseconds OFF
led2.blink(250, 250); // 250 milliseconds ON, 250 milliseconds OFF
led2.blink(100, 100); // 100 milliseconds ON, 100 milliseconds OFF
}
void loop() {
led1.loop(); // MUST call the led1.loop() function in loop()
led2.loop(); // MUST call the led2.loop() function in loop()
led3.loop(); // MUST call the led3.loop() function in loop()
}
The wiring diagram for above code:
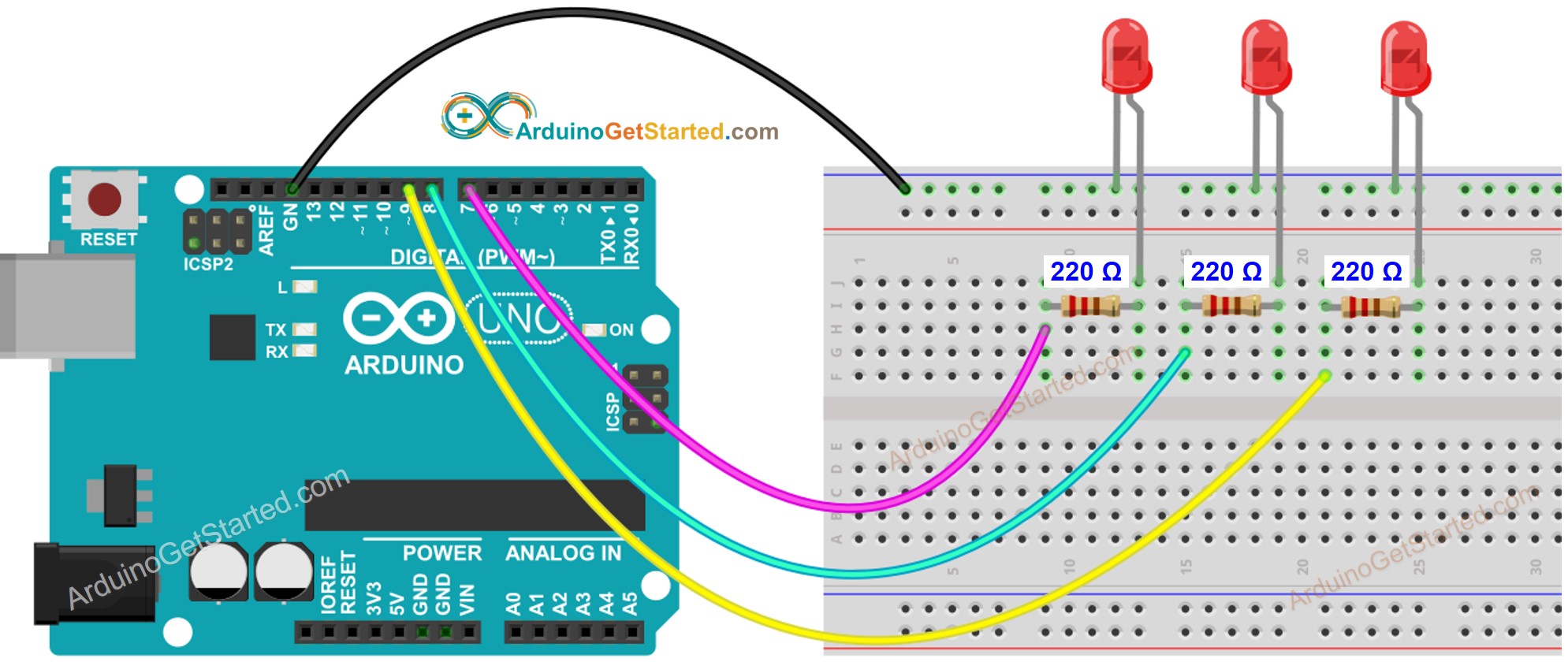
This image is created using Fritzing. Click to enlarge image
Hardware for above code
1 × Arduino UNO Buy on Amazon | |
1 × USB 2.0 cable type A/B Buy on Amazon | |
3 × LED Buy on Amazon | |
3 × 220 ohm resistor Buy on Amazon | |
1 × Breadboard Buy on Amazon | |
1 × Jumper Wires Buy on Amazon |
Disclosure: Some links in this section are Amazon affiliate links. If you make a purchase through these links, we may earn a commission at no extra cost to you.
Additionally, some links direct to products from our own brand, DIYables .
Additionally, some links direct to products from our own brand, DIYables .
Array of LEDs
When you use many LEDs, you can use an array of LEDs to make code clean and short. The below example code use an array for 5 LEDs.
/*
* Created by ArduinoGetStarted.com
*
* This example code is in the public domain
*
* Tutorial page: https://arduinogetstarted.com/faq/how-to-blink-multiple-led
*/
#include <ezOutput.h>
const int LED_NUM = 5;
const int LED_1_PIN = 2;
const int LED_2_PIN = 3;
const int LED_3_PIN = 4;
const int LED_4_PIN = 5;
const int LED_5_PIN = 6;
ezOutput ledArray[] = {
ezOutput(LED_1_PIN),
ezOutput(LED_2_PIN),
ezOutput(LED_3_PIN),
ezOutput(LED_4_PIN),
ezOutput(LED_5_PIN)
};
void setup() {
Serial.begin(9600);
for (byte i = 0; i < LED_NUM; i++) {
ledArray[i].blink(500, 250); // 500 milliseconds ON, 250 milliseconds OFF
}
}
void loop() {
for (byte i = 0; i < LED_NUM; i++)
ledArray[i].loop(); // MUST call the loop() function first
}