Arduino - Output Library
When using Arduino's output pin to control LED, relay..., beginners usually run into the following troubles:
- Blinks multiple output pins without blocking other tasks
- Time offset among blinking multiple output pins
The ezOutput (easy output) library is designed to solve all of the above problems and make it easy to use for not only beginners but also experienced users. It is created by ArduioGetStarted.com.
The library can be used for LED, relay, or an...
Library Features
- Support HIGH, LOW, PULSE and TOGGLE
- Support get state of output pin
- Supports blink without delay
- Supports blink in a period
- Easy to use with multiple output pins
- Support time offset in blinks multiple output pins
- All functions are non-blocking
※ NOTE THAT:
If this library is useful for your work, you can say thanks to us by buying us a coffee: PayPal.Me/arduinogetstarted
How To Install Library
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search “ezOutput”, then find the output library by ArduinoGetStarted
- Click Install button to install ezOutput library.
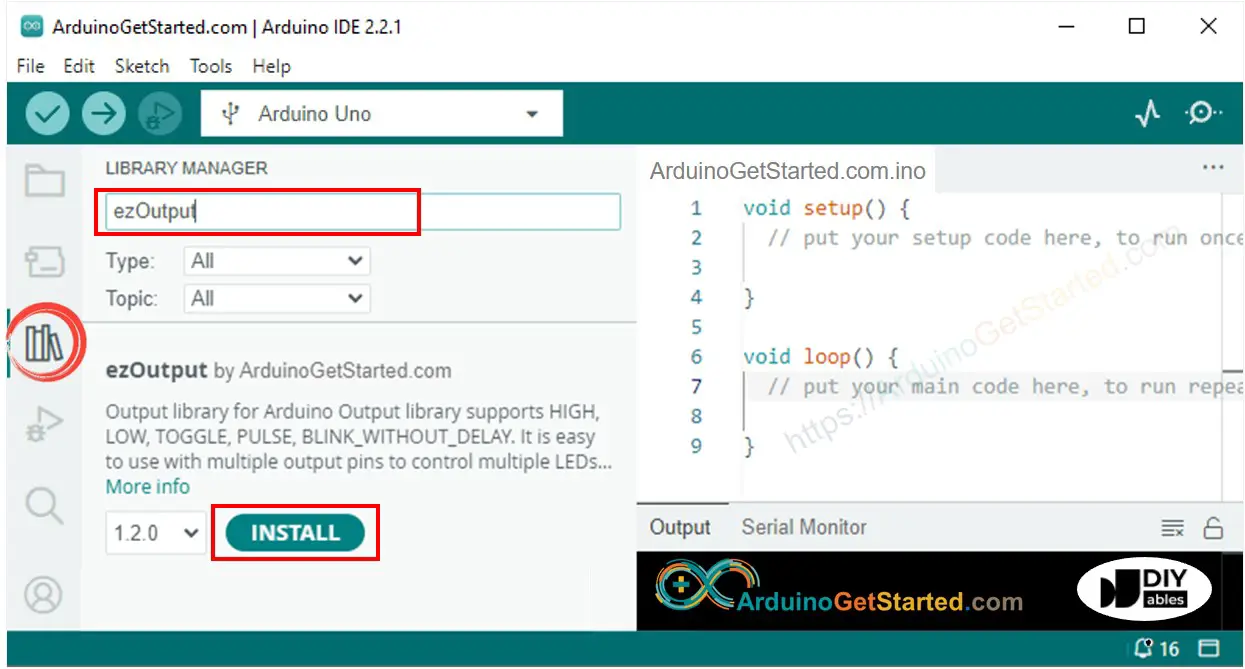
Or you can download it on Github
Examples
Functions
- ezOutput()
- high()
- low()
- toggle()
- pulse()
- blink()
- getState()
- loop()
References
ezOutput()
Description
This function creates a new instance of the ezOutput class that represents a particular output pin of your Arduino board.
Syntax
Parameters
pin: int - Arduino's pin.
Return
A new instance of the ezOutput class.
Example
high()
Description
This function sets output pin to HIGH.
Syntax
Parameters
None
Return
None
Example
low()
Description
This function sets output pin to LOW.
Syntax
Parameters
None
Return
None
Example
toggle()
Description
This function changes the state of output pin. If the current state is HIGH, this function changes the state to LOW, and vice versa.
Syntax
Parameters
- delayTime: unsigned long (in millisecond) - (optional) the time output pin is delayed before toggling. When we use this parameter, We have to call loop() function as fast as possible.
Return
None
Example
pulse()
Description
This function generates a square pulse.
- If the current state is LOW, this function generates a HIGH pulse.
- If the current state is HIGH, this function generates a LOW pulse.
We have to call loop() function as fast as possible.
Syntax
Parameters
- pulseTime: unsigned long (in millisecond) - the duration of pulse.
- delayTime: unsigned long (in millisecond) - (optional) the time output pin is delayed before a pulse starts.
Return
None
Example - HIGH pulse
Example - LOW pulse
blink()
Description
This function blinks (toggles) output pin continuously with specified LOW time and HIGH time. To keep blinking, we have to call loop() function as fast as possible. If the blink time is not specified, the blinking will be disabled only if we call low(), high() or toggle() functions.
Syntax
Parameters
- lowTime: unsigned long (in millisecond) - the time output pin is LOW in milliseconds.
- highTime: unsigned long (in millisecond) - the time output pin is HIGH in milliseconds.
- delayTime: unsigned long (in millisecond) - (optional) the time output pin is delayed before blinking. This is used when we want to blink multiple output pin with delay among the pins. If not set, the blinking is started immediately.
- blinkTimes: long - (optional) The number of times output toggles (changes between LOW to HIGH and HIGH to LOW). If not set, output blinks forever
Return
None
Example 1
Example 2
getState()
This function returns the current state of output pin.
Description
Syntax
Parameters
None
Return
The current state of output pin.
Example
loop()
loop()
Description
This function blinks output. It is used in combination with blink() function.
Syntax
Parameters
None
Return
None