Arduino - How to detect button press event
How to detect if a button is pressed and released using Arduino?
Answer
There are two ways to detect the button press event or release event:
- Using the button library (simple to use, recommended)
- Detecting the state's changes.
Let's see one by one.
The below wiring diagram will be used for both ways.
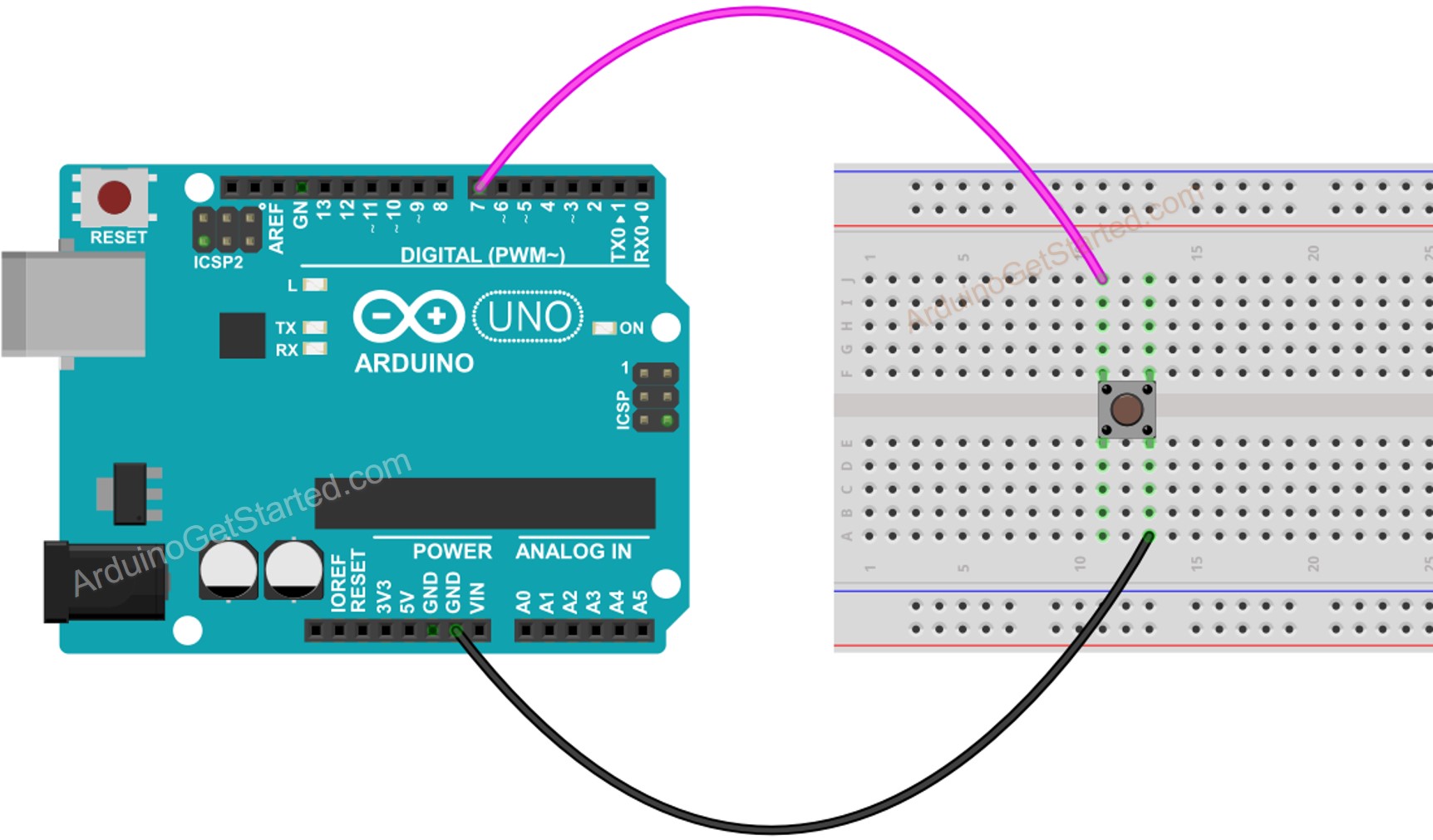
This image is created using Fritzing. Click to enlarge image
Detecting the button press by using library
#include <ezButton.h>
ezButton button(7); // create ezButton object that attach to pin 7;
void setup() {
Serial.begin(9600);
button.setDebounceTime(50); // set debounce time to 50 milliseconds
}
void loop() {
button.loop(); // MUST call the loop() function first
if(button.isPressed())
Serial.println("The button is pressed");
if(button.isReleased())
Serial.println("The button is released");
}
※ NOTE THAT:
- In the above code, the button is debounced. See Why needs to debounce for buttons
- The library is non-blocking, we do NOT need to worry about blocking other code.
Detecting the button press by detecting the state's changes
#define BUTTON_PIN 7
int lastButtonState;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP); // enable the internal pull-up resistor
lastButtonState = digitalRead(BUTTON_PIN);
}
void loop() {
// read the value of the button
int buttonState = digitalRead(BUTTON_PIN);
if (lastButtonState != buttonState) { // state changed
delay(50); // debounce time
if (buttonState == LOW)
Serial.println("The button pressed event");
else
Serial.println("The button released event");
lastButtonState = buttonState;
}
}
※ NOTE THAT:
- In the above code, the button is debounced. See Why needs to debounce for buttons
- The above code used the delay() function. This function blocks other code during the delay time. Depending on applicationm you may need to use millis() instead of delay().
⇒ Using the button library is the simplest way.
Buy Arduino
1 × Arduino UNO Buy on Amazon | |
1 × USB 2.0 cable type A/B Buy on Amazon | |
1 × Jumper Wires Buy on Amazon |
Disclosure: Some links in this section are Amazon affiliate links. If you make a purchase through these links, we may earn a commission at no extra cost to you.
Additionally, some links direct to products from our own brand, DIYables .
Additionally, some links direct to products from our own brand, DIYables .