Arduino - LED - Blink Without Delay
Let's imagine that Arduino has to do two tasks: blinking LED and checking the button state, which can be pressed anytime. If we use the delay() function (described in a previous tutorial), Arduino may miss some of the pressing events. In other words, Arduino cannot fully do the second task.
In this tutorial, we will learn how Arduino blinks LED and checks the button's state without missing any pressing event.
We will run though three below examples and compare the difference between them.
- Arduino blinks LED by using delay() function
- Arduino blinks LED by using millis() function
- Arduino blinks LED by using ezLED library
※ NOTE THAT:
- This method is not just only for blinking LED and checking the button's state. Generally, this method lets Arduino do several tasks at the same time without blocking each other.
- This tutorial provides in-depth knowledge that helps you understand the working principle. To make it easy, you can use Arduino - LED library.
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
Wiring Diagram
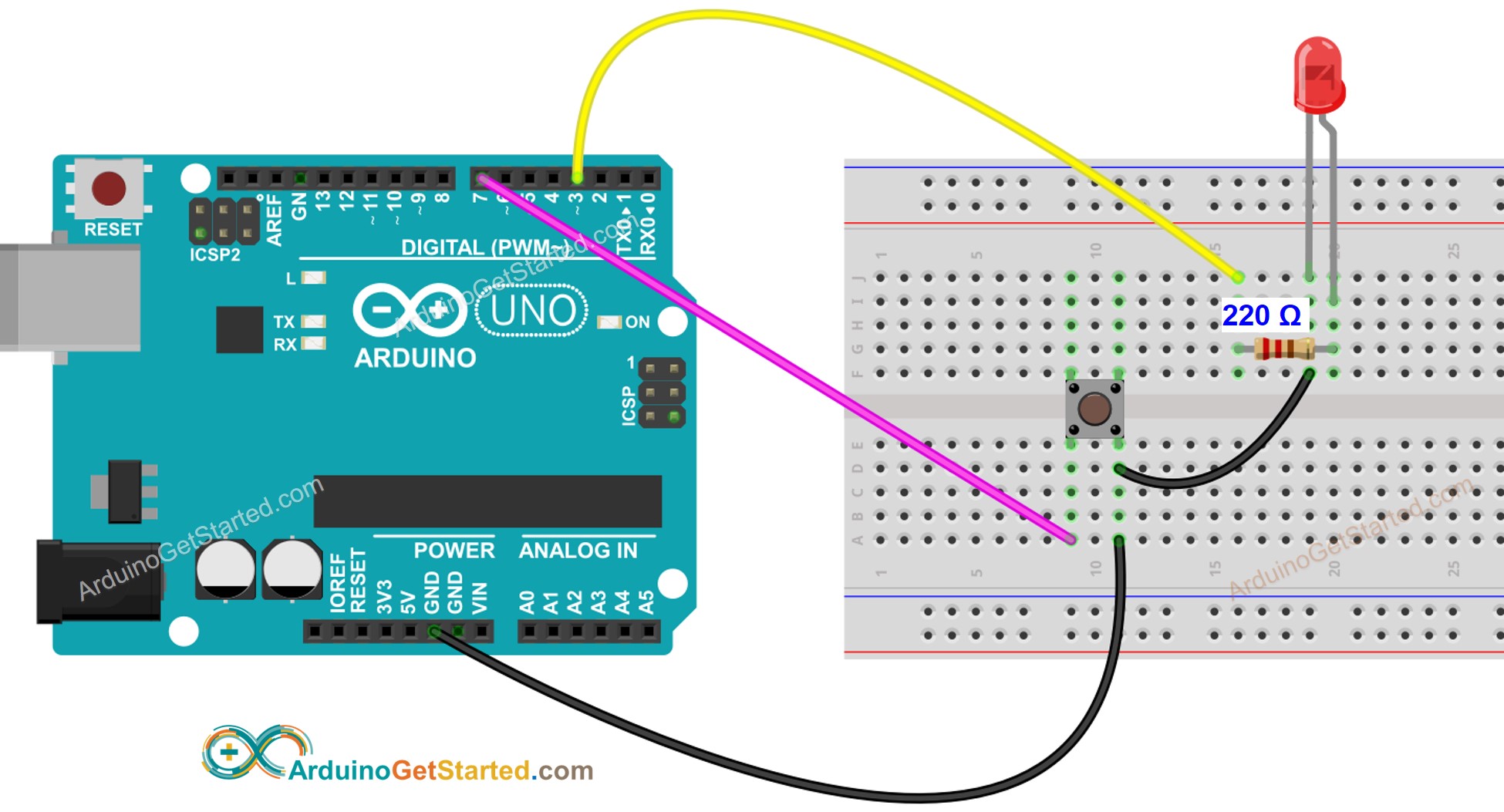
This image is created using Fritzing. Click to enlarge image
Arduino Code - With Delay
Quick Steps
- Connect Arduino to PC via USB cable
- Open Arduino IDE, select the right board and port
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
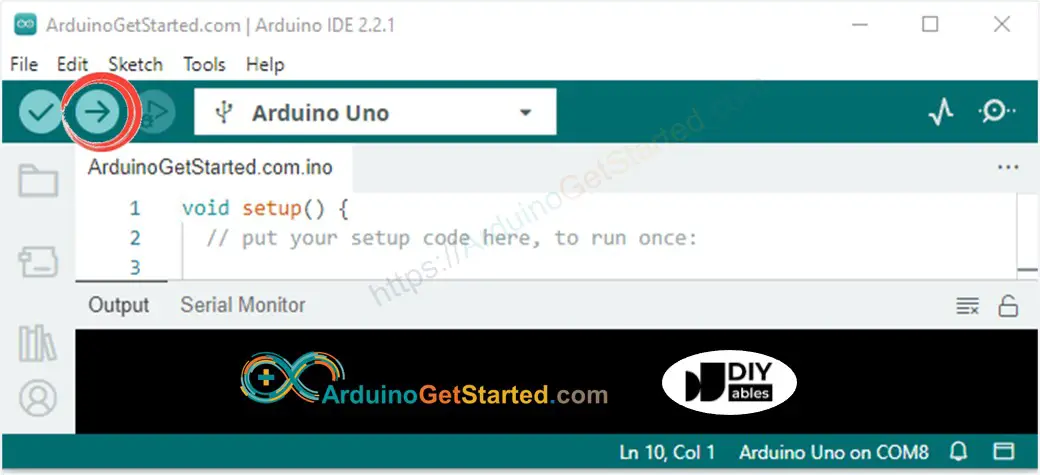
- Open Serial Monitor
- Press the button 4 times
- See the LED: The LED toggles between ON/OFF periodically every second
- See the output in Serial Monitor
- On Serial Monitor, some pressing times were missed. That is because, during delay time, Arduino CANNOT do anything. Therefore, it is unable to detect the pressing event.
Arduino Code - Without Delay
Quick Steps
- Run the above code and press the button 4 times
- See the LED: The LED toggles between ON/OFF periodically every second
- See the output in Serial Monitor
- All pressing events were detected.
Code Explanation
Read the line-by-line explanation in comment lines of code!
Adding More Tasks
The below code blinks two LEDs with different intervals and checks the state of the button.
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.
Extendability
This method can be used to let Arduino do several tasks at the same time without blocking each other. For example, sending a request to the Internet and waiting for the response, while waiting for the response, blink some LED indicators and check the cancel button.
LED on Commercial Products
Small LEDs usually are used to indicate the status of devices. For examples:
Big LEDs usually are used for lighting. They can be combined into groups. For examples: