Arduino - Button Library
When using buttons, beginners usually run into the following troubles:
- Floating input issue
- Chattering issue
- Detecting the pressed and released events
- Managing timestamp when debouncing for multiple buttons
The ezButton (easy button) library is designed to solve all of the above problems and make it easy to use for not only beginners but also experienced users. It is created by ArduioGetStarted.com.
The library can be used for push button, momentary switches, toggle switch, magnetic contact switch (door sensor)...
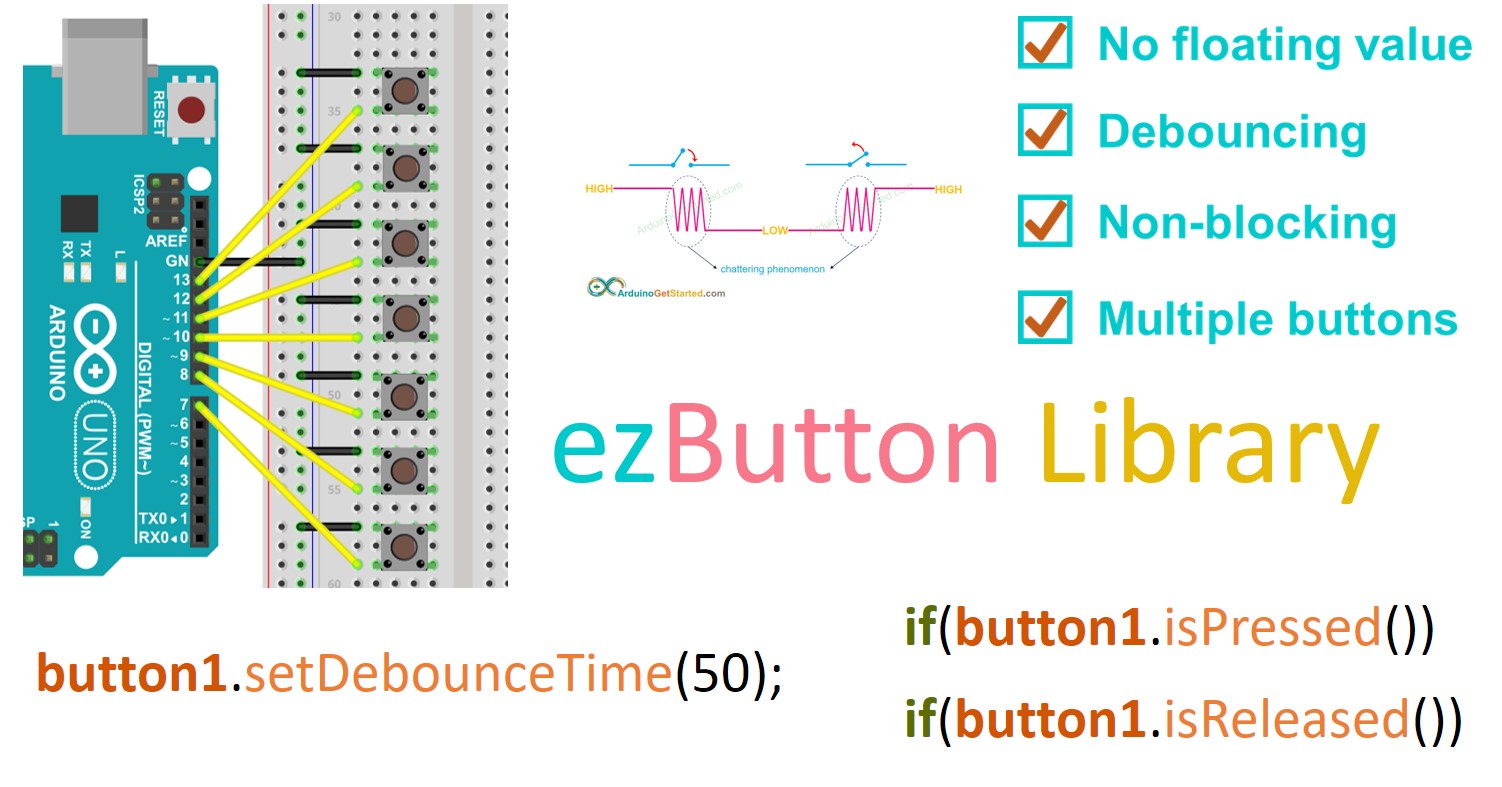
Library Features
- Uses the internal pull-up resistor to avoid the floating value
- Supports debounce to eliminate the chattering phenomenon
- Supports the pressed and released events
- Supports the counting (for FALLING, RISING and BOTH)
- Easy to use with multiple buttons
- All functions are non-blocking
※ NOTE THAT:
If this library is useful for your work, you can say thanks to us by buying us a coffee: PayPal.Me/arduinogetstarted
How To Install Library
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search “ezButton”, then find the button library by ArduinoGetStarted
- Click Install button to install ezButton library.
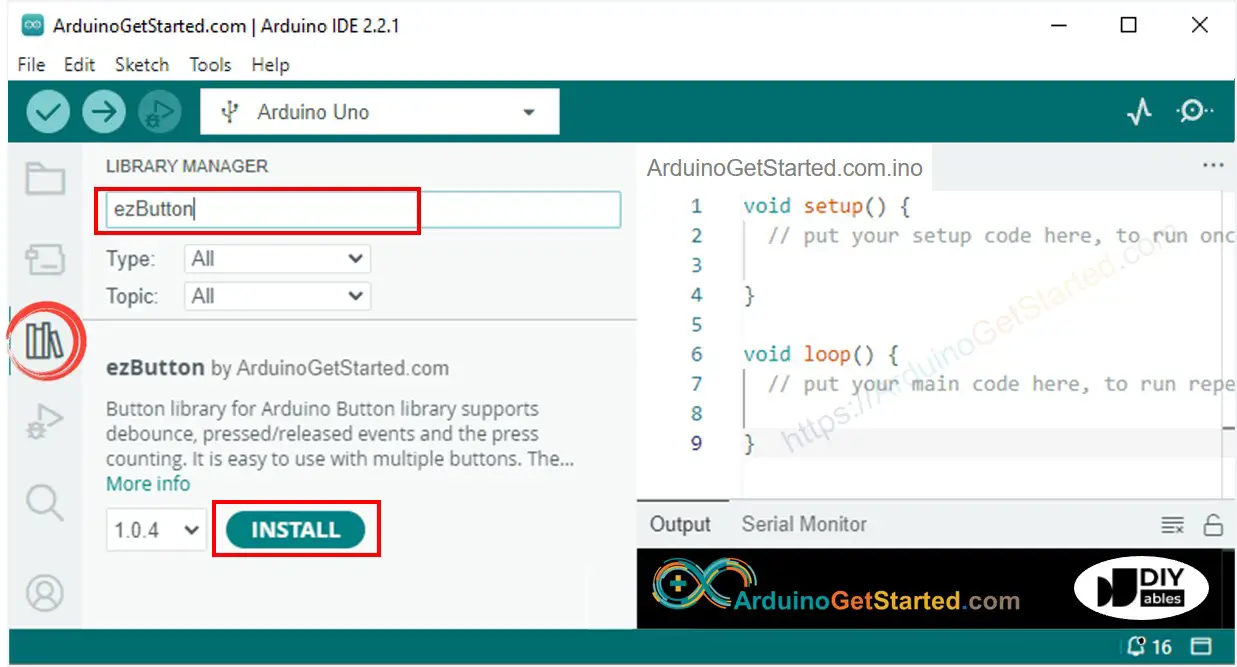
Or you can download it on Github
Examples
Functions
- ezButton()
- setDebounceTime()
- getState()
- getStateRaw()
- isPressed()
- isReleased()
- setCountMode()
- getCount()
- resetCount()
- loop()
References
ezButton()
Description
This function creates a new instance of the ezButton class that represents a particular button attached to your Arduino board.
Syntax
Parameters
pin: int - Arduino's pin that connects to the button.
Return
A new instance of the ezButton class.
Example
setDebounceTime()
Description
This function is used to set the debounce time. The debounce time takes effect on getState(), isPressed(), isReleased() and getCount().
Syntax
Parameters
time: unsigned long - debounce time in milliseconds
Return
None
Example
getState()
Description
This function returns the state of the button. If the debounce time is set, the returned state is the state after debouncing. We MUST call button.loop() function before using this function.
Syntax
Parameters
None
Return
The state of button. Since the ezButton library used the internal pull-up resistor, the state will be HIGH (1) when no press, and LOW (0) when pressed. If the debounce time is set, the returned state is the state after debouncing.
Example
getStateRaw()
This function returns the current state of the button without debouncing. It is equivalent to digitalRead().
Description
Syntax
Parameters
None
Return
The current state of button without debouncing.
Example
isPressed()
Description
This function check whether a button is pressed or not. We MUST call button.loop() function before using this function.
Syntax
Parameters
None
Return
true if the button is pressed, false otherwise
Example
isReleased()
Description
This function check whether a button is released or not. We MUST call button.loop() function before using this function.
Syntax
Parameters
None
Return
true if the button is released, false otherwise
Example
setCountMode()
Description
This function sets the count mode.
Syntax
Parameters
mode: int - count mode. The available count modes include: COUNT_FALLING, COUNT_RISING and COUNT_BOTH.
Return
None
Example
getCount()
Description
This function returns the count value. We MUST call button.loop() function before using this function.
Syntax
Parameters
None.
Return
The count value. If the debounce time is set, the returned value is the value after debouncing.
Example
resetCount()
Description
This function set the counter value to 0.
Syntax
Parameters
None.
Return
None.
Example
loop()
loop()
Description
This function does debounce and updates the state of the button. It MUST be called before using getState(), isPressed(), isReleased() and getCount() functions.
Syntax
Parameters
None
Return
None