How to reset Arduino by programming
How can I reset Arduino using Arduino code?
Answer
There are two way to reset Arduino by coding: hardware reset and software reset
Hardware Reset Arduino by coding
- Connnect an Arduino digital pin to RESET pin
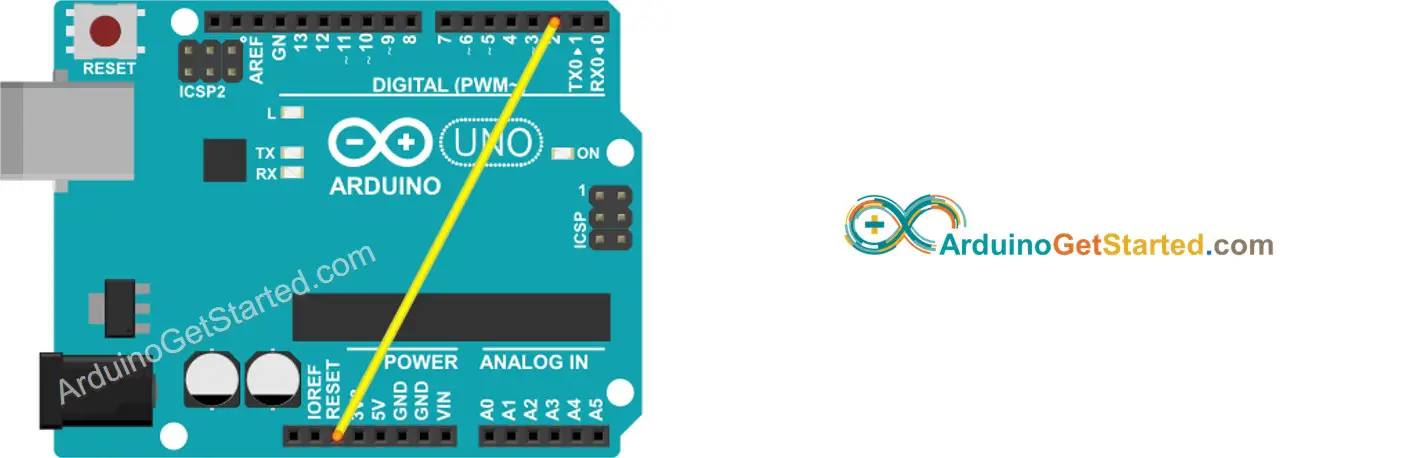
- Config the digital pin as a digital output pin by using pinMode() function.
- Program for the digital pin to LOW to reset Arduino by using digitalWrite() function.
/*
* Created by ArduinoGetStarted.com
*
* This example code is in the public domain
*
* Tutorial page: https://arduinogetstarted.com/faq/how-to-reset-arduino-by-programming
*/
const int OUTPUT_PIN = 2;
void setup() {
digitalWrite(OUTPUT_PIN, HIGH);
pinMode(OUTPUT_PIN, OUTPUT);
Serial.begin(9600);
Serial.println("How to Reset Arduino Programmatically");
}
void loop()
{
Serial.println("Arduino will be reset after 5 seconds");
delay(5000);
digitalWrite(OUTPUT_PIN, LOW);
Serial.println("Arduino never run to this line");
}
Software Reset Arduino by coding
- Declare the reset function
void(* resetFunc) (void) = 0; // declare reset fuction at address 0
- Call the reset function when needed
resetFunc(); //call reset
Buy Arduino
1 × Arduino UNO Buy on Amazon | |
1 × USB 2.0 cable type A/B Buy on Amazon |
Please note: These are Amazon affiliate links. If you buy the components through these links, We will get a commission at no extra cost to you. We appreciate it.