How to use the multiple passwords for keypad
How can I use two passwords, three passwords, four passwords, or more with keypad and Arduino?
Answer
The below Arduino code uses three passwords for keypad. you can easily modify it for two passwords, four passwords, or more.
#include <Keypad.h>
const int ROW_NUM = 4; //four rows
const int COLUMN_NUM = 3; //three columns
char keys[ROW_NUM][COLUMN_NUM] = {
{'1','2','3'},
{'4','5','6'},
{'7','8','9'},
{'*','0','#'}
};
byte pin_rows[ROW_NUM] = {9, 8, 7, 6}; //connect to the row pinouts of the keypad
byte pin_column[COLUMN_NUM] = {5, 4, 3}; //connect to the column pinouts of the keypad
Keypad keypad = Keypad( makeKeymap(keys), pin_rows, pin_column, ROW_NUM, COLUMN_NUM );
const String password_1 = "1234"; // change your password here
const String password_2 = "5642"; // change your password here
const String password_3 = "4545"; // change your password here
String input_password;
void setup(){
Serial.begin(9600);
input_password.reserve(32); // maximum input characters is 33, change if needed
}
void loop(){
char key = keypad.getKey();
if (key){
Serial.println(key);
if(key == '*') {
input_password = ""; // reset the input password
} else if(key == '#') {
if(input_password == password_1 || input_password == password_2 || input_password == password_3) {
Serial.println("password is correct");
// DO YOUR WORK HERE
} else {
Serial.println("password is incorrect, try again");
}
input_password = ""; // reset the input password
} else {
input_password += key; // append new character to input password string
}
}
}
The wiring diagram for above code:
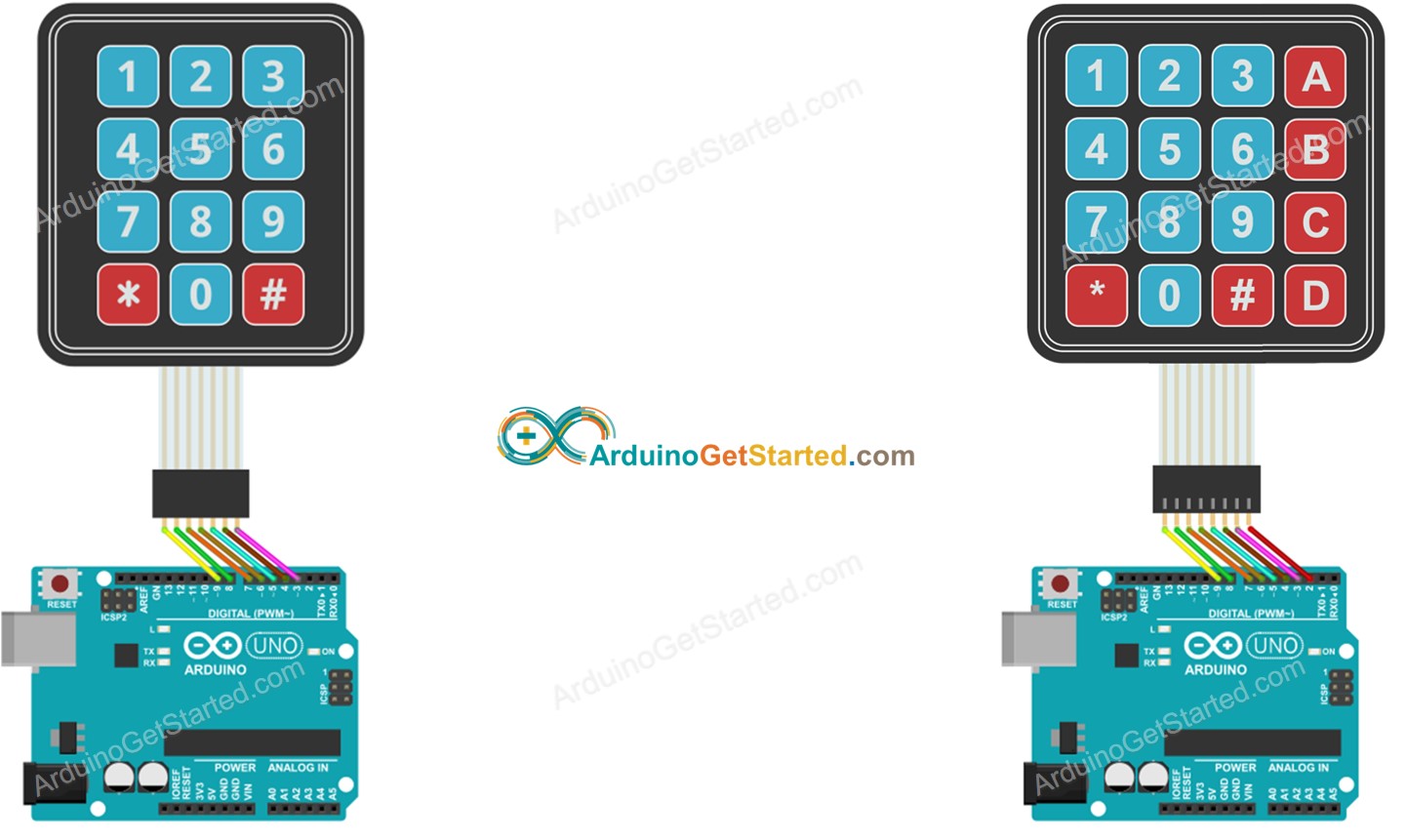
This image is created using Fritzing. Click to enlarge image
Hardware for above code
1 × Arduino UNO Buy on Amazon | |
1 × USB 2.0 cable type A/B Buy on Amazon | |
1 × Keypad 3x4 Buy on Amazon | |
1 × Keypad 4x4 (optional) Buy on Amazon |
Please note: These are Amazon affiliate links. If you buy the components through these links, We will get a commission at no extra cost to you. We appreciate it.
The Best Arduino Starter Kit
See Also
- If you are new to keypad, see Arduino - Keypad tutorial
- If you are new to keypad - password, see Arduino - Keypad - Password tutorial