How to input a multiple digits number using the keypad
Arduino keypad libary returns only a single key when reading. how can I read a multi digit input and put it into a numeric variable? How can read a number from keypad to set servo motor's angle, set temperature threshold... For example, if I press "120" keys on keypad, the value of an integer variable is 120. if I press "12" keys on keypad, the value of an integer variable is 12.
There are some ways to input the multiple digits number to a integer variable. The simplest way is described as follows:
When a key is pressed:
- If the key is a numeric key ("0" to "9", append the key to the input string.
- If the key is "#", end the input ⇒ convert the input string to integer.
- If the key is "*", start new input ⇒ clear the input string
The advantages of this method are simple and able to get one-digit number, two-digit number, three-digit, four-digit number, or any-digit number WITHOUT pre-defineing the mumber of digit.
Arduino Code for Reading the Multiple Digit Number For Keypad 3x4
Arduino Code for Reading the Multiple Digit Number For Keypad 4x4
The wiring diagram for above code:
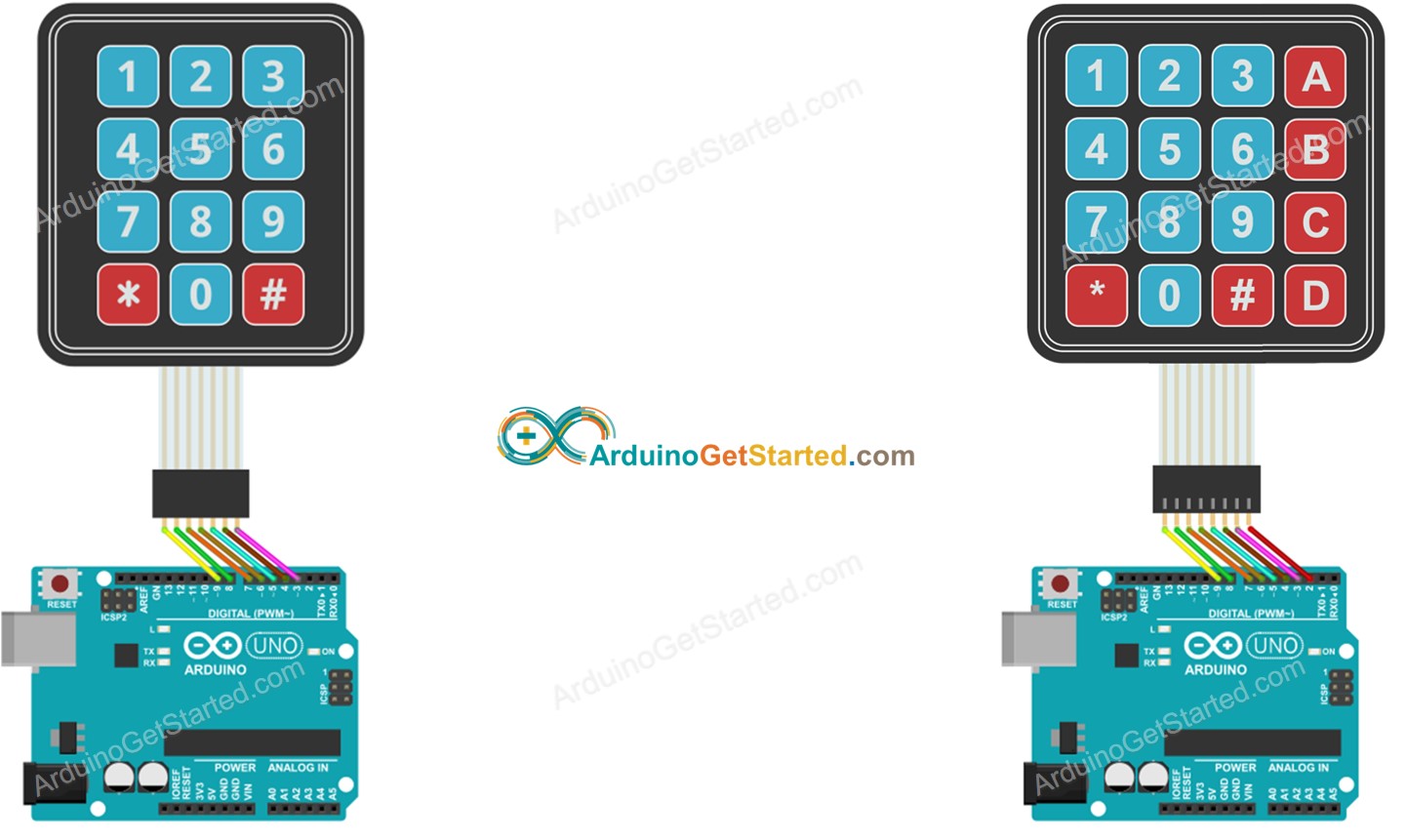
This image is created using Fritzing. Click to enlarge image
※ NOTE THAT:
In the above code, inputString.reserve(10) is used to prevent the memory fragmentation issue on String library
To learn more about keypad, see Arduino - Keypad tutorial
Hardware for above code
1 × Arduino UNO Buy on Amazon | |
1 × USB 2.0 cable type A/B Buy on Amazon | |
1 × Keypad 3x4 Buy on Amazon | |
1 × Keypad 4x4 (optional) Buy on Amazon |
Additionally, some links direct to products from our own brand, DIYables .