How to control speed of servo motor
How to control the speed of servo motor smoothly without using delay() function in Arduino code? How to rotate servo motor from this position to another position in a period of time? How to slow down the speed of servo motor?
Answer
To control the speed of servo motor without blocking other code, we can use millis() instead of delay()
Wiring Diagram
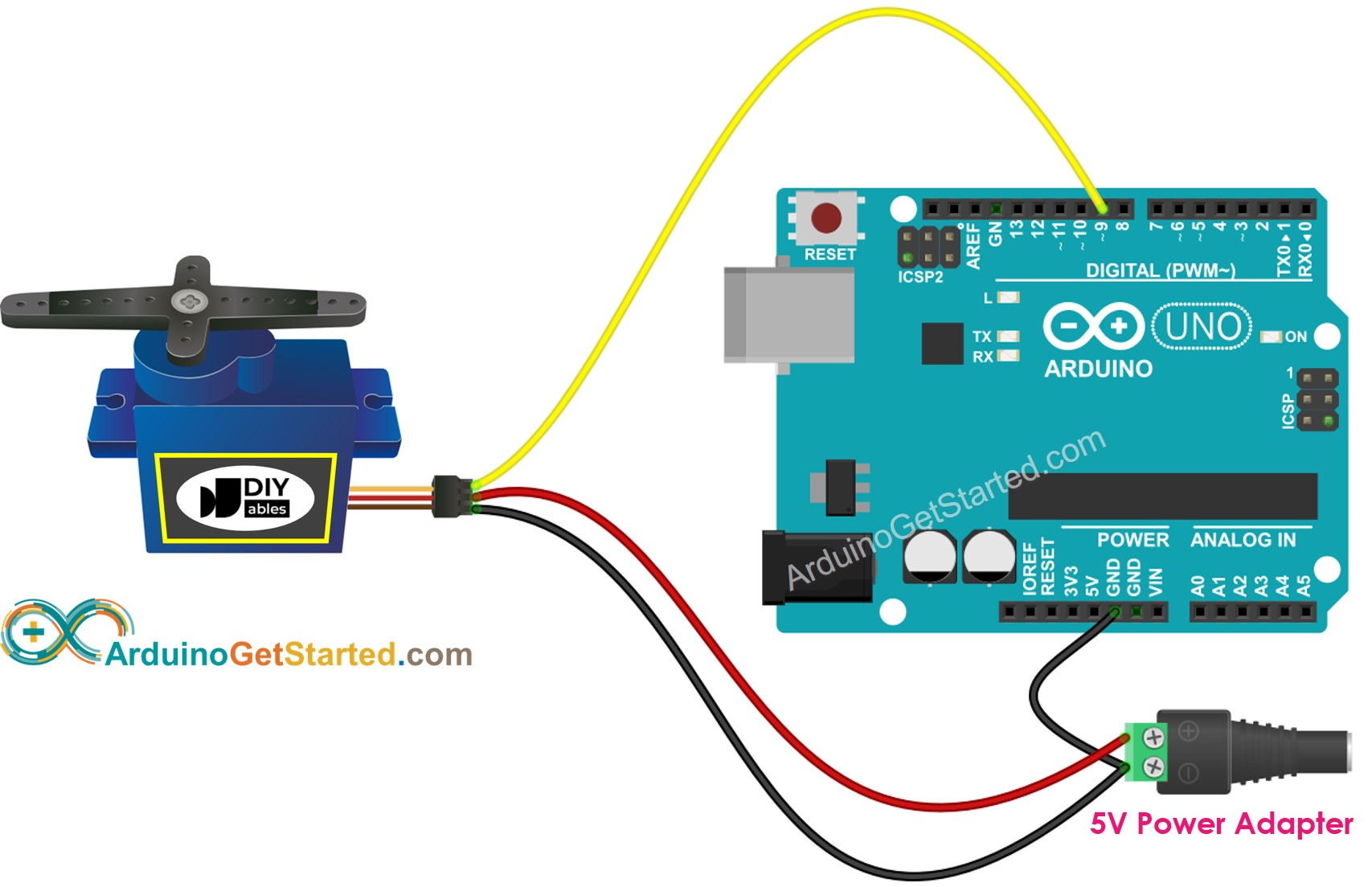
This image is created using Fritzing. Click to enlarge image
Arduino Code
By using map() and millis() functions, we can control the speed of servo motor smoothly without blocking other code.
The below example shows how to move the servo motor from 30° to 90° in 3 seconds.
/*
* Created by ArduinoGetStarted.com
*
* This example code is in the public domain
*
* Tutorial page: https://arduinogetstarted.com/faq/how-to-control-speed-of-servo-motor
*/
#include <Servo.h>
Servo myServo;
unsigned long MOVING_TIME = 3000; // moving time is 3 seconds
unsigned long moveStartTime;
int startAngle = 30; // 30°
int stopAngle = 90; // 90°
void setup() {
myServo.attach(9);
moveStartTime = millis(); // start moving
// TODO: other code
}
void loop() {
unsigned long progress = millis() - moveStartTime;
if (progress <= MOVING_TIME) {
long angle = map(progress, 0, MOVING_TIME, startAngle, stopAngle);
myServo.write(angle);
}
// TODO: other code
}
The Best Arduino Starter Kit
See Also
Function References
Buy Arduino
1 × Arduino UNO Buy on Amazon | |
1 × USB 2.0 cable type A/B Buy on Amazon | |
1 × Jumper Wires Buy on Amazon |
Please note: These are Amazon affiliate links. If you buy the components through these links, We will get a commission at no extra cost to you. We appreciate it.