How to fade LED in a time period
How to fade-in and fade-out LED in a time period without using delay() function?
Answer
To fade LED smoothly without blocking other code, we can use millis() instead of delay(). Let;s see example code for:
- Fade-in LED in a period without using delay()
- Fade-out LED in a period without using delay()
Wiring Diagram
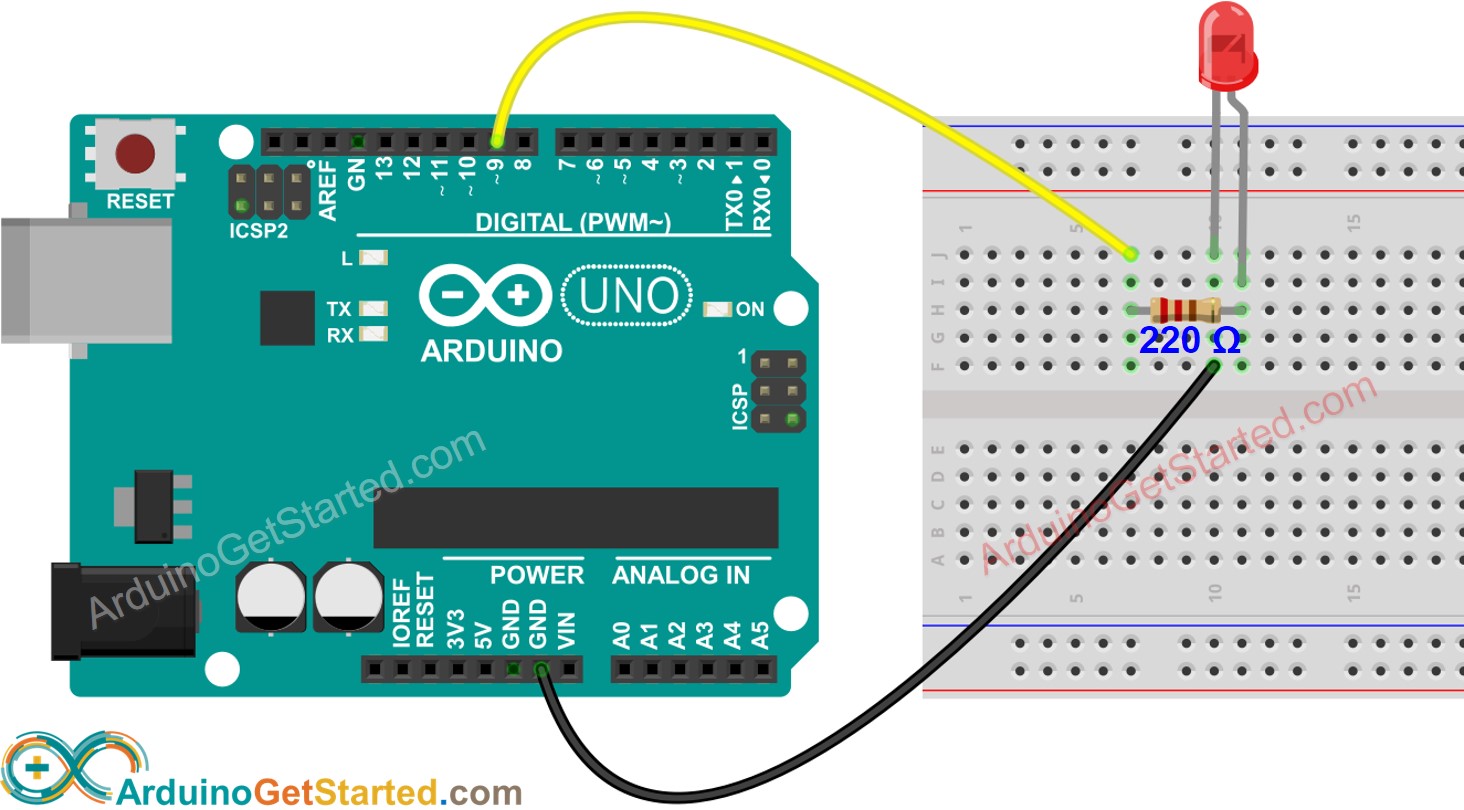
This image is created using Fritzing. Click to enlarge image
How to fade-in LED in a period without using delay()
/*
* Created by ArduinoGetStarted.com
*
* This example code is in the public domain
*
* Tutorial page: https://arduinogetstarted.com/faq/how-to-fade-led-in-a-time-period
*/
const int LED_PIN = 9; // the PWM pin the LED is attached to
unsigned long FADE_PEDIOD = 3000; // fade time is 3 seconds
unsigned long fadeStartTime;
// the setup routine runs once when you press reset
void setup() {
pinMode(LED_PIN, OUTPUT); // declare pin 9 to be an output
fadeStartTime = millis();
}
// fade-in in loop, and restart after finishing
void loop() {
unsigned long progress = millis() - fadeStartTime;
if (progress <= FADE_PEDIOD) {
long brightness = map(progress, 0, FADE_PEDIOD, 0, 255);
analogWrite(LED_PIN, brightness);
}
else {
fadeStartTime = millis(); // restart fade again
}
}
How to fade-out LED in a period without using delay()
/*
* Created by ArduinoGetStarted.com
*
* This example code is in the public domain
*
* Tutorial page: https://arduinogetstarted.com/faq/how-to-fade-led-in-a-time-period
*/
const int LED_PIN = 9; // the PWM pin the LED is attached to
unsigned long FADE_PEDIOD = 3000; // fade time is 3 seconds
unsigned long fadeStartTime;
// the setup routine runs once when you press reset
void setup() {
pinMode(LED_PIN, OUTPUT); // declare pin 9 to be an output
fadeStartTime = millis();
}
// fade-out in loop, and restart after finishing
void loop() {
unsigned long progress = millis() - fadeStartTime;
if (progress <= FADE_PEDIOD) {
long brightness = 255 - map(progress, 0, FADE_PEDIOD, 0, 255);
analogWrite(LED_PIN, brightness);
}
else {
fadeStartTime = millis(); // restart fade again
}
}
The Best Arduino Starter Kit
See Also
Function References
Buy Arduino
1 × Arduino UNO Buy on Amazon | |
1 × USB 2.0 cable type A/B Buy on Amazon | |
1 × Jumper Wires Buy on Amazon |
Please note: These are Amazon affiliate links. If you buy the components through these links, We will get a commission at no extra cost to you. We appreciate it.