How to vertical and horizontal center align text/number on OLED
How to vertical and horizontal center align text/number on OLED display?
Answer
The below code automatically vertical and horizontal center aligns text/number on OLED, regardless of text size.
/*
* Created by ArduinoGetStarted.com
*
* This example code is in the public domain
*
* Tutorial page: https://arduinogetstarted.com/faq/how-to-vertical-and-horizontal-center-align-text-number-on-oled
*/
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
Adafruit_SSD1306 oled(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1); // // create SSD1306 display object connected to I2C
void setup() {
Serial.begin(9600);
// initialize OLED display with address 0x3C for 128x64
if (!oled.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
while (true);
}
delay(2000); // wait for initializing
oled.clearDisplay(); // clear display
oled.setTextSize(2); // text size
oled.setTextColor(WHITE); // text color
oled.setCursor(0, 10); // position to display
}
void loop() {
// display string
String text = "Hi!";
oledDisplayCenter(text);
delay(2000);
// display number
int number = 21;
String str = String(number);
oledDisplayCenter(str);
delay(2000);
}
void oledDisplayCenter(String text) {
int16_t x1;
int16_t y1;
uint16_t width;
uint16_t height;
oled.getTextBounds(text, 0, 0, &x1, &y1, &width, &height);
// display on horizontal and vertical center
oled.clearDisplay(); // clear display
oled.setCursor((SCREEN_WIDTH - width) / 2, (SCREEN_HEIGHT - height) / 2);
oled.println(text); // text to display
oled.display();
}
Quick Steps
- Wiring Diagram
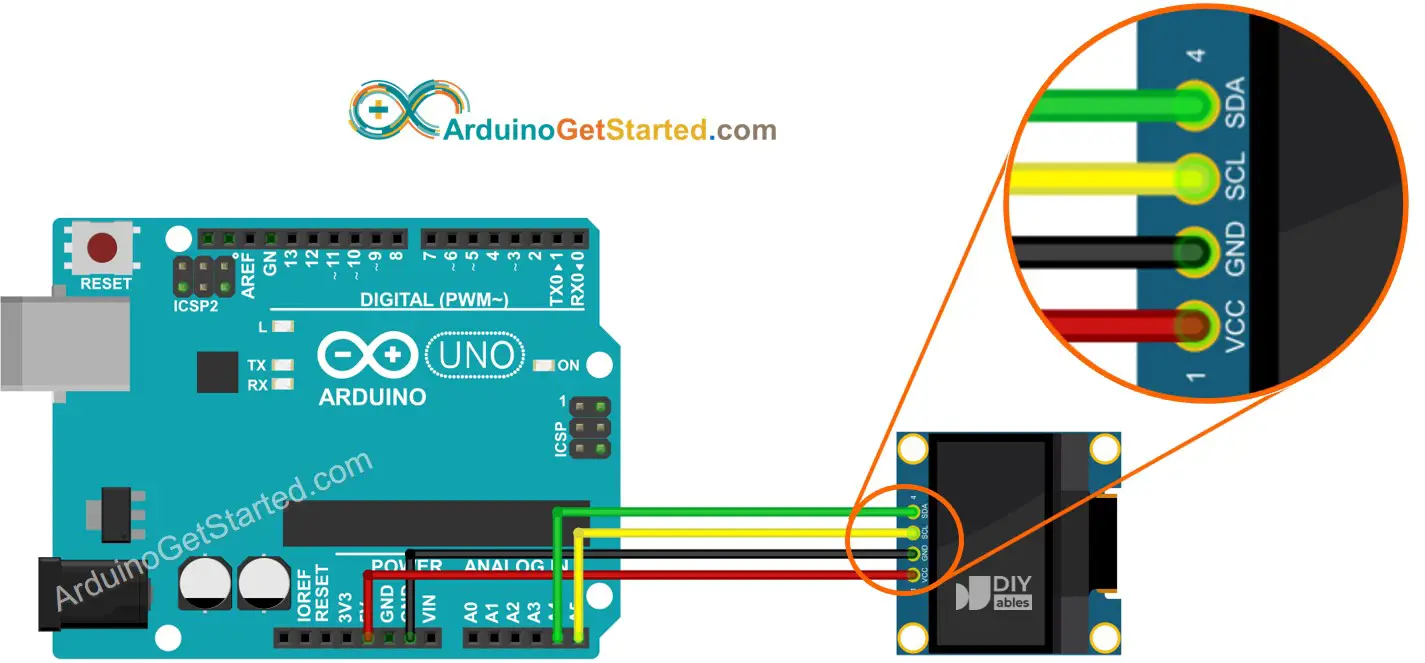
This image is created using Fritzing. Click to enlarge image
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search “SSD1306”, then find the SSD1306 library by Adafruit
- Click Install button to install the library.
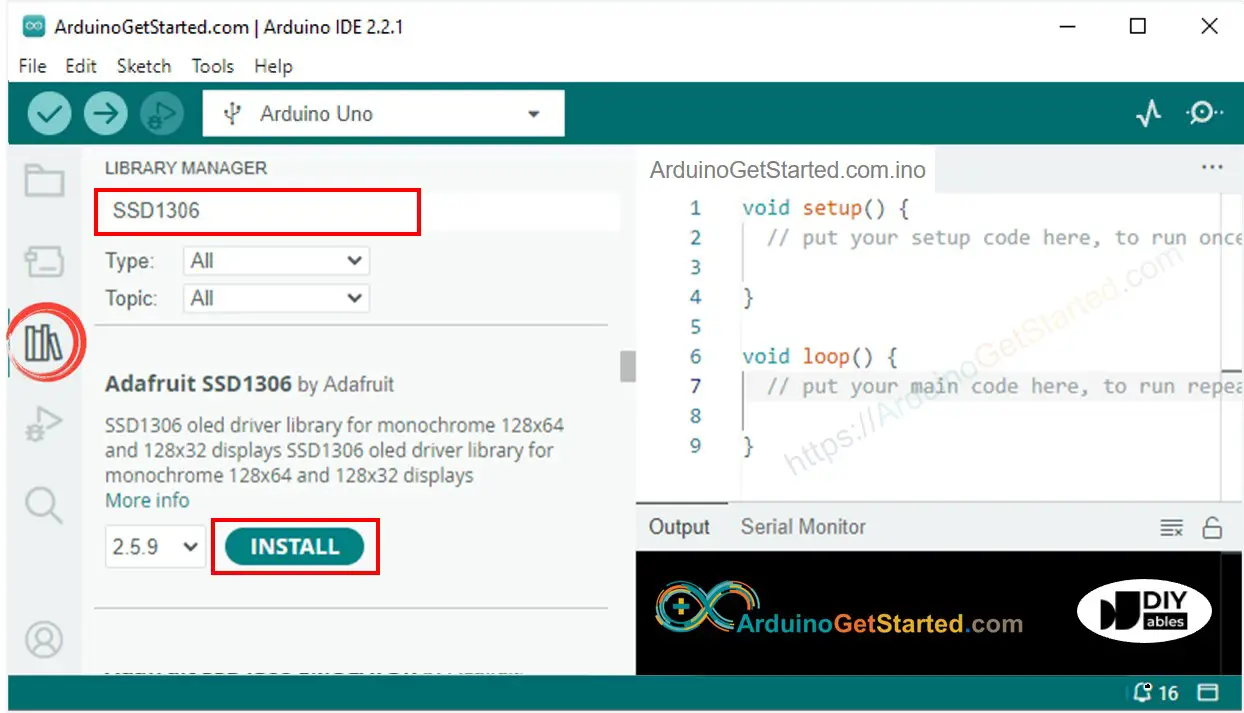
- You will be asked for intalling some other library dependencies
- Click Install All button to install all library dependencies.
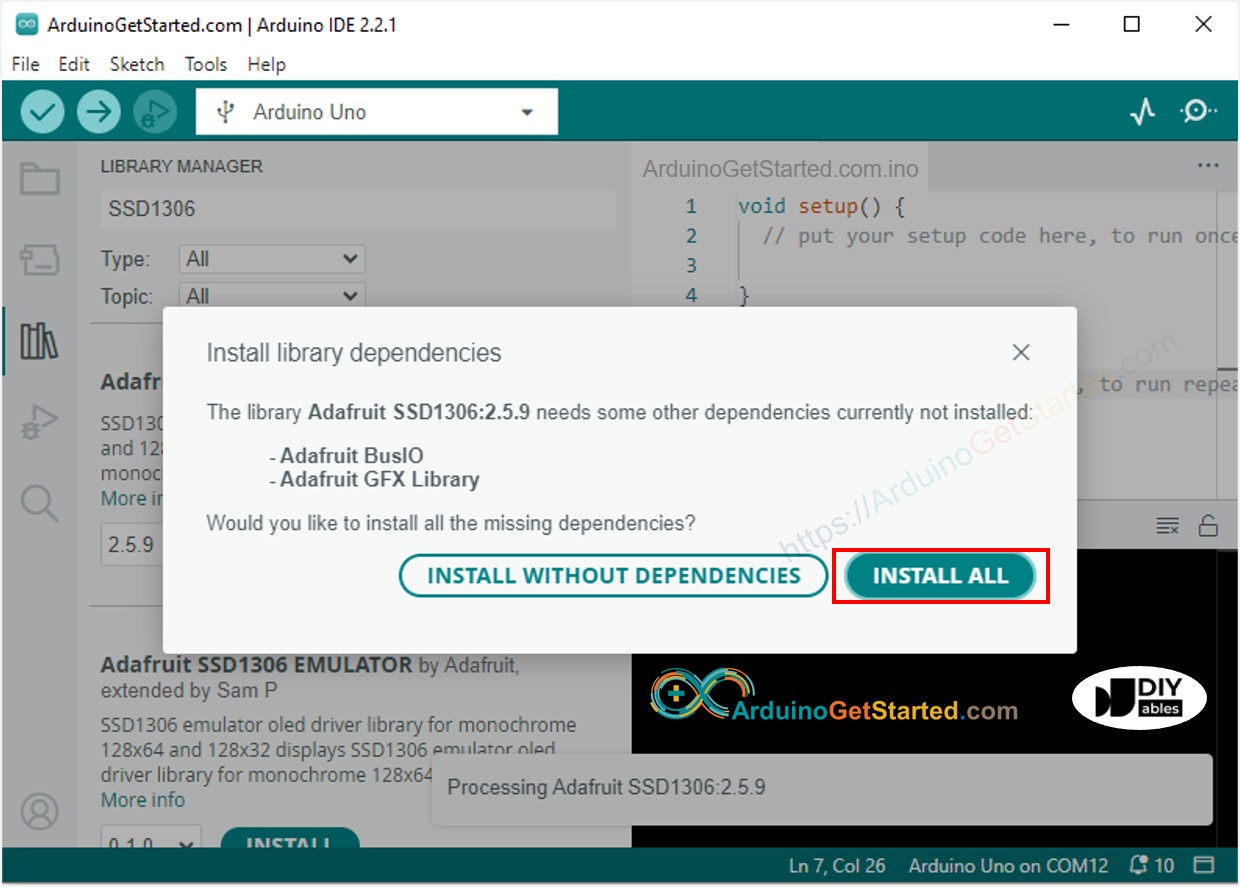
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- See text on OLED
Learn more on Arduino - OLED tutorial
Buy Arduino
1 × Arduino UNO Buy on Amazon | |
1 × USB 2.0 cable type A/B Buy on Amazon | |
1 × Jumper Wires Buy on Amazon |
Disclosure: Some links in this section are Amazon affiliate links. If you make a purchase through these links, we may earn a commission at no extra cost to you.
Additionally, some links direct to products from our own brand, DIYables .
Additionally, some links direct to products from our own brand, DIYables .