DIYables IR Controller - DIYables_IRcontroller_21.getKey()
Description
Check if there is a key pressed on 21-key IR remote controller and received by IR receiver.
Syntax
Key21 getKey();
Parameters
None
Return
- Key21::NONE: if there is no key presed on IR controller and received by IR receiver.
- One of the following values corresponding with the key on the IR remote controller:
- Key21::KEY_CH_MINUS
- Key21::KEY_CH
- Key21::KEY_CH_PLUS
- Key21::KEY_PREV
- Key21::KEY_NEXT
- Key21::KEY_PLAY_PAUSE
- Key21::KEY_VOL_MINUS
- Key21::KEY_VOL_PLUS
- Key21::KEY_EQ
- Key21::KEY_100_PLUS
- Key21::KEY_200_PLUS
- Key21::KEY_0
- Key21::KEY_1
- Key21::KEY_2
- Key21::KEY_3
- Key21::KEY_4
- Key21::KEY_5
- Key21::KEY_6
- Key21::KEY_7
- Key21::KEY_8
- Key21::KEY_9
- Key21::UNKNOWN: if it received a key that is not from the 21-key IR remote controller
Example
Hardware Required
1 | × | Official Arduino Uno | |
1 | × | Alternatively, DIYables ATMEGA328P Development Board | |
1 | × | USB 2.0 cable type A/B | |
1 | × | IR Kit (Remote Controller and Receiver) | |
1 | × | CR2025 Battery | |
1 | × | Jumper Wires |
Disclosure: Some links in this section are Amazon affiliate links. If you make a purchase through these links, we may earn a commission at no extra cost to you.
Additionally, some links direct to products from our own brand, DIYables .
Additionally, some links direct to products from our own brand, DIYables .
Wiring Diagram
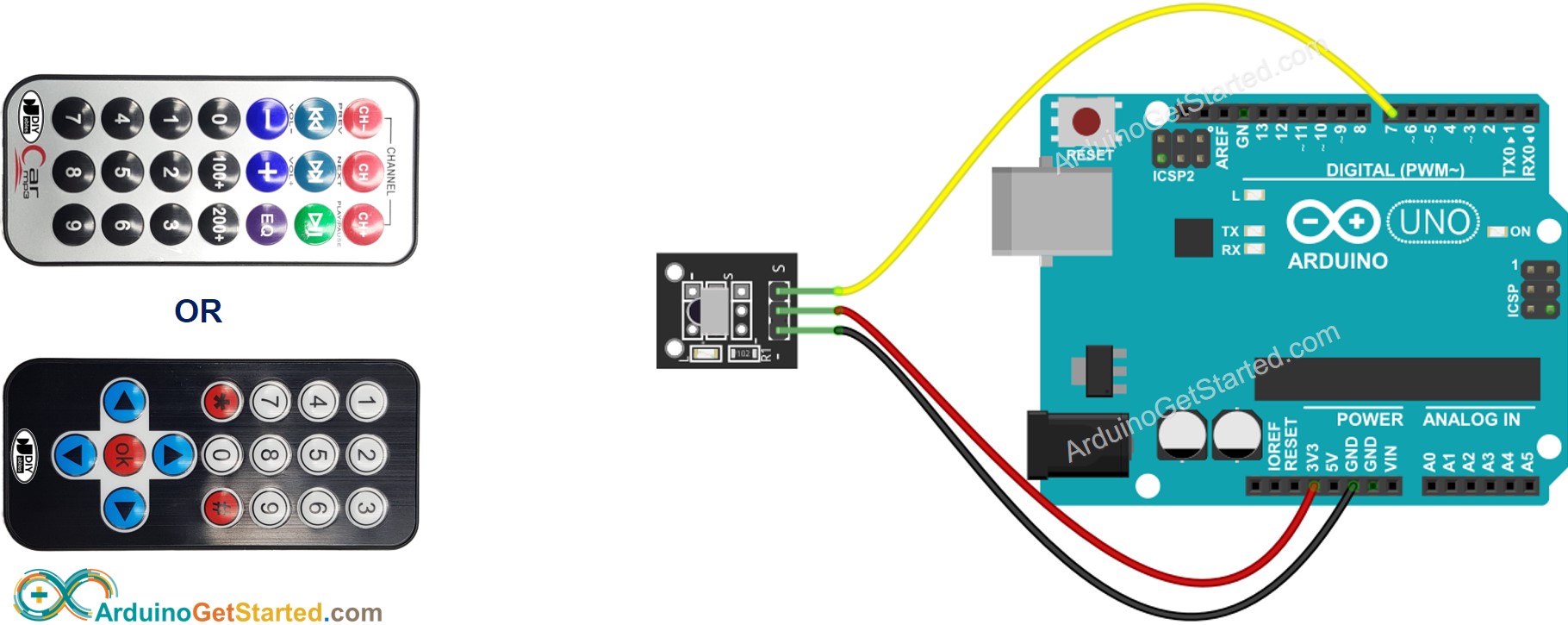
This image is created using Fritzing. Click to enlarge image
Arduino Code
/*
Created by DIYables
This example code is in the public domain
Product page: https://diyables.io/products/infrared-ir-remote-control-kit-with-21-key-controller-and-receiver
*/
#include <DIYables_IRcontroller.h> // DIYables_IRcontroller library
#define IR_RECEIVER_PIN 7 // The Arduino pin connected to IR controller
DIYables_IRcontroller_21 irController(IR_RECEIVER_PIN, 200); // debounce time is 200ms
void setup() {
Serial.begin(9600);
irController.begin();
}
void loop() {
Key21 command = irController.getKey();
if (command != Key21::NONE) {
switch (command) {
case Key21::KEY_CH_MINUS:
Serial.println("CH-");
// TODO: YOUR CONTROL
break;
case Key21::KEY_CH:
Serial.println("CH");
// TODO: YOUR CONTROL
break;
case Key21::KEY_CH_PLUS:
Serial.println("CH+");
// TODO: YOUR CONTROL
break;
case Key21::KEY_PREV:
Serial.println("<<");
// TODO: YOUR CONTROL
break;
case Key21::KEY_NEXT:
Serial.println(">>");
// TODO: YOUR CONTROL
break;
case Key21::KEY_PLAY_PAUSE:
Serial.println(">||");
// TODO: YOUR CONTROL
break;
case Key21::KEY_VOL_MINUS:
Serial.println("–");
// TODO: YOUR CONTROL
break;
case Key21::KEY_VOL_PLUS:
Serial.println("+");
// TODO: YOUR CONTROL
break;
case Key21::KEY_EQ:
Serial.println("EQ");
// TODO: YOUR CONTROL
break;
case Key21::KEY_100_PLUS:
Serial.println("100+");
// TODO: YOUR CONTROL
break;
case Key21::KEY_200_PLUS:
Serial.println("200+");
// TODO: YOUR CONTROL
break;
case Key21::KEY_0:
Serial.println("0");
// TODO: YOUR CONTROL
break;
case Key21::KEY_1:
Serial.println("1");
// TODO: YOUR CONTROL
break;
case Key21::KEY_2:
Serial.println("2");
// TODO: YOUR CONTROL
break;
case Key21::KEY_3:
Serial.println("3");
// TODO: YOUR CONTROL
break;
case Key21::KEY_4:
Serial.println("4");
// TODO: YOUR CONTROL
break;
case Key21::KEY_5:
Serial.println("5");
// TODO: YOUR CONTROL
break;
case Key21::KEY_6:
Serial.println("6");
// TODO: YOUR CONTROL
break;
case Key21::KEY_7:
Serial.println("7");
// TODO: YOUR CONTROL
break;
case Key21::KEY_8:
Serial.println("8");
// TODO: YOUR CONTROL
break;
case Key21::KEY_9:
Serial.println("9");
// TODO: YOUR CONTROL
break;
default:
Serial.println("WARNING: undefined command:");
break;
}
}
}
See Also
※ ARDUINO BUY RECOMMENDATION
Arduino UNO R3 | |
Arduino Starter Kit |