analogRead()
Description
Reads the analog value which is converted from the voltage from the specified analog pin. Arduino boards contain a multichannel, 10-bit analog to digital converter. This means that it will map input voltages between 0 and the operating voltage(5V or 3.3V) into integer values between 0 and 1023. On an Arduino UNO, for example, this yields a resolution between readings of: 5 volts / 1024 units or, 0.0049 volts (4.9 mV) per unit. See the table below for the usable pins, operating voltage and maximum resolution for some Arduino boards.
The input range can be changed using analogReference(), while the resolution can be changed (only for Zero, Due and MKR boards) using analogReadResolution().
On ATmega based boards (UNO, Nano, Mini, Mega), it takes about 100 microseconds (0.0001 s) to read an analog input, so the maximum reading rate is about 10,000 times a second.
Board | Operating voltage | Usable pins | Max resolution |
---|---|---|---|
Uno | 5 Volts | A0 to A5 | 10 bits |
Mini, Nano | 5 Volts | A0 to A7 | 10 bits |
Mega, Mega2560, MegaADK | 5 Volts | A0 to A14 | 10 bits |
Micro | 5 Volts | A0 to A11[1] | 10 bits |
Leonardo | 5 Volts | A0 to A11[1] | 10 bits |
Zero | 3.3 Volts | A0 to A5 | 12 bits[2] |
Due | 3.3 Volts | A0 to A11 | 12 bits[2] |
MKR Family boards | 3.3 Volts | A0 to A6 | 12 bits[2] |
- [1]: A0 through A5 are labelled on the board, A6 through A11 are respectively available on pins 4, 6, 8, 9, 10, and 12
- [2]: The default analogRead() resolution for these boards is 10 bits, for compatibility. You need to use analogReadResolution() to change it to 12 bits.
Syntax
analogRead(pin)
Parameter Values
- pin: the name of the analog input pin to read from (A0 to A5 on most boards, A0 to A6 on MKR boards, A0 to A7 on the Mini and Nano, A0 to A15 on the Mega).
Return Values
- The analog reading on the pin. Although it is limited to the resolution of the analog to digital converter (0-1023 for 10 bits or 0-4095 for 12 bits). Data type: int.
Example Code
The code reads the voltage on analogPin and prints the read value to Serial Monitor.
Hardware Required
Wiring Diagram
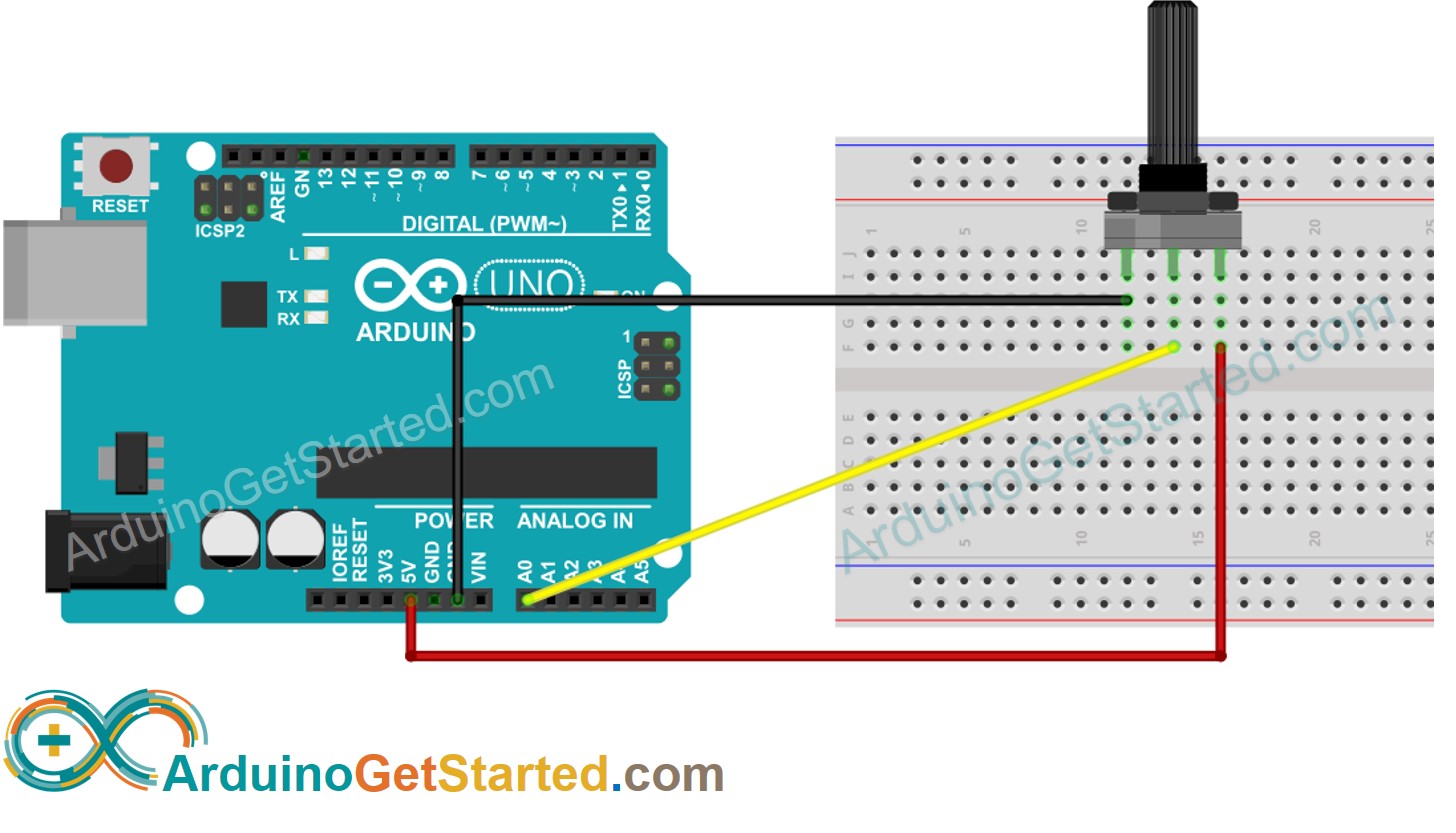
This image is created using Fritzing. Click to enlarge image
Arduino Code
Rotate the potentiometer and see the result on Serial Monitor
For more detailed instruction, see Arduino - Potentiometer
※ NOTES AND WARNINGS:
- If the analog input pin is not connected to anything, the value returned by analogRead() will fluctuate based on a number of factors (e.g. the values of the other analog inputs, how close your hand is to the board, etc.).
- The analogRead() and analogWrite() functions do NOT read and write the same thing. analogRead() function reads the analog value which is converted from the voltage. analogWrite() function writes PWM signal. If you use the analogWrite() function first, and then use analogRead() function to read the value on the same pin, the read value is diferent from the wrote value. In other word, analogRead() function uses ADC (Analog to Digital) converter, but analogWrite() function does NOT use DAC (Digital to Analog) converter.
- The analog pins are set as input by default. It is not necessary to configure them before calling the analogRead() function.
See Also
- Language : analogReference()
- Language : analogWrite()
- Example : Arduino - Potentiometer
- Example : Arduino - Light Sensor
- Example : Arduino - Water Sensor
- Language : analogReadResolution()
- Example : Description of the analog input pins
※ ARDUINO BUY RECOMMENDATION
Arduino UNO R3 | |
Arduino Starter Kit |