tone()
Description
Generates a square wave of the specified frequency (and 50% duty cycle) on a pin. A duration can be specified, otherwise the wave continues until a call to noTone(). The pin can be connected to a piezo buzzer or other speaker to play tones.
Only one tone can be generated at a time. If a tone is already playing on a different pin, the call to tone() will have no effect. If the tone is playing on the same pin, the call will set its frequency.
Use of the tone() function will interfere with PWM output on pins 3 and 11 (on boards other than the Mega).
It is not possible to generate tones lower than 31Hz. For technical details, see Brett Hagman's notes.
Syntax
Parameter Values
- pin: the Arduino pin on which to generate the tone.
- frequency: the frequency of the tone in hertz. Allowed data types: unsigned int.
- duration: the duration of the tone in milliseconds (optional). Allowed data types: unsigned long.
Return Values
- Nothing
Example
Let's to play "Jingle Bells" song with Arduino.
Hardware Required
Wiring Diagram
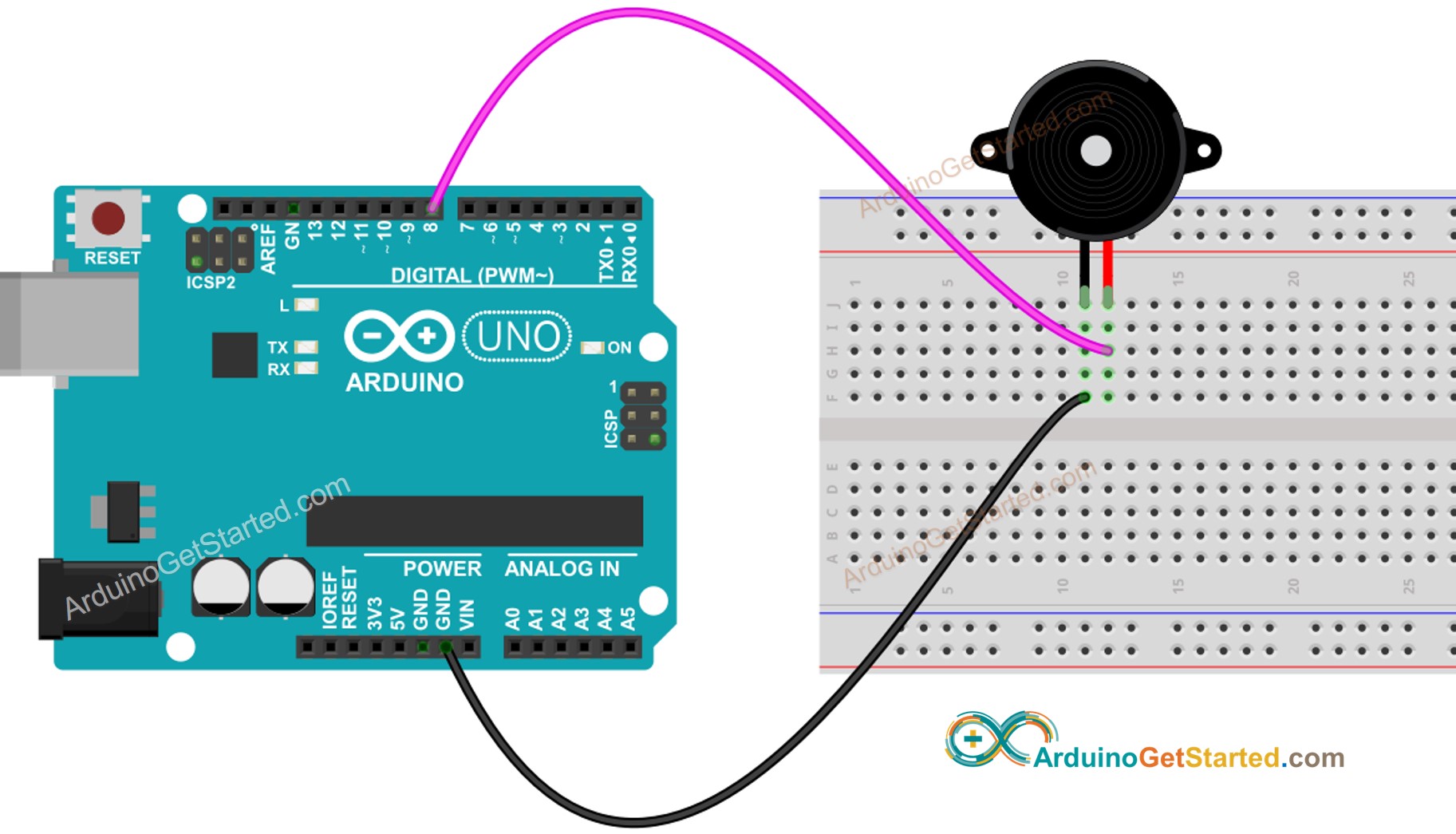
This image is created using Fritzing. Click to enlarge image
Arduino Code
For more detailed instruction, see Arduino - Piezo Buzzer
※ NOTES AND WARNINGS:
If you want to play different pitches on multiple pins, you need to call noTone() on one pin before calling tone() on the next pin.
See Also
- Language : noTone()
- Language : pulseIn()
- Language : pulseInLong()
- Language : shiftIn()
- Language : shiftOut()
- Example : Arduino - Piezo Buzzer
※ ARDUINO BUY RECOMMENDATION
Arduino UNO R3 | |
Arduino Starter Kit |