Arduino - Button - Debounce
When a button is pressed/released or when a switch is toggled, newbies usually think simply that its state is changed from LOW to HIGH or HIGH to LOW. In practice, it is not exactly like that. Because of the mechanical and physical characteristics, the state of the button (or switch) might be toggled between LOW and HIGH several times. This phenomenon is called chattering. The chattering phenomenon makes a single press that may be read as multiple presses, resulting in a malfunction in some kinds of applications. This tutorial shows how to eliminate this phenomenon (called debounce the input).
Or you can buy the following sensor kits:
Please note: These are Amazon affiliate links. If you buy the components through these links, We will get a commission at no extra cost to you. We appreciate it.
If you do not know about button (pinout, how it works, how to program ...), learn about them in the following tutorials:
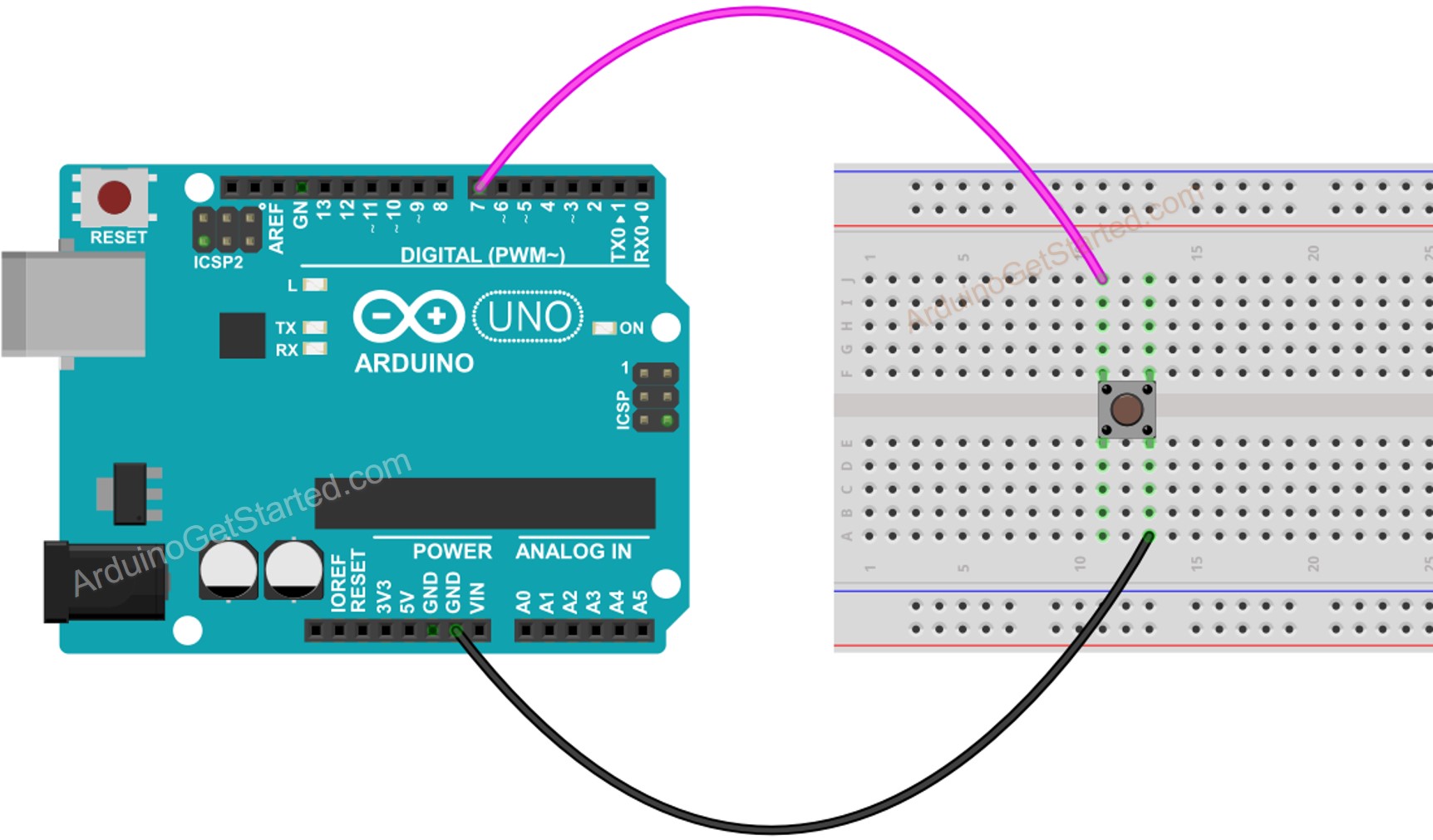
This image is created using Fritzing. Click to enlarge image
Let's see and compare Arduino code between WITHOUT and WITH debounce and their behaviors.
Before learning about debouncing, just see the code without debouncing and its behavior.
Connect Arduino to PC via USB cable
Open Arduino IDE, select the right board and port
Copy the below code and open with Arduino IDE
const int BUTTON_PIN = 7;
int lastState = LOW;
int currentState;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
currentState = digitalRead(BUTTON_PIN);
if(lastState == HIGH && currentState == LOW)
Serial.println("The button is pressed");
else if(lastState == LOW && currentState == HIGH)
Serial.println("The button is released");
lastState = currentState;
}
The button is pressed
The button is pressed
The button is pressed
The button is released
The button is released
⇒ As you can see, you pressed and released the button just the once. However, Arduino recognizes it as multiple presses and releases.
const int BUTTON_PIN = 7;
const int DEBOUNCE_DELAY = 50;
int lastSteadyState = LOW;
int lastFlickerableState = LOW;
int currentState;
unsigned long lastDebounceTime = 0;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
currentState = digitalRead(BUTTON_PIN);
if (currentState != lastFlickerableState) {
lastDebounceTime = millis();
lastFlickerableState = currentState;
}
if ((millis() - lastDebounceTime) > DEBOUNCE_DELAY) {
if (lastSteadyState == HIGH && currentState == LOW)
Serial.println("The button is pressed");
else if (lastSteadyState == LOW && currentState == HIGH)
Serial.println("The button is released");
lastSteadyState = currentState;
}
}
Click Upload button on Arduino IDE to upload code to Arduino
Open Serial Monitor
Keep pressing the button several seconds and then release it.
See the result on Serial Monitor
The button is pressed
The button is released
⇒ As you can see, you pressed and released the button just the once. Arduino recognizes it as the single press and release. The chatter is eliminated.
To make it much easier for beginners, especially when using multiple buttons, we created a library, called ezButton. You can learn about ezButton library here.
#include <ezButton.h>
ezButton button(7);
void setup() {
Serial.begin(9600);
button.setDebounceTime(50);
}
void loop() {
button.loop();
if(button.isPressed())
Serial.println("The button is pressed");
if(button.isReleased())
Serial.println("The button is released");
}
#include <ezButton.h>
ezButton button1(6);
ezButton button2(7);
ezButton button3(8);
void setup() {
Serial.begin(9600);
button1.setDebounceTime(50);
button2.setDebounceTime(50);
button3.setDebounceTime(50);
}
void loop() {
button1.loop();
button2.loop();
button3.loop();
if(button1.isPressed())
Serial.println("The button 1 is pressed");
if(button1.isReleased())
Serial.println("The button 1 is released");
if(button2.isPressed())
Serial.println("The button 2 is pressed");
if(button2.isReleased())
Serial.println("The button 2 is released");
if(button3.isPressed())
Serial.println("The button 3 is pressed");
if(button3.isReleased())
Serial.println("The button 3 is released");
}
The wiring diagram for above code:
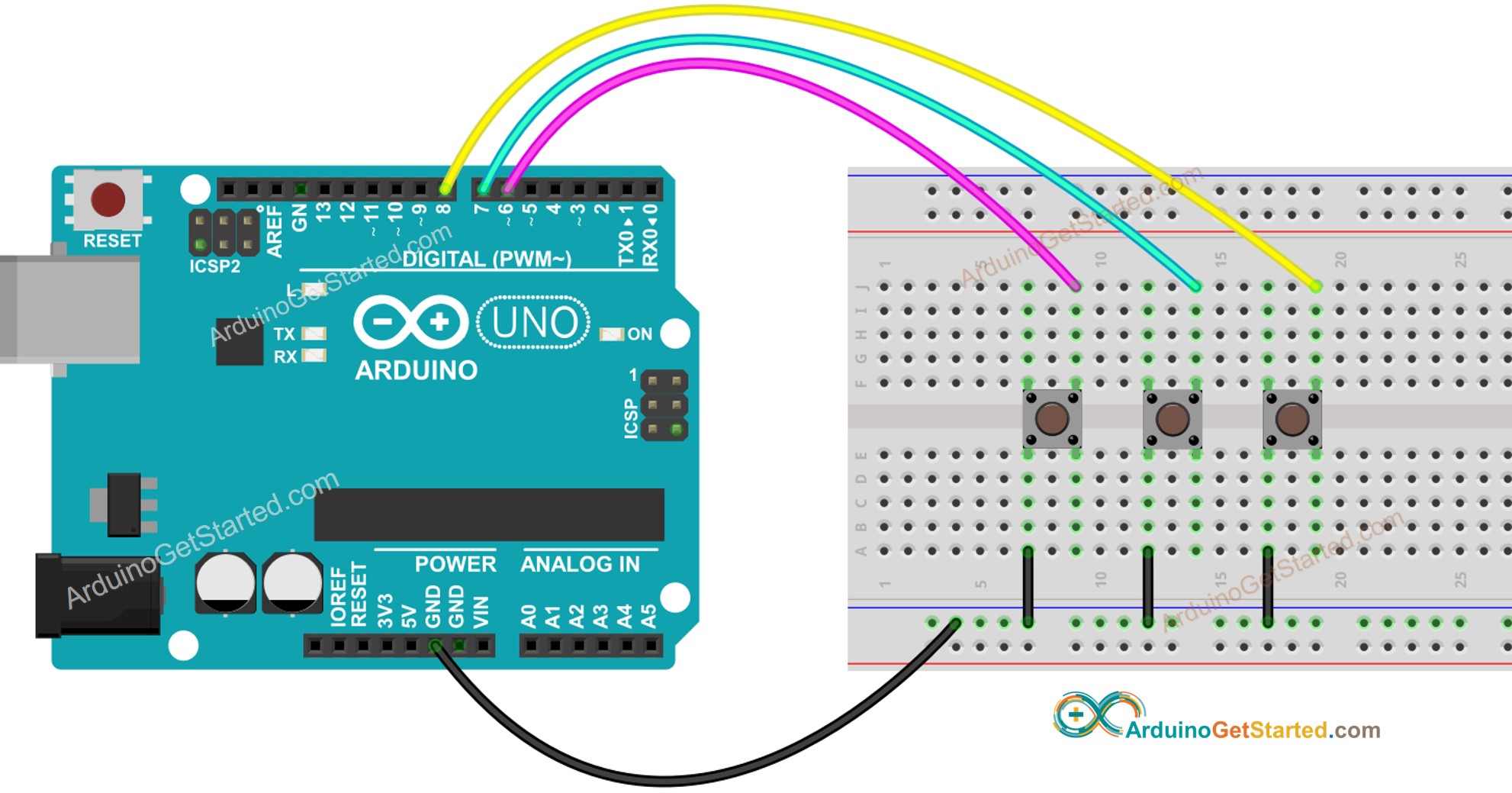
This image is created using Fritzing. Click to enlarge image
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.
The debounce method can apply for switch, touch sensor ...
Most electronic products have a reset button. Additionally, the button also keeps other functionalities in many products.
※ OUR MESSAGES
You can share the link of this tutorial anywhere. Howerver, please do not copy the content to share on other websites. We took a lot of time and effort to create the content of this tutorial, please respect our work!