Arduino - LED Library
This library is designed for Arduino, ESP32, ESP8266... to control LED: on, off, toggle, fade in/out, blink, blink the number of times, blink in a period of time. It is designed for not only beginners but also experienced users.
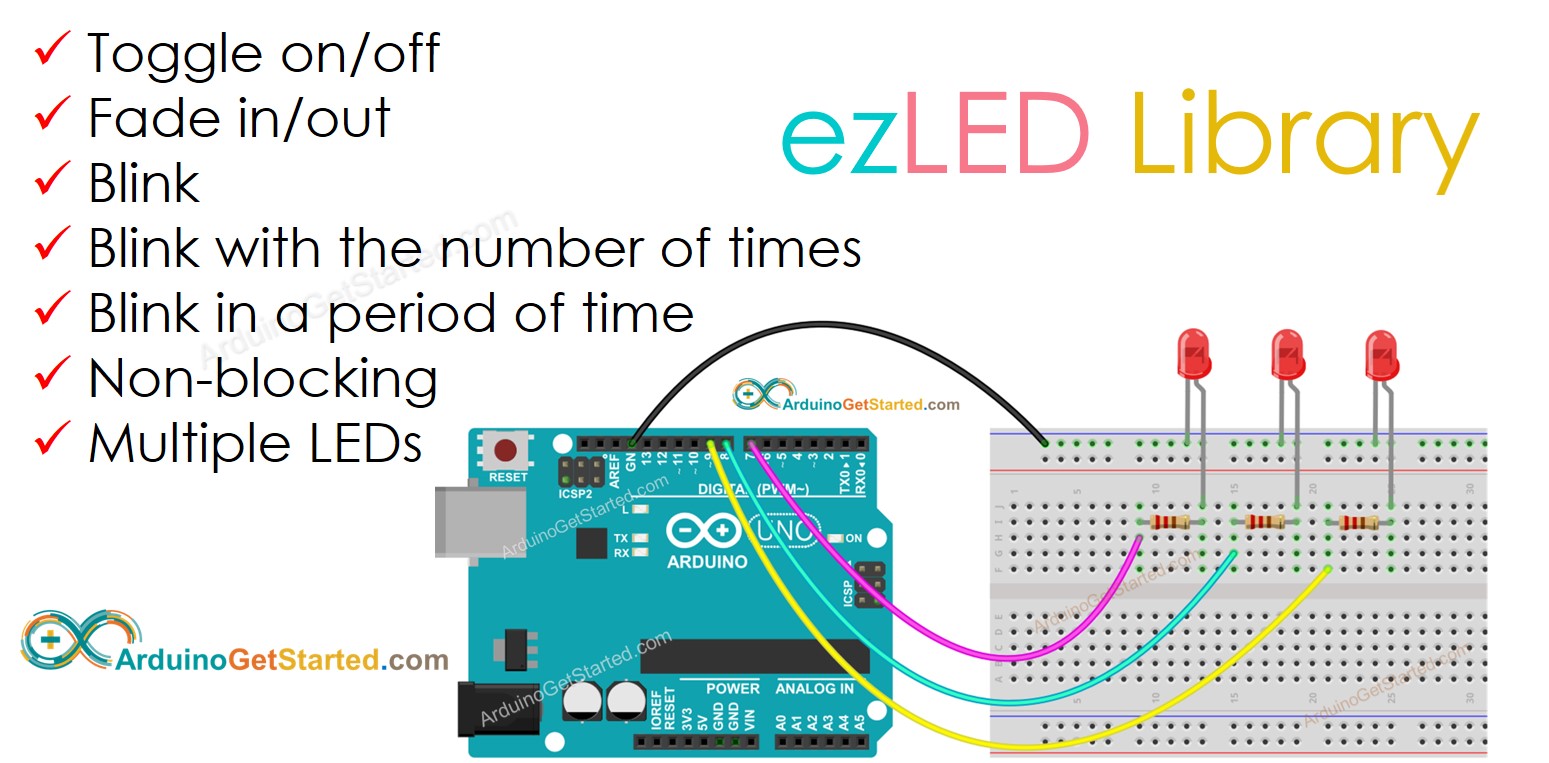
Library Features
- Turn on/off
- Toggle between on and off
- Fade in/out
- Blink
- Blink with the number of times
- Blink in a period of time
- Cancel the blinking or fading anytime
- Support both control modes: CTRL_ANODE and CTRL_CATHODE
- Get the on/off LED's states: LED_OFF, LED_ON
- Get the operation LED's state: LED_IDLE, LED_DELAY, LED_FADING, LED_BLINKING
- All functions are non-blocking (without using delay() function)
- Easy to use with multiple LEDs
Available Functions
- ezLED(int pin)
- ezLED(int pin, int mode)
- void turnON()
- void turnON(unsigned long delayTime)
- void turnOFF()
- void turnOFF(unsigned long delayTime)
- void toggle()
- void toggle(unsigned long delayTime)
- void fade(int fadeFrom, int fadeTo, unsigned long fadeTime)
- void fade(int fadeFrom, int fadeTo, unsigned long fadeTime, unsigned long delayTime)
- void blink(unsigned long onTime, unsigned long offTime)
- void blink(unsigned long onTime, unsigned long offTime, unsigned long delayTime)
- void blinkInPeriod(unsigned long onTime, unsigned long offTime, unsigned long blinkTime)
- void blinkInPeriod(unsigned long onTime, unsigned long offTime, unsigned long blinkTime, unsigned long delayTime)
- void blinkNumberOfTimes(unsigned long onTime, unsigned long offTime, unsigned int numberOfTimes)
- void blinkNumberOfTimes(unsigned long onTime, unsigned long offTime, unsigned int numberOfTimes, unsigned long delayTime)
- void cancel(void)
- int getOnOff(void)
- int getState(void)
- void loop(void)
Available Examples
※ NOTE THAT:
If this library is useful for your work, you can say thanks to us by buying us a coffee: PayPal.Me/arduinogetstarted . We appreciate it.
How To Install Library
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search “ezLED”, then find the led library by ArduinoGetStarted
- Click Install button to install ezLED library.
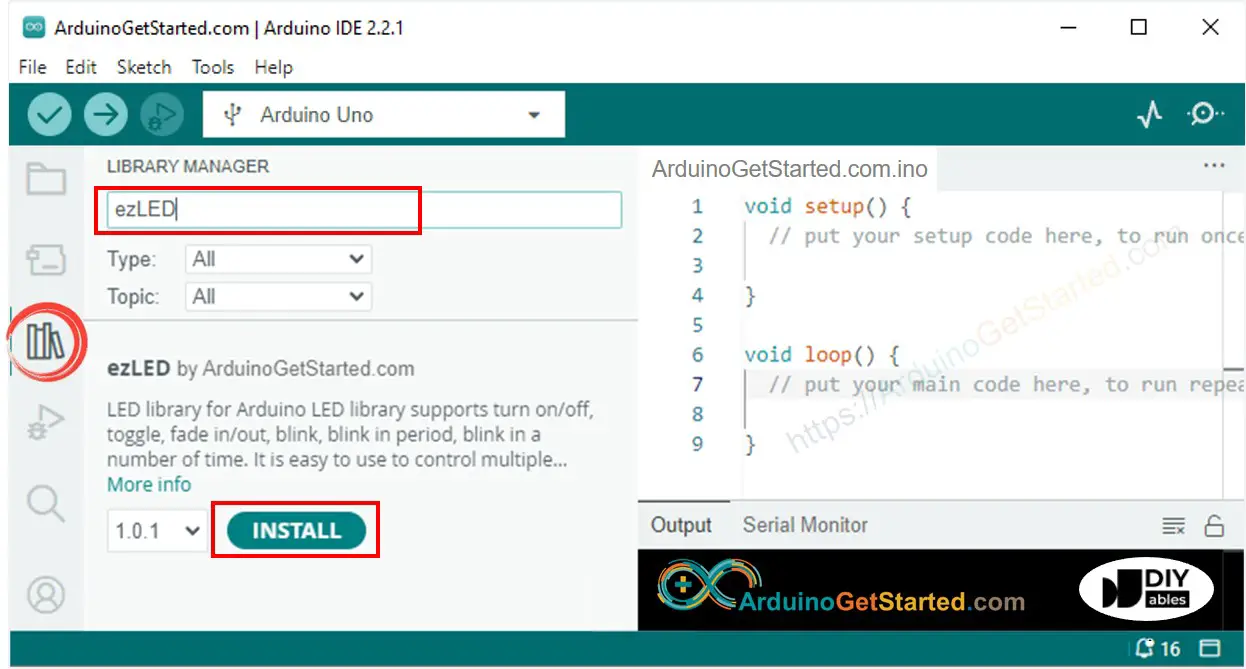
Or you can download it on Github
References
ezLED()
Description
This function creates a new instance of the ezLED class that represents a particular led pin of your Arduino board.
Syntax
Parameters
- pin: Arduino's pin that connects to LED.
- mode: (Optional)The controlling mode. There are two mode:
- CTRL_ANODE: The LED's Anode is connected to Arduino Pin, The LED's Cathode is connected to GND. This is default mode if mode is not set
- CTRL_CATHODE: The LED's Cathode is connected to Arduino Pin, The LED's Anode is connected to VCC.
- delayTime: (Optional) The time (in ms) delay before turning LED ON. If not set, LED is turned ON immediately. This function is non-blocking even if the delayTime is set.
- delayTime: (Optional) The time (in ms) delay before turning LED OFF. If not set, LED is turned OFF immediately. This function is non-blocking even if the delayTime is set.
- delayTime: (Optional) The time (in ms) delay before toggling LED . If not set, LED is toggled immediately. This function is non-blocking even if the delayTime is set.
- fadeFrom: the value that LED start to fade (0 to 255).
- fadeTo: the value that LED fade to (0 to 255).
- fadeTime: The duration (in ms) that LED fades.
- delayTime: (Optional) The time (in ms) delay before fading LED . If not set, LED is faded immediately. This function is non-blocking even if the delayTime is set.
- The fading can be canceled immediately by calling led.cancel() function
- To check if the fading is finished or not, call led.getState() function. If the state is LED_IDLE, the fading is finished
- onTime: the time (in ms) LED is on in a blink cycle.
- offTime: the time (in ms) LED is on in a blink cycle.
- delayTime: (Optional) The time (in ms) delay before blinking LED . If not set, LED is blinked immediately. This function is non-blocking even if the delayTime is set.
- onTime: the time (in ms) LED is on in a blink cycle.
- offTime: the time (in ms) LED is on in a blink cycle.
- blinkTime: the time period (in ms) that LED is blinking. After this time is passed, the blinking is stopped and LED is set to OFF
- delayTime: (Optional) The time (in ms) delay before blinking LED . If not set, LED is blinked immediately. This function is non-blocking even if the delayTime is set.
- The blinking can be canceled immediately by calling led.cancel() function
- To check if the blinking is finished or not, call led.getState() function. If the state is LED_IDLE, the blinking is finished
- onTime: the time (in ms) LED is on in a blink cycle.
- offTime: the time (in ms) LED is on in a blink cycle.
- blinkTime: the time period (in ms) that LED is blinking. After this time is passed, the blinking is stopped and LED is set to OFF
- delayTime: (Optional) The time (in ms) delay before blinking LED . If not set, LED is blinked immediately. This function is non-blocking even if the delayTime is set.
- The blinking can be canceled immediately by calling led.cancel() function
- To check if the blinking is finished or not, call led.getState() function. If the state is LED_IDLE, the blinking is finished
- LED_OFF: If the LED is off
- LED_ON: If the LED is on
- LED_IDLE: If the LED is not in any operation. The LED can be ON or OFF in this operation state
- LED_DELAY: If the LED is in delay time
- LED_FADING: If the LED is fading
- LED_BLINKING: If the LED is blinking
Return
A new instance of the ezLED class.
Example
turnON()
Description
This function turns LED on.
Syntax
Parameters
Return
None
Example
turnOFF()
Description
This function turns LED OFF.
Syntax
Parameters
Return
None
Example
toggle()
Description
This function toggles the state of LED. If the currect state is ON, turn LED off, and vice versa.
Syntax
Parameters
Return
None
Example
fade()
Description
This function fades in/out LED.
Syntax
Parameters
If fadeFrom < fadeTo, LED is faded in
If fadeFrom > fadeTo, LED is faded out
Return
None
Example
See LED Fade In Fade Out example
※ NOTE THAT:
blink()
Description
This function blinks LED forever. The blinking can be canceled immediately by calling led.cancel() function
Syntax
Parameters
Return
None
Example
See LED Blink example
blinkInPeriod()
Description
This function blinks LED in a period of time.
Syntax
Parameters
Return
None
Example
See LED Blink In Period example
※ NOTE THAT:
blinkNumberOfTimes()
Description
This function blinks LED in a number of times.
Syntax
Parameters
Return
None
Example
See LED Blink Number Of Times example
※ NOTE THAT:
low()
Description
This function cancels any ongoing operation and set LED to OFF.
Syntax
Parameters
None
Return
None
Example
getOnOff()
Description
This function returns the current ON/OFF state of LED.
Syntax
Parameters
None
Return
Example
See LED On Off example.
getState()
Description
This function returns the current operation state of LED.
Syntax
Parameters
None
Return
Example
See LED Blink Number Of Times example.
loop()
loop()
Description
This function does tasks such as checking delay, fading, and blinking on the background. Call this function as many as possible.
Syntax
Parameters
None
Return
None
Example
See: