Arduino Fire Alarm
In this tutorial, we are going to learn how to make a fire alarm system that detects the fire and make alarm via siren and light.
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
About Fire Alarm System
The fire alarm system includes two parts:
- The fire detection: can use the smoke sensor (MQ2 gas sensor) and/or flame sensor
In the fire detection, you can use one of two sensors. However, to increase the reliability, we recommend to use both the smoke sensor and flame sensor. If you use one sensor, the system may not detect the fire in some location in your momitoring area.
If you do not know about mq2 gas sensor and flame sensor (pinout, how it works, how to program ...), learn about them in the following tutorials:
- Arduino - MQ2 Gas Sensor tutorial
- Arduino - Flame Sensor tutorial
How Fire Alarm System Works
Arduino reads the states from the smoke sensor and flame sensor, if either smoke or flame is detected, Arduino activate the relay to trigger an alarm.
Wiring Diagram
- Wiring diagram with a siren
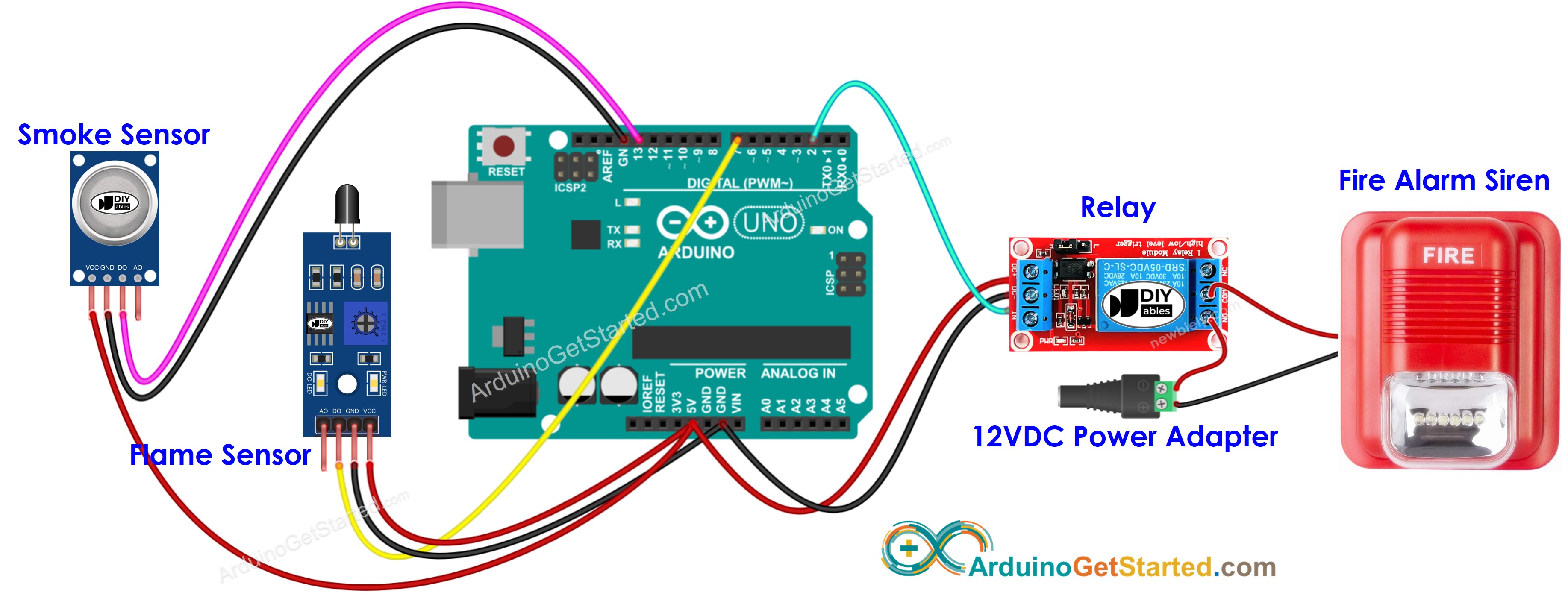
This image is created using Fritzing. Click to enlarge image
- Wiring diagram with a 12V buzzer
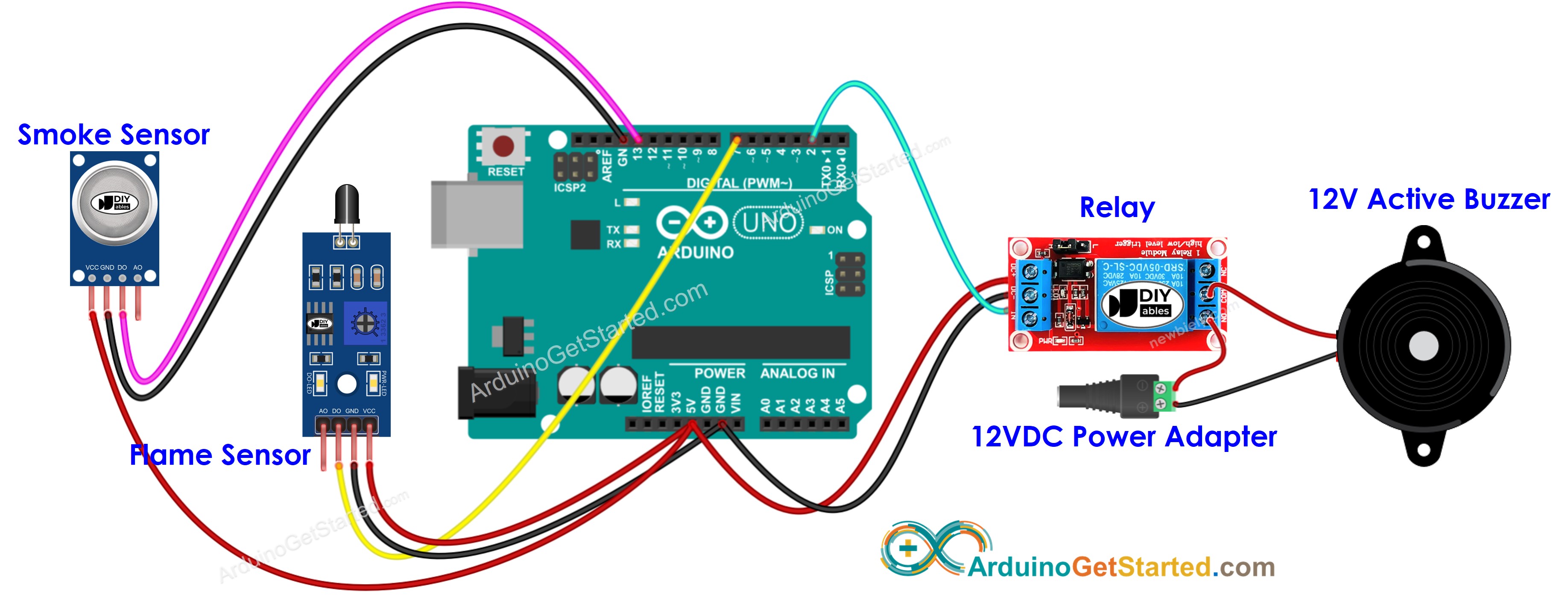
This image is created using Fritzing. Click to enlarge image
Arduino Code
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Open Serial Monitor on Arduino IDE
- Upload the code to Arduino
- Create some smoke around the smoke sensor
- Create a flame in front of the flame sensor
- Listen to sound alarm from the sire or buzzer
- See the result on Serial Monitor.
Code Explanation
Read the line-by-line explanation in the comment lines of the source code!
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.