Arduino control Servo Motor via Bluetooth
In this tutorial, we will learn how to program an Arduino to control a Servo Motor using either Bluetooth (HC-05 module) or BLE (HM-10 module). Instructions for both modules are provided.
We will use the Bluetooth Serial Monitor App on smartphone to send the angle value to Arduino. Arduino will control the servo motor according to the received value.
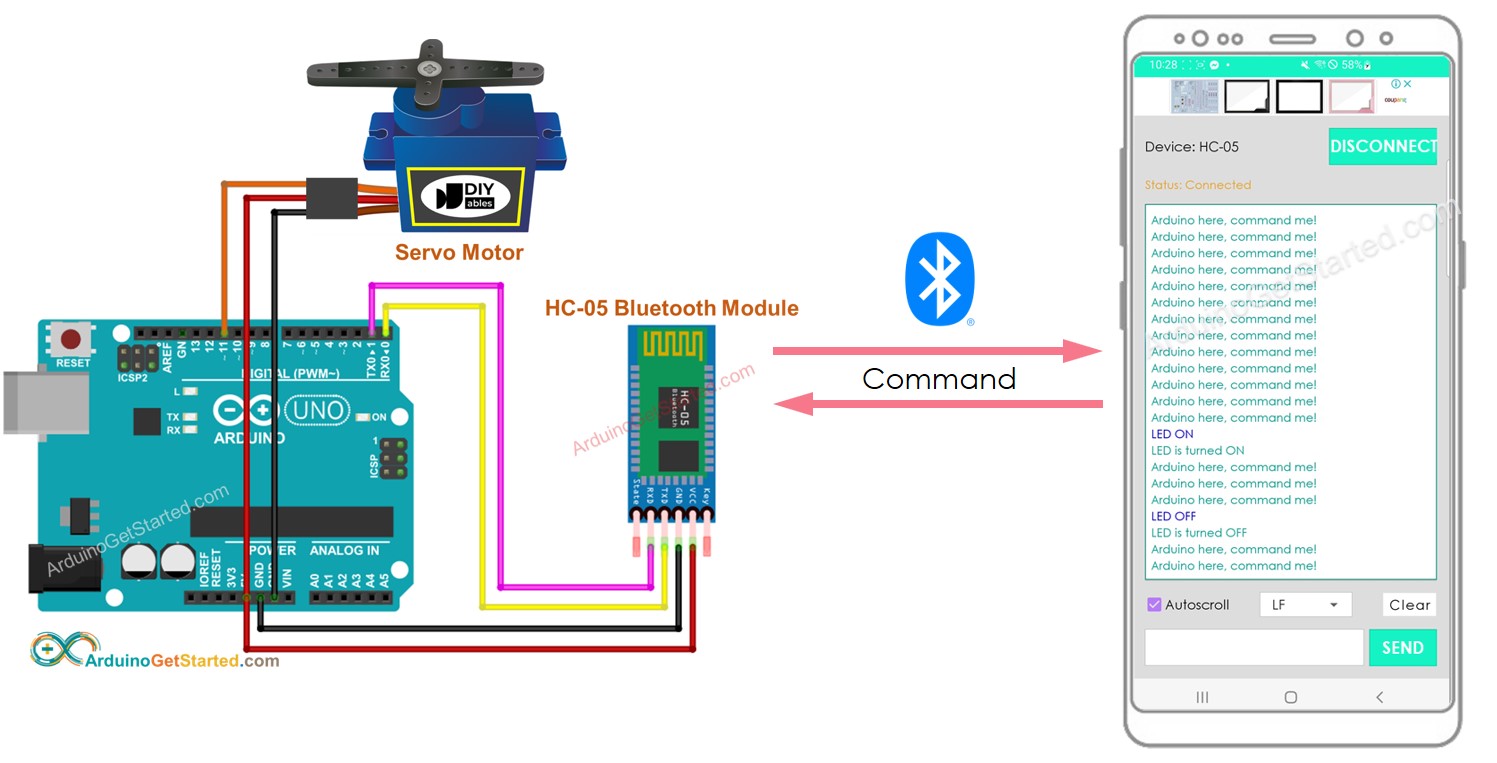
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
About Servo Motor and Bluetooth Module
If you are unfamiliar with Servo Motors and Bluetooth Modules, including their pinouts, functionality, and programming, please refer to the following tutorials for more information:
Wiring Diagram
- To control a Servo Motor using Classic Bluetooth, use the HC-05 Bluetooth module, and refer to the wiring diagram provided below.
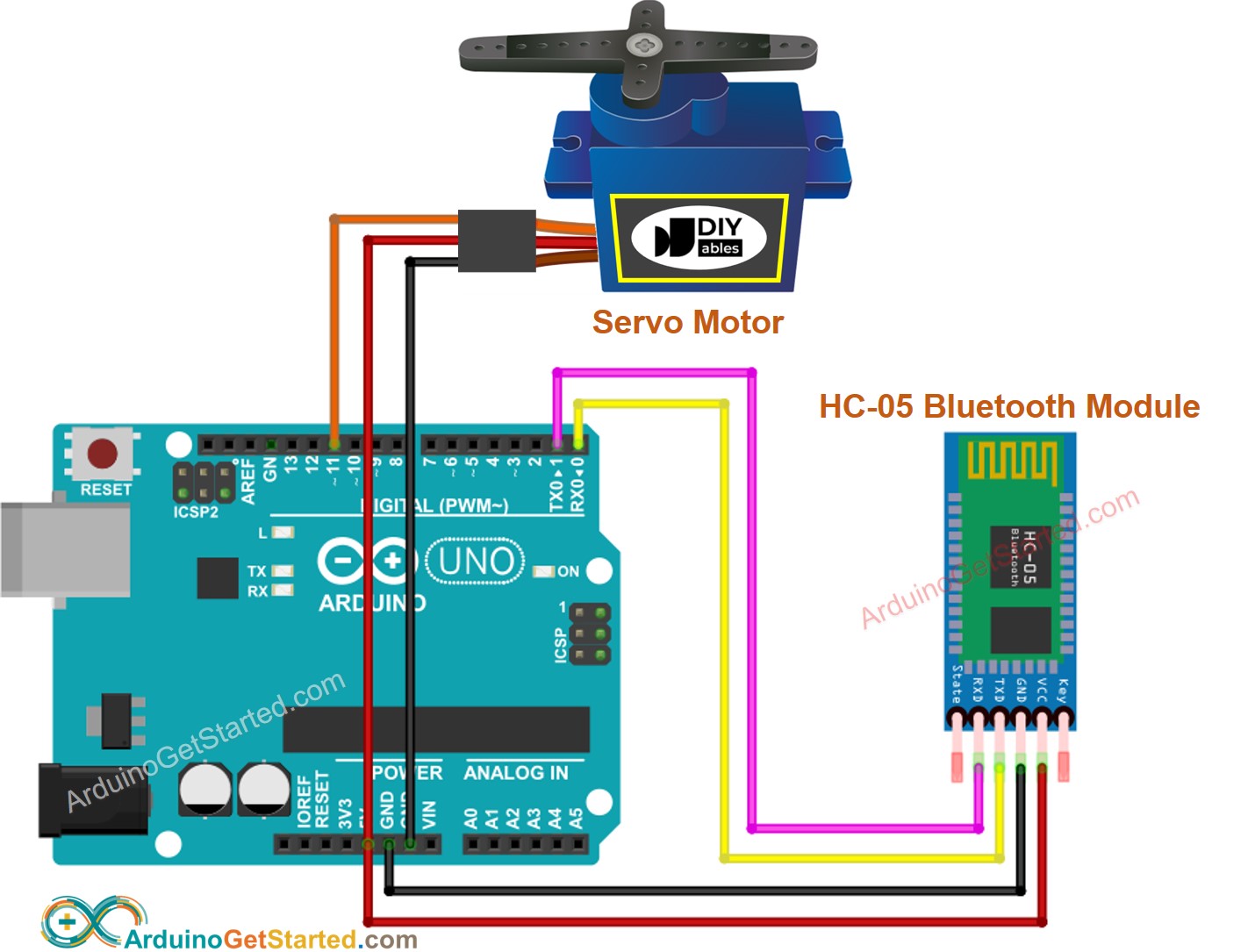
This image is created using Fritzing. Click to enlarge image
- To control a Servo Motor using BLE, use the HM-10 BLE module, and refer to the wiring diagram provided below.
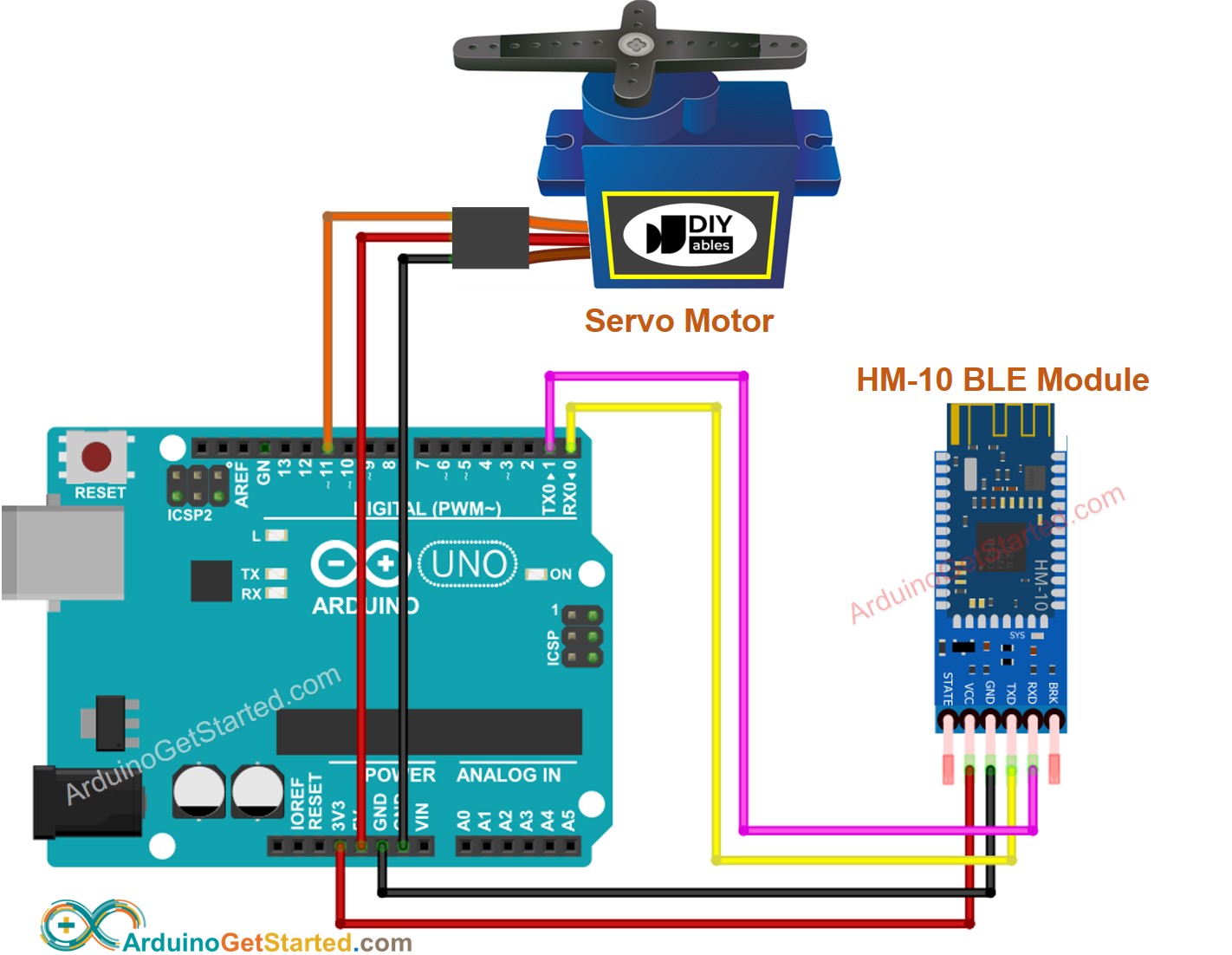
This image is created using Fritzing. Click to enlarge image
Arduino Code - controls Servo Motor via Bluetooth/BLE
The code provided below is compatible with both the HC-10 Bluetooth module and the HM-10 BLE module.
Quick Steps
- Install Bluetooth Serial Monitor App on your smartphone
- Copy the provided code and open it with the Arduino IDE, then upload it to your Arduino board.
- If you have trouble uploading the code, try disconnecting the TX and RX pins from the Bluetooth module, uploading the code, and then reconnecting the RX/TX pins again.
- Open the Bluetooth Serial Monitor App on your smartphone and select the Classic Bluetooth or BLE option, depending on the module you are using.
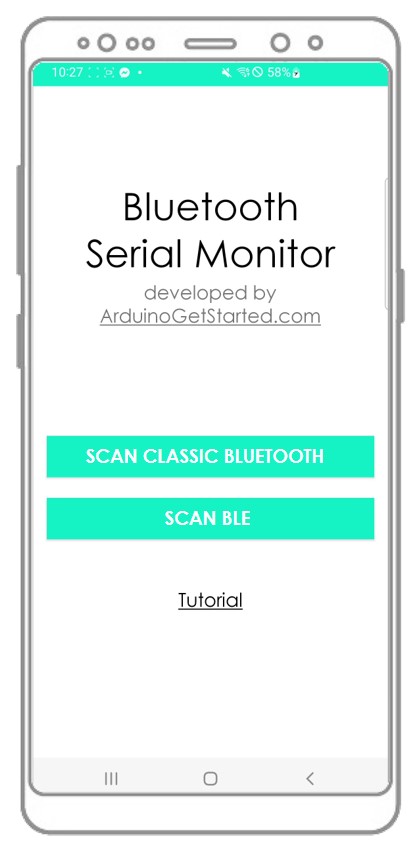
- Pair the app with the HC-05 Bluetooth module or HM-10 BLE module.
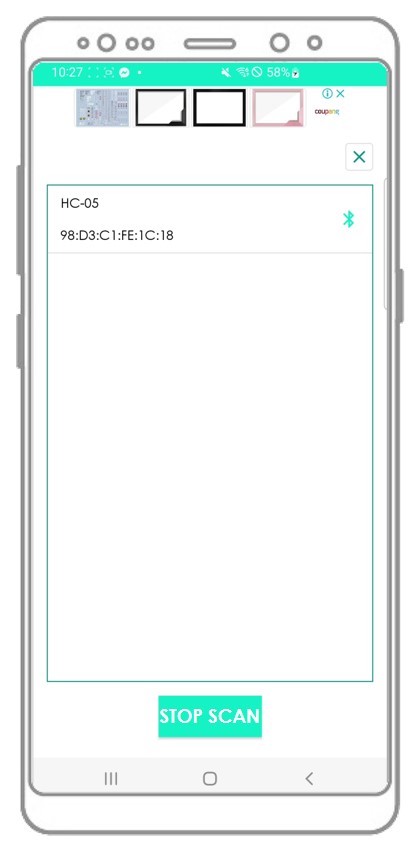
- Type in an angle value (e.g. 45 or 90) and click the Send button.
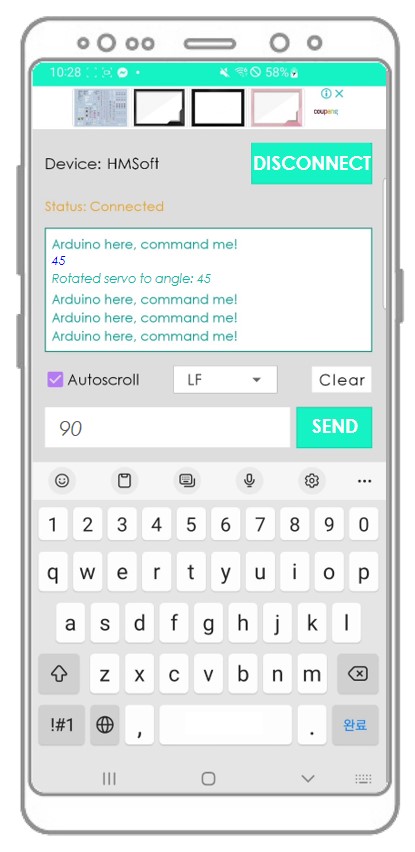
- You should see the Servo Motor's angle change.
- Observe the results on the Android App.
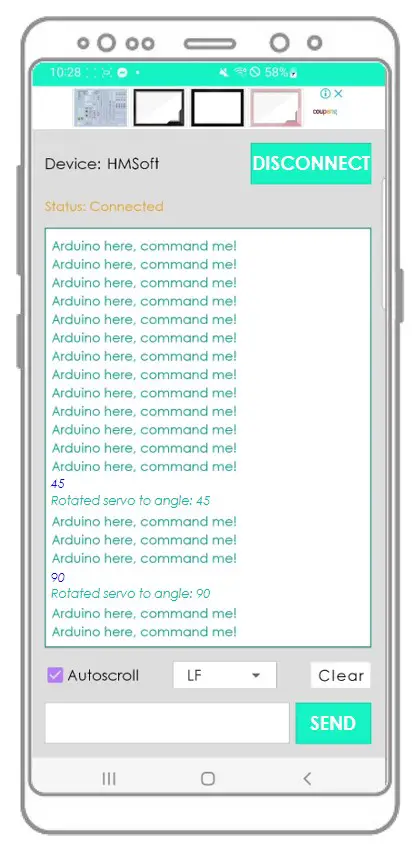
If the Bluetooth Serial Monitor app is useful for you, please give it a 5-star rating on Play Store. Thank you!
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.