Arduino - 7-segment Clock
In this tutorial, we are going to learn how to make 7-segment clock using Arduino. In detail, we will learn two cases:
- Arduino reads time (minute and second) from DS3231 RTC module and display it on the TM1637 4-digit 7-segment module
- Arduino reads time (hour and minute) from DS3231 RTC module and display it on the TM1637 4-digit 7-segment module
You can also use the DS1307 RTC module instead of the DS3231 RTC module by changing a single line of code. See DS3231 vs DS1307
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About TM1637 display, DS3231 and DS1307 RTC modules
If you do not know about TM1637 7-segment display, DS3231 and DS1307 (pinout, how it works, how to program ...), learn about them in the following tutorials:
Install TM1637 and RTC Libraries
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search “TM1637”, then find the TM1637Display library by Avishay Orpaz
- Click Install button.
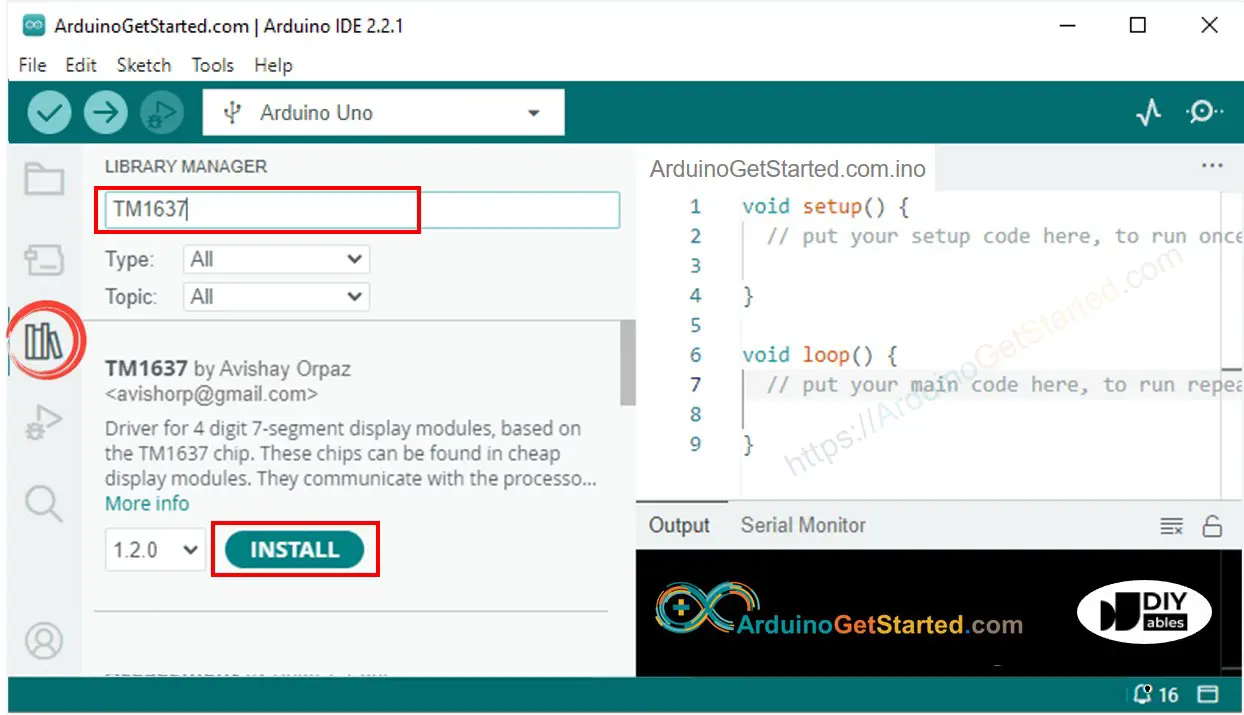
- Search “RTClib”, then find the RTC library by Adafruit. This library works with both DS3231 and DS1307
- Click Install button to install RTC library.
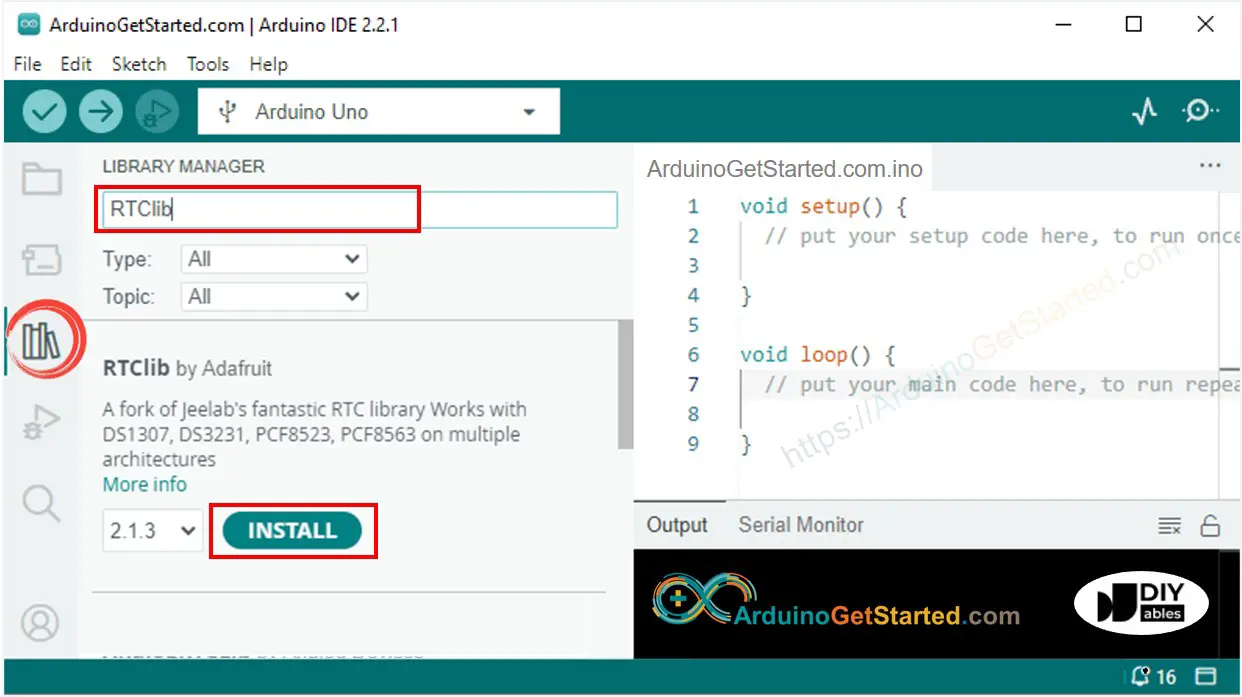
- You may be asked for intalling some other library dependencies
- Click Install All button to install all library dependencies.
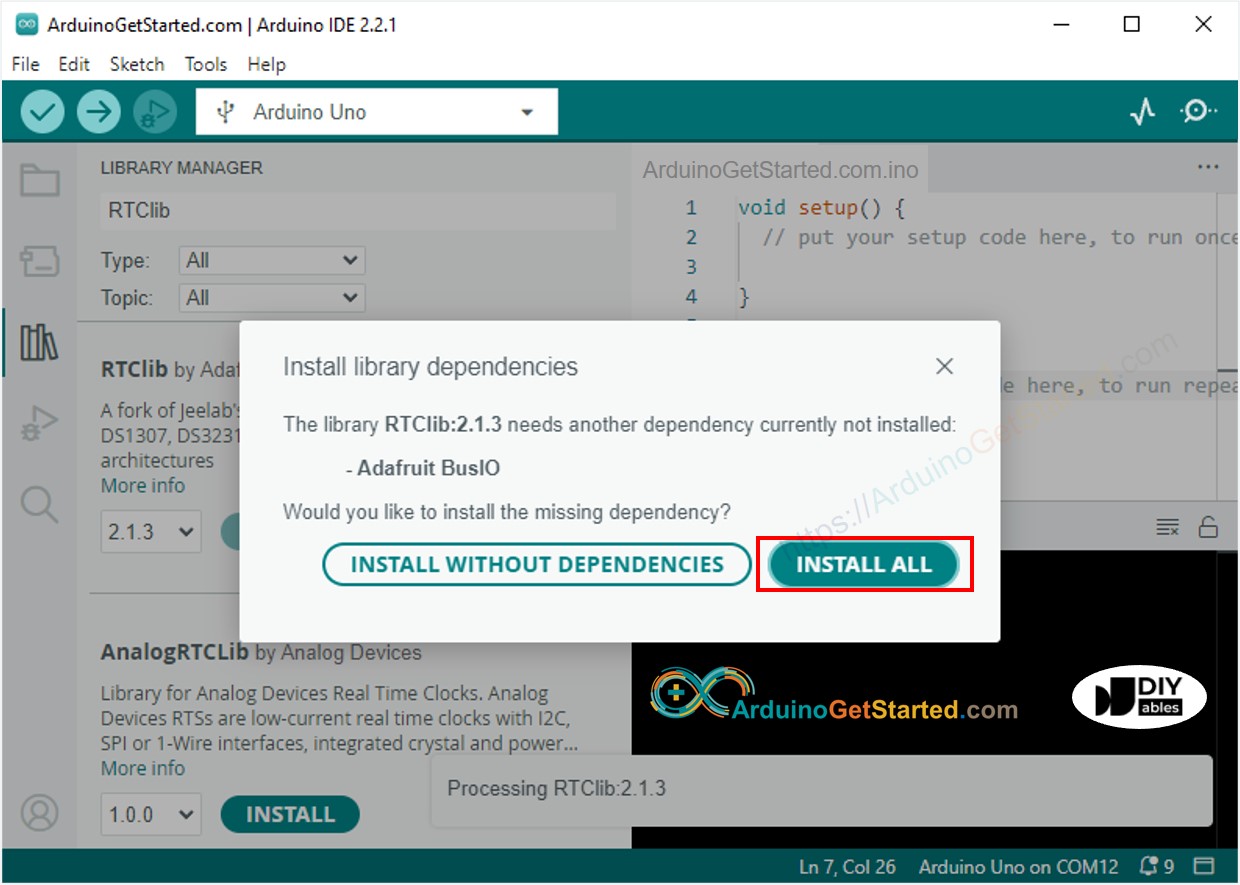
Wiring Diagram
- The wiring diagram between Arduino, TM1637 4-digit 7-segment display and DS3231 RTC module.
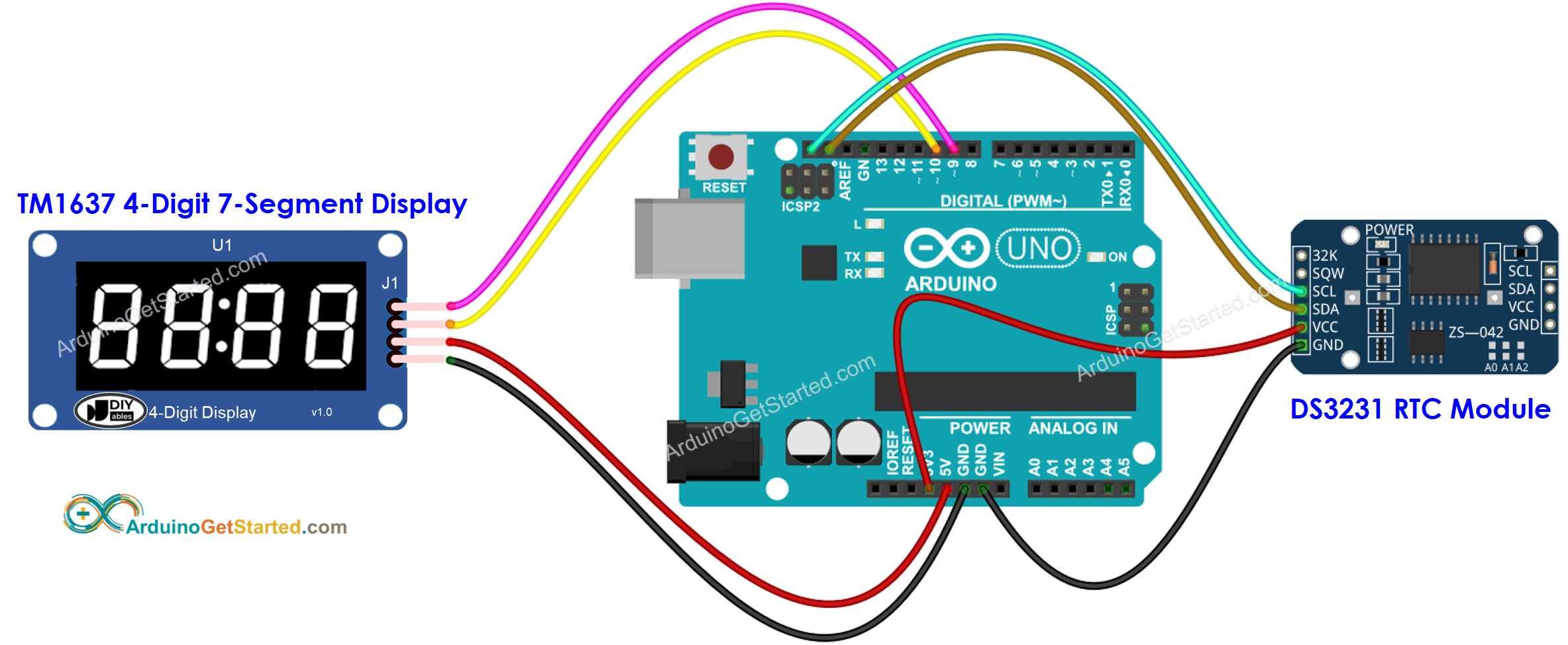
This image is created using Fritzing. Click to enlarge image
- The wiring diagram between Arduino, TM1637 4-digit 7-segment display and DS1307 RTC module.
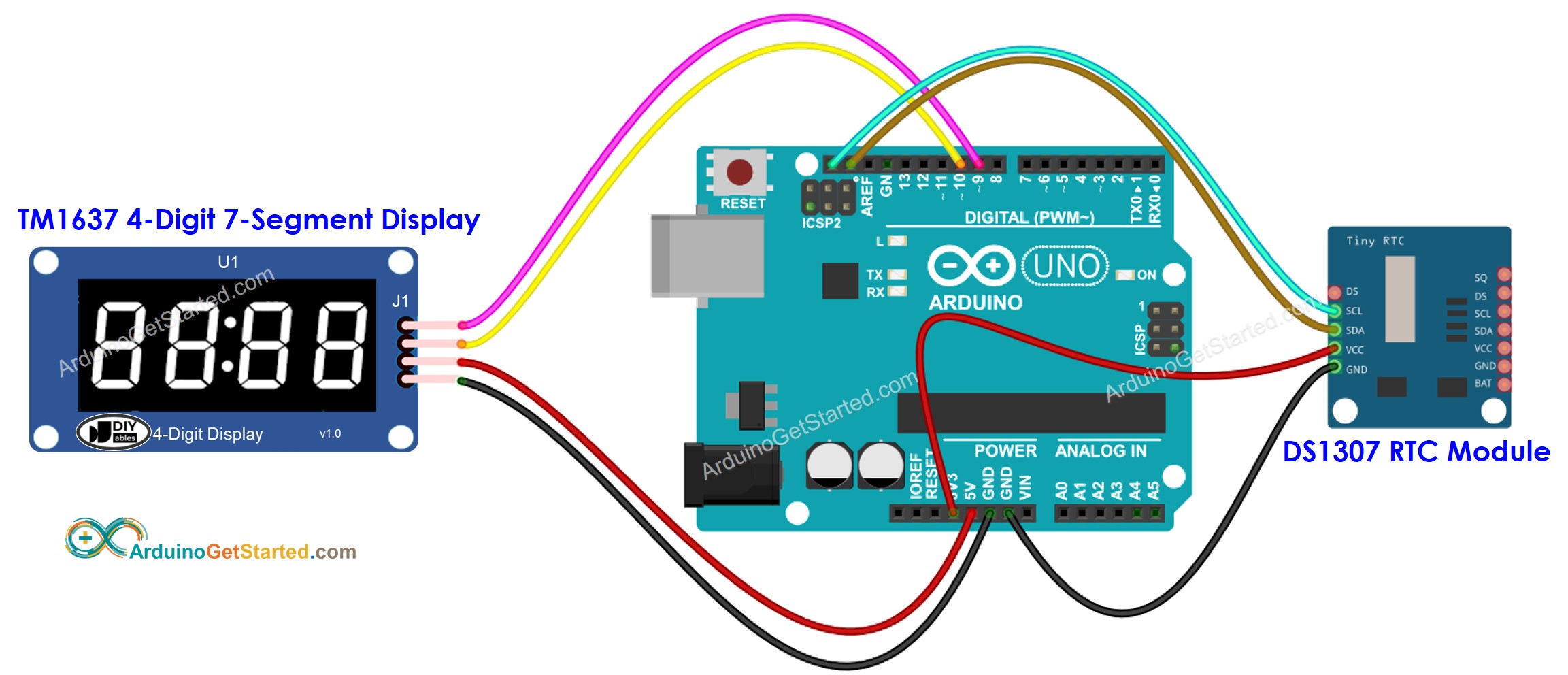
This image is created using Fritzing. Click to enlarge image
Arduino Code - Displaying minute and seconds on the 7-segment display
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Check out the result on the Serial Monitor and TM1637 display.
Code Explanation
Read the line-by-line explanation in comment lines of source code!
If you want to use the DS1307 RTC module instead of DS3231 RTC module, you just need to commment/uncomment a line specified in the code
Arduino Code - Displaying hour and minute on the 7-segment display
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.