Arduino - Car
One of the most exciting projects for Arduino beginners is creating an Arduino robot car. In this tutorial, we will discover how to use Arduino to build a robot car and control it using an IR remote controller. We will also explore another tutorial where we will learn to transform it into a Bluetooth-controlled car.
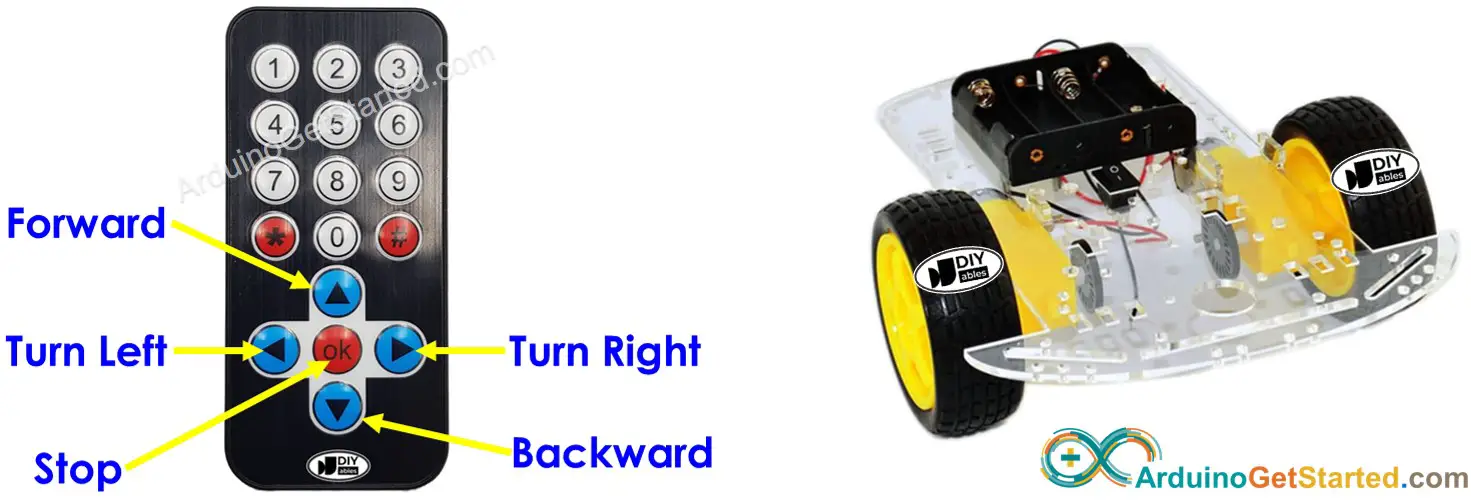
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About Robot Car
In the Arduino context, the robot car is commonly referred to as the robot car, RC car, remote control car, smart car, or DIY car. It can be controlled remotely and wirelessly using either an IR remote controller or a smartphone app via Bluetooth/WiFi... The robot car can turn left or right and move forward or backward.
A 2WD (Two-Wheel Drive) car for Arduino is a small robotic vehicle that you can build and control using an Arduino board. It typically consists of the following components:
- Chassis: The base or frame of the car,where all other components are mounted.
- Wheels: The two wheels that provide locomotion to the car. They are attached to two DC motor.
- Motors: Two DC motors are used to drive the two wheels.
- Motor Driver: The motor driver board is an essential component that interfaces between the Arduino and the motors. It takes signals from the Arduino and provides the necessary power and control to the motors.
- Arduino Board: The brain of the car. It reads inputs from sensors and user commands and controls the motors accordingly.
- Power Source: The 2WD car requires a power source, usually batteries and a battery holder, to power the motors and the Arduino board.
- Wireless receiver: an infrared, Bluetooth or WiFi module for wireless communication with a remote control or smartphone.
- Optional Components: Depending on your project's complexity, you may add various optional components like sensors (e.g., ultrasonic sensors for obstacle avoidance, line-following sensors), and more.
In this tutorial, to make it simple, we will use:
- 2WD Car kit (including Chassis, wheels, motors, battery holder)
- L298N Motor Driver
- IR infrared kit (including IR controller and IR receiver)
Check the hardware list at the top of this page.
How It Works
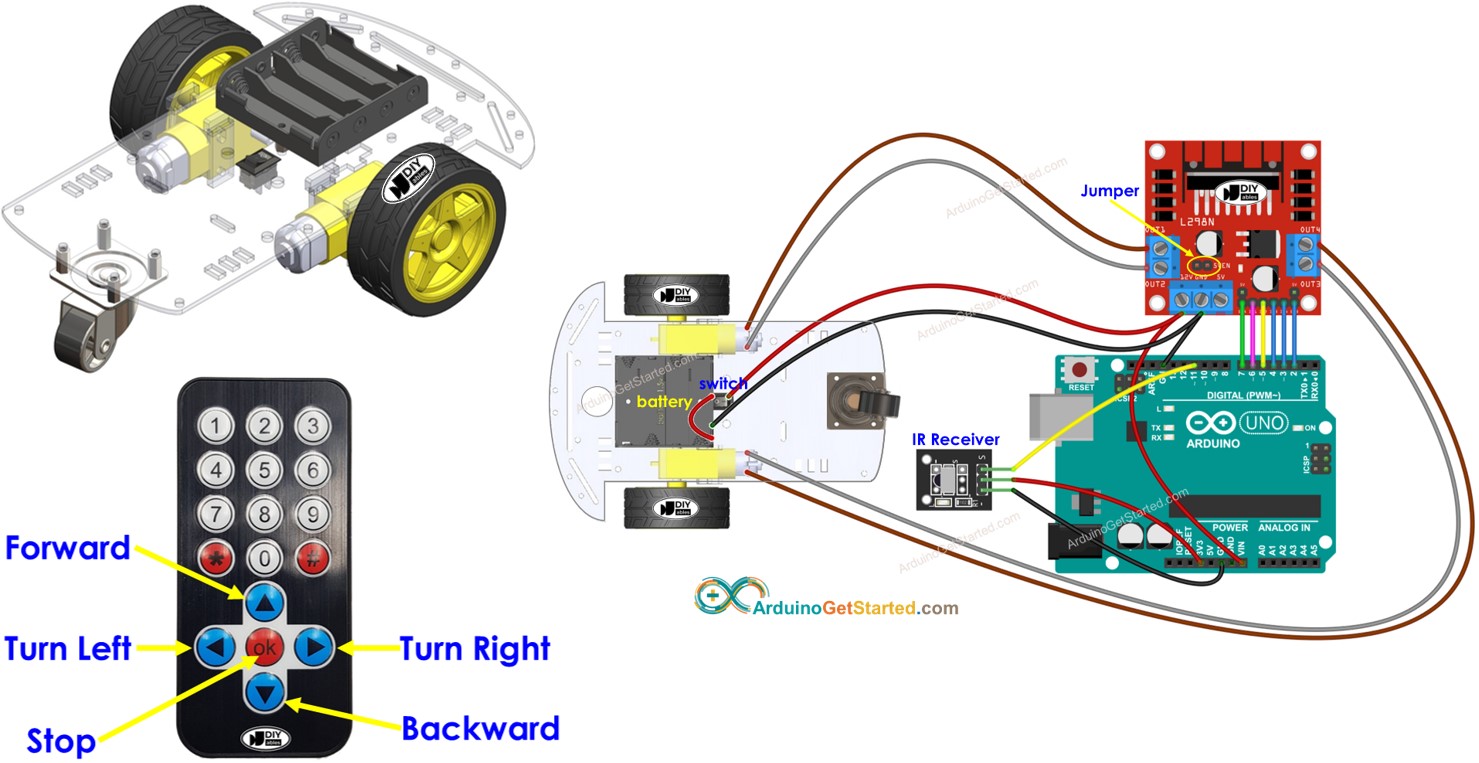
- Arduino connects to the DC motors of the robot car through L298N motor driver module.
- Arduino connects to an IR receiver.
- The battery powers Arduino, DC motors, motor driver, and IR receiver.
- Users press the UP/DOWN/LEFT/RIGHT/OK keys on the IR remote controller.
- Arduino receives the UP/DOWN/LEFT/RIGHT/OK commands through the IR receiver.
- Arduino controls the car to move FORWARD/BACKWARD/LEFT/RIGHT/STOP by controlling the DC motor via the motor driver.
Wiring Diagram
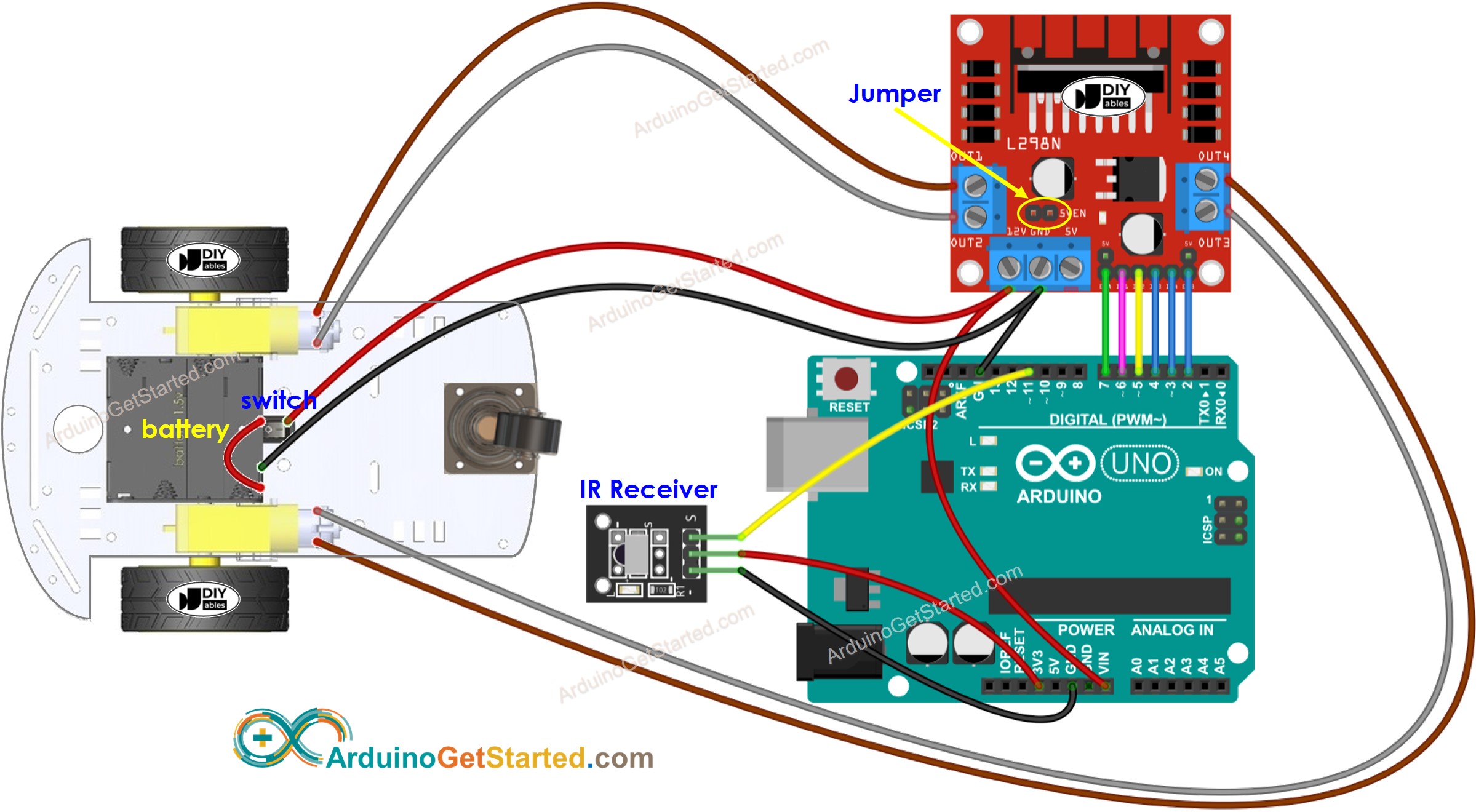
This image is created using Fritzing. Click to enlarge image
Usually, we need two power sources:
- One power source for the motor (indirectly via L298N module).
- The other power source for the Arduino board, the L298N module, the IR receiver.
However, there's a way to use just one power source for everything. You can use four 1.5V batteries (totaling 6V) for this purpose. Here's how we can do it:
- Connect the batteries to the L298N module by following the above diagram.
- Remove two small connectors (jumpers) that link the ENA and ENB pins to 5 volts on the L298N module.
- Put a special connector (jumper) labeled 5VEN, marked with a yellow circle on the above diagram.
- Connect the 12V pin on the screw terminal of the L298N module to the Vin pin on the Arduino board. This powers the Arduino directly from battery (6V in total).
Please note that during the development period, when you need to connect the USB cable to the Arduino for programming, you MUST disconnect the power between the battery and the Arduino board by remove a wiring from Vin pin. The Arduino board should not be powered by two power sources simultaneously.
Arduino Code
Quick Steps
- Install DIYables_IRcontroller library on Arduino IDE by following this instruction
- Do the wiring as the diagram shown above.
- Disconnect the wire from the Vin pin on the Arduino because we will power Arduino via USB cable when uploading code.
- Flip the car upside down so that the wheels are on top.
- Connect the Arduino to your computer using the USB cable.
- Copy the provided code and open it in the Arduino IDE.
- Click the Upload button in the Arduino IDE to transfer the code to the Arduino.
- Use the IR remote controller to make the car go forward, backward, left, right, or stop.
- Check if the wheels move correctly according to your commands.
- If the wheels move the wrong way, swap the wires of the DC motor on the L298N module.
- You can also see the results on the Serial Monitor in the Arduino IDE.
- If everything is working fine, disconnect the USB cable from the Arduino, and connect back the wire to Vin pin to power the Arduino from the battery.
- Flip the car back to its normal position with the wheels on the ground.
- Have fun controlling the car!
Code Explanation
Read the line-by-line explanation in comment lines of code!
You can learn more about the code by checking the following tutorials:
- Arduino - Infrared Remote Control tutorial
- Arduino - DC motor tutorial
You can extend this project by:
- Adding obstacle avoidance sensors to immidilately stop the car if an obstacle is detected.
- Adding function to control the speed of car (see Arduino - DC motor tutorial). The provided code controls car with full speed.
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.