Arduino - Button - Pump
We are going to learn how to use Arduino to turn on a pump several seconds and then turn off when a button is pressed.
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some links in this section are Amazon affiliate links. If you make a purchase through these links, we may earn a commission at no extra cost to you.
Additionally, some links direct to products from our own brand, DIYables .
Additionally, some links direct to products from our own brand, DIYables .
Wiring Diagram
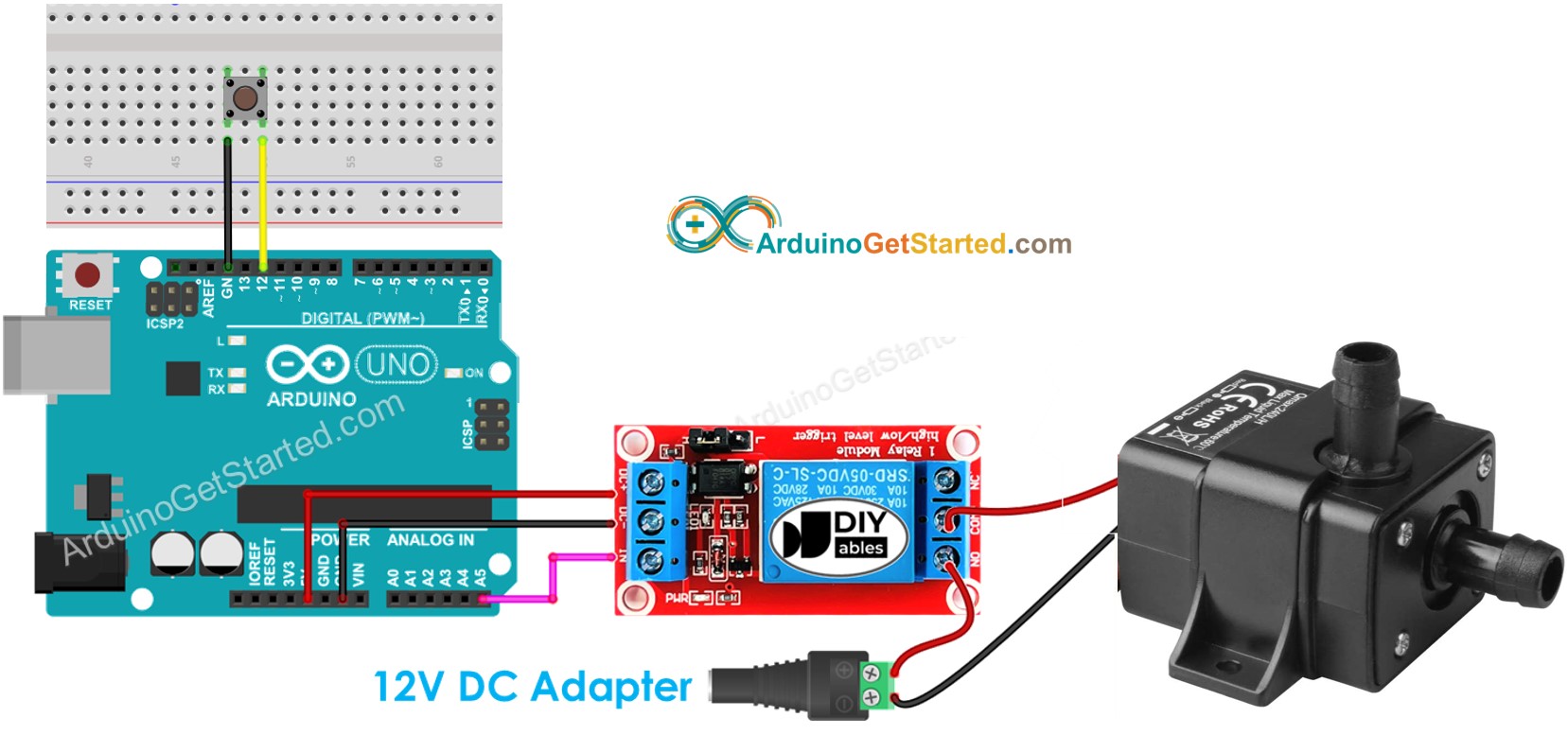
This image is created using Fritzing. Click to enlarge image
Arduino Code
/*
* Created by ArduinoGetStarted.com
*
* This example code is in the public domain
*
* Tutorial page: https://arduinogetstarted.com/tutorials/arduino-button-pump
*/
#include <ezButton.h> // include ezButton library
#include <ezOutput.h> // include ezOutput library
ezOutput pump(A5); // create ezOutput object attached to pin A5
ezButton button(12); // create ezButton object attached to pin 12
void setup() {
Serial.begin(9600);
button.setDebounceTime(50); // set debounce time to 50 milliseconds
pump.low(); // turn pump off
}
void loop() {
pump.loop(); // MUST call the loop() function first
button.loop(); // MUST call the loop() function first
if (button.isPressed()) {
Serial.println("Pump is started");
pump.low();
pump.pulse(10000); // turn on for 10000 milliseconds ~ 10 seconds
// after 10 seconds, pump will be turned off by pump.loop() function
}
}
※ NOTE THAT:
The above code does:
- Debounced for the button (supported by ezButton library). See Why do we need debouncing?
- If the button is pressed, turned pump on 10 seconds and then turn it off (supported by ezOutput library)
- All code is non-blocking code (supported by ezButton and ezOutput libraries)
Quick Steps
- Connect Arduino to PC via USB cable
- Open Arduino IDE, select the right board and port
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search “ezButton”, then find the button library by ArduinoGetStarted
- Click Install button to install ezButton library.
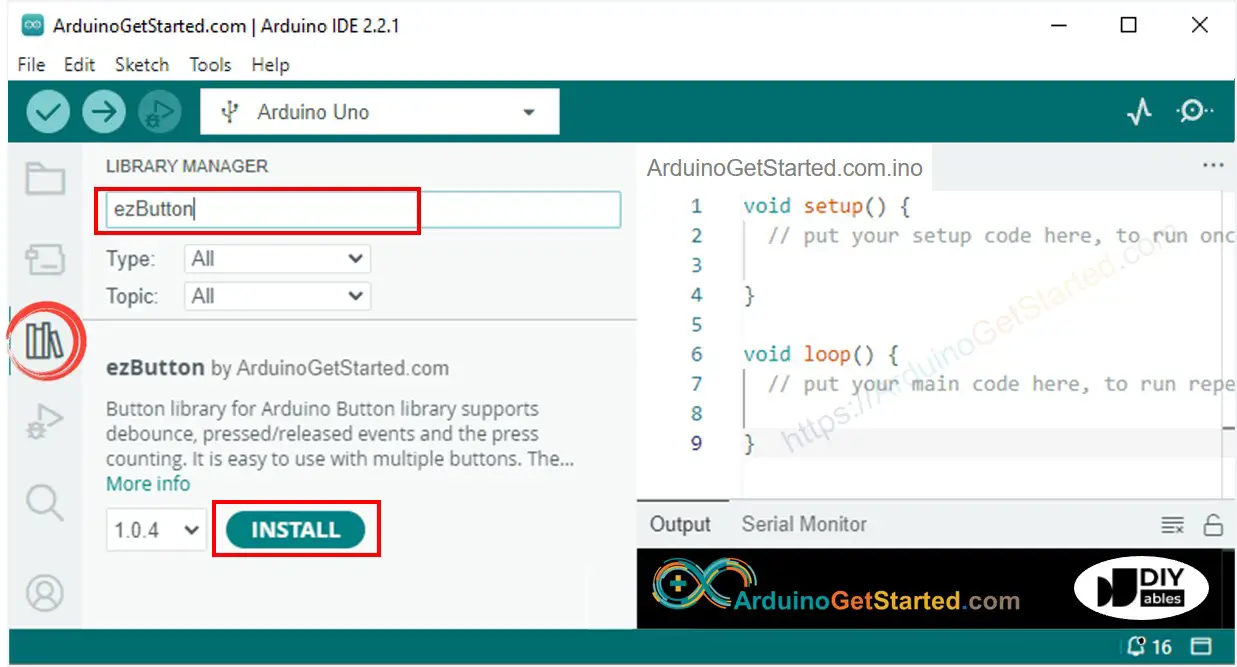
- Search “ezOutput”, then find the output library by ArduinoGetStarted
- Click Install button to install ezOutput library.
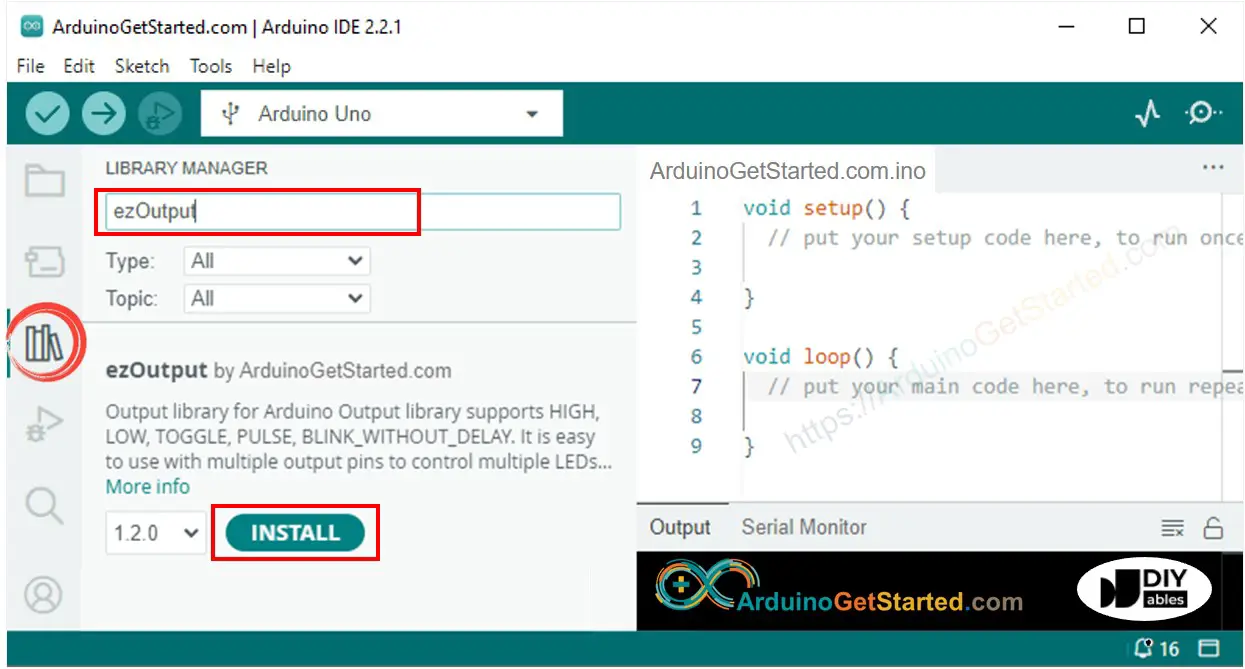
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
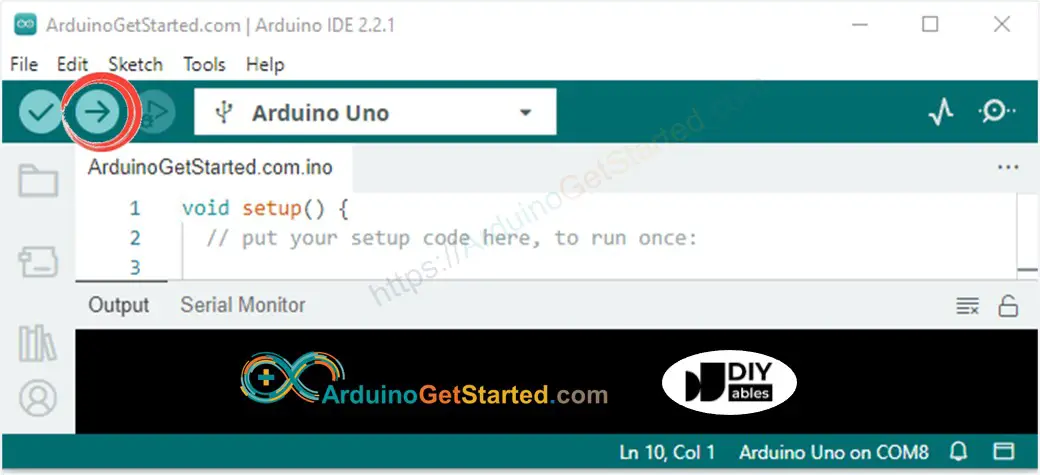
- Press the button
- See the pump's state
Code Explanation
Read the line-by-line explanation in comment lines of source code!
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.