Arduino - LM35 Temperature Sensor
Hardware Required
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About LM35 Temperature Sensor
Pinout
LM35 temperature sensor has three pins:
- GND pin: needs to be connected to GND (0V)
- VCC pin: needs to be connected to VCC (5V)
- OUT pin: signal pin gives the output voltage that is linearly proportional to the temperature, should be connected to a analog pin on Arduino.
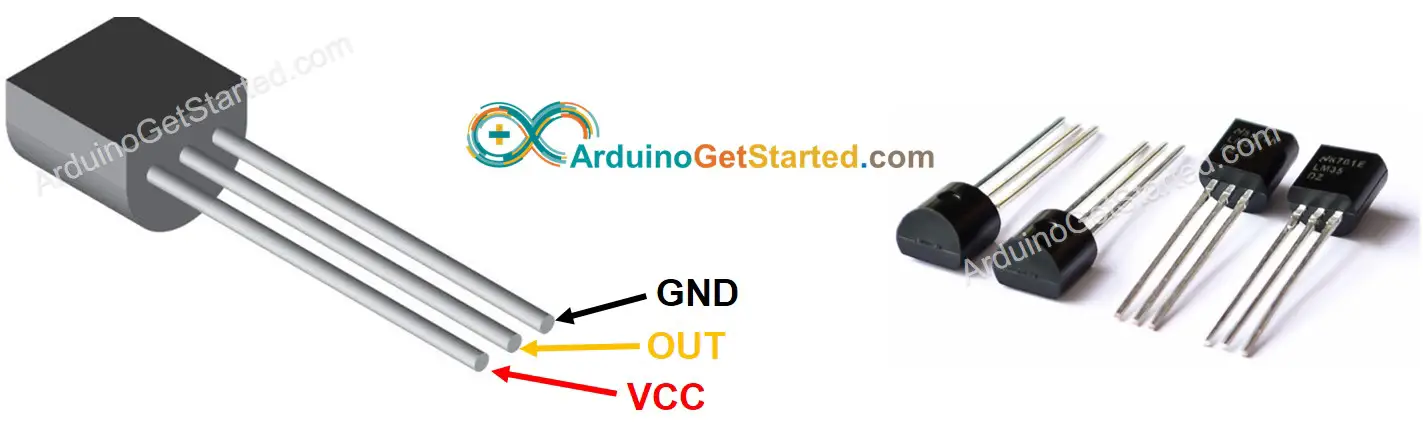
How It Works
The LM35 outputs the voltage linearly proportional to the Centigrade temperature. The output scale factor of the LM35 is 10 mV/°C. It means that the temperature is calculated by dividing the voltage (mV) in output pin by 10.
Wiring Diagram
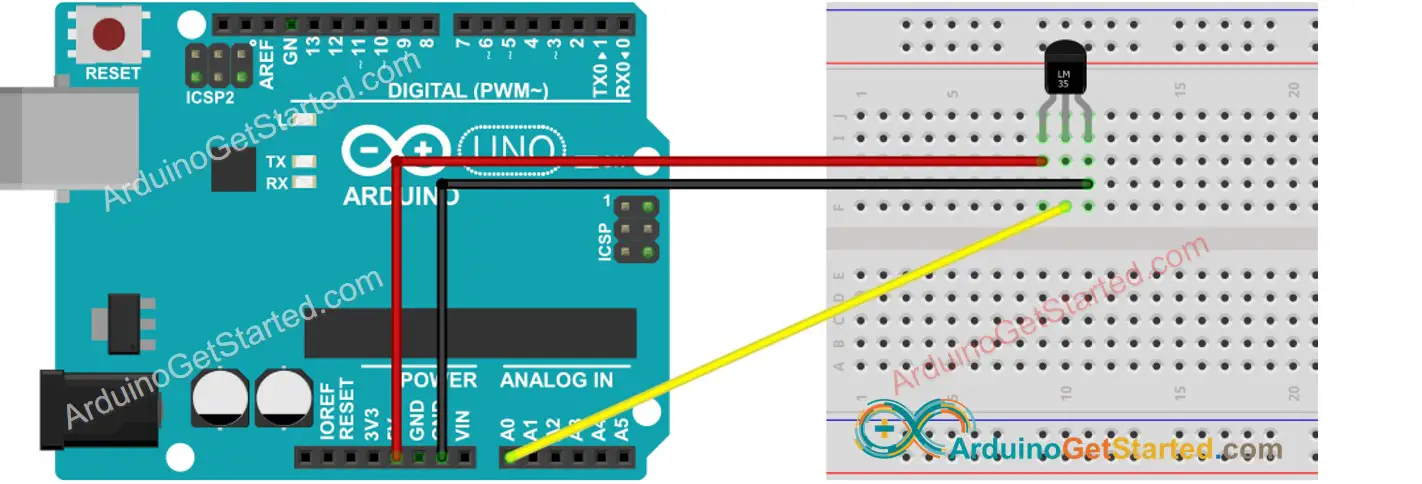
This image is created using Fritzing. Click to enlarge image
How To Program For LM35 Temperature Sensor
- Get the ADC value from the temperature sensor by using analogRead() function.
- Convert the ADC value to voltage in millivolt
- Convert the voltage to the temperature in Celsius
- (Optional) Convert the Celsius to Fahrenheit
Arduino Code
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Grasp the sensor by your hand
- See the result on Serial Monitor.
Improving the Temperature Precision
In the above code, we use ADC reference voltage by default (5V~5000mV). We can increase the temperature resolution by changing reference voltage to INTERNAL (1.1V~1100mV). This reference voltage can be changed using the analogReference() function.
The below table show the diffrent between using 5000mV and 1100mV reference voltage
Vref(mV) | 5000 mV (by default) | 1100 mV (INTERNAL) |
---|---|---|
Reading Resolution | 5000/1024 = 4.88 mV | 1100/1024 = 1.07 mV |
Temperature Resolution | 0.488 °C | 0.107 °C |
Temperature Range | 0 to 500 °C | 0 to 110 °C |
Arduino Code
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.