Arduino - Temperature via Web
In this tutorial, we will learn how to program Arduino to become a web server that provides you with the temperature via the web. You can access the web page provided by Arduino to check the temperature from a DS18B20 temperature sensor. The below is how it works:
Arduino is programmed as a web server.
You type the IP address of Arduino in a web browser on your smartphone or PC.
Arduino responds to the request from the web browser with a web page that contains the temperature read from the DS18B20 sensor.
We will go through two example codes:
Arduino code that provides a very simple web page that shows the temperature from the DS18B20 sensor. This makes it easy for you to understand how it works.
Arduino code that provides a graphic web page that shows the temperature from the DS18B20 sensor.
Or you can buy the following kits:
Disclosure: Some links in this section are Amazon affiliate links. If you make a purchase through these links, we may earn a commission at no extra cost to you.
Additionally, some links direct to products from our own brand,
DIYables .
Buy Note: Many DS18B20 sensors on the market are low-quality. We highly recommend buying the sensor from the DIYables brand using the link above. We tested it, and it worked well.
If you do not know about Arduino Uno R4 and DS18B20 temperature sensor (pinout, how it works, how to program ...), learn about them in the following tutorials:
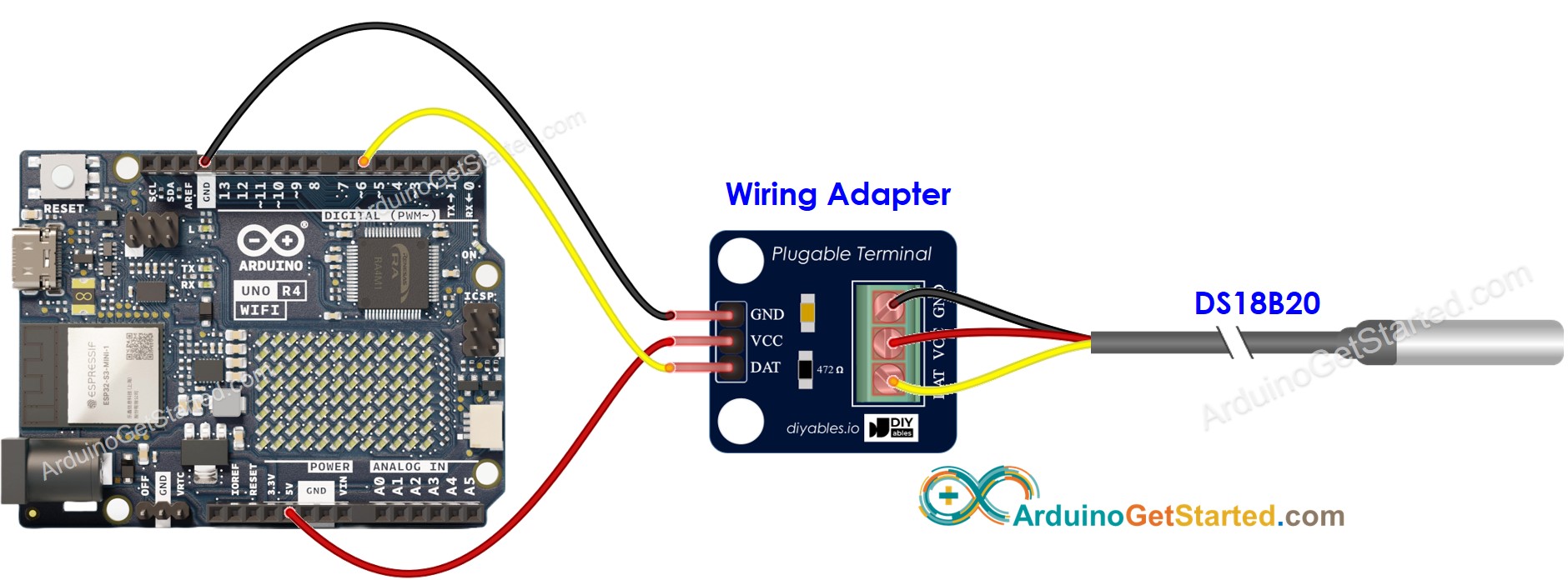
This image is created using Fritzing. Click to enlarge image
#include <WiFiS3.h>
#include <OneWire.h>
#include <DallasTemperature.h>
const char ssid[] = "YOUR_WIFI";
const char pass[] = "YOUR_WIFI_PASSWORD";
const int SENSOR_PIN = 6;
OneWire oneWire(SENSOR_PIN);
DallasTemperature tempSensor(&oneWire);
int status = WL_IDLE_STATUS;
WiFiServer server(80);
float getTemperature() {
tempSensor.requestTemperatures();
float tempCelsius = tempSensor.getTempCByIndex(0);
return tempCelsius;
}
void setup() {
Serial.begin(9600);
tempSensor.begin();
String fv = WiFi.firmwareVersion();
if (fv < WIFI_FIRMWARE_LATEST_VERSION)
Serial.println("Please upgrade the firmware");
while (status != WL_CONNECTED) {
Serial.print("Attempting to connect to SSID: ");
Serial.println(ssid);
status = WiFi.begin(ssid, pass);
delay(10000);
}
server.begin();
printWifiStatus();
}
void loop() {
WiFiClient client = server.available();
if (client) {
while (client.connected()) {
if (client.available()) {
String HTTP_header = client.readStringUntil('\n');
if (HTTP_header.equals("\r"))
break;
Serial.print("<< ");
Serial.println(HTTP_header);
}
}
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: text/html");
client.println("Connection: close");
client.println();
client.println("<!DOCTYPE HTML>");
client.println("<html>");
client.println("<head>");
client.println("<link rel=\"icon\" href=\"data:,\">");
client.println("</head>");
client.println("<p>");
client.print("Temperature: <span style=\"color: red;\">");
float temperature = getTemperature();
client.print(temperature, 2);
client.println("°C</span>");
client.println("</p>");
client.println("</html>");
client.flush();
delay(10);
client.stop();
}
}
void printWifiStatus() {
Serial.print("IP Address: ");
Serial.println(WiFi.localIP());
Serial.print("signal strength (RSSI):");
Serial.print(WiFi.RSSI());
Serial.println(" dBm");
}
Copy the above code and open with Arduino IDE
Change the wifi information (SSID and password) in the code to yours
Click Upload button on Arduino IDE to upload code to Arduino
Open the Serial Monitor
Check out the result on Serial Monitor.
Attempting to connect to SSID: YOUR_WIFI
IP Address: 192.168.0.2
signal strength (RSSI):-39 dBm
Attempting to connect to SSID: YOUR_WIFI
IP Address: 192.168.0.2
signal strength (RSSI):-41 dBm
New HTTP Request
<< GET / HTTP/1.1
<< Host: 192.168.0.2
<< Connection: keep-alive
<< Cache-Control: max-age=0
<< Upgrade-Insecure-Requests: 1
<< User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64)
<< Accept: text/html,application/xhtml+xml,application/xml
<< Accept-Encoding: gzip, deflate
<< Accept-Language: en-US,en;q=0.9
As a graphic web page contains a large amount of HTML content, embedding it into the Arduino code as before becomes inconvenient. To address this, we need to separate the Arduino code and the HTML code into different files:
The Arduino code will be placed in a .ino file.
The HTML code (including HTML, CSS, and Javascript) will be placed in a .h file.
Open Arduino IDE and create new sketch, Give it a name, for example, ArduinoGetStarted.com.ino
Copy the below code and open with Arduino IDE
#include <WiFiS3.h>
#include "index.h"
#include <OneWire.h>
#include <DallasTemperature.h>
const char ssid[] = "YOUR_WIFI";
const char pass[] = "YOUR_WIFI_PASSWORD";
const int SENSOR_PIN = 6;
OneWire oneWire(SENSOR_PIN);
DallasTemperature tempSensor(&oneWire);
int status = WL_IDLE_STATUS;
WiFiServer server(80);
float getTemperature() {
tempSensor.requestTemperatures();
float tempCelsius = tempSensor.getTempCByIndex(0);
return tempCelsius;
}
void setup() {
Serial.begin(9600);
tempSensor.begin();
String fv = WiFi.firmwareVersion();
if (fv < WIFI_FIRMWARE_LATEST_VERSION)
Serial.println("Please upgrade the firmware");
while (status != WL_CONNECTED) {
Serial.print("Attempting to connect to SSID: ");
Serial.println(ssid);
status = WiFi.begin(ssid, pass);
delay(10000);
}
server.begin();
printWifiStatus();
}
void loop() {
WiFiClient client = server.available();
if (client) {
while (client.connected()) {
if (client.available()) {
String HTTP_header = client.readStringUntil('\n');
if (HTTP_header.equals("\r"))
break;
Serial.print("<< ");
Serial.println(HTTP_header);
}
}
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: text/html");
client.println("Connection: close");
client.println();
float temp = getTemperature();
String html = String(HTML_CONTENT);
html.replace("TEMPERATURE_MARKER", String(temp, 2));
client.println(html);
client.flush();
delay(10);
client.stop();
}
}
void printWifiStatus() {
Serial.print("IP Address: ");
Serial.println(WiFi.localIP());
Serial.print("signal strength (RSSI):");
Serial.print(WiFi.RSSI());
Serial.println(" dBm");
}
Change the WiFi information (SSID and password) in the code to yours
Create the index.h file On Arduino IDE by:
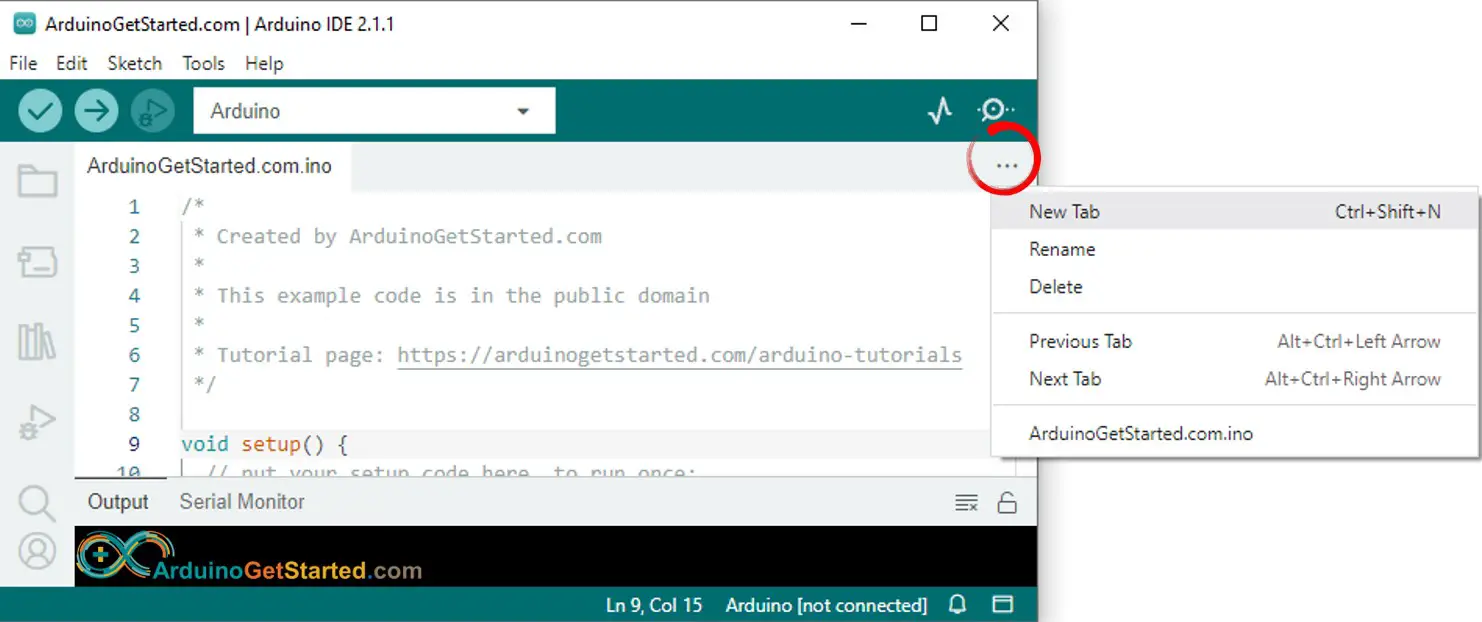
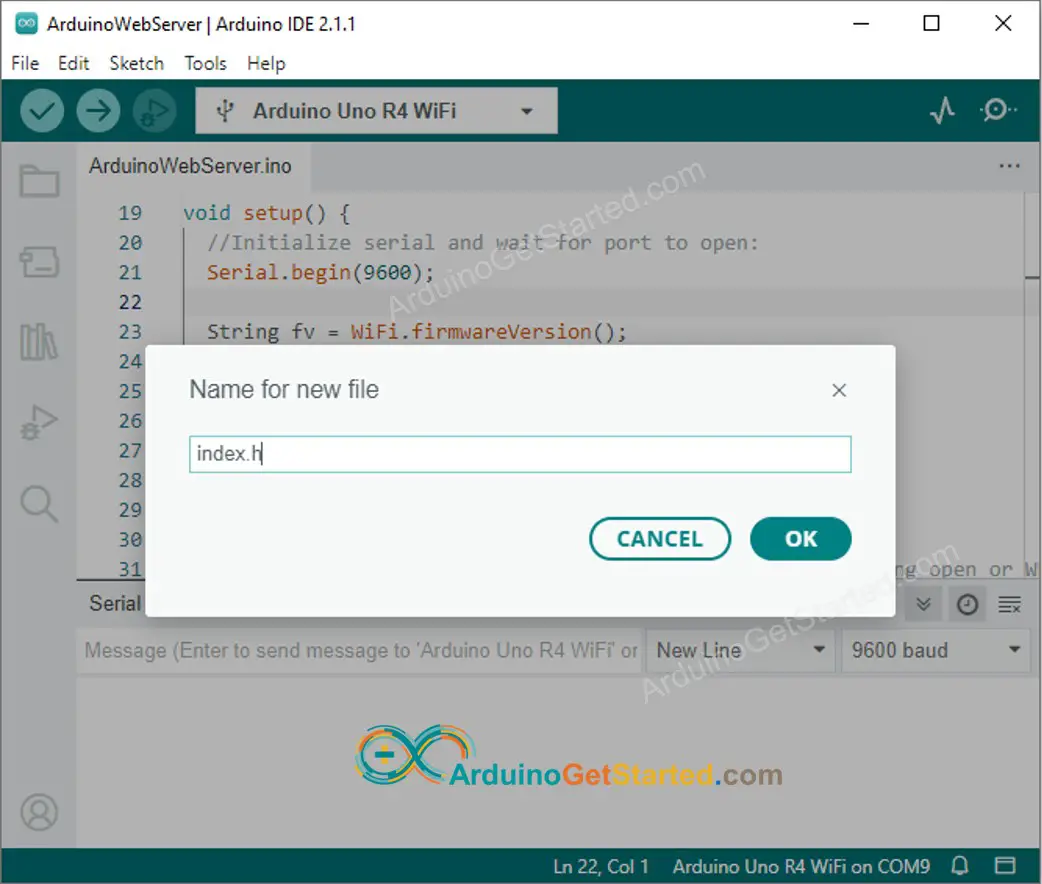
const char *HTML_CONTENT = R""""(
<!DOCTYPE html>
<html>
<head>
<title>Arduino - Web Temperature</title>
<meta name="viewport" content="width=device-width, initial-scale=0.7, maximum-scale=0.7">
<meta charset="utf-8">
<link rel="icon" href="https://diyables.io/images/page/diyables.svg">
<style>
body { font-family: "Georgia"; text-align: center; font-size: width/2pt;}
h1 { font-weight: bold; font-size: width/2pt;}
h2 { font-weight: bold; font-size: width/2pt;}
button { font-weight: bold; font-size: width/2pt;}
</style>
<script>
var cvs_width = 200, cvs_height = 450;
function init() {
var canvas = document.getElementById("cvs");
canvas.width = cvs_width;
canvas.height = cvs_height + 50;
var ctx = canvas.getContext("2d");
ctx.translate(cvs_width/2, cvs_height - 80);
update_view(TEMPERATURE_MARKER);
}
function update_view(temp) {
var canvas = document.getElementById("cvs");
var ctx = canvas.getContext("2d");
var radius = 70;
var offset = 5;
var width = 45;
var height = 330;
ctx.clearRect(-cvs_width/2, -350, cvs_width, cvs_height);
ctx.strokeStyle="blue";
ctx.fillStyle="blue";
var x = -width/2;
ctx.lineWidth=2;
for (var i = 0; i <= 100; i+=5) {
var y = -(height - radius)*i/100 - radius - 5;
ctx.beginPath();
ctx.lineTo(x, y);
ctx.lineTo(x - 20, y);
ctx.stroke();
}
ctx.lineWidth=5;
for (var i = 0; i <= 100; i+=20) {
var y = -(height - radius)*i/100 - radius - 5;
ctx.beginPath();
ctx.lineTo(x, y);
ctx.lineTo(x - 25, y);
ctx.stroke();
ctx.font="20px Georgia";
ctx.textBaseline="middle";
ctx.textAlign="right";
ctx.fillText(i.toString(), x - 35, y);
}
ctx.lineWidth=16;
ctx.beginPath();
ctx.arc(0, 0, radius, 0, 2 * Math.PI);
ctx.stroke();
ctx.beginPath();
ctx.rect(-width/2, -height, width, height);
ctx.stroke();
ctx.beginPath();
ctx.arc(0, -height, width/2, 0, 2 * Math.PI);
ctx.stroke();
ctx.fillStyle="#e6e6ff";
ctx.beginPath();
ctx.arc(0, 0, radius, 0, 2 * Math.PI);
ctx.fill();
ctx.beginPath();
ctx.rect(-width/2, -height, width, height);
ctx.fill();
ctx.beginPath();
ctx.arc(0, -height, width/2, 0, 2 * Math.PI);
ctx.fill();
ctx.fillStyle="#ff1a1a";
ctx.beginPath();
ctx.arc(0, 0, radius - offset, 0, 2 * Math.PI);
ctx.fill();
temp = Math.round(temp * 100) / 100;
var y = (height - radius)*temp/100.0 + radius + 5;
ctx.beginPath();
ctx.rect(-width/2 + offset, -y, width - 2*offset, y);
ctx.fill();
ctx.fillStyle="red";
ctx.font="bold 34px Georgia";
ctx.textBaseline="middle";
ctx.textAlign="center";
ctx.fillText(temp.toString() + "°C", 0, 100);
}
window.onload = init;
</script>
</head>
<body>
<h1>Arduino - Web Temperature</h1>
<canvas id="cvs"></canvas>
</body>
</html>
)"""";
Now you have the code in two files: ArduinoGetStarted.com.ino and index.h
Click Upload button on Arduino IDE to upload code to Arduino
Access the web page of Arduino board via web browser as before. You will see it as below:
※ NOTE THAT:
If you make changes to the HTML content within the index.h file but don't modify anything in the ArduinoGetStarted.com.ino file, the Arduino IDE won't refresh or update the HTML content when you compile and upload the code to the ESP32.
To force the Arduino IDE to update the HTML content in this situation, you need to make a modification in the ArduinoGetStarted.com.ino file. For example, you can add an empty line or insert a comment. This action triggers the IDE to recognize that there have been changes in the project, ensuring that your updated HTML content gets included in the upload.
※ OUR MESSAGES
You can share the link of this tutorial anywhere. Howerver, please do not copy the content to share on other websites. We took a lot of time and effort to create the content of this tutorial, please respect our work!