Arduino - GPS
In this tutorial, we are going to learn how to get GPS coordinates (longitude, latitude, altitude), GPS speed (km/h), and date time from NEO-6M GPS module. We also learn how to calculate the distance from current GPS position to a predefined GPS coordinates (ex. coordinates of London)
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About NEO-6M GPS module
Pinout
The NEO-6M GPS module includes 4 pins:
- VCC pin: needs to be connected to VCC (5V)
- GND pin: needs to be connected to GND (0V)
- TX pin: is used for serial communication, needs to be connect to Serial (or SoftwareSerial) RX pin on Arduino.
- RX pin: is used for serial communication, needs to be connect to Serial (or SoftwareSerial) TX pin on Arduino.
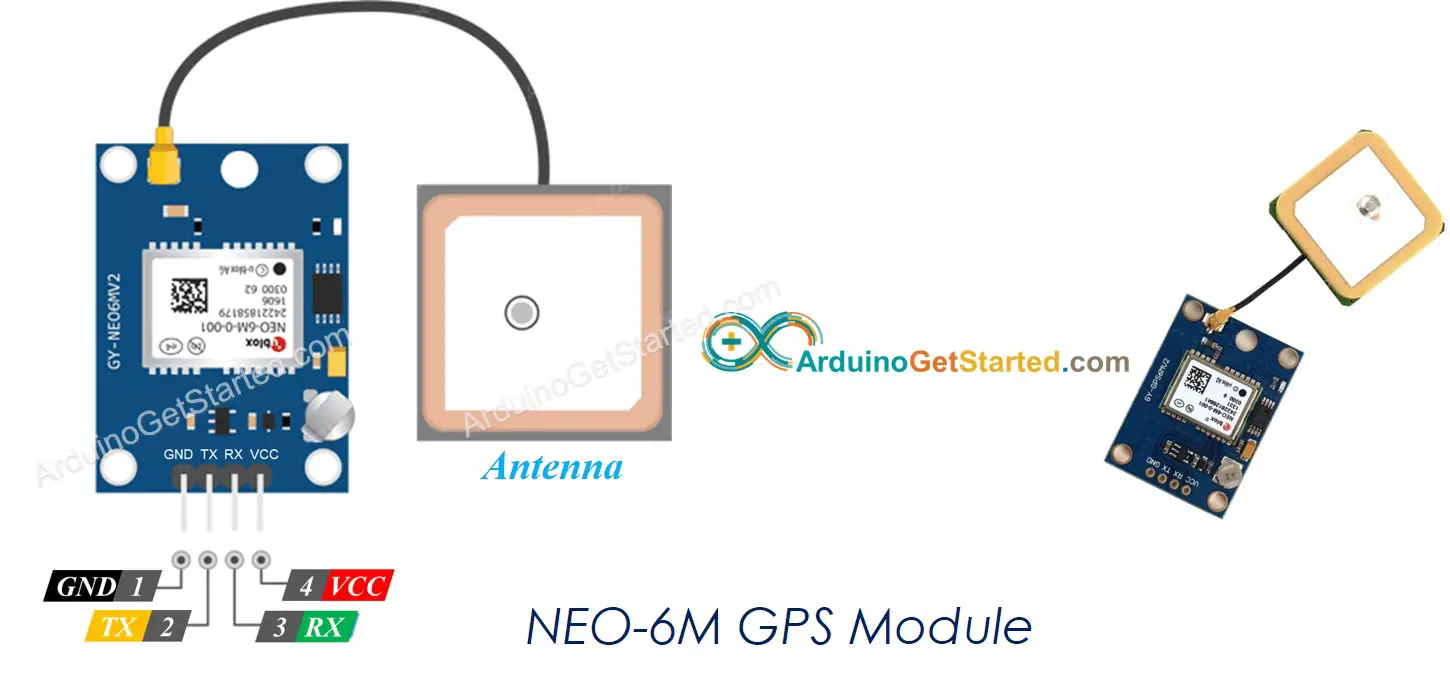
Wiring Diagram
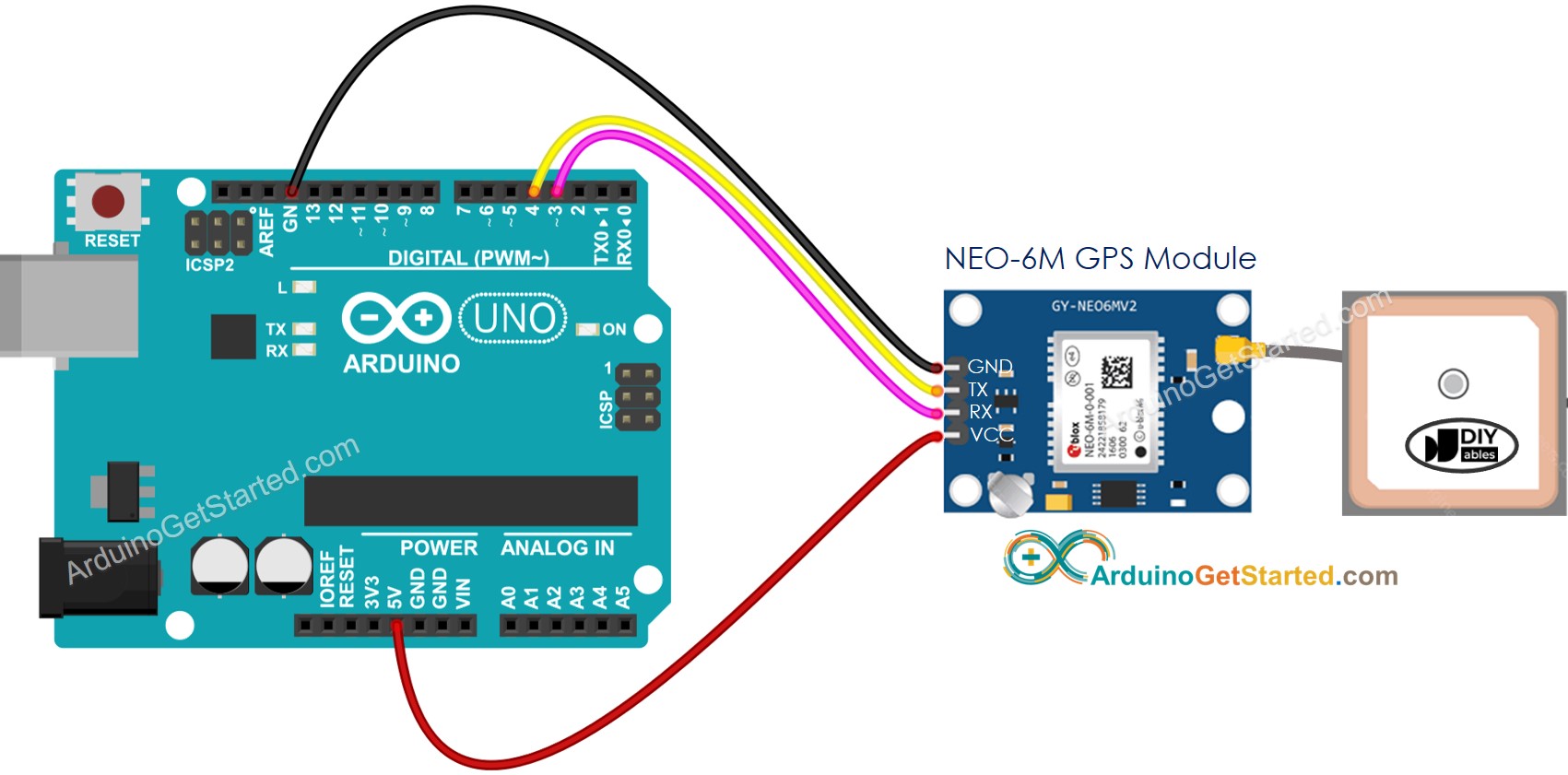
This image is created using Fritzing. Click to enlarge image
Please be aware that while the wiring diagram provided above might work, it is not advisable. The Arduino's TX pin outputs at 5V, but the GPS module's RX pin can only accept 3.3V. To ensure safety, it is recommended to use a voltage divider between the Arduino's TX pin and the RX pin of the GPS module, as shown in the diagram below.
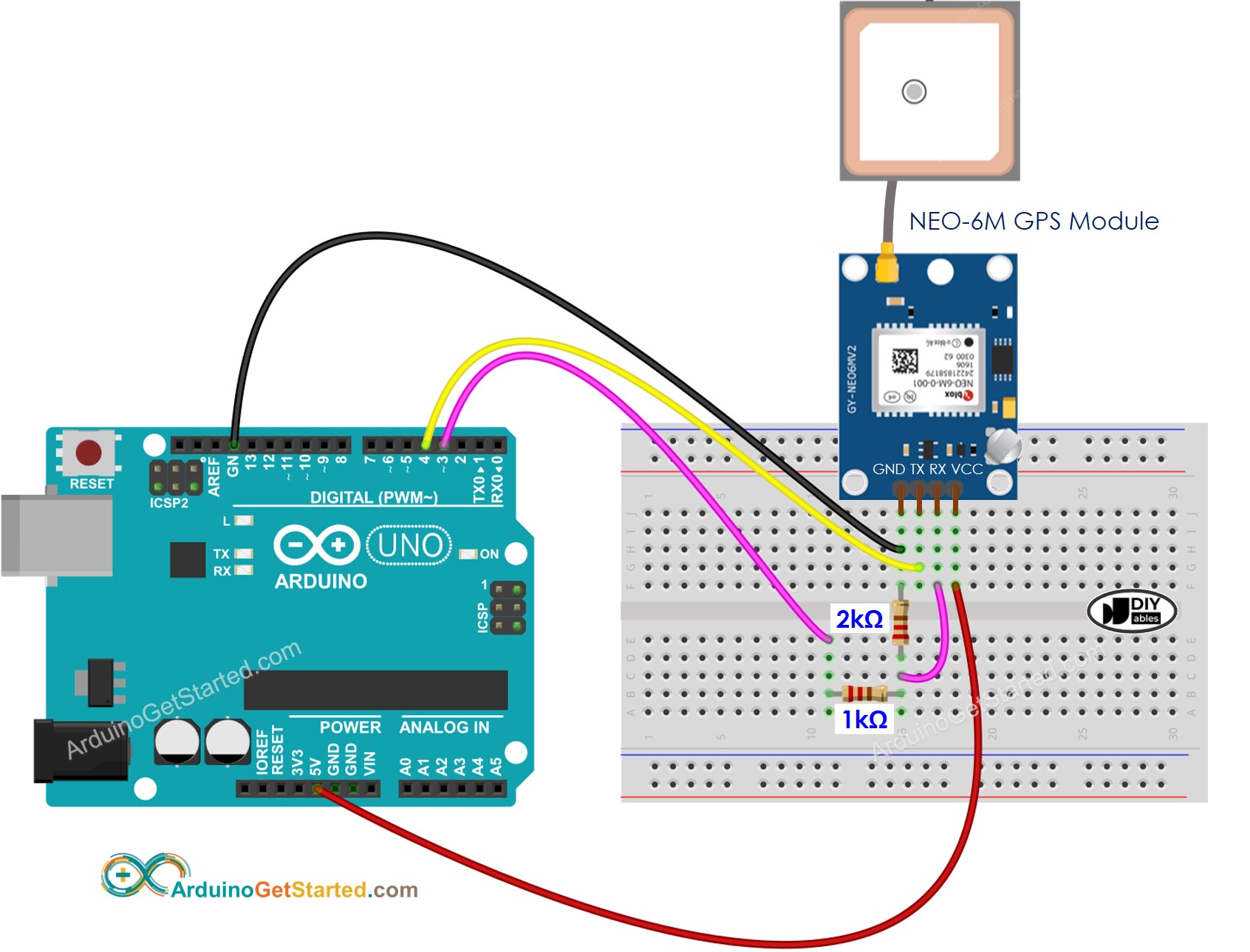
This image is created using Fritzing. Click to enlarge image
Arduino Code
Reading GPS coordinates, speed (km/h), and date time
Quick Steps
- Open Arduino IDE
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search “TinyGPSPlus”, then find the TinyGPSPlus library by Mikal Hart
- Click Install button to install TinyGPSPlus library.
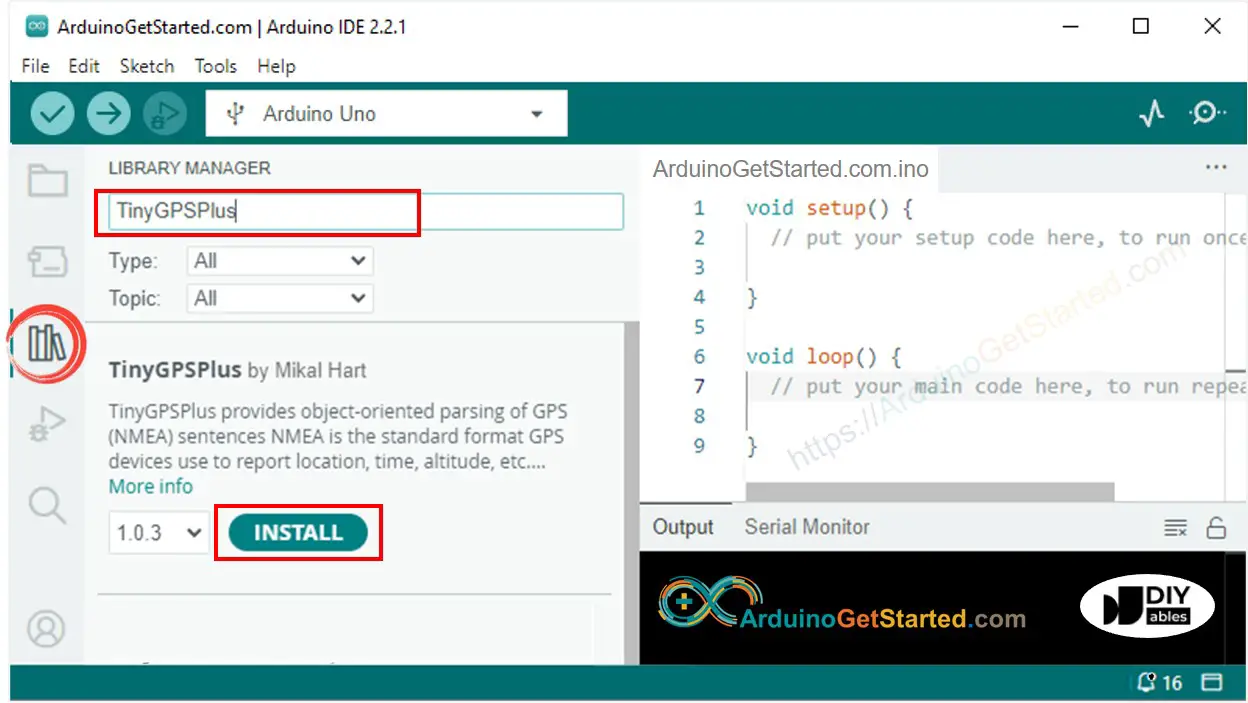
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- See the result on Serial Monitor:
Calculating the distance from current location to a predefined location
The below code calculates the distance between the current location and London (lat:51.508131 , long: -0.128002)
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- See the result on Serial Monitor:
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.
WARNING
Note that this tutorial is incomplete. We will post on our Facebook Page when the tutorial is complete. Like it to get updated.