Arduino - Solenoid Lock
The Solenoid Lock is also known as the Electric Strike Lock. It can be use to lock/unlock cabinet, drawer, door. In this tutorial, we are going to learn how to control the solenoid lock using Arduino.
An alternative to the Solenoid Lock is Electromagnetic Lock. You can learn more in Arduino - Electromagnetic Lock tutorial
Hardware Required
Or you can buy the following kits:
1 | × | DIYables STEM V3 Starter Kit (Arduino included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About Solenoid Lock
Pinout
Solenoid Lock includes two wires:
- Positive (+) wire (red): needs to be connected to 12V of DC power supply
- Negative (-) wire (black): needs to be connected to GND of DC power supply
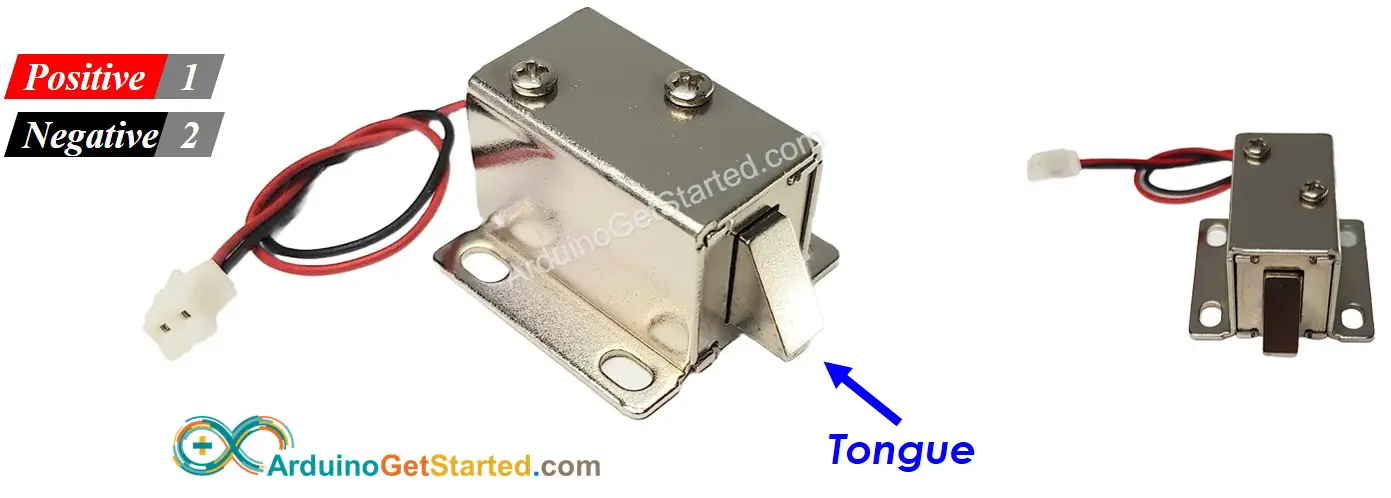
How It Works
- When the Solenoid Lock is powered, the lock tongue (strike) is extended ⇒ the door is locked
- When the Solenoid Lock is NOT powered, the lock tongue (strike) retracted ⇒ the door is unlocked
※ NOTE THAT:
The solenoid lock usually uses 12V, 24V or 48V power supply. Therefore, we CANNOT connect the solenoid lock directly to Arduino pin. We have to connect it to Arduino pin via a relay
If we connect the solenoid lock to a relay (normally open mode):
- When relay is open, door is unlocked
- When relay is closed, door is locked
By connecting Arduino to the relay, we can program for Arduino to control the solenoid lock. Learn more about relay in Arduino - Relay tutorial.
Wiring Diagram
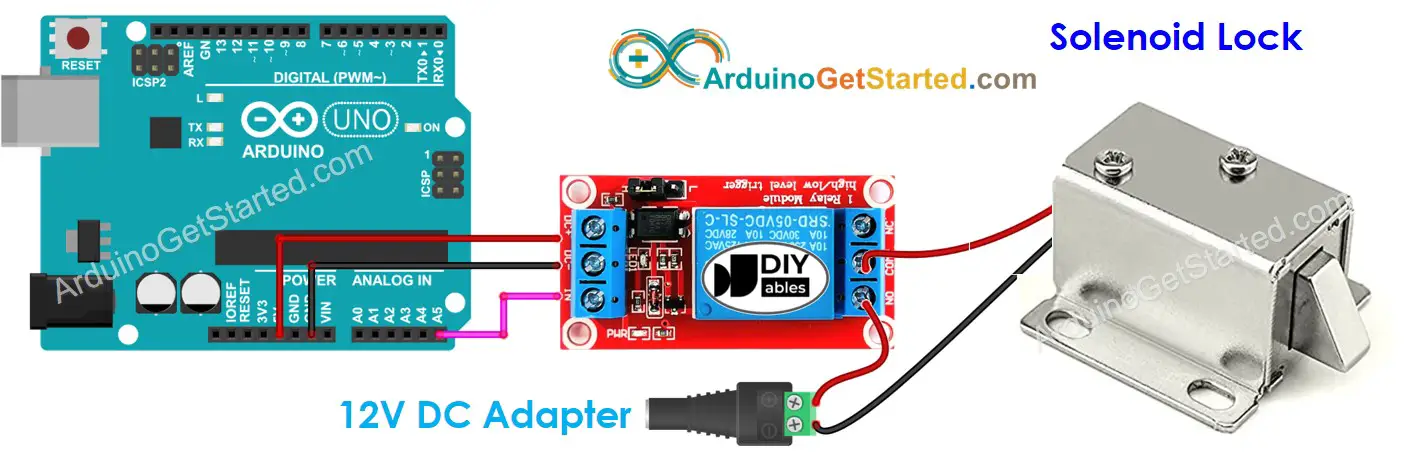
This image is created using Fritzing. Click to enlarge image
The real wiring diagram:
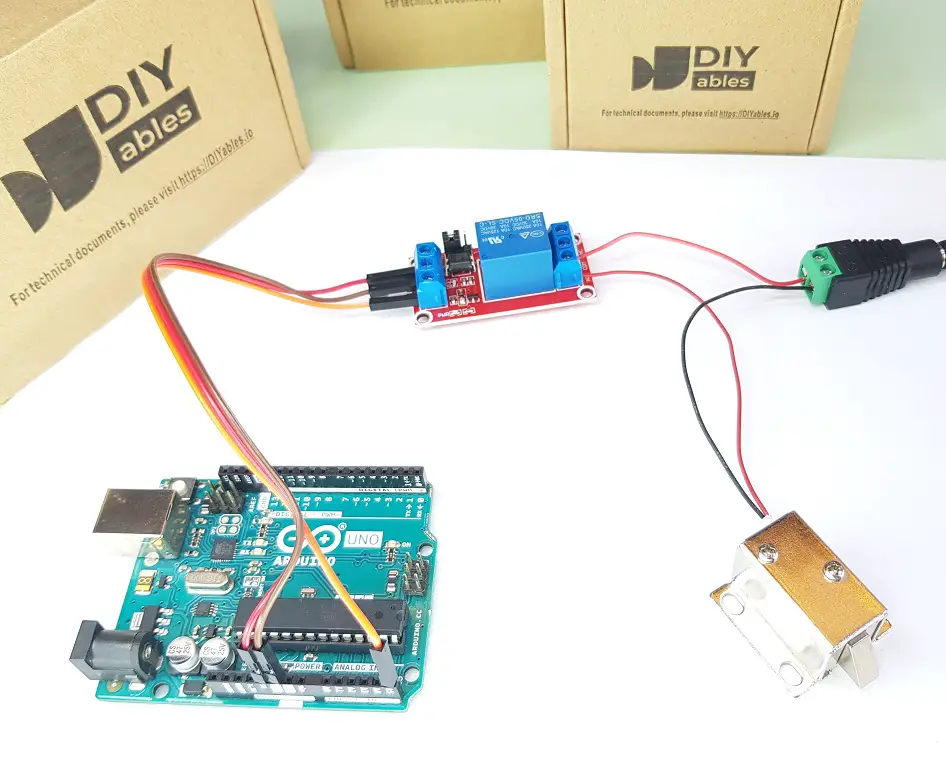
Arduino Code
The below code lock/unlock the door every 5 seconds
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- See the the lock tongue's state
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.