Arduino - Write Variable to SD Card
In this tutorial, we are going to learn how to write variable to Micro SD Card with Arduino. In detail, we will learn:
Arduino - How to write a string variable to Micro SD Card
Arduino - How to write a int variable to Micro SD Card
Arduino - How to write a float variable to Micro SD Card
Arduino - How to write a char array variable to Micro SD Card
Arduino - How to write a byte array variable to Micro SD Card
Arduino - How to write variable as key-value to Micro SD Card
To read the key-value from the Micro SD Card and convert it to int, float, string, See Arduino - Read Config from SD Card
Or you can buy the following kits:
Disclosure: Some links in this section are Amazon affiliate links. If you make a purchase through these links, we may earn a commission at no extra cost to you.
Additionally, some links direct to products from our own brand,
DIYables .
If you do not know about Micro SD Card Module (pinout, how it works, how to program ...), learn about them in the Arduino - Micro SD Card tutorial.
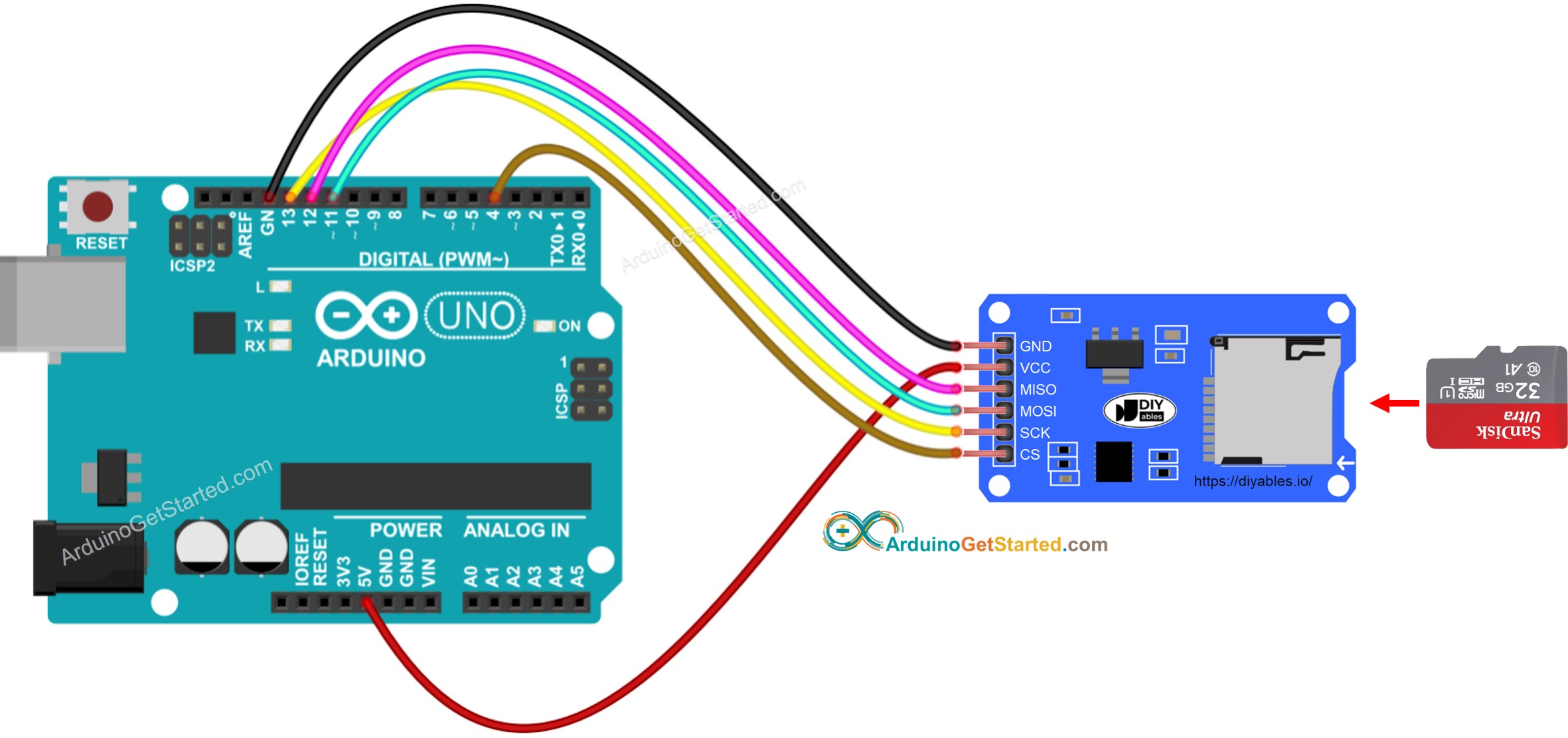
This image is created using Fritzing. Click to enlarge image
※ NOTE THAT:
If you use an Ethernet shield or any shield that has a Micro SD Card Holder, you do not need to use the Micro SD Card Module. You just need to insert the Micro SD Card to the Micro SD Card Holder on the shield.
The below code does:
Write a int variable to Micro SD Card
Write a float variable to Micro SD Card
Write a string variable to Micro SD Card
Write a char array to Micro SD Card
Write a byte array to Micro SD Card
#include <SD.h>
#define PIN_SPI_CS 4
File myFile;
int myInt = -52;
float myFloat = -12.7;
String myString = "HELLO";
char myCharArray[] = "ArduinoGetStarted.com";
byte myByteArray[] = {'1', '2', '3', '4', '5'};
void setup() {
Serial.begin(9600);
if (!SD.begin(PIN_SPI_CS)) {
Serial.println(F("SD CARD FAILED, OR NOT PRESENT!"));
while (1);
}
Serial.println(F("SD CARD INITIALIZED."));
Serial.println(F("--------------------"));
SD.remove("arduino.txt");
myFile = SD.open("arduino.txt", FILE_WRITE);
if (myFile) {
myFile.println(myInt);
myFile.println(myFloat);
myFile.println(myString);
myFile.println(myCharArray);
myFile.write(myByteArray, 5);
myFile.write("\n");
for (int i = 0; i < 5; i++) {
myFile.write(myByteArray[i]);
if (i < 4)
myFile.write(",");
}
myFile.write("\n");
myFile.close();
} else {
Serial.print(F("SD Card: error on opening file arduino.txt"));
}
myFile = SD.open("arduino.txt", FILE_READ);
if (myFile) {
while (myFile.available()) {
char ch = myFile.read();
Serial.print(ch);
}
myFile.close();
} else {
Serial.print(F("SD Card: error on opening file arduino.txt"));
}
}
void loop() {
}
Make sure that the Micro SD Card is formatted FAT16 or FAT32 (Google for it)
Copy the above code and open with Arduino IDE
Click Upload button on Arduino IDE to upload code to Arduino
See the result on Serial Monitor.
SD CARD INITIALIZED.
--------------------
-52
-12.70
HELLO
ArduinoGetStarted.com
12345
1,2,3,4,5
Detach the Micro SD Card from the Micro SD Card module
Insert the Micro SD Card to an USB SD Card reader
Connect the USB SD Card reader to the PC
Open the arduino.txt file on your PC, it looks like below
#include <SD.h>
#define PIN_SPI_CS 4
File myFile;
int myInt = -52;
float myFloat = -12.7;
String myString = "HELLO";
char myCharArray[] = "ArduinoGetStarted.com";
byte myByteArray[] = {'1', '2', '3', '4', '5'};
void setup() {
Serial.begin(9600);
if (!SD.begin(PIN_SPI_CS)) {
Serial.println(F("SD CARD FAILED, OR NOT PRESENT!"));
while (1);
}
Serial.println(F("SD CARD INITIALIZED."));
Serial.println(F("--------------------"));
SD.remove("arduino.txt");
myFile = SD.open("arduino.txt", FILE_WRITE);
if (myFile) {
myFile.print("myInt=");
myFile.println(myInt);
myFile.print("myFloat=");
myFile.println(myFloat);
myFile.print("myString=");
myFile.println(myString);
myFile.print("myCharArray=");
myFile.println(myCharArray);
myFile.print("myByteArray=");
myFile.write(myByteArray, 5);
myFile.write("\n");
myFile.print("myByteArray2=");
for (int i = 0; i < 5; i++) {
myFile.write(myByteArray[i]);
if (i < 4)
myFile.write(",");
}
myFile.write("\n");
myFile.close();
} else {
Serial.print(F("SD Card: error on opening file arduino.txt"));
}
myFile = SD.open("arduino.txt", FILE_READ);
if (myFile) {
while (myFile.available()) {
char ch = myFile.read();
Serial.print(ch);
}
myFile.close();
} else {
Serial.print(F("SD Card: error on opening file arduino.txt"));
}
}
void loop() {
}
Copy the above code and open with Arduino IDE
Click Upload button on Arduino IDE to upload code to Arduino
See the result on Serial Monitor.
SD CARD INITIALIZED.
--------------------
myInt=-52
myFloat=-12.70
myString=HELLO
myCharArray=ArduinoGetStarted.com
myByteArray=12345
myByteArray2=1,2,3,4,5
Detach the Micro SD Card from the Micro SD Card module
Insert the Micro SD Card to an USB SD Card reader
Connect the USB SD Card reader to the PC
Open the arduino.txt file on your PC, it looks like below
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.
※ OUR MESSAGES
You can share the link of this tutorial anywhere. Howerver, please do not copy the content to share on other websites. We took a lot of time and effort to create the content of this tutorial, please respect our work!