Arduino - LDR Module
The LDR light sensor module is capable of detecting and measuring light in the surrounding environment. The module provides two outputs: a digital output (LOW/HIGH) and an analog output.
In this tutorial, we will learn how to use an Arduino and an LDR light sensor module to detect and measure the light level. Specifically, we will cover the following:
- How to connect the LDR light sensor module to an Arduino.
- How to program the Arduino to detect light by reading the digital signal from the LDR light sensor module.
- How to program the Arduino to measure the light level by reading the analog signal from the LDR light sensor module.
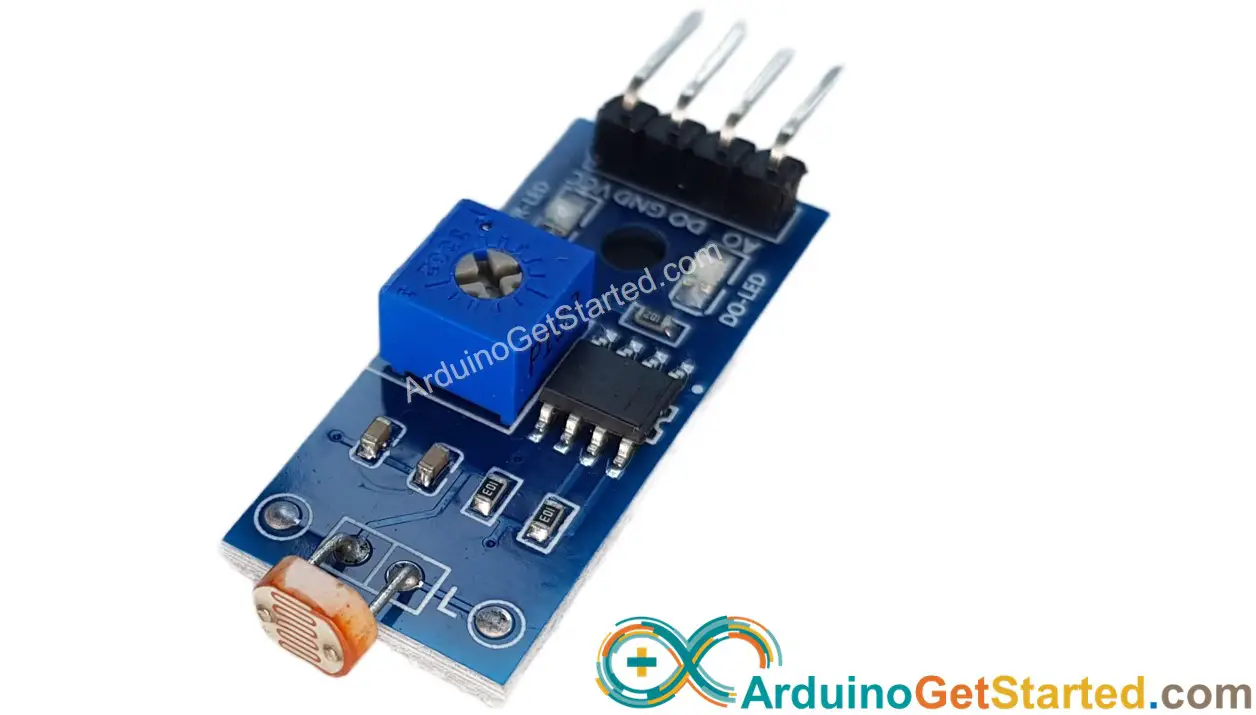
Afterward, you can modify the code to activate an LED or a light bulb (via a relay) when it detects light.
If you prefer a light sensor in its raw form, I suggest exploring the tutorial on the Arduino - Light Sensor.
Hardware Required
Or you can buy the following kits:
1 | × | DIYables STEM V3 Starter Kit (Arduino included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About LDR Light Sensor Module
The LDR light sensor module can be utilized to detect the presence of light or measure the light level in the surrounding environment. It provides two options via a digital output pin and analog output pin.
Pinout
The LDR light sensor module includes four pins:
- VCC pin: It needs to be connected to VCC (3.3V to 5V).
- GND pin: It needs to be connected to GND (0V).
- DO pin: It is a digital output pin. It is HIGH if it is dark and LOW if it is light. The threshold value between darkness and lightness can be adjusted using a built-in potentiometer.
- AO pin: It is an analog output pin. The output value decreases as the light gets brighter, and it increases as the light gets darker.
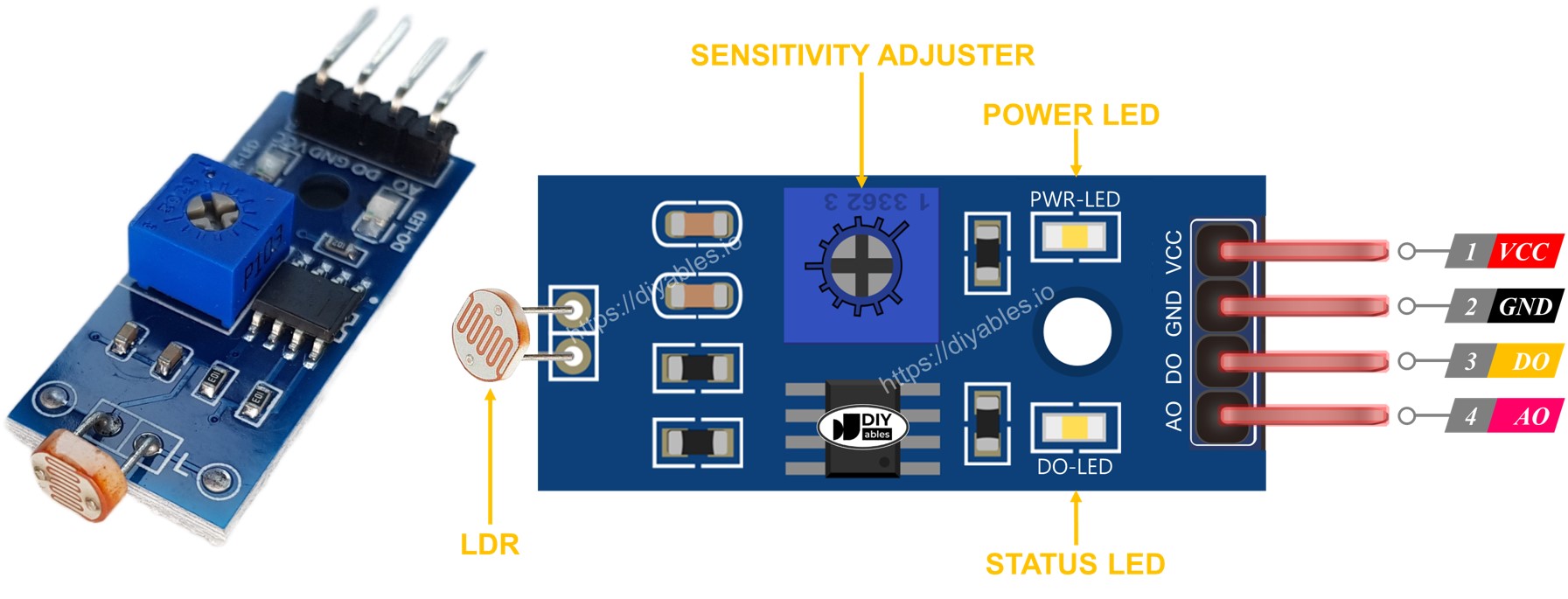
Furthermore, it has two LED indicators:
- One PWR-LED indicator for power.
- One DO-LED indicator for the light state on the DO pin: it is on when light is present and off when it is dark.
How It Works
For the DO pin:
- The module has a built-in potentiometer for setting the light threshold (sensitivity).
- When the light intensity in the surrounding environment is above the threshold value (light), the output pin of the sensor is LOW, and the DO-LED is on.
- When the light intensity in the surrounding environment is below the threshold value (dark), the output pin of the sensor is HIGH, and the DO-LED is off.
For the AO pin:
- The higher the light intensity in the surrounding environment (light), the lower the value read from the AO pin.
- The lower the light intensity in the surrounding environment (dark), the higher the value read from the AO pin.
Note that the potentiometer does not affect the value on the AO pin.
Wiring Diagram
Since the light sensor module has two outputs, you can choose to use one or both of them, depending on what you need.
- The wiring diagram between Arduino and the LDR light sensor module when using DO only.
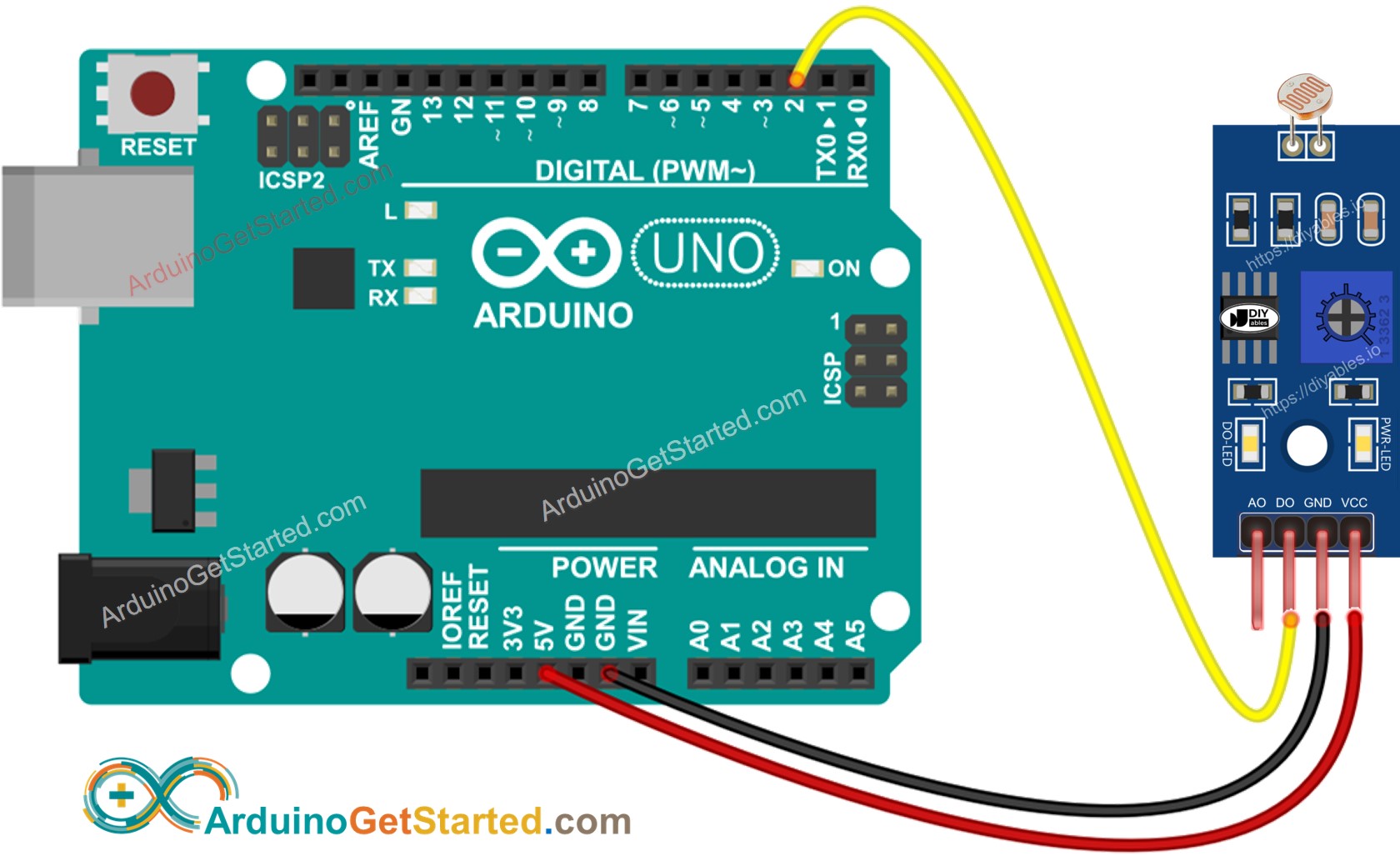
This image is created using Fritzing. Click to enlarge image
- The wiring diagram between Arduino and the LDR light sensor module when using AO only.
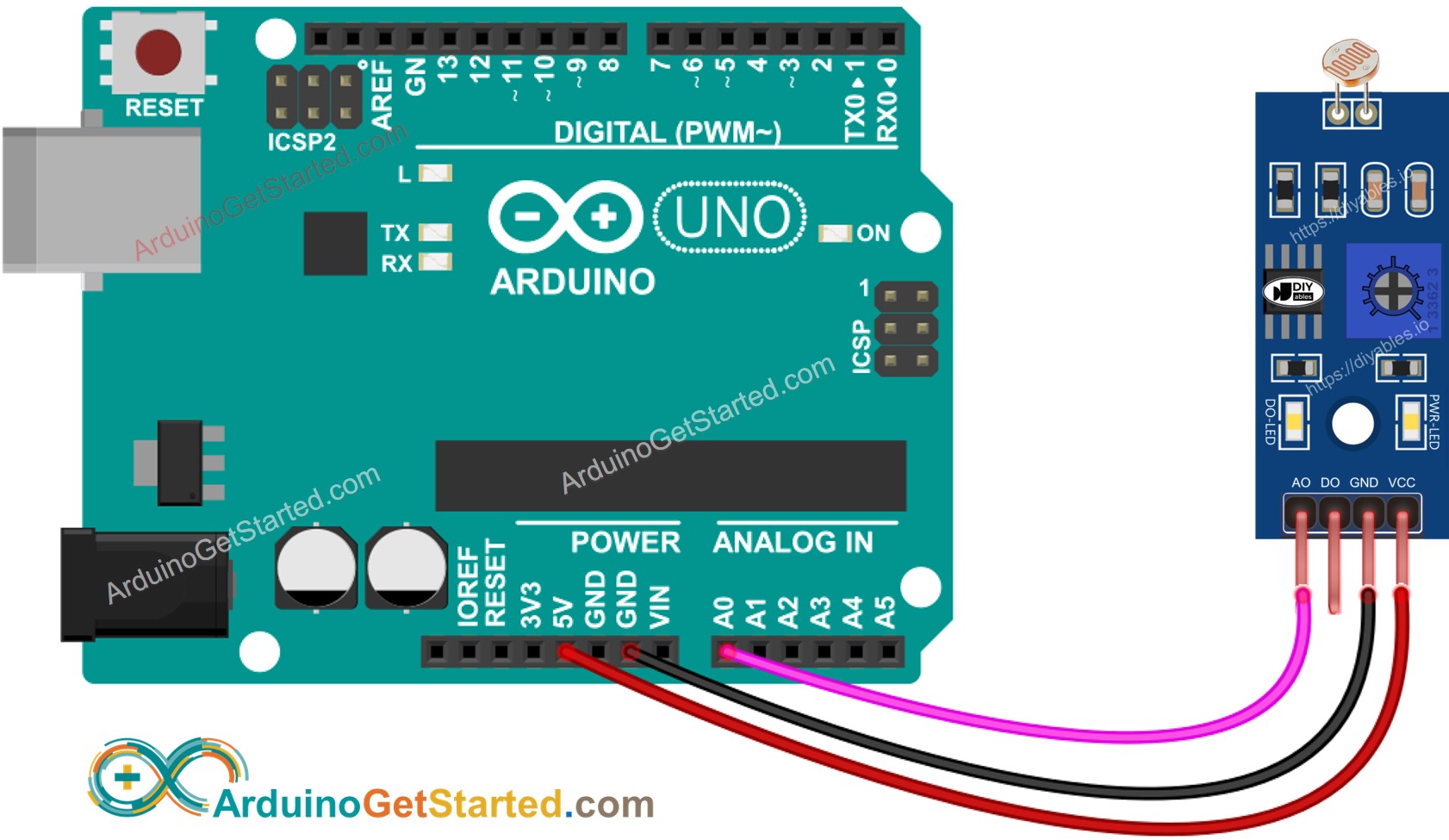
This image is created using Fritzing. Click to enlarge image
- The wiring diagram between Arduino and the LDR light sensor module when using both AO an DO.
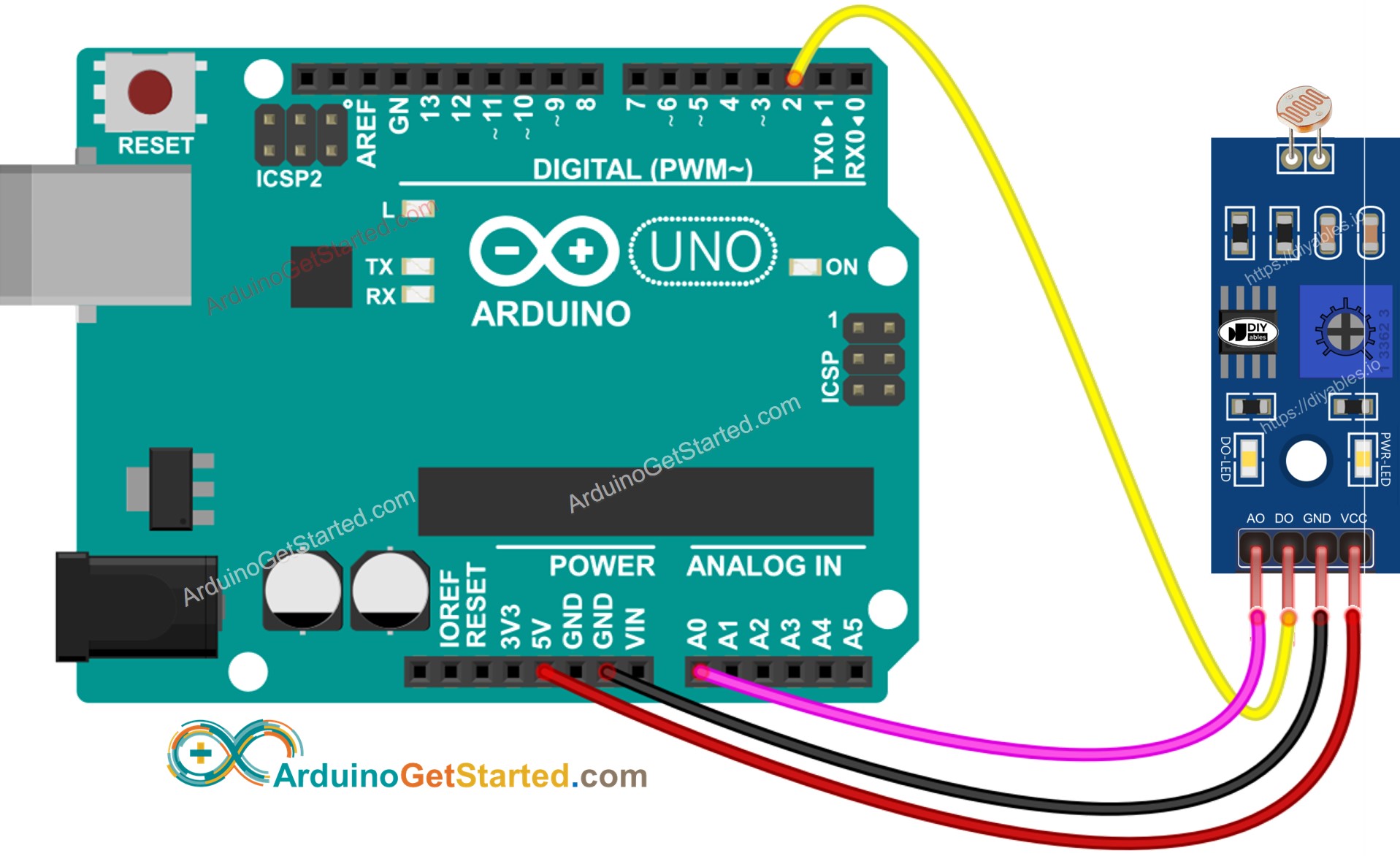
This image is created using Fritzing. Click to enlarge image
The real wiring:
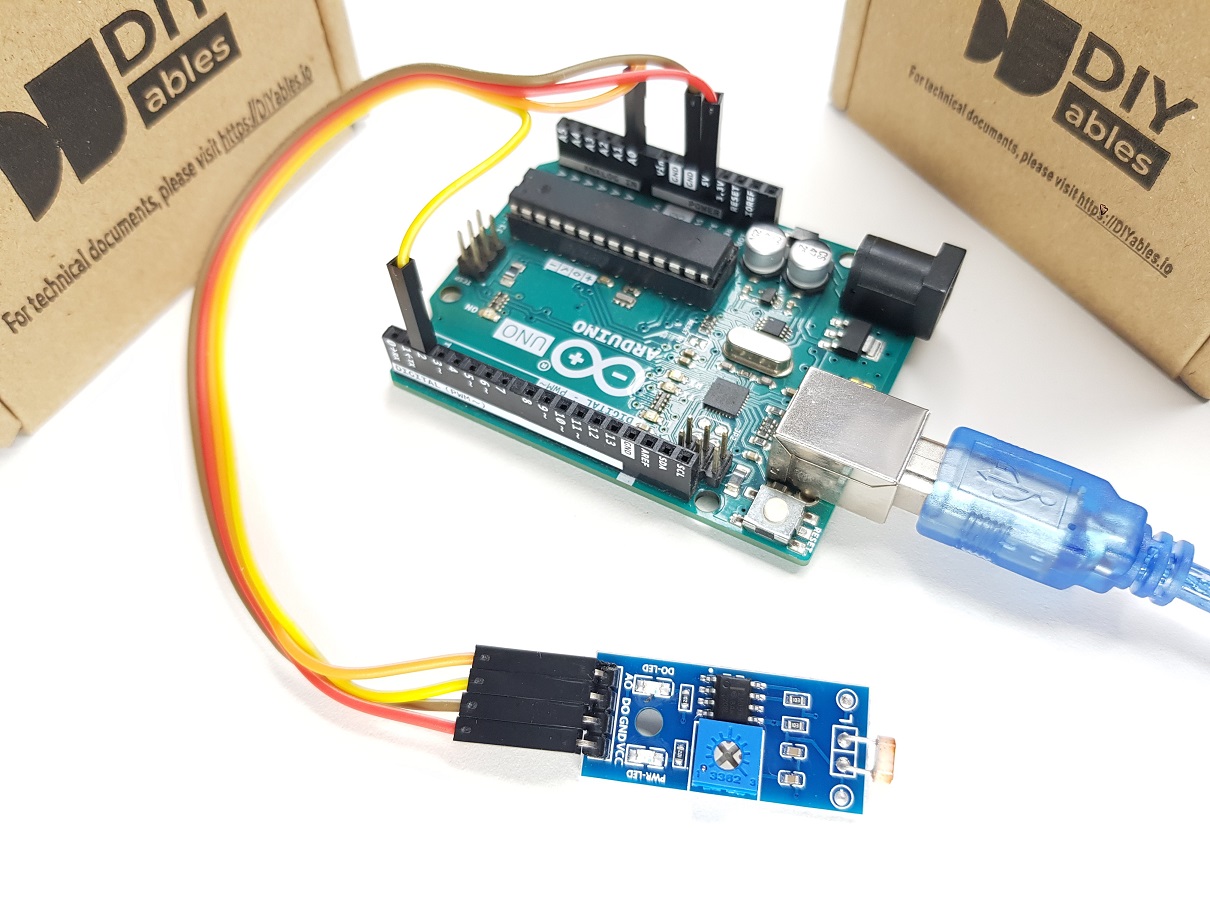
Arduino Code - Read value from DO pin
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Cover and uncover the LDR light sensor module by your hand or something
- See the result on Serial Monitor.
Please keep in mind that if you notice the LED status remaining on constantly or off even when there is light, you can adjust the potentiometer to fine-tune the light sensitivity of the sensor.
Now we can customize the code to activate an LED or a light when the light is detected, or even make a servo motor rotate. You can find more information and step-by-step instructions in the tutorials provided at the end of this tutorial.
Arduino Code - Read value from AO pin
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Cover and uncover the LDR light sensor module by your hand or something
- See the result on Serial Monitor.
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.