Arduino - RFID/NFC Door Lock System
In this tutorial, we are going to learn how to make Arduino RFID/NFC Door Lock System using Arduino, RFID/NFC RC522 Kit, and electromagnetic lock or solenoid lock.
※ NOTE THAT:
We also have others Arduino - Door lock system using keypad tutorials.
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About RFID/NFC RC522 Module and Electromagnetic Lock
If you do not know about RFID/NFC RC522 Module, Electromagnetic Lock and Solenoid Lock (pinout, how it works, how to program ...), learn about them in the following tutorials:
System Components
The door lock system includes two main parts:
- Door Lock: Arduino + RFID/NFC reader + Electromagnetic lock
- Door Key: RFID/NFC tags
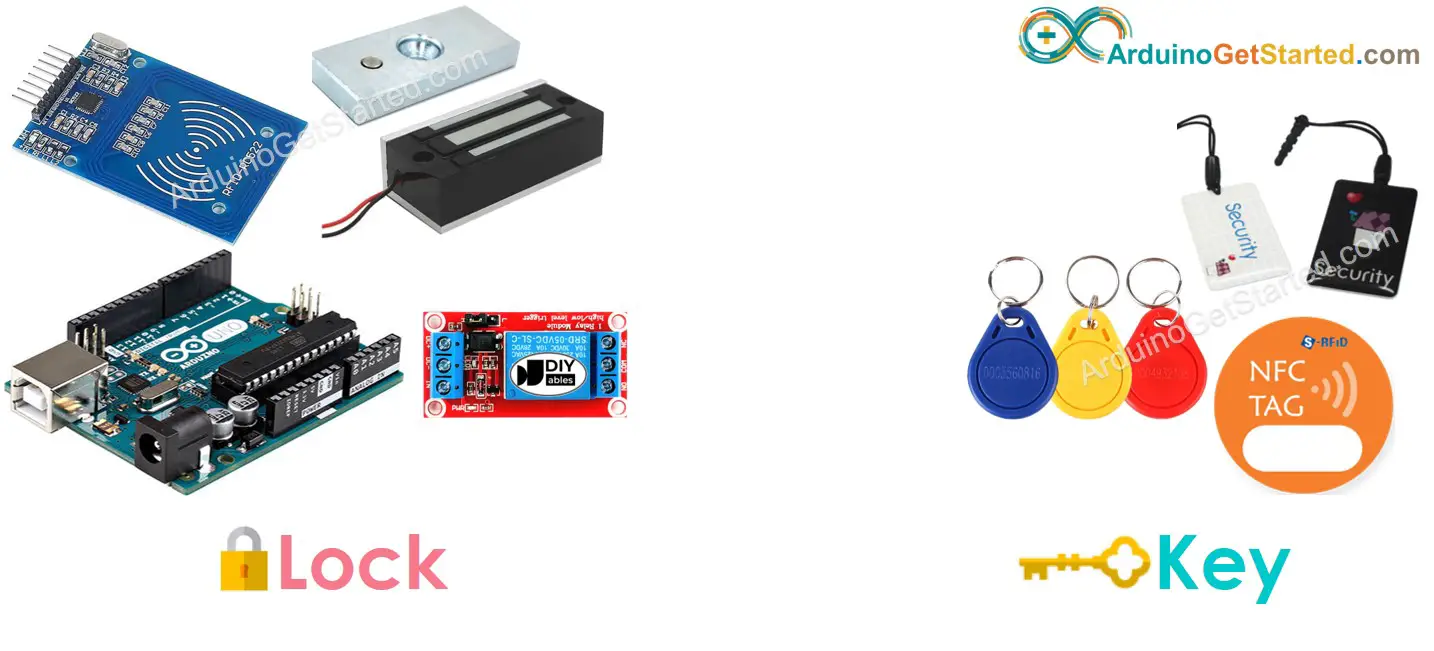
There are some types of RFID/NFC readers that can work with Arduino. This tutorial uses RFID-RC522 as an RFID/NFC reader because it is cheap.
How RFID/NFC Door Lock Works
- Some tags that have UIDs set in Arduino code acts as the authorized keys
- User taps an RFID/NFC tag on RFID/NFC reader
- The reader reads UID from the tag.
- Arduino gets the UID from the reader
- Arduino compares the read UID with the predefined UIDs
- If the UID is matched with one of the predefined UIDs, Arduino deactivates the electromagnetic lock to unlock the door.
Wiring Diagram
- RFID RC522 Door Lock with Solenoid Lock
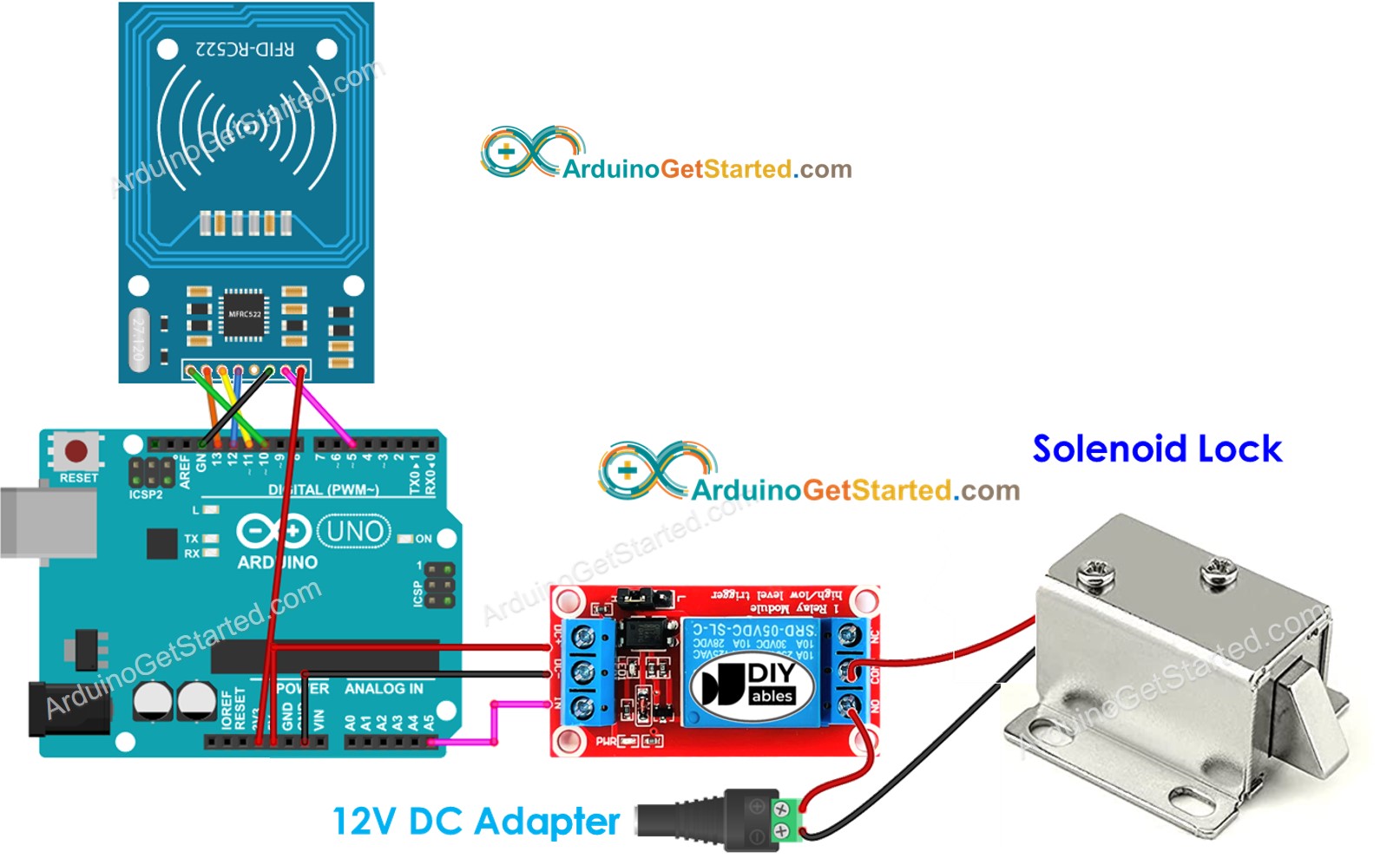
This image is created using Fritzing. Click to enlarge image
- RFID RC522 Door Lock with Electromagnetic Lock
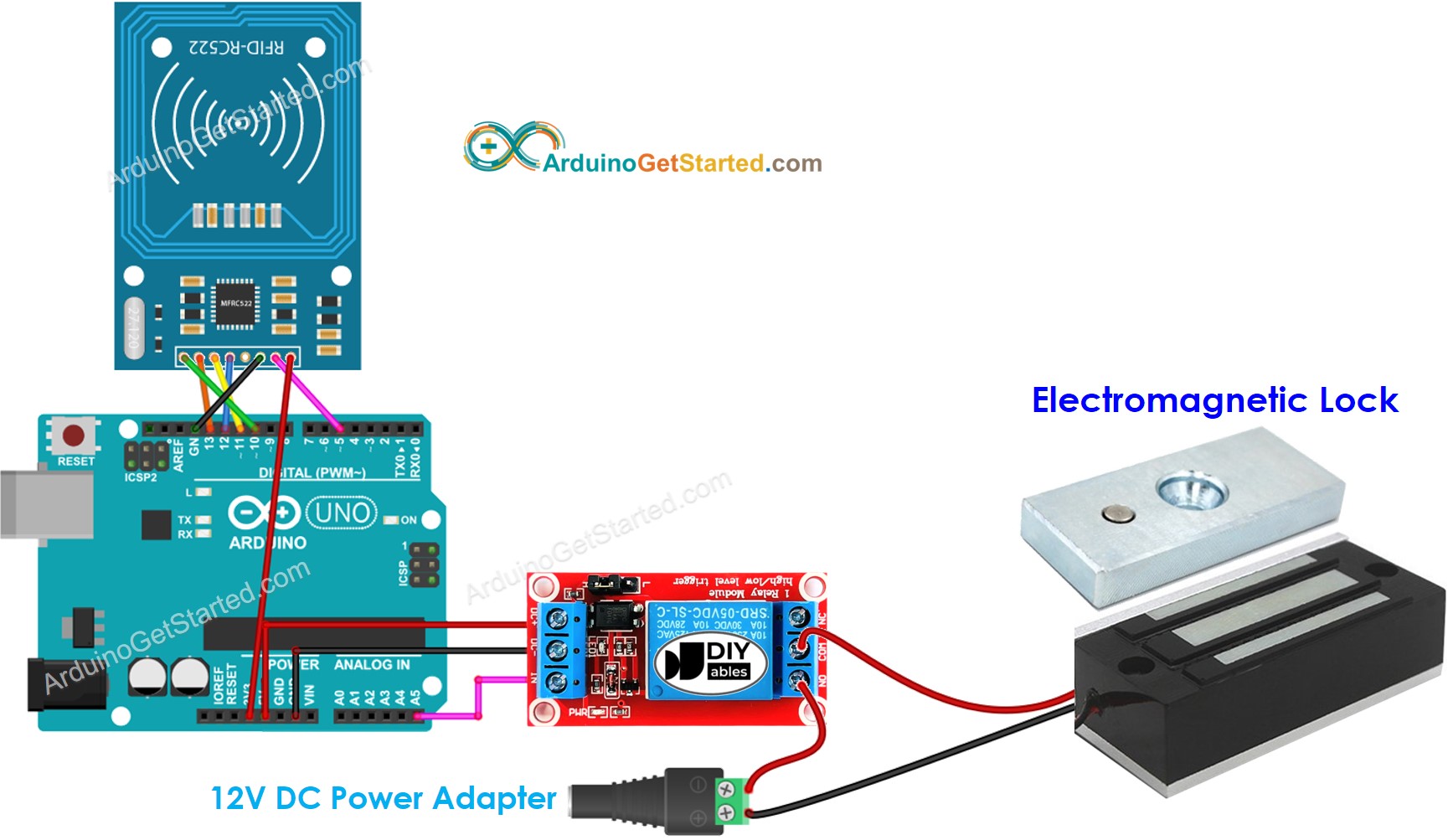
This image is created using Fritzing. Click to enlarge image
To make it simple, the RC522 module's pins are directly connected to the Arduino's pins. However, this may cause the Arduino to stop functioning properly in certain cases since the output pins of the Arduino produce 5V, whereas the pins of the RC522 module function normally at 3.3V. Therefore, it is recommended to regulate the voltage between the Arduino pins and the RC522 module's pins. For more detailed instructions, please refer to the Arduino - RFID RC522 tutorial. The diagram below illustrates how to regulate 5V to 3.3V using resistors:
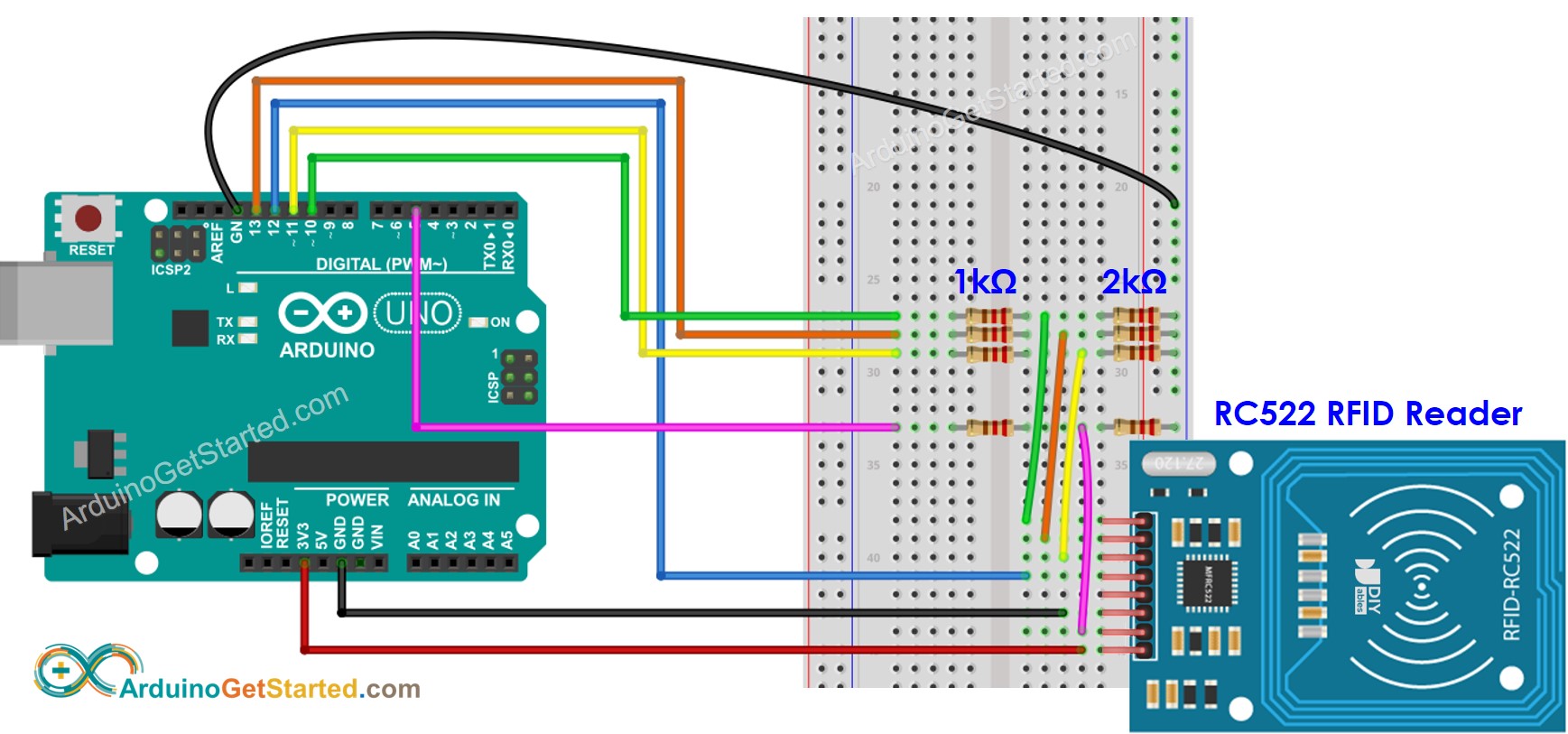
This image is created using Fritzing. Click to enlarge image
※ NOTE THAT:
The order of pins can vary according to manufacturers. ALWAYS use the labels printed on the module. The above image shows the pinout of the modules from DIYables manufacturer.
Arduino Code - Single Key
Quick Steps
Because UID is usually not printed on RFID/NFC tag, The first step we need to do is to find out the tag's UID. This can be done by:
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Open Serial Monitor
- Tap an RFID/NFC tag on RFID-RC522 module
- Get UID on Serial Monitor
After having UID:
- Update UID in the line 18 of the above code. For example, change byte keytagUID[4] = {0xFF, 0xFF, 0xFF, 0xFF}; TO byte keytagUID[4] = {0x3A, 0xC9, 0x6A, 0xCB};
- Upload the code to Arduino again
- Tap an RFID/NFC tag on RFID-RC522 module
- See output on Serial Monitor
- Check the electromagnetic lock, it should be unlocked
- Tap another RFID/NFC tag on RFID-RC522 module
- See output on Serial Monitor
※ NOTE THAT:
- To make it easy to test, the unlocked time is set to 2 seconds, it should be increased in practical use/demonstration
- It need to install MFRC522 library. See Arduino - RFID/NFC RC522 tutorial
Arduino Code - Multiple Keys
Let's imagine that a room allows the manager and secretary to unlock the door only.
In this case, we need to use two RFID/NFC tag: one for the manager and the other for the secretary. We have to specify the UIDs of two tags in the code
Do the similar steps as the above, and then tap one by one tag on RFID-RC522 module. The result on Serial Monitor looks like below:
You can extend the above code for three, four, or more tags.
※ NOTE THAT:
In the above code, the keys are hard-coded. In the practice, it should be able to add/delete keys dynamically via a special mode. If so, the keys should be saved on EEPROM memory. The number of keys can be saved depends on the EEPROM's size. The code will become complicated. If you want to build such system, we provide a coding service. Please feel free to contact us.
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.