Arduino - Log Data with Timestamp to SD Card
In this tutorial, we are going to learn how to write log with timestamp to the Micro SD Card using Arduino. In detail, we will learn:
Arduino - How to log data with timestamp a file on Micro SD Card
Arduino - How to log data with timestamp a to multiple files on Micro SD Card , one file per day
The time information is get from a RTC module and written to Micro SD Card along with data.
The data that is logged to the Micro SD Card can be anything. For example:
To be simple, this tutorial reads value from two analog pins as an example of data. You can easily to adapt the code for any kind of data.
Or you can buy the following kits:
Disclosure: Some links in this section are Amazon affiliate links. If you make a purchase through these links, we may earn a commission at no extra cost to you.
Additionally, some links direct to products from our own brand,
DIYables .
If you do not know about Micro SD Card Module and RTC module (pinout, how it works, how to program ...), learn about them in the following tutorials:
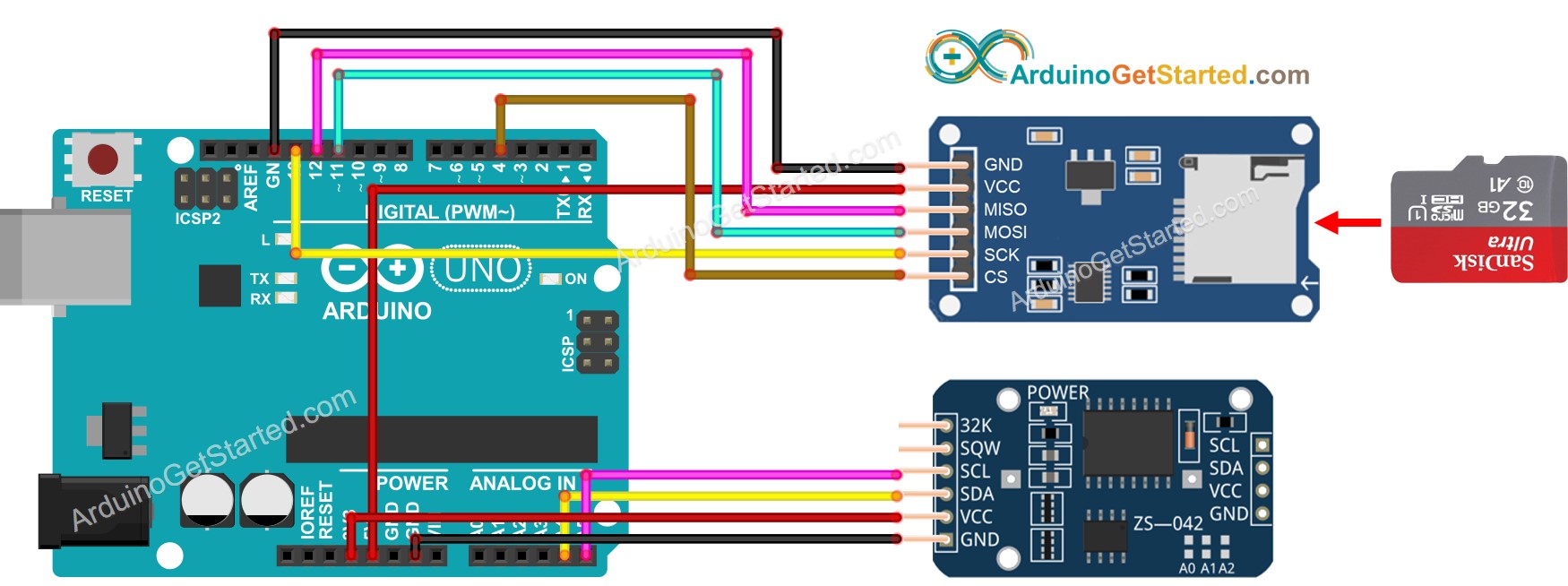
This image is created using Fritzing. Click to enlarge image
※ NOTE THAT:
If you use an Ethernet shield or any shield that has a Micro SD Card Holder, you do not need to use the Micro SD Card Module. You just need to insert the Micro SD Card to the Micro SD Card Holder on the shield.
#include <SD.h>
#include <RTClib.h>
#define PIN_SPI_CS 4
#define FILE_NAME "log.txt"
RTC_DS3231 rtc;
File myFile;
void setup() {
Serial.begin(9600);
if (!rtc.begin()) {
Serial.println(F("Couldn't find RTC"));
while (1);
}
if (!SD.begin(PIN_SPI_CS)) {
Serial.println(F("SD CARD FAILED, OR NOT PRESENT!"));
while (1);
}
Serial.println(F("SD CARD INITIALIZED."));
Serial.println(F("--------------------"));
}
void loop() {
myFile = SD.open(FILE_NAME, FILE_WRITE);
if (myFile) {
Serial.println(F("Writing log to SD Card"));
DateTime now = rtc.now();
myFile.print(now.year(), DEC);
myFile.print('-');
myFile.print(now.month(), DEC);
myFile.print('-');
myFile.print(now.day(), DEC);
myFile.print(' ');
myFile.print(now.hour(), DEC);
myFile.print(':');
myFile.print(now.minute(), DEC);
myFile.print(':');
myFile.print(now.second(), DEC);
myFile.print(" ");
int analog_1 = analogRead(A0);
int analog_2 = analogRead(A1);
myFile.print("analog_1 = ");
myFile.print(analog_1);
myFile.print(", ");
myFile.print("analog_2 = ");
myFile.print(analog_2);
myFile.write("\n");
myFile.close();
} else {
Serial.print(F("SD Card: error on opening file "));
Serial.println(FILE_NAME);
}
delay(2000);
}
Make sure that the Micro SD Card is formatted FAT16 or FAT32 (Google for it)
Copy the above code and open with Arduino IDE
Click Upload button on Arduino IDE to upload code to Arduino
See the result on Serial Monitor.
SD CARD INITIALIZED.
--------------------
Writing log to SD Card
Writing log to SD Card
Writing log to SD Card
Writing log to SD Card
Writing log to SD Card
Writing log to SD Card
Writing log to SD Card
Detach the Micro SD Card from the Micro SD Card module
Insert the Micro SD Card to an USB SD Card reader
Connect the USB SD Card reader to the PC
Open the log.txt file on your PC, it looks like below
If you do not have an USB SD Card reader, you can check the content of log file by running the below Arduino Code.
#include <SD.h>
#define PIN_SPI_CS 4
#define FILE_NAME "log.txt"
File myFile;
void setup() {
Serial.begin(9600);
if (!SD.begin(PIN_SPI_CS)) {
Serial.println(F("SD CARD FAILED, OR NOT PRESENT!"));
while (1);
}
Serial.println(F("SD CARD INITIALIZED."));
myFile = SD.open(FILE_NAME, FILE_READ);
if (myFile) {
while (myFile.available()) {
char ch = myFile.read();
Serial.print(ch);
}
myFile.close();
} else {
Serial.print(F("SD Card: error on opening file "));
Serial.println(FILE_NAME);
}
}
void loop() {
}
Writing log to a single file results in a big file size overtime and makes it difficult to check. The below code will write log file in multiple:
#include <SD.h>
#include <RTClib.h>
#define PIN_SPI_CS 4
RTC_DS3231 rtc;
File myFile;
char filename[] = "yyyymmdd.txt";
void setup() {
Serial.begin(9600);
if (!rtc.begin()) {
Serial.println(F("Couldn't find RTC"));
while (1);
}
if (!SD.begin(PIN_SPI_CS)) {
Serial.println(F("SD CARD FAILED, OR NOT PRESENT!"));
while (1);
}
Serial.println(F("SD CARD INITIALIZED."));
Serial.println(F("--------------------"));
}
void loop() {
DateTime now = rtc.now();
int year = now.year();
int month = now.month();
int day = now.day();
filename[0] = (year / 1000) + '0';
filename[1] = ((year % 1000) / 100) + '0';
filename[2] = ((year % 100) / 10) + '0';
filename[3] = (year % 10) + '0';
filename[4] = (month / 10) + '0';
filename[5] = (month % 10) + '0';
filename[6] = (day / 10) + '0';
filename[7] = (day % 10) + '0';
myFile = SD.open(filename, FILE_WRITE);
if (myFile) {
Serial.println(F("Writing log to SD Card"));
myFile.print(now.year(), DEC);
myFile.print('-');
myFile.print(now.month(), DEC);
myFile.print('-');
myFile.print(now.day(), DEC);
myFile.print(' ');
myFile.print(now.hour(), DEC);
myFile.print(':');
myFile.print(now.minute(), DEC);
myFile.print(':');
myFile.print(now.second(), DEC);
myFile.print(" ");
int analog_1 = analogRead(A0);
int analog_2 = analogRead(A1);
myFile.print("analog_1 = ");
myFile.print(analog_1);
myFile.print(", ");
myFile.print("analog_2 = ");
myFile.print(analog_2);
myFile.write("\n");
myFile.close();
} else {
Serial.print(F("SD Card: error on opening file "));
Serial.println(filename);
}
delay(2000);
}
After a long run, If you:
Detach the Micro SD Card from the Micro SD Card module
Insert the Micro SD Card to an USB SD Card reader
Connect the USB SD Card reader to the PC
You will see the files as follows:
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.
※ OUR MESSAGES
You can share the link of this tutorial anywhere. Howerver, please do not copy the content to share on other websites. We took a lot of time and effort to create the content of this tutorial, please respect our work!