Arduino - Button - Long Press Short Press
We will learn:
- How to detect the button's short press
- How to detect the button's long press
- How to detect both the button's long press and short press
- Long press and short press with debouncing
In the first three parts, we learn how to detect in principle.
In the last part, we learn how to detect in practical use by applying the debounce. See why do we need to debounce for button. Without debouncing, we may detect wrong the button short press.
Hardware Required
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
Wiring Diagram
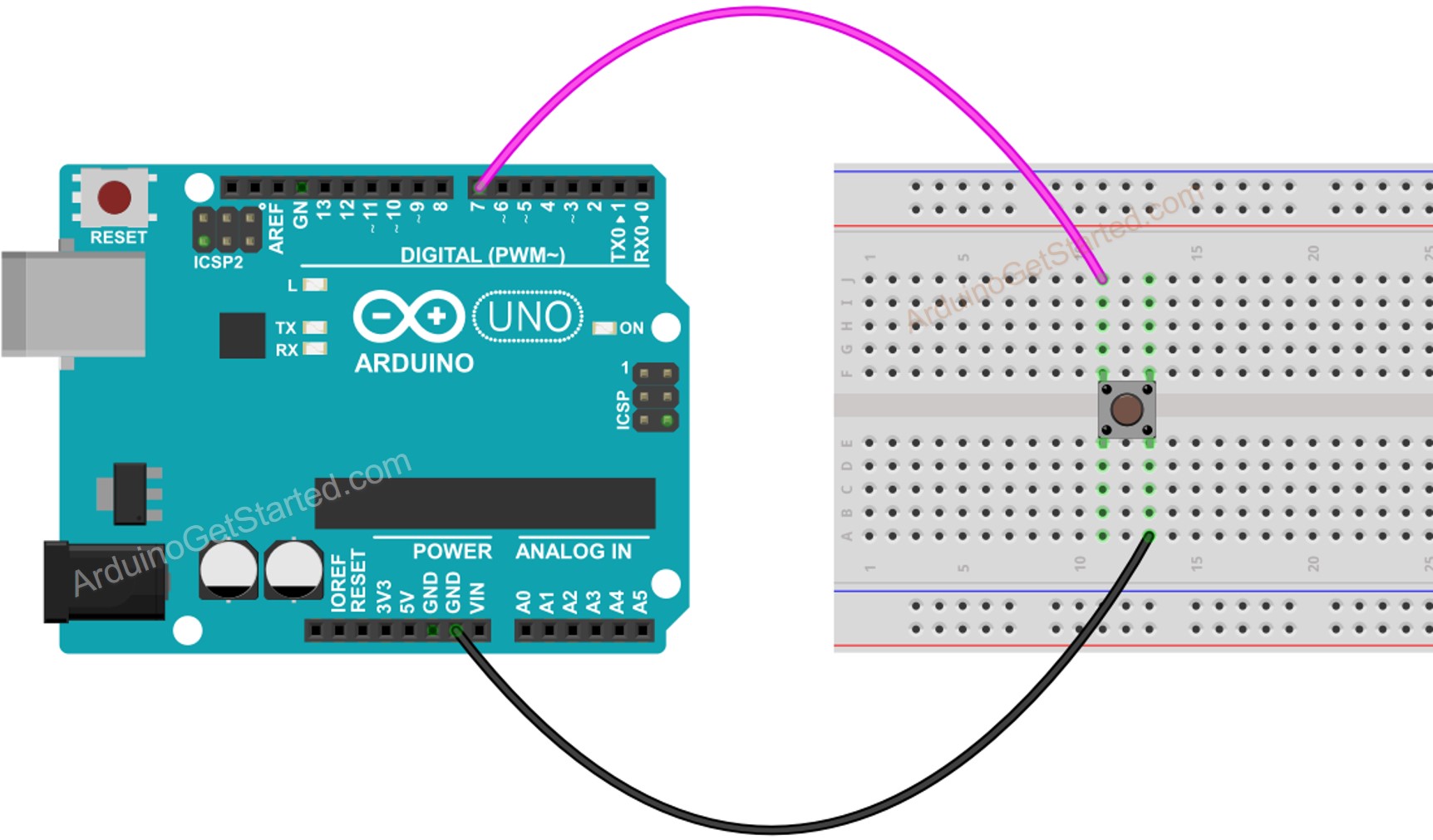
This image is created using Fritzing. Click to enlarge image
In this tutorial, we will use the internal pull-up resistor. Therefore, the state of the button is HIGH when normal and LOW when pressed.
How To Detect Short Press
We measure the time duration between the pressed and released events. If the duration is shorter than a defined time, the short press event is detected.
Let's see step by step:
- Define how long the maximum of short press lasts
- Detect the button is pressed and save the pressed time
- Detect the button is released and save the released time
- Calculate press duration and
- Determine the short press by comparing the press duration with the defined short press time.
Arduino Code for detecting the short press
Quick Steps
- Upload the above code to Arduino via Arduino IDE
- Press the button shortly several times.
- See the result on Serial Monitor
※ NOTE THAT:
The Serial Monitor may show several short press detection for one press. This is the normal behavior of the button. This behavior is called the “chattering phenomenon”. The issue will be solved in the last part of this tutorial.
How To Detect Long Press
There are two use cases for detecting the long press.
- The long-press event is detected right after the button is released
- The long-press event is detected during the time the button is being pressed, even the button is not released yet.
In the first use case, We measure the time duration between the pressed and released events. If the duration is longer than a defined time, the long-press event is detected.
In the second use case, After the button is pressed, We continuously measure the pressing time and check the long-press event until the button is released. During the time button is being pressed. If the duration is longer than a defined time, the long-press event is detected.
Arduino Code for detecting long press when released
Quick Steps
- Upload the above code to Arduino via Arduino IDE
- Press and release the button after one second.
- See the result on Serial Monitor
The long-press event is only detected right after the button is released
Arduino Code for detecting long press during pressing
Quick Steps
- Upload the above code to Arduino via Arduino IDE
- Press and release the button after several seconds.
- See the result on Serial Monitor
The long-press event is only detected when the button is not released yet
How To Detect Both Long Press and Short Press
Short Press and Long Press after released
Quick Steps
- Upload the above code to Arduino via Arduino IDE
- Long and short press the button.
- See the result on Serial Monitor
※ NOTE THAT:
The Serial Monitor may show several short press detection when long press. This is the normal behavior of the button. This behavior is called the “chattering phenomenon”. The issue will be solved in the last part of this tutorial.
Short Press and Long Press During pressing
Quick Steps
- Upload the above code to Arduino via Arduino IDE
- Long and short press the button.
- See the result on Serial Monitor
※ NOTE THAT:
The Serial Monitor may show several short press detection when long press. This is the normal behavior of the button. This behavior is called the “chattering phenomenon”. The issue will be solved in the last part of this tutorial.
Long Press and Short Press with Debouncing
It is very important to debounce the button in many applications.
Debouncing is a little complicated, especially when using multiple buttons. To make it much easier for beginners, we created a library, called ezButton.
We will use this library in below codes
Short Press and Long Press with debouncing after released
Quick Steps
- Install ezButton library. See How To
- Upload the above code to Arduino via Arduino IDE
- Long and short press the button.
- See the result on Serial Monitor
Short Press and Long Press with debouncing During Pressing
Quick Steps
- Install ezButton library. See How To
- Upload the above code to Arduino via Arduino IDE
- Long and short press the button.
- See the result on Serial Monitor
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.
Why Needs Long Press and Short Press
- To save the number of buttons. A single button can keep two or more functionalities. For example, short press for changing operation mode, long press for turn off the device.
- Use of long press to reduce the short press by accident. For example, some kinds of devices use the button for factory reset. If the button is pressed by accident, it is dangerous. To avoid it, the device is implemented to be factory reset only when the button is long-press (e.g over 5 seconds)