Arduino - RFID/NFC - Relay
In this tutorial, we are going to learn how to use RFID/NFC tag to activate relay using Arduino. You can extend this tutorial by using the relay to control motor, actuator...
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables.
About RFID/NFC RC522 Module and Relay
If you do not know about RFID/NFC RC522 Module and relay (pinout, how it works, how to program ...), learn about them in the following tutorials:
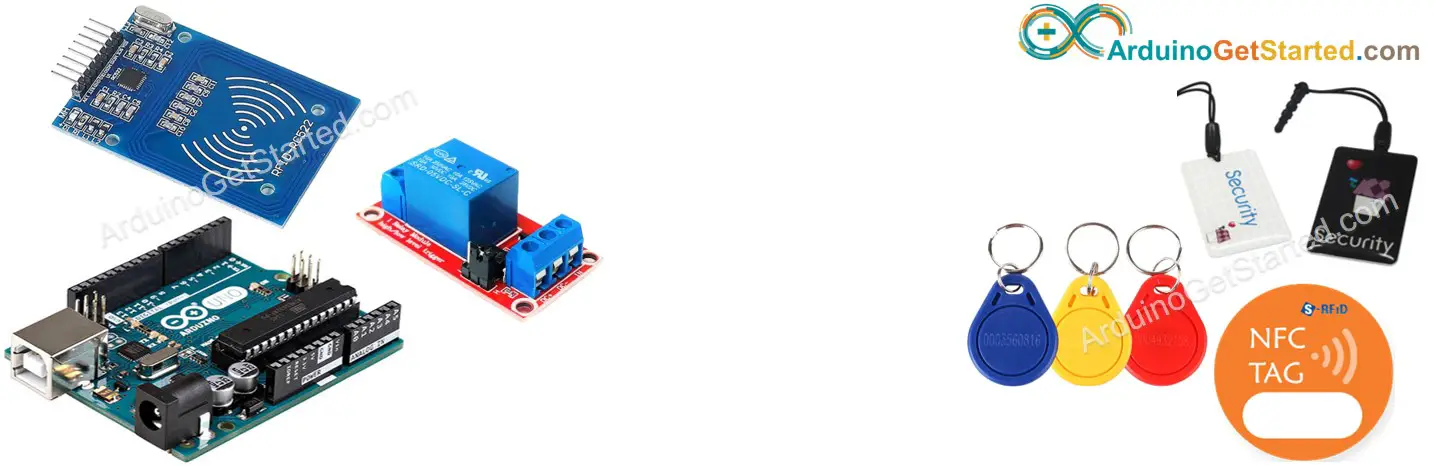
How It Works
- The UIDs of some RFID/NFC tags are preset in Arduino code
- User taps an RFID/NFC tag on RFID/NFC reader
- The reader reads UID from the tag.
- Arduino gets the UID from the reader
- Arduino compares the read UID with the predefined UIDs
- If the UID is matched with one of the predefined UIDs, Arduino activates the relay.
Wiring Diagram
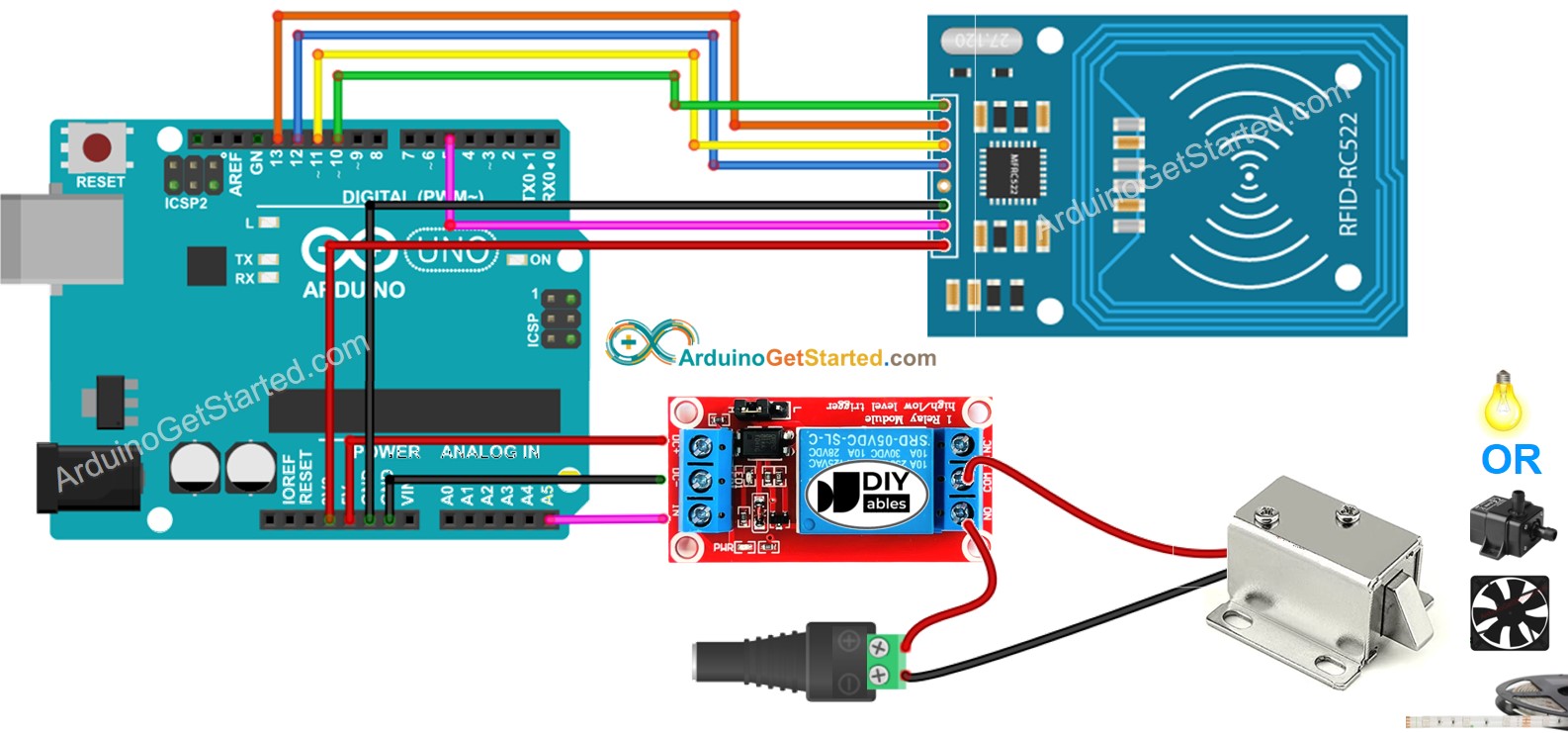
This image is created using Fritzing. Click to enlarge image
To simplify the setup, the pins of the RC522 module are directly connected to the pins of the Arduino. However, this can cause the Arduino to stop working in certain situations, as the output pins of the Arduino supply 5V while the RC522 module's pins are designed to function at 3.3V. It is therefore advisable to regulate the voltage between the Arduino pins and the RC522 module's pins. For additional information, please refer to the Arduino - RFID RC522 tutorial. The following diagram illustrates how to use resistors to regulate 5V to 3.3V:
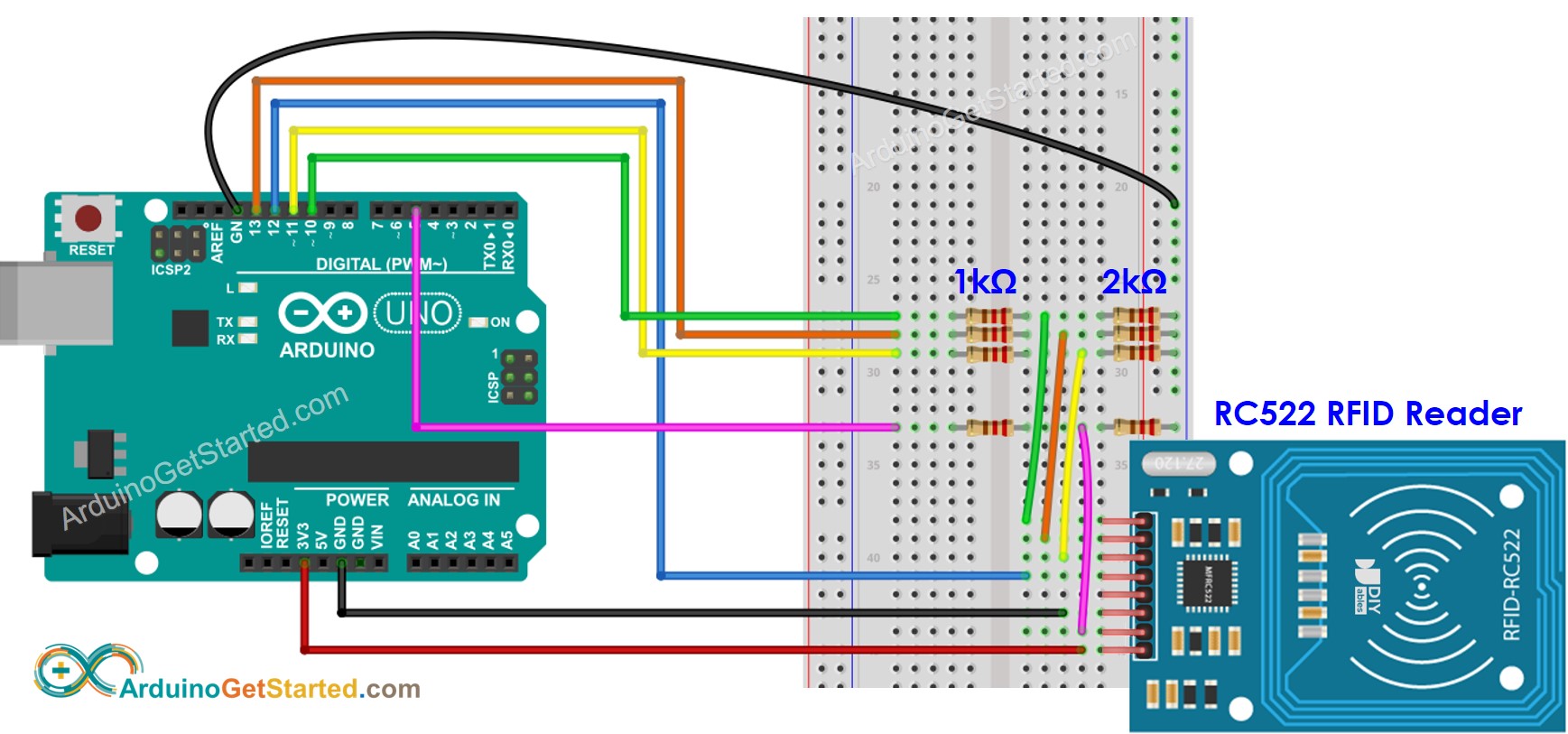
This image is created using Fritzing. Click to enlarge image
※ NOTE THAT:
The order of pins can vary according to manufacturers. ALWAYS use the labels printed on the module. The above image shows the pinout of the modules from DIYables manufacturer.
Arduino Code - Single RFID/NFC Tag
Quick Steps
Because UID is usually not printed on RFID/NFC tag, The first step we need to do is to find out the tag's UID. This can be done by:
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Open Serial Monitor
- Tap an RFID/NFC tag on RFID-RC522 module
- Get UID on Serial Monitor
After having UID:
- Update UID in the line 18 of the above code. For example, change byte authorizedUID[4] = {0xFF, 0xFF, 0xFF, 0xFF}; TO byte authorizedUID[4] = {0x3A, 0xC9, 0x6A, 0xCB};
- Upload the code to Arduino again
- Tap an RFID/NFC tag on RFID-RC522 module
- See output on Serial Monitor
- Tap another RFID/NFC tag on RFID-RC522 module
- See output on Serial Monitor
※ NOTE THAT:
- To make it easy to test, The active time is 2 seconds, it should be increased in practical use/demonstration
- It need to install MFRC522 library. See Arduino - RFID/NFC RC522 tutorial
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.