Arduino - 74HC595 4-Digit 7-Segment Display
A standard 4-digit 7-segment display is needed for clock, timer and counter projects, but it usually requires 12 connections. The 74HC595 module makes it easier by only requiring 5 connections: 2 for power and 3 for controlling the segments.
This tutorial will not overload you by deep driving into hardware. Instead, We will learn how to connect the 4-digit 7-segment display to Arduino, how to program it do display what we want.
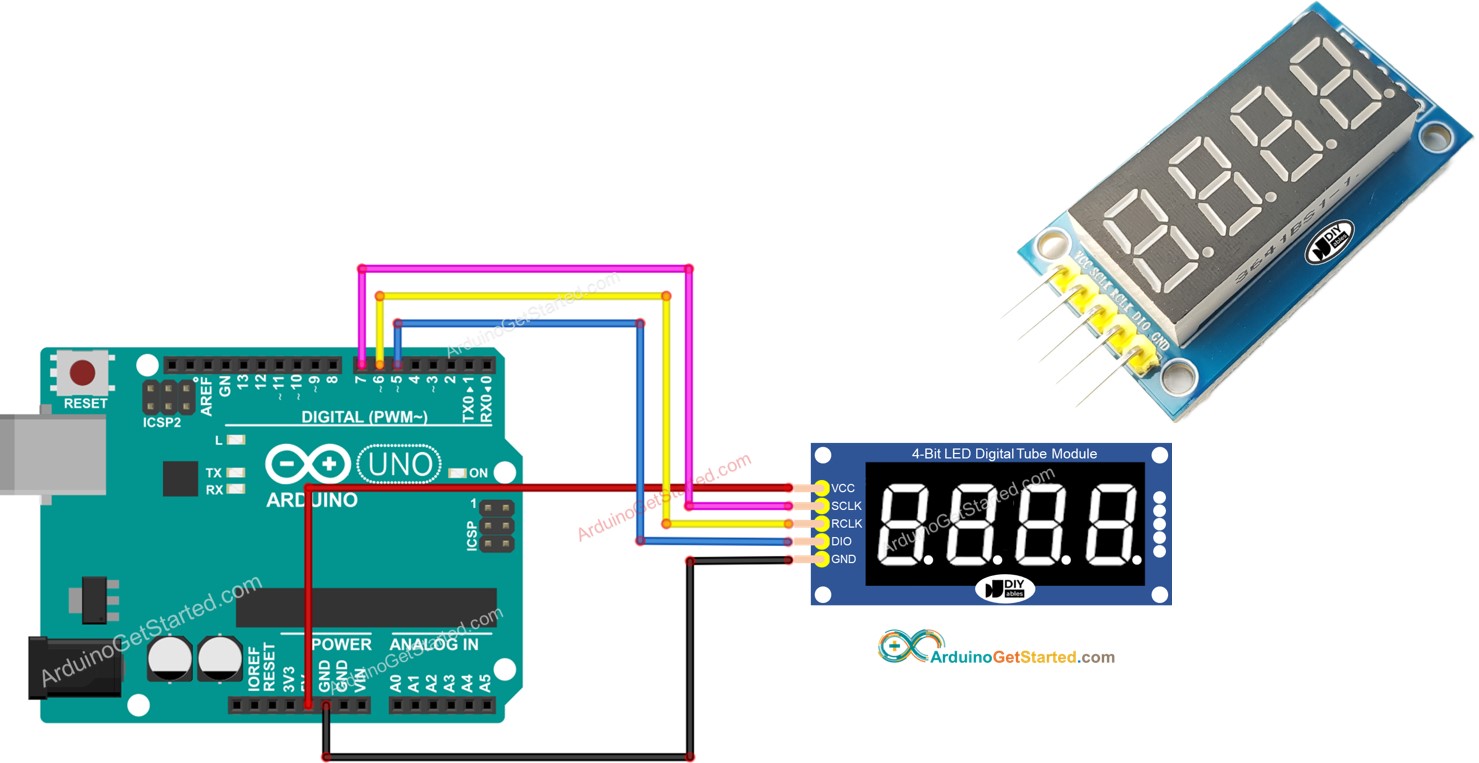
This tutorial are going to use the 4-dot 4-digit 7-segment display module that is able to displayed the float values. If you want to display a colon separator, please use the TM1637 4-digit 7-segment Display Module
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
About 74HC595 4-digit 7-segment Display
A 74HC595 4-digit 7-segment display module typically consists of 4 7-segment LEDs, 4 dot-shaped LEDs, and a 74HC595 driver for each digit: It is ideal for displaying the temperature or any decimal value.
Pinout
The 74HC595 4-digit 7-segment display module includes 5 pins:
- SCLK pin: is a clock input pin. Connect to any digital pin on Arduino.
- RCLK pin: is a clock input pin. Connect to any digital pin on Arduino.
- DIO pin: is a Data I/O pin. Connect to any digital pin on Arduino.
- VCC pin: supplies power to the module. Connect it to the 3.3V to 5V power supply.
- GND pin: is a ground pin.
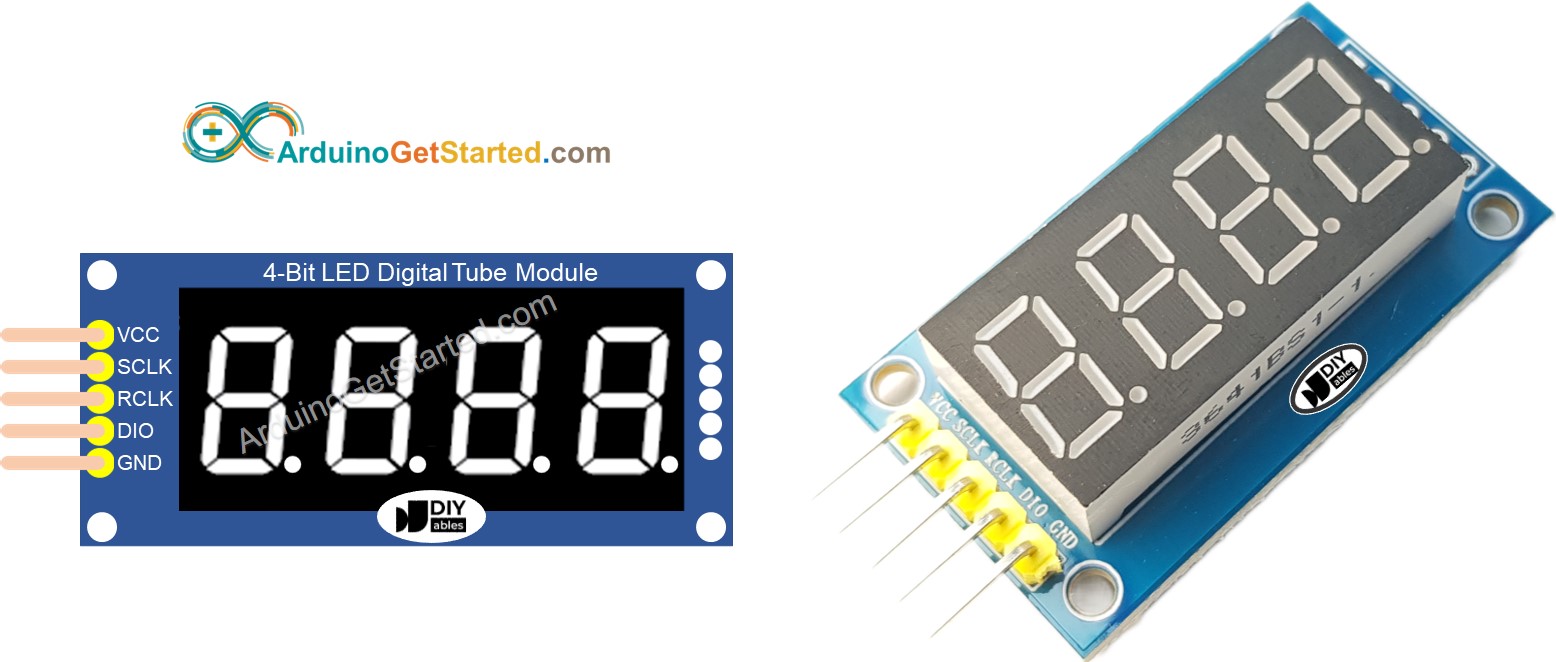
Wiring Diagram
The table below shown the wiring between Arduino pins and a 74HC595 4-digit 7-segment display pins:
Arduino | 74HC595 7-segment display |
---|---|
5V | 5V |
7 | SCLK |
6 | RCLK |
5 | DIO |
The pin numbers in the code should be changed if different pins are used.
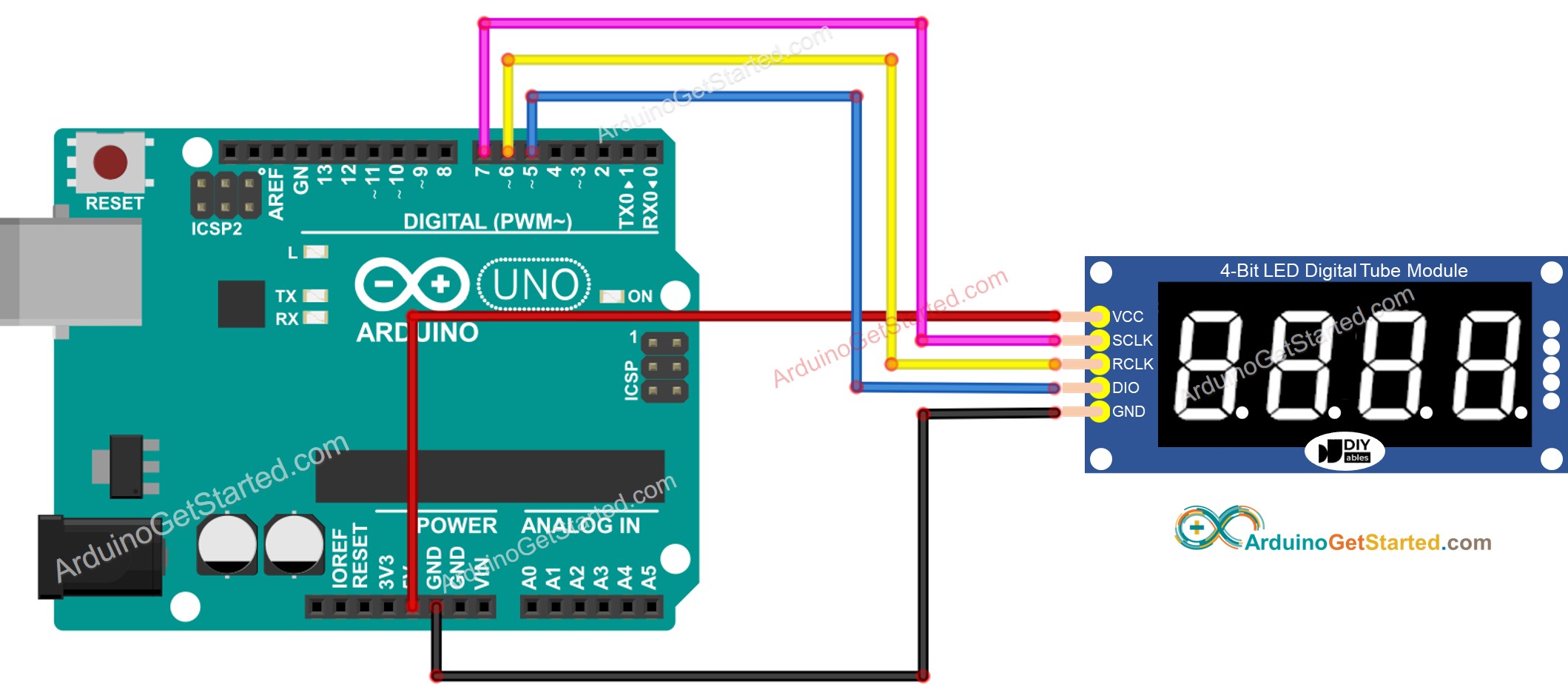
This image is created using Fritzing. Click to enlarge image
Library Installation
To program easily for 74HC595 4-digit 7-segment Display, we need to install DIYables_4Digit7Segment_74HC595 library by DIYables.io. Follow the below steps to install the library:
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search “DIYables_4Digit7Segment_74HC595”, then find the DIYables_4Digit7Segment_74HC595 library by DIYables.io
- Click Install button.
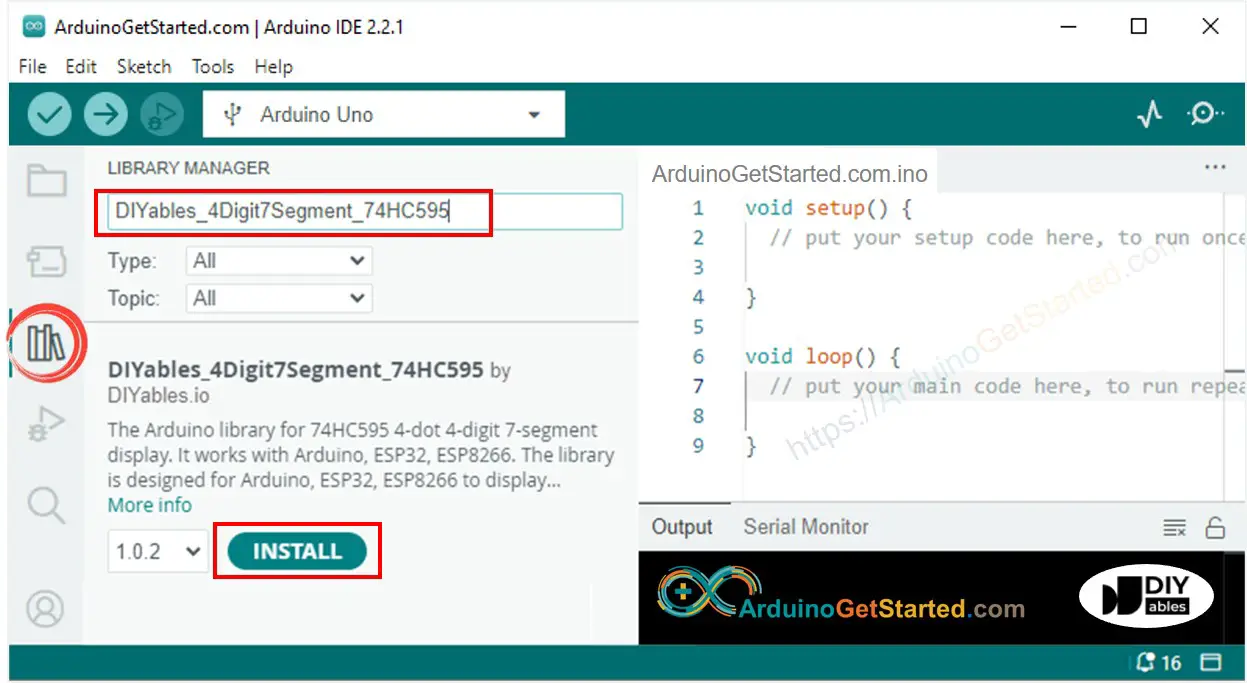
You can also see this library on Github
How To Program For 74HC595 4-digit 7-segment using Arduino
- Include the library
- Define Arduino's pins that connects to SCLK, RCLK and DIO of the display module. For example, pin D7, D6 and D5
- Create a display object of type DIYables_4Digit7Segment_74HC595
- Then you can display the integer numbers with the zero-padding option, supporting the negative number:
- You can display the float numbers with the decimal place, zero-padding options, supporting the negative number:
- You can also display number, decimal point, character digit-by-digit by using lower-level functions:
- Because the 74HC595 4-digit 7-segment module uses the multiplexing technique to control individual segments and LEDs, Arduino code MUST:
- Call display.show() function in the main loop
- Not use delay() function in the main loop
You can see more detail in the the library reference
Arduino Code - Display Integer
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- See the states of the 7-segment display
Arduino Code - Display Float
Arduino Code - Display Temperature
The result is as the below image:
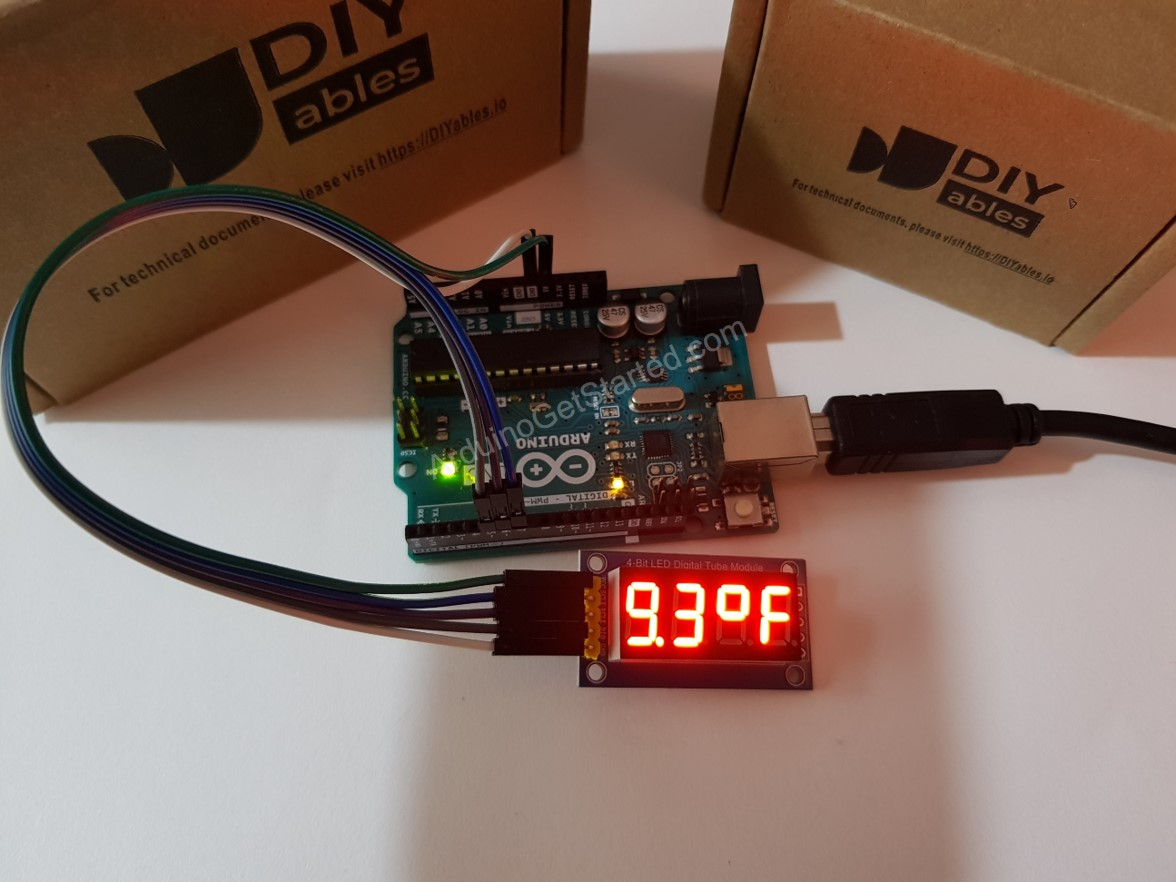
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.