Arduino - RTC
In this tutorial, we are going to learn:
How to get data and time (seconds, minutes, hours, day, date, month, and year) using Arduino and Real-Time Clock DS3231 Module.
How to make daily schedule with Arduino
How to make weekly schedule with Arduino
How to make schedule on speficic date with Arduino
Or you can buy the following sensor kits:
Disclosure: Some links in this section are Amazon affiliate links. If you make a purchase through these links, we may earn a commission at no extra cost to you.
Additionally, some links direct to products from our own brand,
DIYables .
Arduino itself has some time-related functions such as millis(), micros(). However, they can not provide the date and time (seconds, minutes, hours, day, date, month, and year). To get date and time, we needs to use a Real-Time Clock (RTC) module such as DS3231, DS1370. DS3231 Module has higher precision than DS1370. See DS3231 vs DS1307
Real-Time Clock DS3231 Module includes 10 pins:
32K pin: outputs the stable(temperature compensated) and accurate reference clock.
SQW pin: outputs a nice square wave at either 1Hz, 4kHz, 8kHz or 32kHz and can be handled programmatically. This can further be used as an interrupt due to alarm condition in many time-based applications.
SCL pin: is a serial clock pin for I2C interface.
SDA pin: is a serial data pin for I2C interface.
VCC pin: supplies power for the module. It can be anywhere between 3.3V to 5.5V.
GND pin: is a ground pin.
For normal use, it needs to use 4 pins: VCC, GND, SDA, SCL
The DS3231 Module also has a battery holder.
If we insert a CR2032 battery, It keeps the time on module running when the main power is off.
If we do not insert the battery, the time information is lost if the main power is off and you need to set the time again.
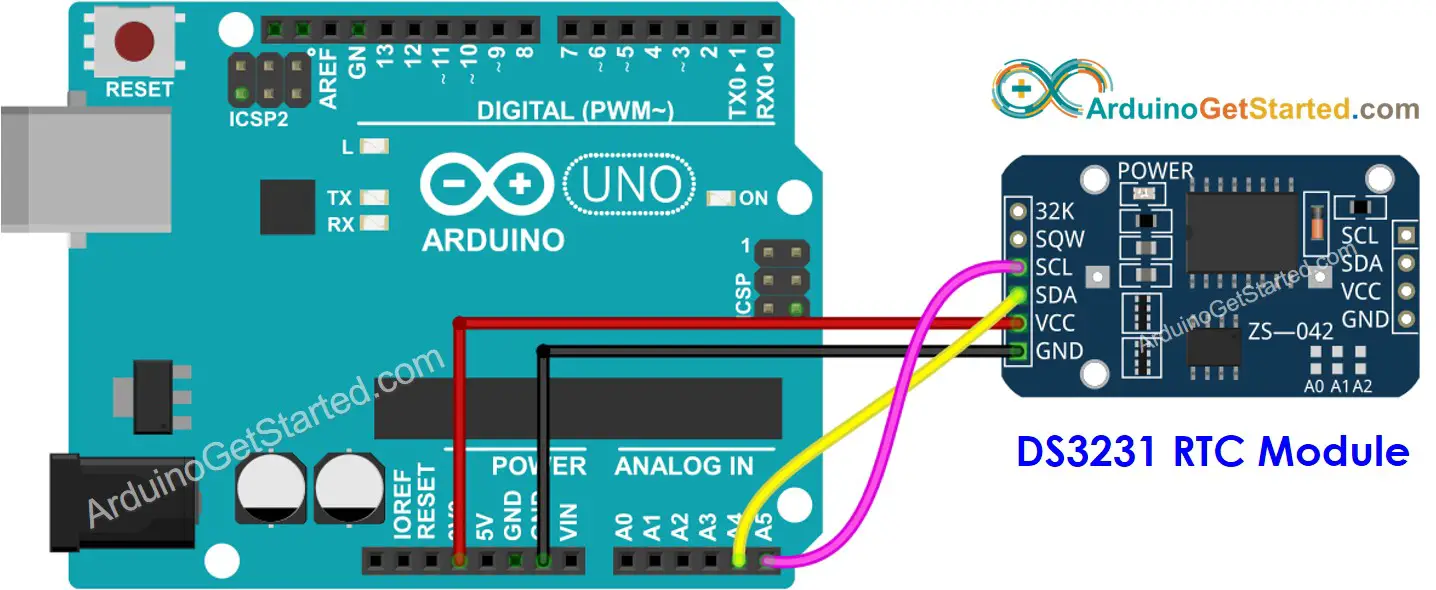
This image is created using Fritzing. Click to enlarge image
DS3231 RTC Module | Arduino Uno, Nano | Arduino Mega |
Vin | 3.3V | 3.3V |
GND | GND | GND |
SDA | A4 | 20 |
SCL | A5 | 21 |
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
while (1);
}
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
DateTime now = rtc.now();
Serial.print("Date & Time: ");
Serial.print(now.year(), DEC);
Serial.print('/');
Serial.print(now.month(), DEC);
Serial.print('/');
Serial.print(now.day(), DEC);
Serial.print(" (");
Serial.print(now.dayOfTheWeek());
Serial.print(") ");
Serial.print(now.hour(), DEC);
Serial.print(':');
Serial.print(now.minute(), DEC);
Serial.print(':');
Serial.println(now.second(), DEC);
#include <RTClib.h>
RTC_DS3231 rtc;
char daysOfTheWeek[7][12] = {
"Sunday",
"Monday",
"Tuesday",
"Wednesday",
"Thursday",
"Friday",
"Saturday"
};
void setup () {
Serial.begin(9600);
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
Serial.flush();
while (1);
}
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
}
void loop () {
DateTime now = rtc.now();
Serial.print("Date & Time: ");
Serial.print(now.year(), DEC);
Serial.print('/');
Serial.print(now.month(), DEC);
Serial.print('/');
Serial.print(now.day(), DEC);
Serial.print(" (");
Serial.print(daysOfTheWeek[now.dayOfTheWeek()]);
Serial.print(") ");
Serial.print(now.hour(), DEC);
Serial.print(':');
Serial.print(now.minute(), DEC);
Serial.print(':');
Serial.println(now.second(), DEC);
delay(1000);
}
Navigate to the Libraries icon on the left bar of the Arduino IDE.
Search “RTClib”, then find the RTC library by Adafruit
Click Install button to install RTC library.
Copy the above code and open with Arduino IDE
Click Upload button on Arduino IDE to upload code to Arduino
Open Serial Monitor
See the result on Serial Monitor.
Date & Time: 2021/10/6 (Wednesday) 11:27:35
Date & Time: 2021/10/6 (Wednesday) 11:27:36
Date & Time: 2021/10/6 (Wednesday) 11:27:37
Date & Time: 2021/10/6 (Wednesday) 11:27:38
Date & Time: 2021/10/6 (Wednesday) 11:27:39
Date & Time: 2021/10/6 (Wednesday) 11:27:40
Date & Time: 2021/10/6 (Wednesday) 11:27:41
Date & Time: 2021/10/6 (Wednesday) 11:27:42
Date & Time: 2021/10/6 (Wednesday) 11:27:43
Date & Time: 2021/10/6 (Wednesday) 11:27:44
#include <RTClib.h>
uint8_t DAILY_EVENT_START_HH = 13;
uint8_t DAILY_EVENT_START_MM = 50;
uint8_t DAILY_EVENT_END_HH = 14;
uint8_t DAILY_EVENT_END_MM = 10;
RTC_DS3231 rtc;
char daysOfTheWeek[7][12] = {
"Sunday",
"Monday",
"Tuesday",
"Wednesday",
"Thursday",
"Friday",
"Saturday"
};
void setup () {
Serial.begin(9600);
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
while (1);
}
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
}
void loop () {
DateTime now = rtc.now();
if (now.hour() >= DAILY_EVENT_START_HH &&
now.minute() >= DAILY_EVENT_START_MM &&
now.hour() < DAILY_EVENT_END_HH &&
now.minute() < DAILY_EVENT_END_MM) {
Serial.println("It is on scheduled time");
} else {
Serial.println("It is NOT on scheduled time");
}
printTime(now);
}
void printTime(DateTime time) {
Serial.print("TIME: ");
Serial.print(time.year(), DEC);
Serial.print('/');
Serial.print(time.month(), DEC);
Serial.print('/');
Serial.print(time.day(), DEC);
Serial.print(" (");
Serial.print(daysOfTheWeek[time.dayOfTheWeek()]);
Serial.print(") ");
Serial.print(time.hour(), DEC);
Serial.print(':');
Serial.print(time.minute(), DEC);
Serial.print(':');
Serial.println(time.second(), DEC);
}
#include <RTClib.h>
#define SUNDAY 0
#define MONDAY 1
#define TUESDAY 2
#define WEDNESDAY 3
#define THURSDAY 4
#define FRIDAY 5
#define SATURDAY 6
uint8_t WEEKLY_EVENT_DAY = MONDAY;
uint8_t WEEKLY_EVENT_START_HH = 13;
uint8_t WEEKLY_EVENT_START_MM = 50;
uint8_t WEEKLY_EVENT_END_HH = 14;
uint8_t WEEKLY_EVENT_END_MM = 10;
RTC_DS3231 rtc;
char daysOfTheWeek[7][12] = {
"Sunday",
"Monday",
"Tuesday",
"Wednesday",
"Thursday",
"Friday",
"Saturday"
};
void setup () {
Serial.begin(9600);
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
while (1);
}
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
}
void loop () {
DateTime now = rtc.now();
if (now.dayOfTheWeek() == WEEKLY_EVENT_DAY &&
now.hour() >= WEEKLY_EVENT_START_HH &&
now.minute() >= WEEKLY_EVENT_START_MM &&
now.hour() < WEEKLY_EVENT_END_HH &&
now.minute() < WEEKLY_EVENT_END_MM) {
Serial.println("It is on scheduled time");
} else {
Serial.println("It is NOT on scheduled time");
}
printTime(now);
}
void printTime(DateTime time) {
Serial.print("TIME: ");
Serial.print(time.year(), DEC);
Serial.print('/');
Serial.print(time.month(), DEC);
Serial.print('/');
Serial.print(time.day(), DEC);
Serial.print(" (");
Serial.print(daysOfTheWeek[time.dayOfTheWeek()]);
Serial.print(") ");
Serial.print(time.hour(), DEC);
Serial.print(':');
Serial.print(time.minute(), DEC);
Serial.print(':');
Serial.println(time.second(), DEC);
}
#include <RTClib.h>
#define SUNDAY 0
#define MONDAY 1
#define TUESDAY 2
#define WEDNESDAY 3
#define THURSDAY 4
#define FRIDAY 5
#define SATURDAY 6
#define JANUARY 1
#define FEBRUARY 2
#define MARCH 3
#define APRIL 4
#define MAY 5
#define JUNE 6
#define JULY 7
#define AUGUST 8
#define SEPTEMBER 9
#define OCTOBER 10
#define NOVEMBER 11
#define DECEMBER 12
DateTime EVENT_START(2021, AUGUST, 15, 13, 50);
DateTime EVENT_END(2021, SEPTEMBER, 29, 14, 10);
RTC_DS3231 rtc;
char daysOfTheWeek[7][12] = {
"Sunday",
"Monday",
"Tuesday",
"Wednesday",
"Thursday",
"Friday",
"Saturday"
};
void setup () {
Serial.begin(9600);
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
Serial.flush();
while (1);
}
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
}
void loop () {
DateTime now = rtc.now();
if (now.secondstime() >= EVENT_START.secondstime() &&
now.secondstime() < EVENT_END.secondstime()) {
Serial.println("It is on scheduled time");
} else {
Serial.println("It is NOT on scheduled time");
}
printTime(now);
}
void printTime(DateTime time) {
Serial.print("TIME: ");
Serial.print(time.year(), DEC);
Serial.print('/');
Serial.print(time.month(), DEC);
Serial.print('/');
Serial.print(time.day(), DEC);
Serial.print(" (");
Serial.print(daysOfTheWeek[time.dayOfTheWeek()]);
Serial.print(") ");
Serial.print(time.hour(), DEC);
Serial.print(':');
Serial.print(time.minute(), DEC);
Serial.print(':');
Serial.println(time.second(), DEC);
}
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.
※ OUR MESSAGES
You can share the link of this tutorial anywhere. Howerver, please do not copy the content to share on other websites. We took a lot of time and effort to create the content of this tutorial, please respect our work!