Arduino - Button Count - OLED
In this tutorial, we are going to use Arduino:
- Count the number of times a button is pressed
- Display the count number on OLED display.
- Auto vertical and horizontal center align the count number on OLED display.
In this tutorial, the button also is debounced without using delay() function. See Why do we need debouncing?
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some links in this section are Amazon affiliate links. If you make a purchase through these links, we may earn a commission at no extra cost to you.
Additionally, some links direct to products from our own brand, DIYables .
Additionally, some links direct to products from our own brand, DIYables .
Wiring Diagram
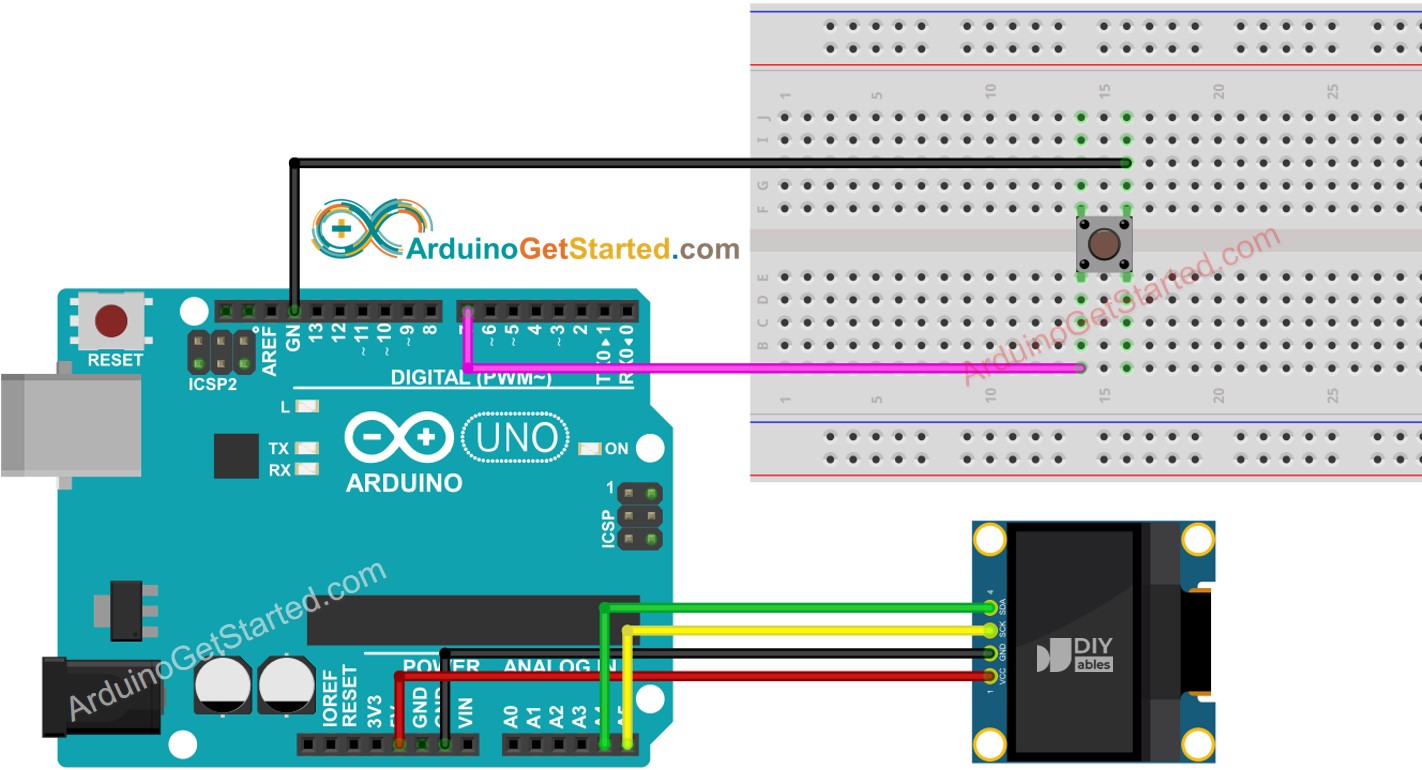
This image is created using Fritzing. Click to enlarge image
Arduino Code - Vertical and Horizontal Center Align on OLED
/*
* Created by ArduinoGetStarted.com
*
* This example code is in the public domain
*
* Tutorial page: https://arduinogetstarted.com/tutorials/arduino-button-count-oled
*/
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#include <ezButton.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
Adafruit_SSD1306 oled(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1); // create SSD1306 display object connected to I2C
ezButton button(7); // create ezButton object that attach to pin 7;
unsigned long lastCount = 0;
void setup() {
Serial.begin(9600);
// initialize OLED display with address 0x3C for 128x64
if (!oled.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
while (true);
}
delay(2000); // wait for initializing
oled.clearDisplay(); // clear display
oled.setTextSize(2); // text size
oled.setTextColor(WHITE); // text color
oled.setCursor(0, 10); // position to display
button.setDebounceTime(50); // set debounce time to 50 milliseconds
button.setCountMode(COUNT_FALLING);
}
void loop() {
button.loop(); // MUST call the loop() function first
unsigned long count = button.getCount();
if (lastCount != count) {
Serial.println(count); // print count to Serial Monitor
String text = String(count);
oledDisplayCenter(text);
lastCount != count;
}
}
void oledDisplayCenter(String text) {
int16_t x1;
int16_t y1;
uint16_t width;
uint16_t height;
oled.getTextBounds(text, 0, 0, &x1, &y1, &width, &height);
// display on horizontal and vertical center
oled.clearDisplay(); // clear display
oled.setCursor((SCREEN_WIDTH - width) / 2, (SCREEN_HEIGHT - height) / 2);
oled.println(text); // text to display
oled.display();
}
※ NOTE THAT:
The about code automatically horizontal and vertical center aligns the text on OLED display. See How to vertical/horizontal center on OLED for more detail.
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.