Arduino - MQTT
In this tutorial, we will learn how to use Arduino to send/receive data to MQTT broker using MQTT protocol. In detail, we will learn:
- How to connect Arduino to MQTT broker
- How to program Arduino to send data to MQTT broker by publishing data to a MQTT topic
- How to program Arduino to receive data by subscribing to a MQTT topic.
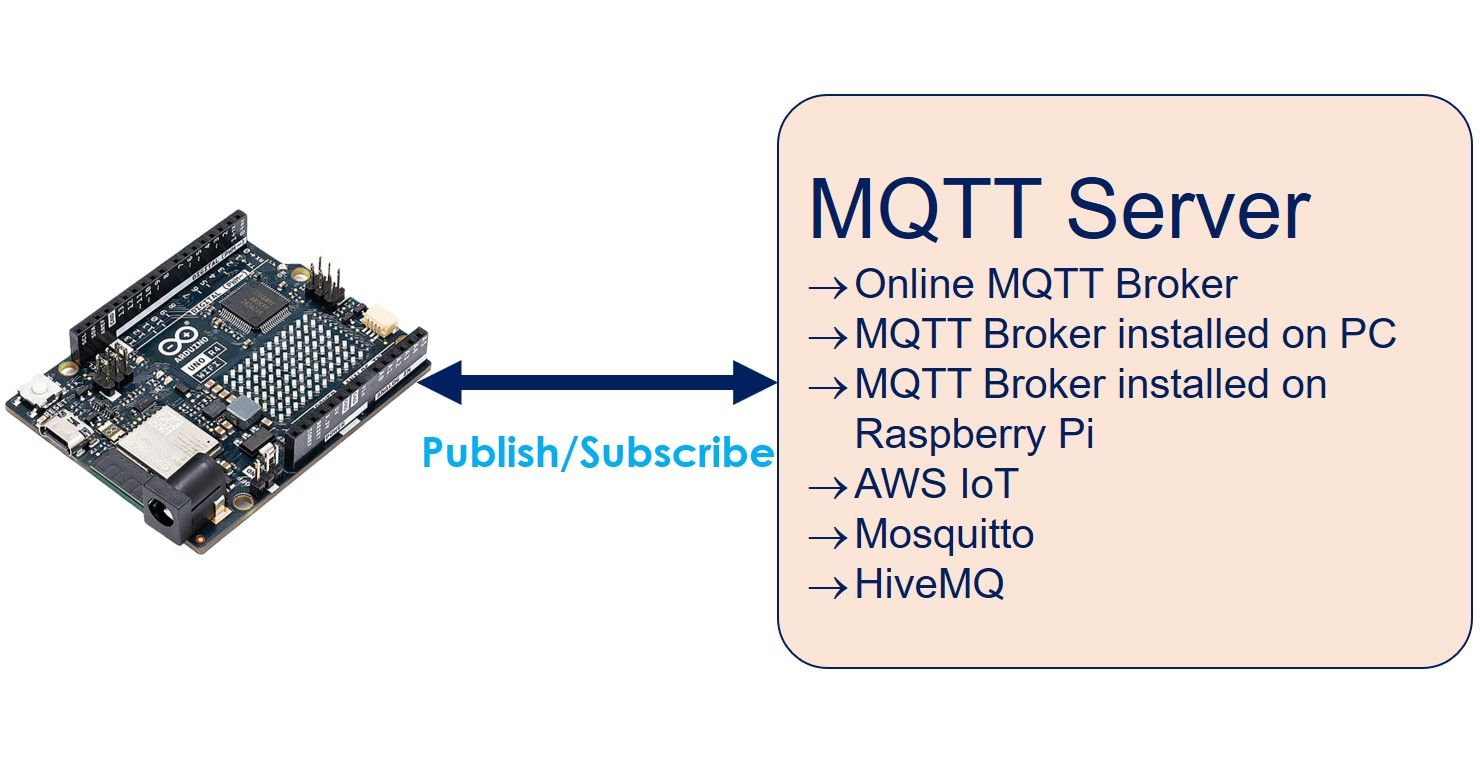
We'll explore two different use cases:
- Using Arduino with an online MQTT broker.
- Using Arduino with the MQTT broker installed on your PC.
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About Arduino and MQTT
Assuming you're already familiar with the MQTT protocol. If not, please conduct research on the Internet. This tutorial focuses on programming Arduino to send and receive data using the MQTT protocol.
The below are use-cases of Arduino with MQTT:
- Arduino connects to online MQTT broker, e.g.: online Mosquitto broker, AWS IoT...
- Arduino connects to MQTT broker installed on your PC, e.g.: Mosquitto broker, HiveMQ broker
- Arduino connects to MQTT broker installed on your Raspberry Pi, e.g.: Mosquitto broker
- Arduino connects to MQTT broker installed on cloud: e.g.: Mosquitto or HiveMQ broker on AWS EC2
In this tutorial, we'll begin by quickly checking if Arduino can connect to an online Mosquitto broker. Arduino will publish and subscribe to this broker over the internet.
Next, we'll move on to installing the Mosquitto broker on our PC. We'll then connect Arduino to the MQTT broker installed on our PC, continuing to publish and subscribe data through this local broker.
Once you've completed this tutorial, you can explore further by learning about Arduino with MQTT in the following tutorials:
Connect Arduino to an online MQTT broker
In this part, we will learn how to connect Arduino to test.mosquitto.org, an online MQTT broker created by Mosquitto. Please note that this broker should be used for the testing purpose only.
Arduino Code
The below Arduino code does:
- Connect to the MQTT broker
- Subscribe to a topic
- Periodically publish messages to the same topic that it subscribes
Quick Steps
- If this is the first time you use Arduino Uno R4, see how to setup environment for Arduino Uno R4 on Arduino IDE.
- Open Arduino IDE on your PC
- Open the Library Manager by clicking on the Library Manager icon on the left navigation bar of Arduino IDE
- Type MQTT on the search box, then look for the MQTT library by Joel Gaehwiler.
- Click Install button to install MQTT library.
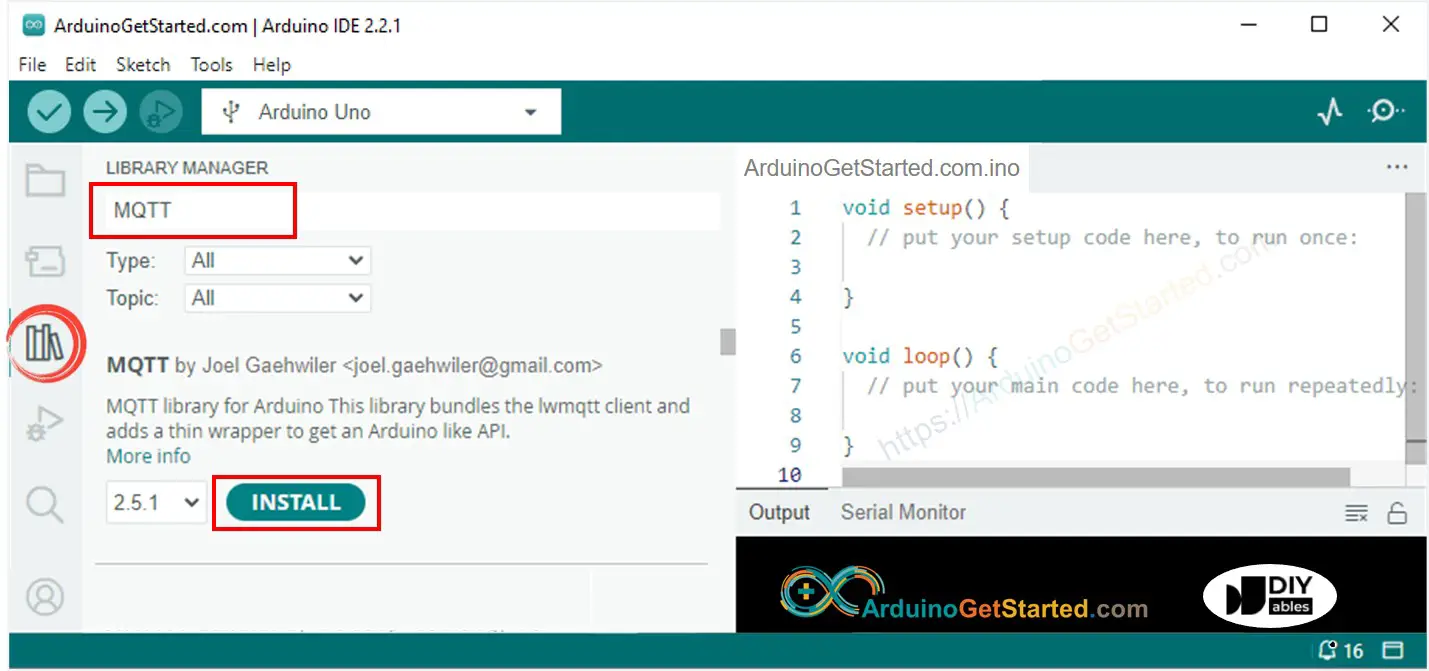
- Type ArduinoJson on the search box, then look for the ArduinoJson library by Benoit Blanchon.
- Click Install button to install ArduinoJson library.
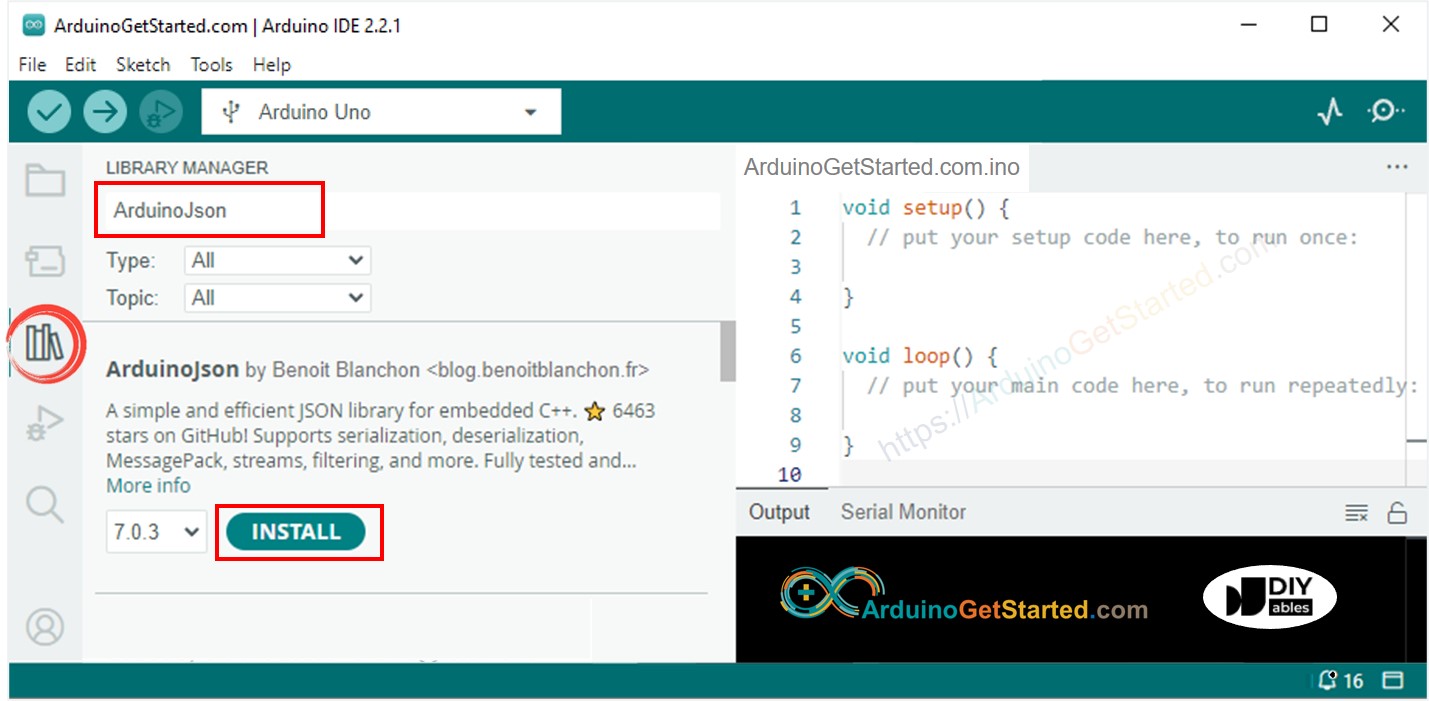
- Copy the above code and open with Arduino IDE
- Replace the WiFi information (SSID and password) in the code with your own.
- In the code, you will see the word 'YOUR-NAME' three times. Replace this word with your name or random characters (alphabet characters only, no spaces). This is necessary because if you do not make the change, there may be multiple people running this code at the same time, which could lead to conflicts because the MQTT client IDs and topics are the same for everyone.
- Click Upload button on Arduino IDE to upload code to Arduino
- Open the Serial Monitor
- See the result on Serial Monitor.
As you can see, Arduino publishes messages to the MQTT broker, then receives back the same message. That is because the above code subscribes to the same topic that it publishes data to. If you do not want Arduino to receive the message that it publishes, simply make the SUBSCRIBE topic different from the PUBLISH topic.
Connect Arduino to the MQTT broker installed on your PC
Installing Mosquitto MQTT Broker
- Download the Mosquitto MQTT Broker
- Install it on the D: drive instead of the C: drive. Avoid installing the Mosquitto broker on the C: drive to prevent potential issues.
Run Mosquitto MQTT broker
Now, let's check if the MQTT broker is functioning properly by following these steps:
- Go to the directory where Mosquitto was installed. For instance: D:\Draft\mosquitto>
- Make a new file named test.conf, copy the content below, and save it in that directory:
- Run a Command Prompt as Administrator on your PC. Let's call it Broker Window. Do not close it until the end of the tutorial.
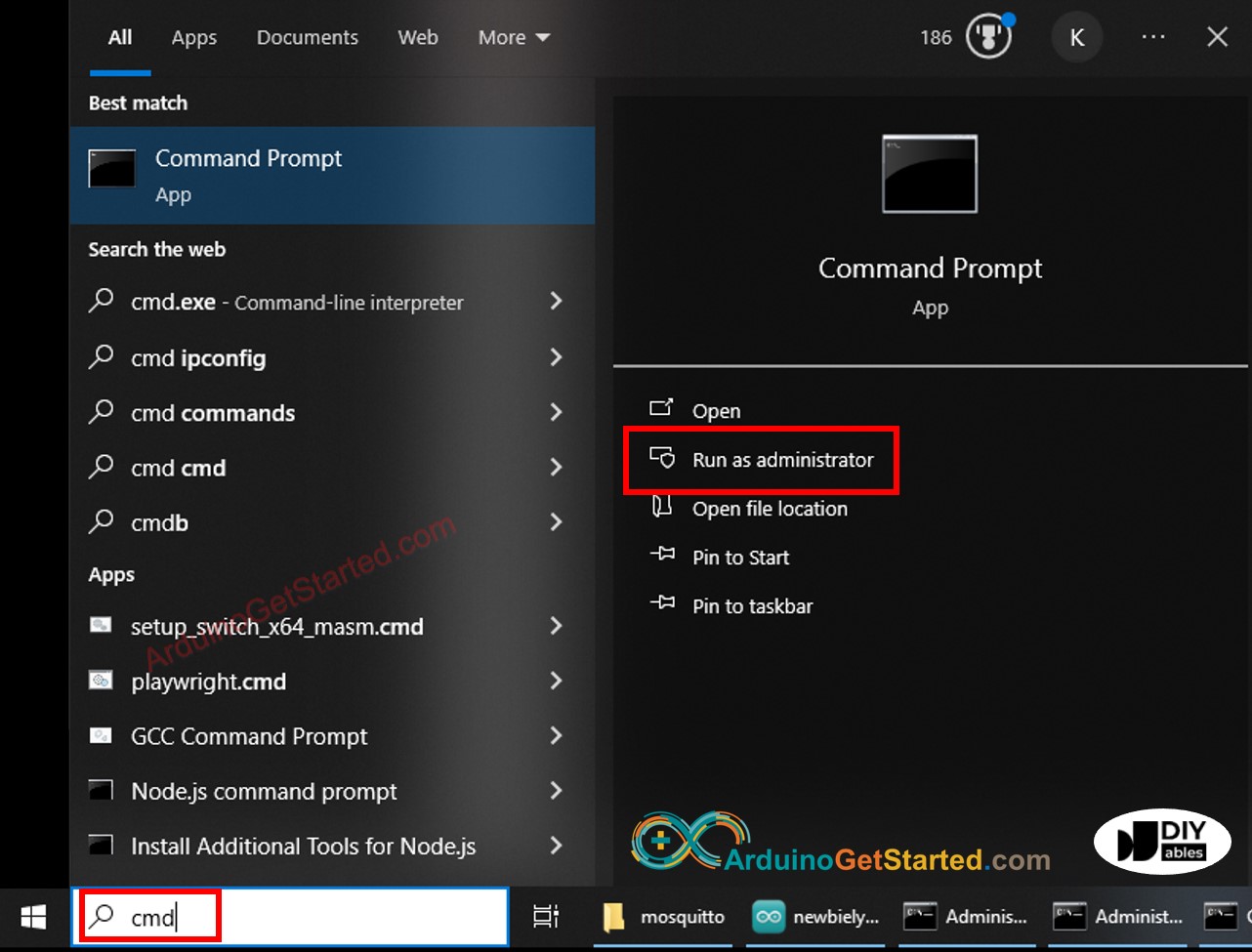
- Run the below commands one by one:
- You will see:
- Open another Command Prompt as Administrator on your PC.
- Find the IP address of your PC by running the below command:
- Write down the IP address for later use. In the above example: 192.168.0.26
Test if the Mosquitto Broker works
- Open another Command Prompt as Administrator on your PC. Let's call it Subscriber Window
- Subscribe to a topic by running the below commands one by one (replace by your IP address):
- Open another Command Prompt as Administrator on your PC. Let's call it Publisher Window
- Publish a message to the same topic by running the below commands one by one (replace by your IP address):
- You will see:
You will see that message is forwarded to the Subscriber Window as follows:
Now, you installed successfully the Mosquitto MQTT broker on your PC. Please do NOT close three windows: Broker Window, Subscriber Window, and Publisher Window. We will use them next.
Arduino Code
The below Arduino code does:
- Connect to the MQTT broker
- Subscribe to a topic
- Periodically publish messages to another topic
Quick Steps
- Copy the above code and open with Arduino IDE
- Replace the WiFi information (SSID and password) in the code with your own.
- Replace the MQTT broker address in the code (domain name or IP address).
- Click Upload button on Arduino IDE to upload code to Arduino
Send message from Arduino to PC via MQTT
Arduino codes publishes data to the MQTT topic arduino-001/send, Subscriber Window on PC subscribe that topic to receive the data.
- Open the Serial Monitor, you will see Arduino periodically publish a message to a topic.
- Check the Subscriber Window, you will see that it receives the message published by Arduino as below:
Send message from PC to Arduino via MQTT
Arduino subscribes to the topic arduino-001/receive, Publisher Window on PC publish a message to that topic to send it to the Arduino.
- Publish a message to the topic that Arduino subscribed by running the following command on Publisher Window:
- You will see this message is received by Arduino on Serial Monitor as below:
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.