Arduino - Analog Keypad Library
This library is designed for the following use cases:
- The project uses a keypad but IO pins are not enough ⇒ use an Analog Keypad, which connects to a single analog input pin.
- The project uses multiple buttons but IO pins are not enough ⇒ build a button array, which connects to a single analog input pin.
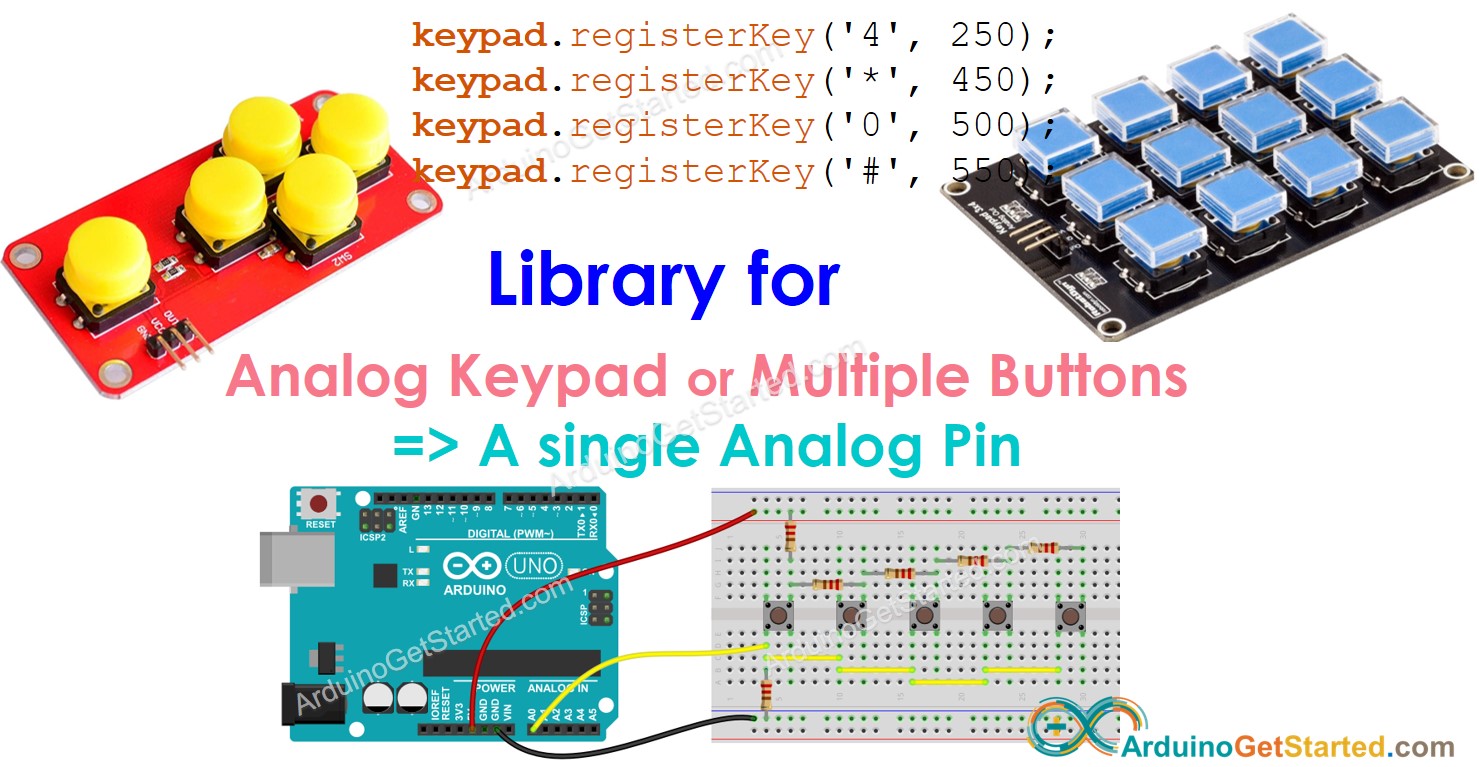
The ezAnalogKeypad (easy Analog Keypad) library is created by ArduioGetStarted.com.
Library Features
- Can be used with the Analog Keypad 3x4, 4x4 or any
- Can be used with the array of buttons that connects to a single Analog Input pin
- All functions are non-blocking (no delay() function)
Examples
Functions
- ezAnalogKeypad(analogPin)
- SetDebounceTime(time)
- SetNoPressValue(analogValue)
- RegisterKey(key, analogValue)
- GetKey()
How it works
For the analog keypad or array of buttons that connects to a single analog input pin, when a key/button is pressed, the analog value is changed to a specific value. This value is diffrent from each key/button. This library reads the analog value determine which key/button is pressed by finding a key/button that has a nearest value.
⇒ What we needs to do first: determine the analog value for each key when it is pressed, and also the analog value when no key is pressed
The analog value when a key/button is pressed is depending on the following factors:
- How keys/buttons are wired
- The value of resistors
- The voltage supplies for the keypad/array of buttons
- The voltage reference of ADC
- The resolution of ADC (e.g. Arduino Uno is 10-bit ADC, ESP32 is 12-bit ADC)
⇒ The simplest way to find the analog values is run a test for callibration. By doing this way, we do not need to care about the above factors. See the example tutorial for detaied how to do callibration.
How To Install Library
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search “ezAnalogKeypad”, then find the library by ArduinoGetStarted
- Click Install button to install ezAnalogKeypad library.
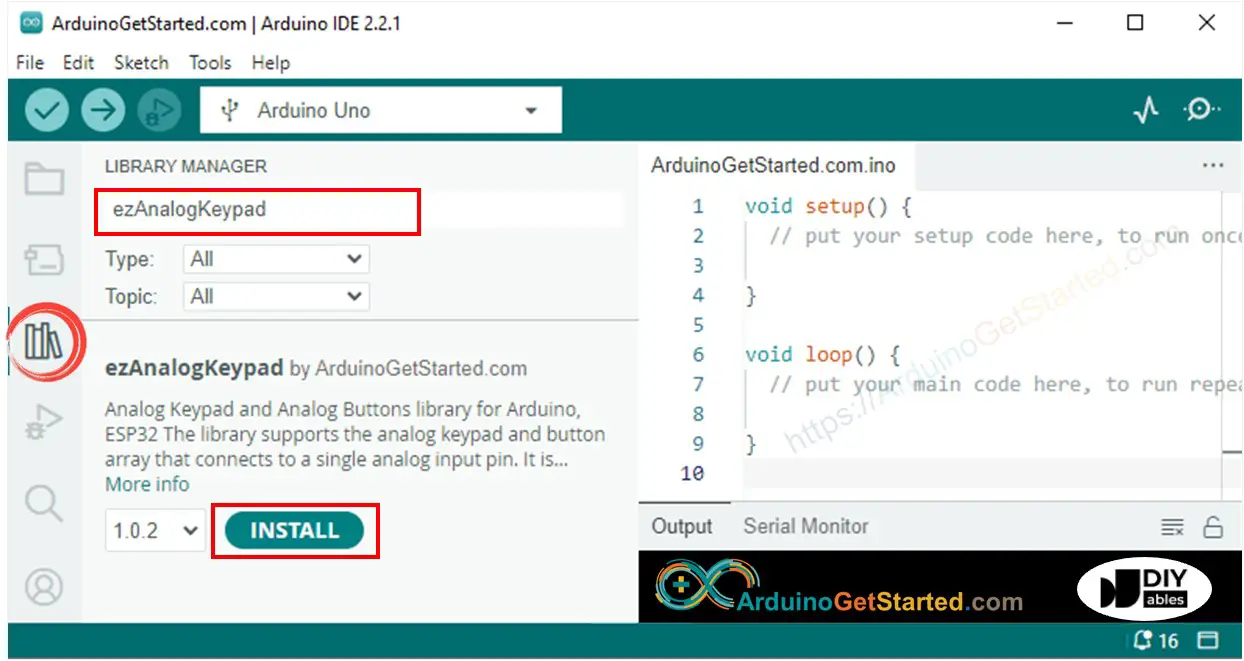
Or you can download it on Github
※ NOTE THAT:
If this library is useful for your work, you can say thanks to us by buying us a coffee: PayPal.Me/arduinogetstarted
References
ezAnalogKeypad()
Description
This function creates a new instance of the ezAnalogKeypad class that represents a particular analog keypad or an array of button that connected to a single analog input pin.
Syntax
Parameters
pin: int - an analog input pin.
Return
A new instance of the ezAnalogKeypad class.
Example
setDebounceTime()
Description
This function is used to set the debounce time. If you do not use this function, the 50 milliseconds will be used as the default value.
Syntax
Parameters
time: unsigned long - debounce time in milliseconds
Return
None
setNoPressValue()
Description
This function is used to set the analog value when there is no key is pressed. You needs to run the callibration code (in the Examples) to know this value. This function MUST be used one and only one time.
Syntax
Parameters
analogValue: int - The analog value (from analogRead() function) when there is no key pressed
※ NOTE THAT:
analogValue can be fluctuated a little. You can choose a middle value. The library does not compare the analogValue equally. Instead, the library find the nearest match value.
Return
None
registerKey()
Description
This function is used to set the analog value for a key when it is pressed. You needs to run the callibration code (in the Examples) to know this value. This function MUST be used for all keys or buttons.
Syntax
Parameters
key: unsigned char - The key name. It can be a character, or an integer number from 1 to 255.
analogValue: int - The analog value (from analogRead() function) when the key is pressed
※ NOTE THAT:
- For key, you can use '0' character but please do not use 0 number.
- analogValue can be fluctuated a little. You can choose a middle value. The library does not compare the analogValue equally. Instead, the library find the nearest match value.
Return
None
getKey()
Description
This function is used to check whether a key or button is pressed. The function returns 0 if there is no key/button is pressed, and the key name if otherwise
Syntax
Parameters
None
Return
- key: when no key is pressed
- key name when a button is pressed. key is the value is set in registerKey() function.