Arduino - Keypad - Solenoid Lock
In this tutorial, we are going to learn how to use a keypad, solenoid lock, and Arduino together. In detail, If a user inputs the password on the keypad correctly, Arduino turns the solenoid lock on.
The tutorial also provides the code that turns on a solenoid lock in a period of time and then turns off without using delay() function. The Arduino code also supports multiple passwords.
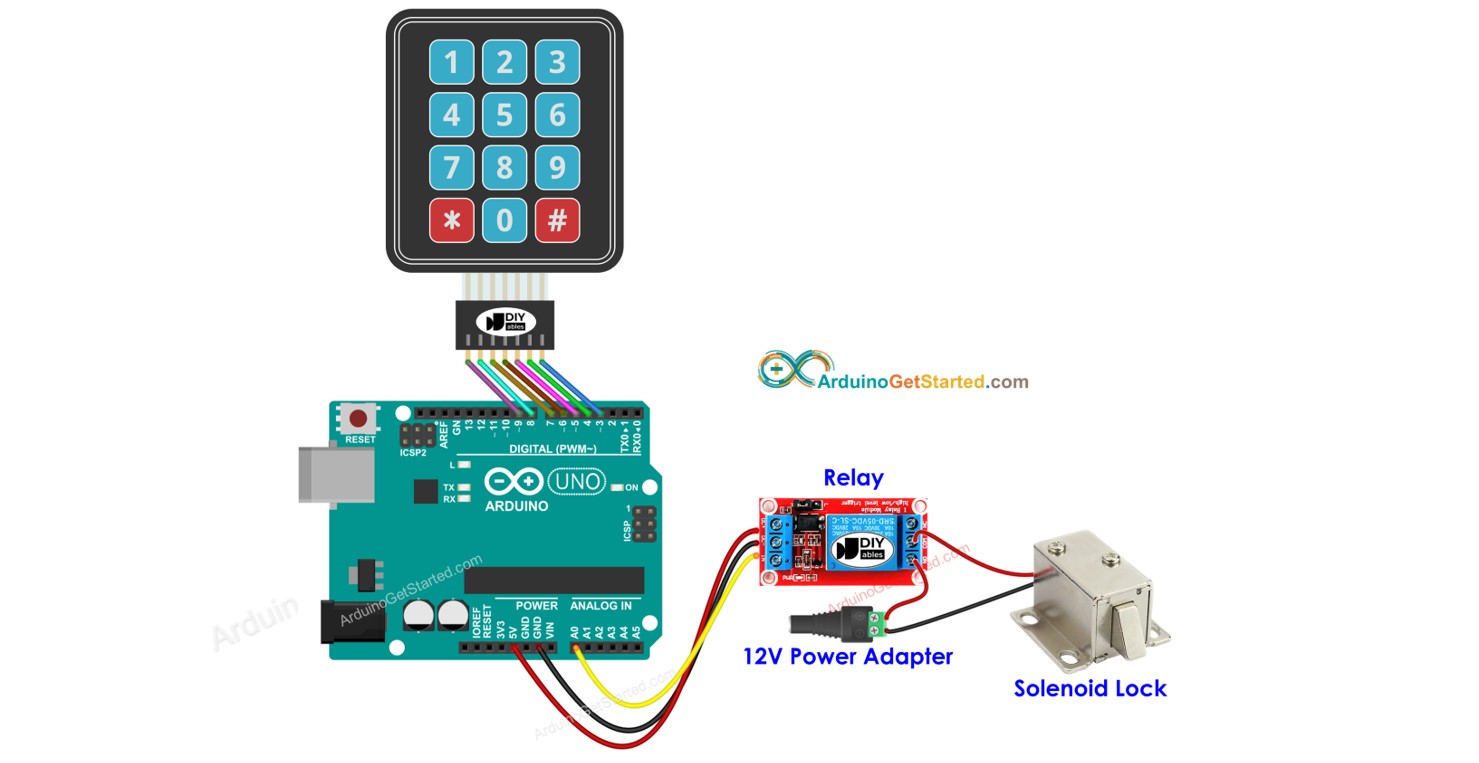
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
About Keypad and Solenoid Lock
If you do not know about keypad and Solenoid Lock (pinout, how it works, how to program ...), learn about them in the following tutorials:
- Arduino - Keypad tutorial
- Arduino - Solenoid Lock tutorial
Wiring Diagram
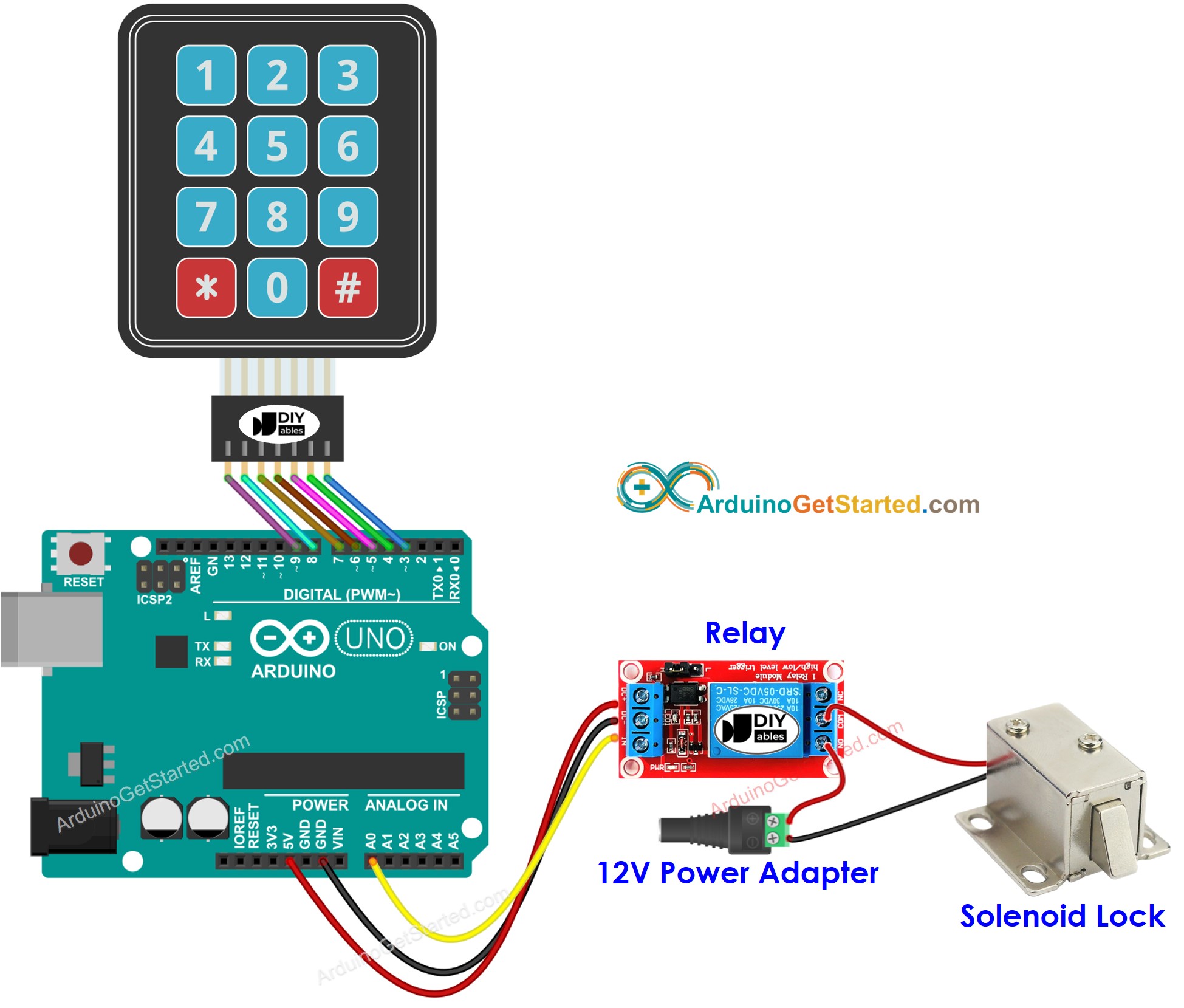
This image is created using Fritzing. Click to enlarge image
Arduino Code - turn solenoid lock on if the password is correct
The below codes turn a solenoid lock on if the password is correct
Quick Steps
- Connect Arduino to PC via USB cable
- Open Arduino IDE, select the right board and port
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search “keypad”, then find the keypad library by Mark Stanley, Alexander Brevig
- Click Install button to install keypad library.
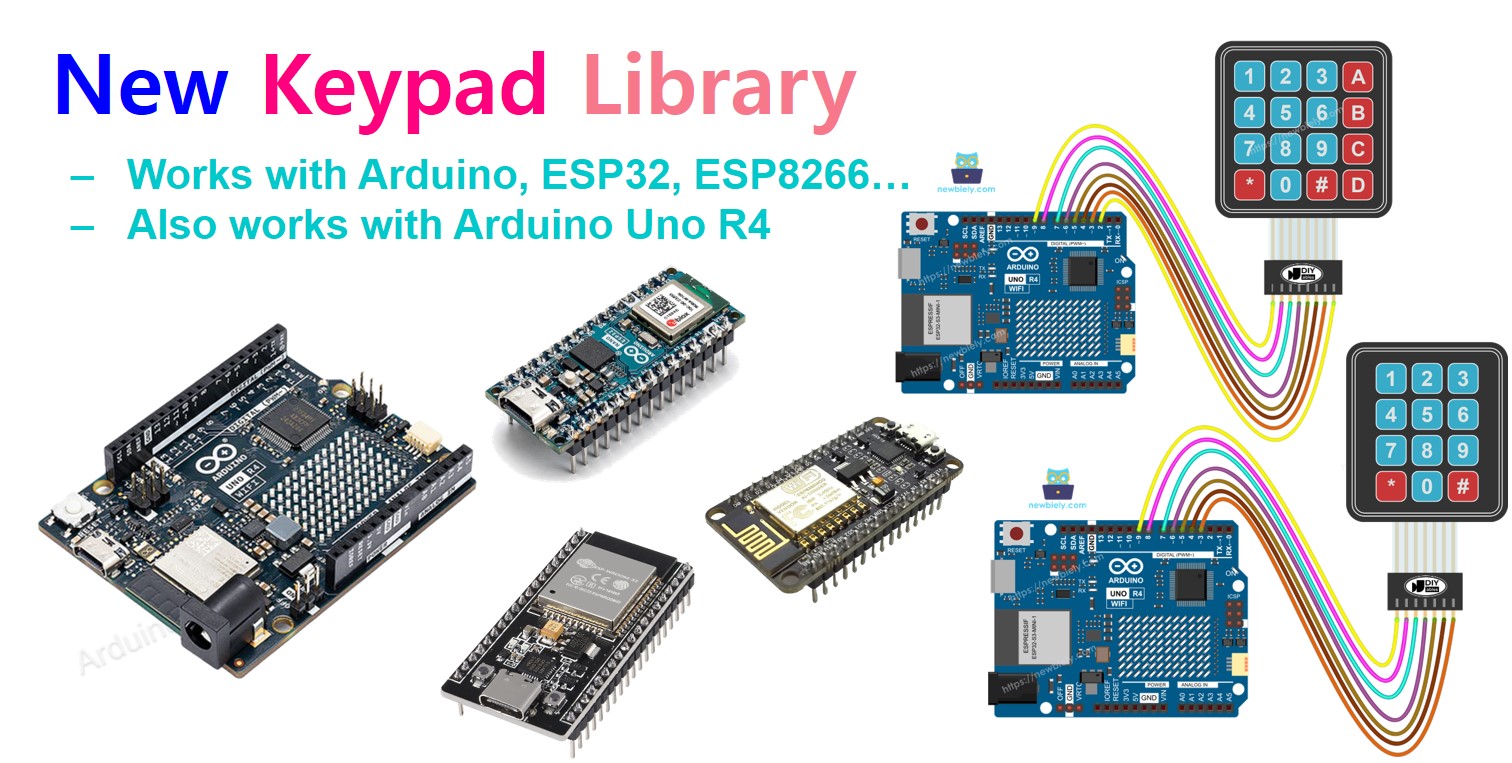
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
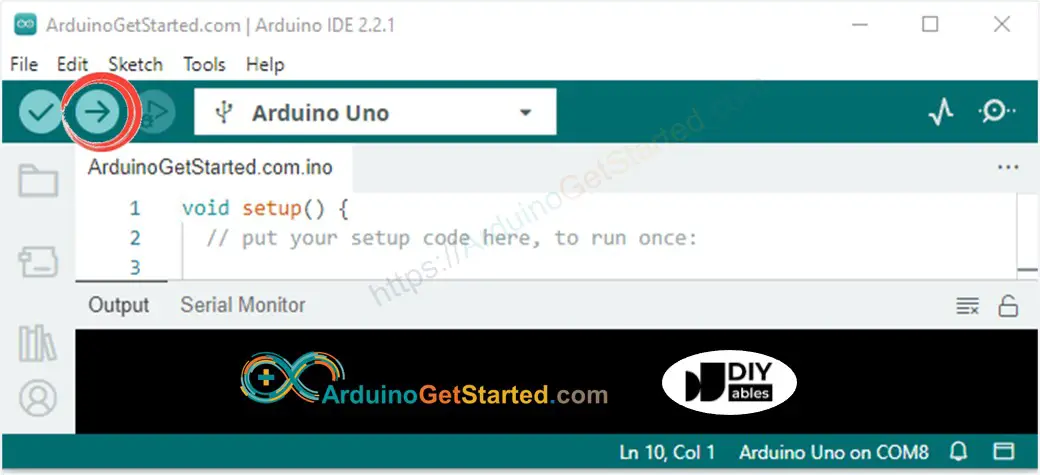
- Press 7124 keys and press #
- Press 1234 keys and press #
- See the result on Serial Monitor and the state of solenoid lock
Code Explanation
Authorized passwords are pre-defined in the Arduino code.
A string is used to store the password inputted by users, called input string. In keypad, two keys (* and #) are used for special purposes: clear password and terminate password. When a key on keypad is pressed:
- If the pressed key is not two special keys, it is appended to the input string
- If the pressed key is *, input string is clear. You can use it to start or re-start inputing the password
- If the pressed key is #:
- The input string is compared with the pre-defined passwords. If it matched with one of the pre-defined passwords, the solenoid lock is turned on.
- No matter the password is correct or not, the input string is clear for the next input
Arduino Code - turn a solenoid lock on in a period of time if the password is correct
The below code turns the solenoid lock on for 5 seconds if the password is correct. After 5 seconds, the solenoid lock is turned off.
Please note that, the above code use ezOutput library, which makes it easy to manage time in non-blocking maner. You can refer to ezOutput Library Installization Guide
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.