Arduino - Button Toggle Relay
In a previous tutorial, We have learned how to turn on the relay if the button is pressed, and turn off relay if the button is released. In this tutorial, We are going to learn how to toggle relay each time button is pressed.
By connecting relay to light bulb, led strip, motor or actuator... We can use the button to toggle light bulb, led strip, motor or actuator...
The tutorial includes two main parts:
Or you can buy the following sensor kits:
Disclosure: Some links in this section are Amazon affiliate links. If you make a purchase through these links, we may earn a commission at no extra cost to you.
Additionally, some links direct to products from our own brand, DIYables.
If you do not know about relay and button (pinout, how it works, how to program ...), learn about them in the following tutorials:
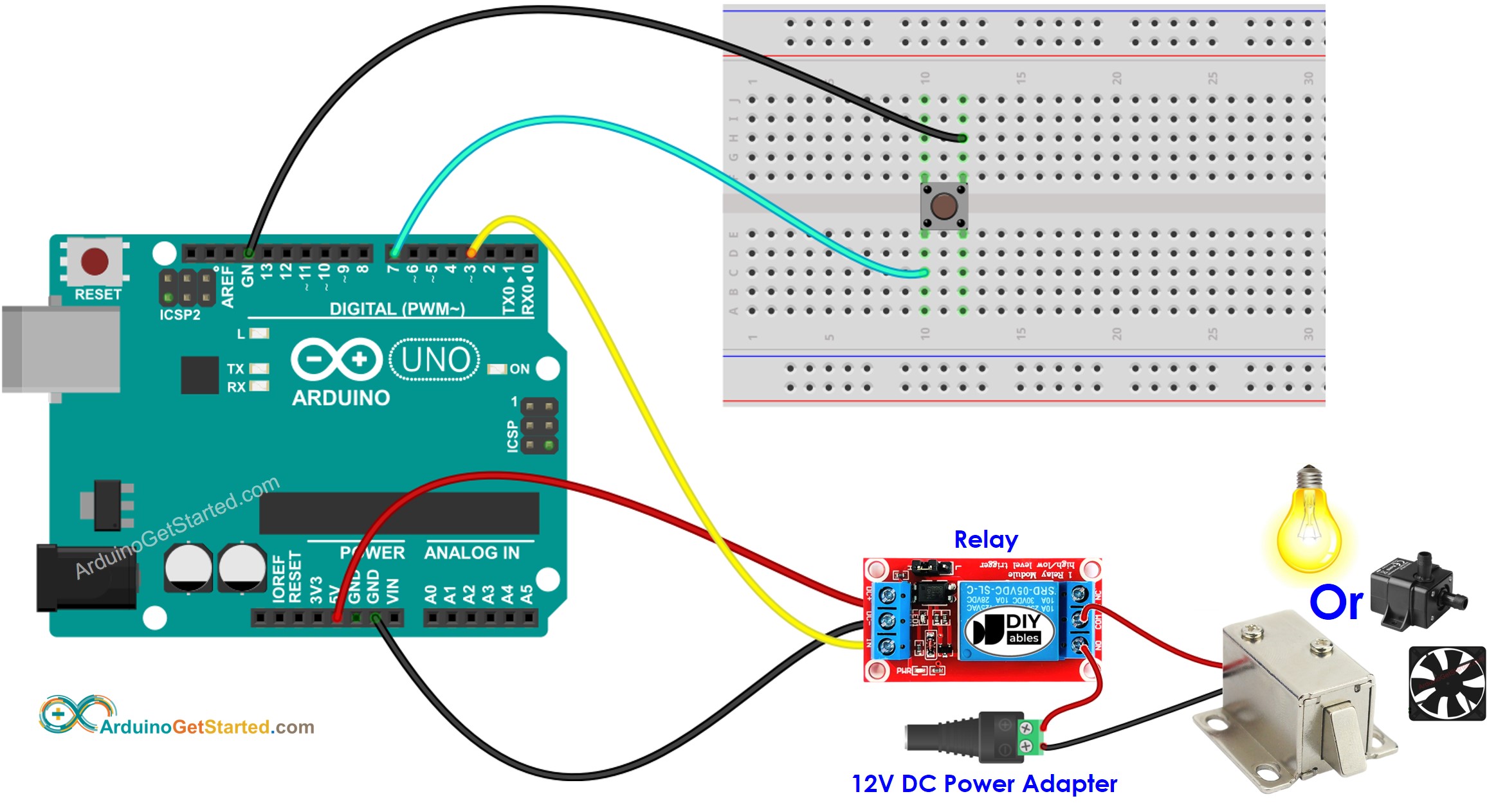
This image is created using Fritzing. Click to enlarge image
const int BUTTON_PIN = 7;
const int RELAY_PIN = 3;
int relayState = LOW;
int lastButtonState;
int currentButtonState;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
pinMode(RELAY_PIN, OUTPUT);
currentButtonState = digitalRead(BUTTON_PIN);
}
void loop() {
lastButtonState = currentButtonState;
currentButtonState = digitalRead(BUTTON_PIN);
if(lastButtonState == HIGH && currentButtonState == LOW) {
Serial.println("The button is pressed");
relayState = !relayState;
digitalWrite(RELAY_PIN, relayState);
}
}
Connect Arduino to PC via USB cable
Open Arduino IDE, select the right board and port
Copy the above code and open with Arduino IDE
Click Upload button on Arduino IDE to upload code to Arduino
Keep pressing the button several seconds and then release it.
See the change of relay's state
Read the line-by-line explanation in comment lines of code!
In the code, relayState = !relayState is equivalent to the following code:
if(relayState == LOW)
relayState = HIGH;
else
relayState = LOW;
※ NOTE THAT:
In practice, the above code does not work correctly sometimes. To make it always work correctly, we need to debounce for the button. Debouncing for the button is not easy for beginners. Fortunately, thanks to the ezButton library, We can do it easily.
Why do we need debouncing? ⇒ see Arduino - Button Debounce tutorial
#include <ezButton.h>
const int BUTTON_PIN = 7;
const int RELAY_PIN = 3;
ezButton button(BUTTON_PIN);
int relayState = LOW;
void setup() {
Serial.begin(9600);
pinMode(RELAY_PIN, OUTPUT);
button.setDebounceTime(50);
}
void loop() {
button.loop();
if(button.isPressed()) {
Serial.println("The button is pressed");
relayState = !relayState;
digitalWrite(RELAY_PIN, relayState);
}
}
Install ezButton library. See
How To
Copy the above code and open with Arduino IDE
Click Upload button on Arduino IDE to upload code to Arduino
Press button several times
See the change of relay's state
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.
Most electronic products have a reset button. Additionally, the button also keeps other functionalities in many products.
※ OUR MESSAGES
You can share the link of this tutorial anywhere. Howerver, please do not copy the content to share on other websites. We took a lot of time and effort to create the content of this tutorial, please respect our work!