Arduino Serial to WiFi Converter
In this tutorial, we will learn how to use the Arduino Uno R4 WiFi to create a Serial to WiFi converter. The Arduino reads data from the Serial port and sends it to a TCP server located either on the same LAN network or remotely on the internet, and vice versa. Additionally, by connecting RS232, RS485, or RS422 modules to the Arduino, we can create RS232 to WiFi, RS485 to WiFi, and RS422 to WiFi converters.
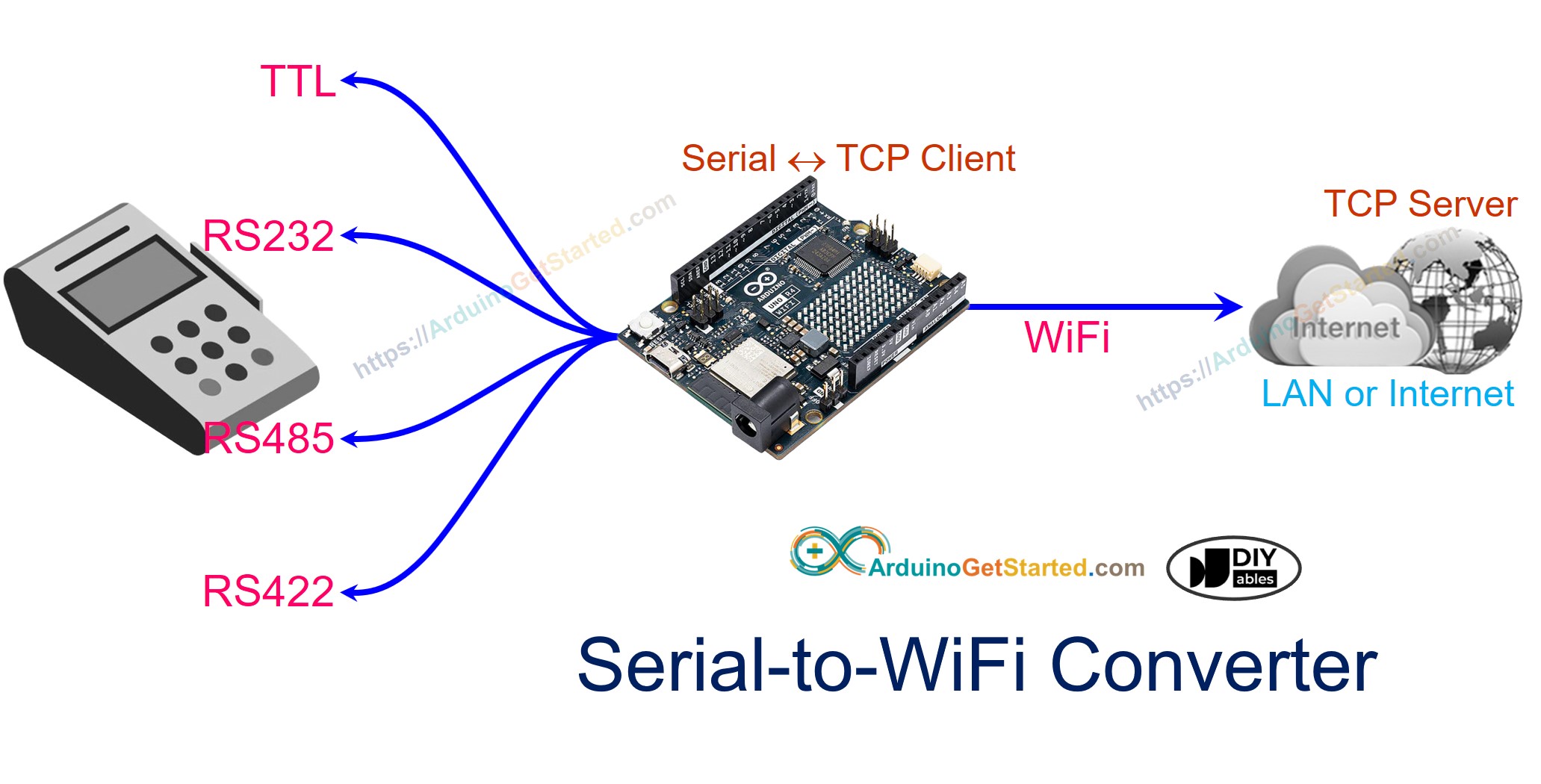
In this tutorial, we will learn how to create a Serial to WiFi converter in general. For specific type of Serial interaface, we will learn in other tutorials below:
These steps will enable you to establish versatile communication bridges between serial devices and WiFi networks using Arduino.
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
How It Works
- Arduino connects to a serial device via serial interface (TTL, RS232, RS485, or RS422)
- Arduino works as a TCP client that connects to a TCP server (can be TCP server software on your PC or another Arduino TCP server)
- Arduino reads data from the serial interface and sends it to the TCP server
- Arduino reads data from the TCP connection and sends it to the serial interface
Arduino Code
Testing
You can do a test by sending data in the following flows:
- Serial Monitor (on your PC) → Serial → Arduino → WiFi → TCP Server Software (on your PC).
- TCP Server Software (on your PC) → WiFi → Arduino → Serial → Serial Monitor (on your PC).
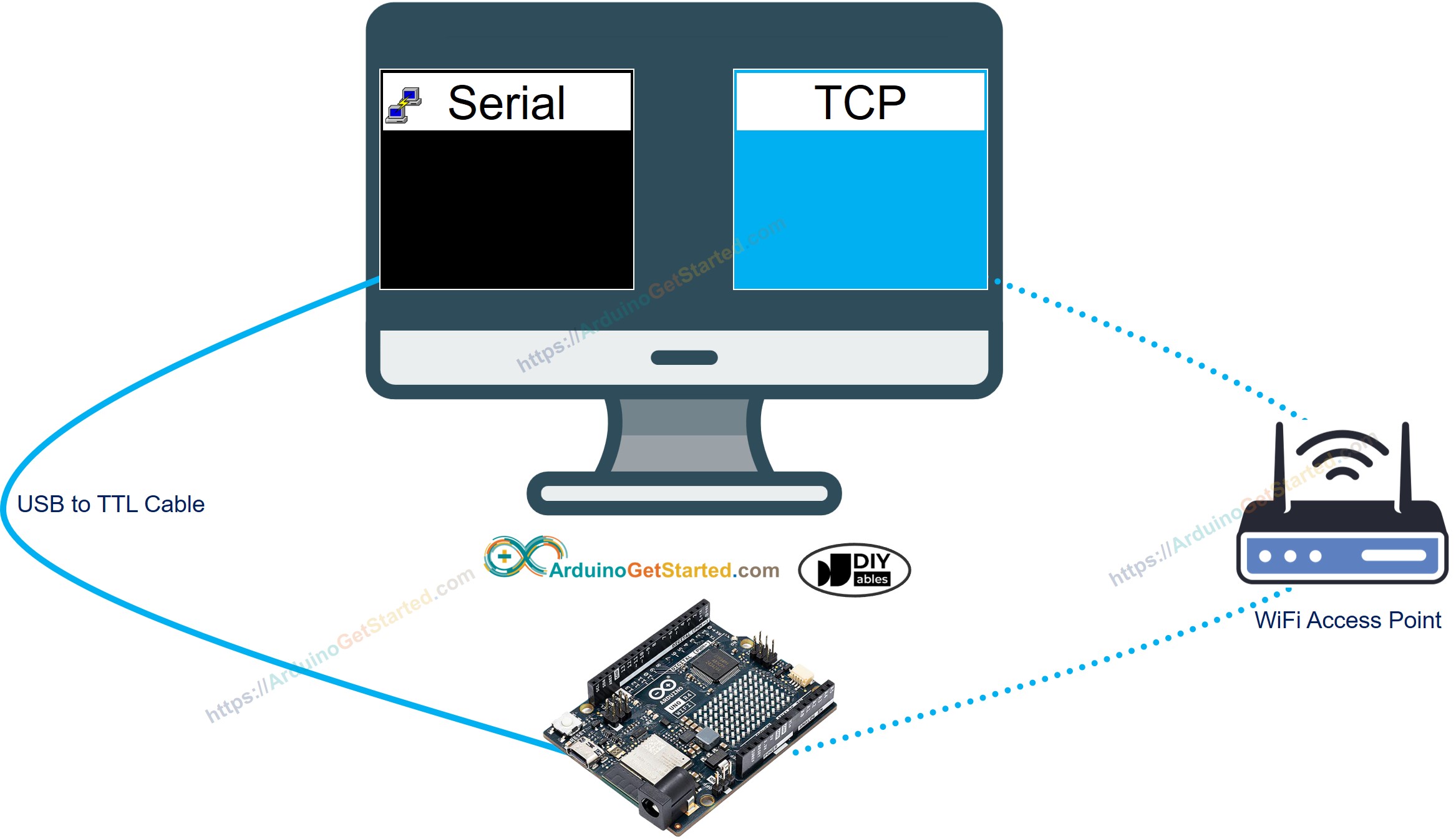
To do it, follow the below steps:
- Connect Arduino to your PC via USB Type-C cable
- Install a TCP server software program like ezTerm
- Open the Serial Monitor
- Open the TCP server program and configure it as TCP Server, then click Listen button
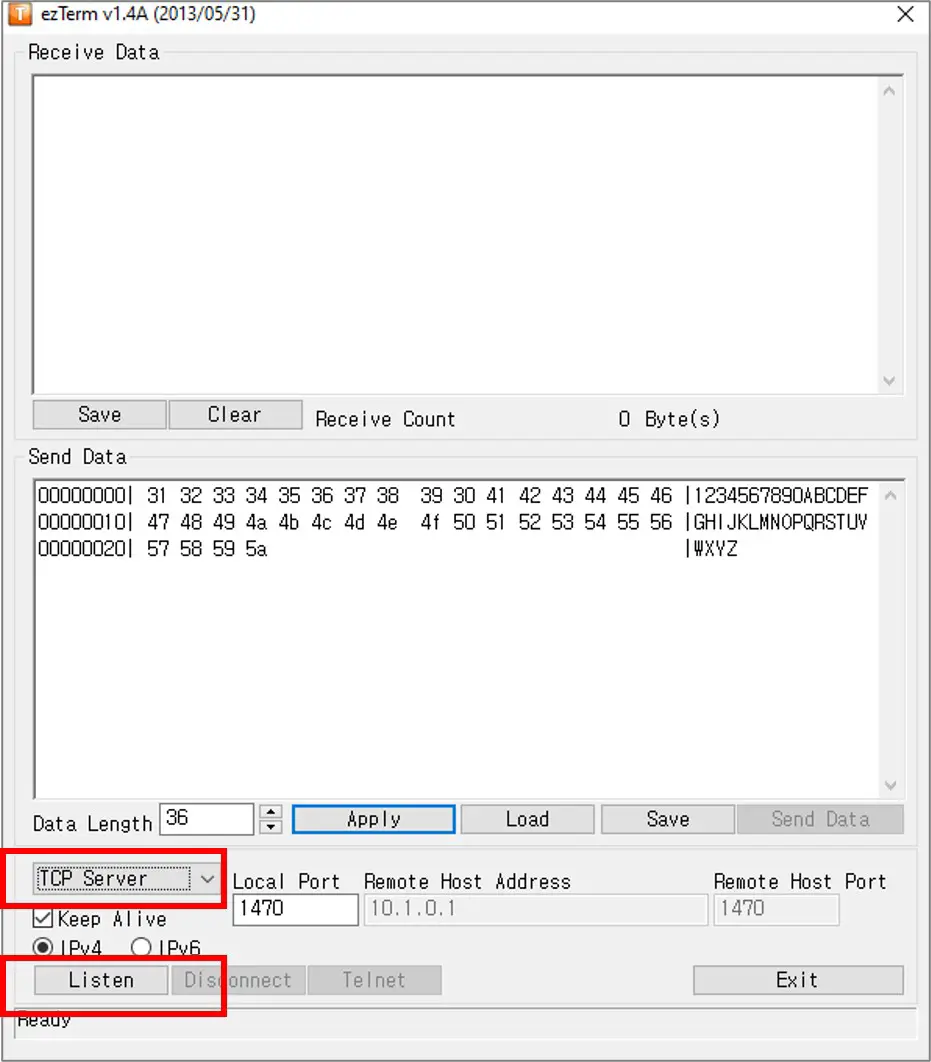
- Open Command Prompt on your PC.
- Find the IP address of your PC by running the below command:
- The output looks like below:
- Change the IP address of your TCP Server (Your PC) in the Arduino code. In the example above, it's indicated by 192.168.0.26.
- Compile and upload the code to the Arduino board by clicking on the Upload button in the Arduino IDE.
- Type some data in the Serial Monitor to send it to the Arduino through Serial.
- If everything works correctly, you'll see the data on the TCP server software.
- Type some data in the TCP server program to send it to the Arduino through TCP.
- If successful, you'll see the data in the Serial Monitor.
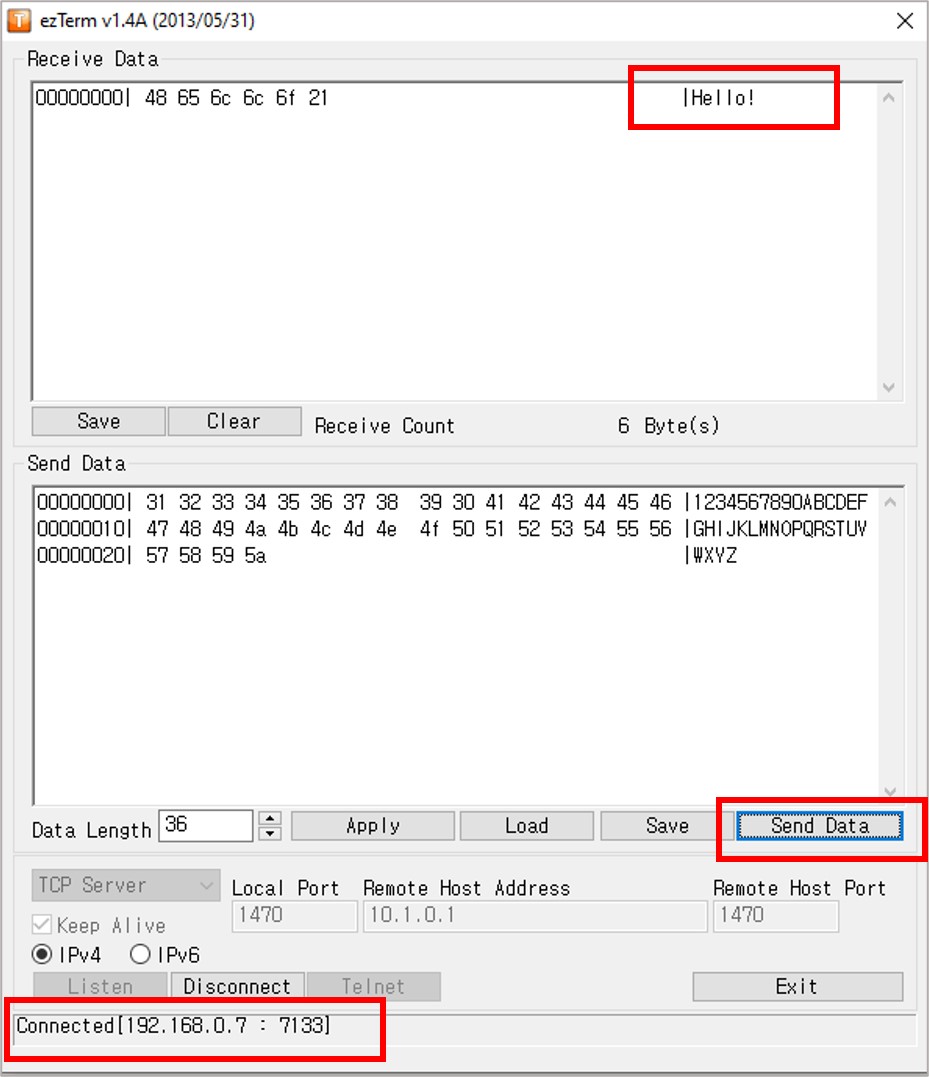
If you want to use a comercial Serial-To-Ethernet converter, you can buy the following commericial products: