Arduino - NeoPixel LED Strip
The NeoPixel RGB LED strip is a strip of LEDs that the color and brightness of each LED on the strip can be controlled individually. In this tutorial, we are going to learn how to use Arduino to control NeoPixel RGB LED strip. To control all LEDs on the NeoPixel strip, we just need to use a single Arduino pin.
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About NeoPixel RGB LED Strip
Pinout
the NeoPixel RGB LED Strip has three pins:
- GND pin: needs to be connected to GND (0V)
- VCC pin: needs to be connected to 5V of external power supply
- Din pin: is pin that receives the control signal. It should be connected to a Arduino pin.
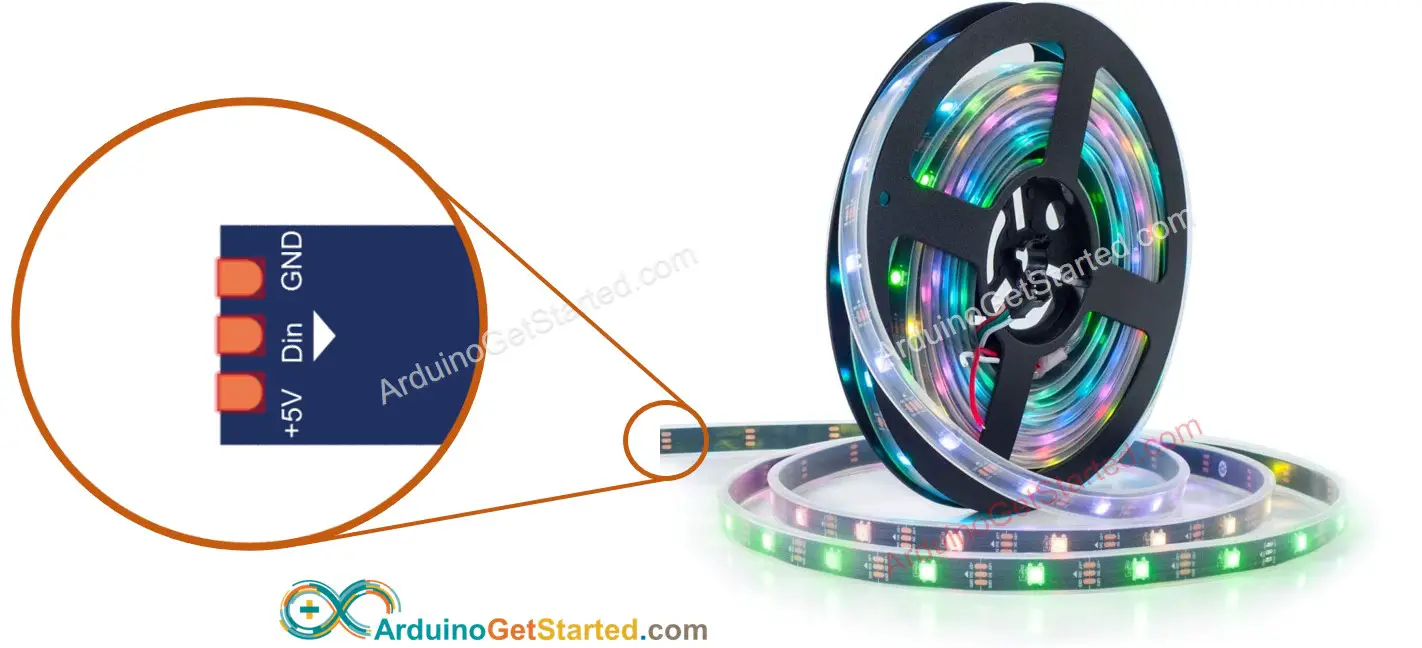
※ NOTE THAT:
The order of pins can vary between manufacturers. ALWAYS use the labels printed on the LED Strip.
Wiring Diagram
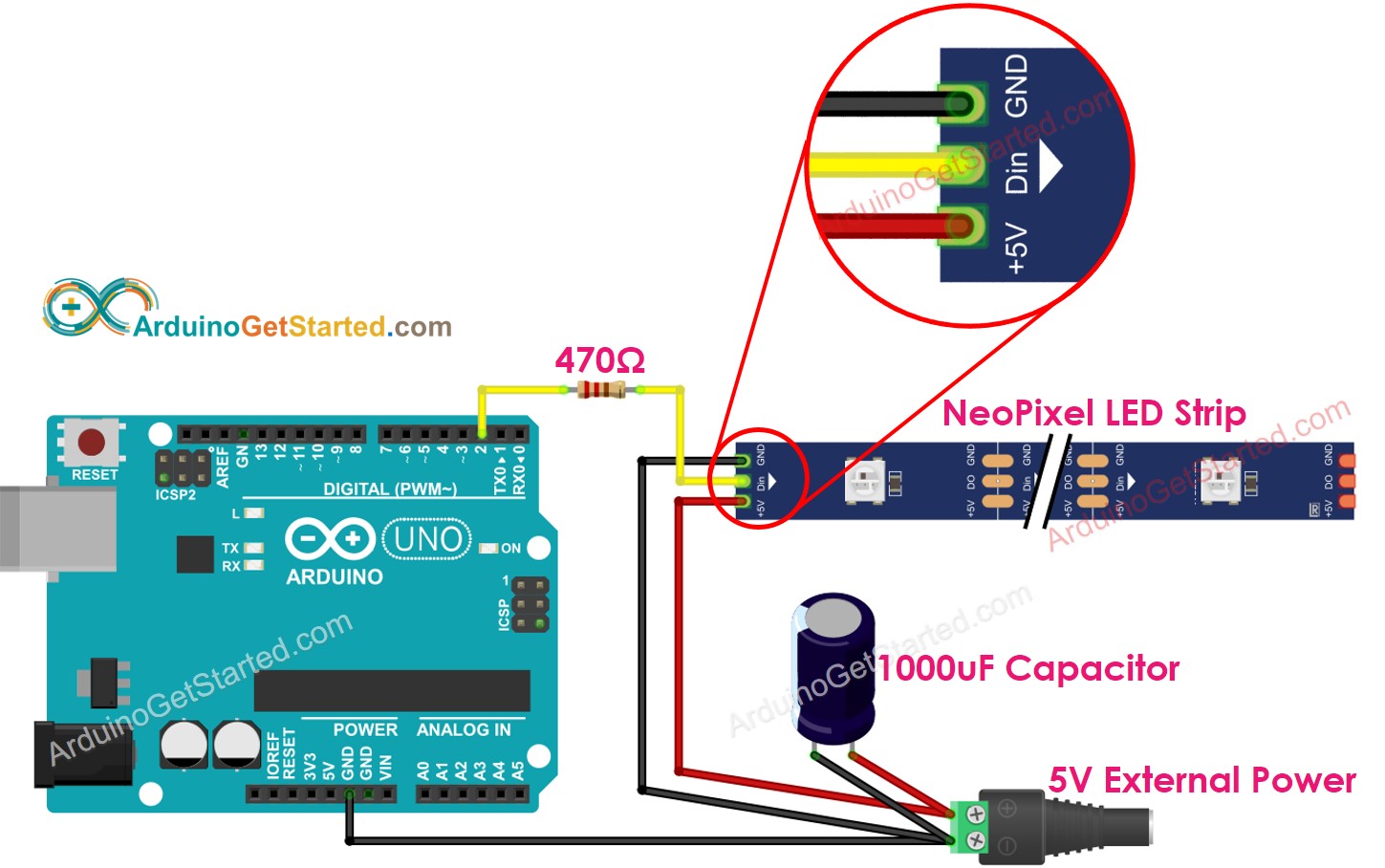
This image is created using Fritzing. Click to enlarge image
How To Program For NeoPixel RGB LED Strip
- Declare a NeoPixel object
- Initializes the NeoPixel
- Set color of each individual LED (called pixel).
- Set brightness of all strip.
※ NOTE THAT:
- NeoPixel.setBrightness() is used for all pixel on LED strip. To set the brightness for each individual pixel, we can scale the color value.
- The values set by NeoPixel.setBrightness() and NeoPixel.setPixelColor() only take effect when NeoPixel.show() is called.
Arduino Code
The below code does:
- Turn pixels to green one by one with a delay between each pixel
- Turn off all pixels for two seconds
- Turn on all pixels to red at the same time for two seconds
- Repeat the above process infinitely
Quick Steps
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search “Adafruit NeoPixel”, then find the NeoPixel library by Adafruit
- Click Install button to install NeoPixel library.
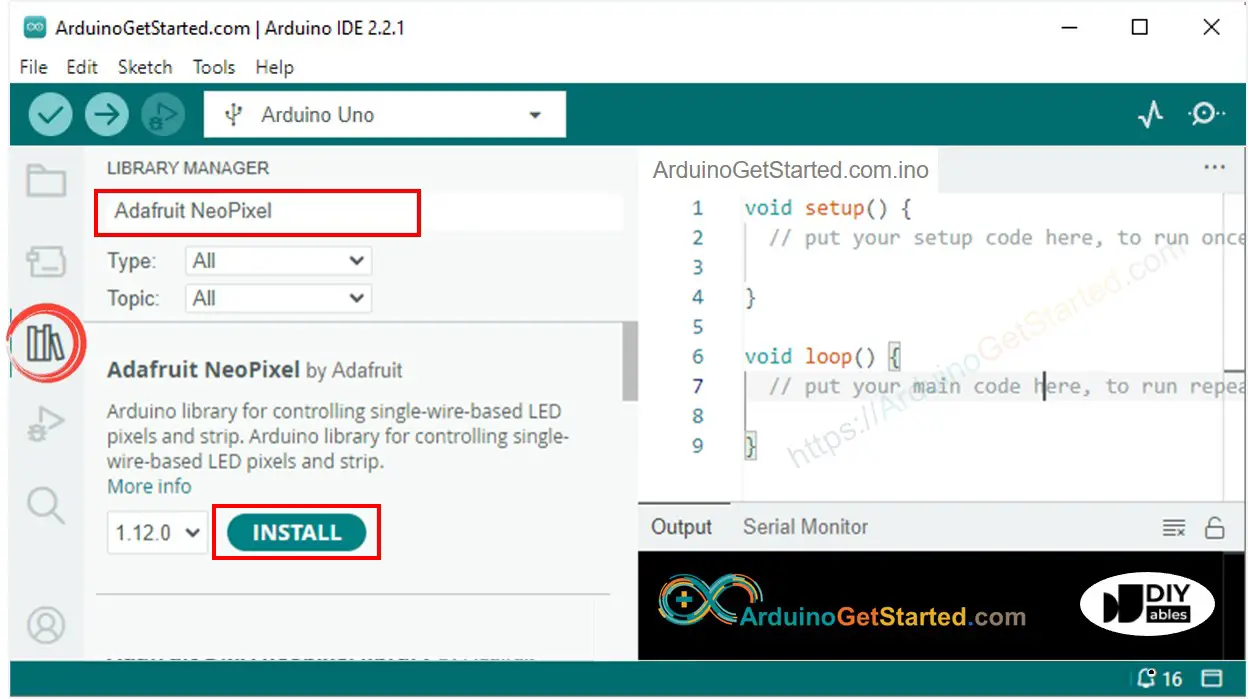
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- See the LED effect
※ NOTE THAT:
For any complicated LED effect, we offer the paid programming service
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.