Arduino - Web Server
In this tutorial, we will learn how to turn an Arduino Uno R4 WiFi into a web server. By accessing the web pages hosted on the Arduino Web Server through a web browser on your PC or smartphone, you'll be able to read values from the Arduino and even control it. Here's a breakdown of what we'll learn to program the Arduino Uno WiFi to achieve:
- Arduino Web Server - Single page: This allows us to monitor sensor values from the Arduino via a web interface.
- Arduino Web Server - Single page: We'll enable the ability to control the Arduino using a web browser.
- Arduino Web Server - Single page: We'll learn to separate HTML content (HTML, CSS, and Javascript) into another file within the Arduino IDE.
- Arduino Web Server - Multiple pages: In this step, we'll create multiple pages such as index.html, temperature.html, led.html, error_404.html, and error_405.html..., and more.
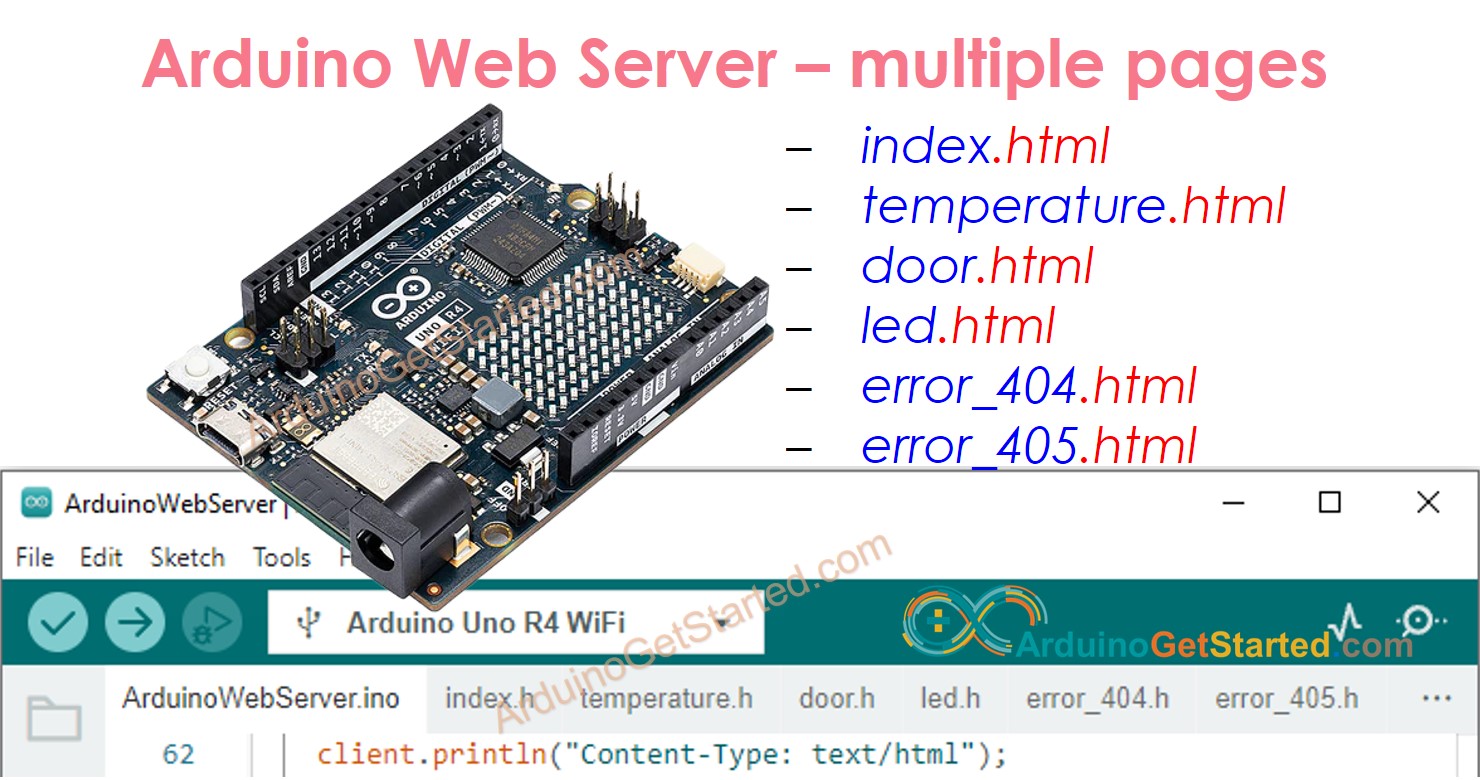
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Reading the sensor value from Arduino via Web
This is relatively simple. The Arduino code does the following tasks:
- Creating a web server that listens for HTTP requests from a web browser.
- Upon receiving a request from a web browser, the Arduino responds with the following information:
- HTTP header
- HTTP body: This includes HTML content and the value read from the sensor.
- If this is the first time you use Arduino Uno R4, see how to setup environment for Arduino Uno R4 on Arduino IDE.
- Copy the above code and open with Arduino IDE
- Change the wifi information (SSID and password) in the code to yours
- Click Upload button on Arduino IDE to upload code to Arduino
- Open the Serial Monitor
- Check out the result on Serial Monitor.
- Take note of the IP address displayed, and enter this address into the address bar of a web browser on your smartphone or PC.
- As a result, you will see the following output on the Serial Monitor.
- Once you access the web browser using the IP address, you will be presented with a very basic web page displaying information about the Arduino board. The page will look like the following:
Below is the Arduino code that performs the above tasks:
Quick Steps
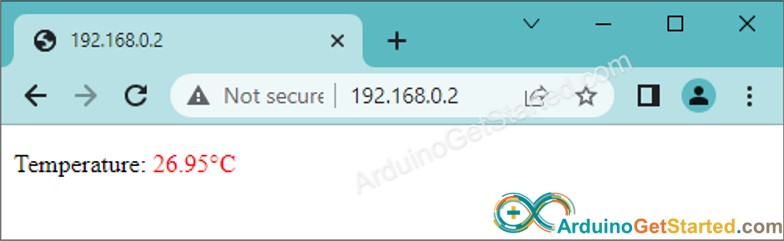
To make the web page look fantastic with a graphic user interface (UI), check out the final section of this tutorial.
Controlling the Arduino via Web
Controlling something connected to Arduino is a bit more challenging than just reading a value. That's because Arduino has to understand the request it receives from the web browser to know what action to take. Here's what the Arduino code does in this case:
- Creating a web server that listens for HTTP requests from a web browser.
- Handling the request received from the web browser by doing the following:
- Reads the HTTP request header.
- Analyzes the HTTP request header to determine the specific control command needed.
- Controls the device or thing connected to the Arduino based on the received control command.
- Sends back an HTTP response.
- Additionally, it can send an HTTP response body with HTML content to display information about the control state (if needed).
For a more comprehensive and detailed example, I recommend checking out the tutorials listed below:
Separating HTML content into another file on Arduino IDE
If you want to create a simple web page with minimal content, you can embed the HTML directly into the Arduino code, as explained earlier.
However, if you want to make a more sophisticated and impressive web page with larger content, it becomes inconvenient to include all the HTML, CSS, and Javascript directly in the Arduino code. In this situation, you can use a different approach to manage the code:
- The Arduino code will be placed in a .ino file, just like before.
- The HTML code (HTML, CSS, Javascript) will be placed in a separate .h file. This allows you to keep the web page content separate from the Arduino code, making it easier to manage and modify.
To do so, we need to do two major steps:
- Preparing HTML content
- Programming Arduino
Preparing HTML content
- Create an HTML file on your local PC that contains the HTML content (HTML, CSS, and Javascript) for your UI design.
- In the HTML file, where data from Arduino should be displayed, use an arbitrary value.
- Test and modify it until you are satisfied.
- In the HTML file, where data from Arduino should be displayed, replace the arbitrary value with a special name, for example, TEMPERATURE_MARKER. Later, in the Arduino code, we will use the String.replace("TEMPERATURE_MARKER", real_value); function to update the value provided by Arduino.
- We will put the HTML content in the .h file on the Arduino IDE. See the next step.
Programming Arduino
- Open the Arduino IDE and create a new sketch. Give it a name, for example, ArduinoGetStarted.com.ino.
- Copy the code provided below and paste it to the created file.
- Change the WiFi information (SSID and password) in the code to yours
- Create the index.h file On Arduino IDE by:
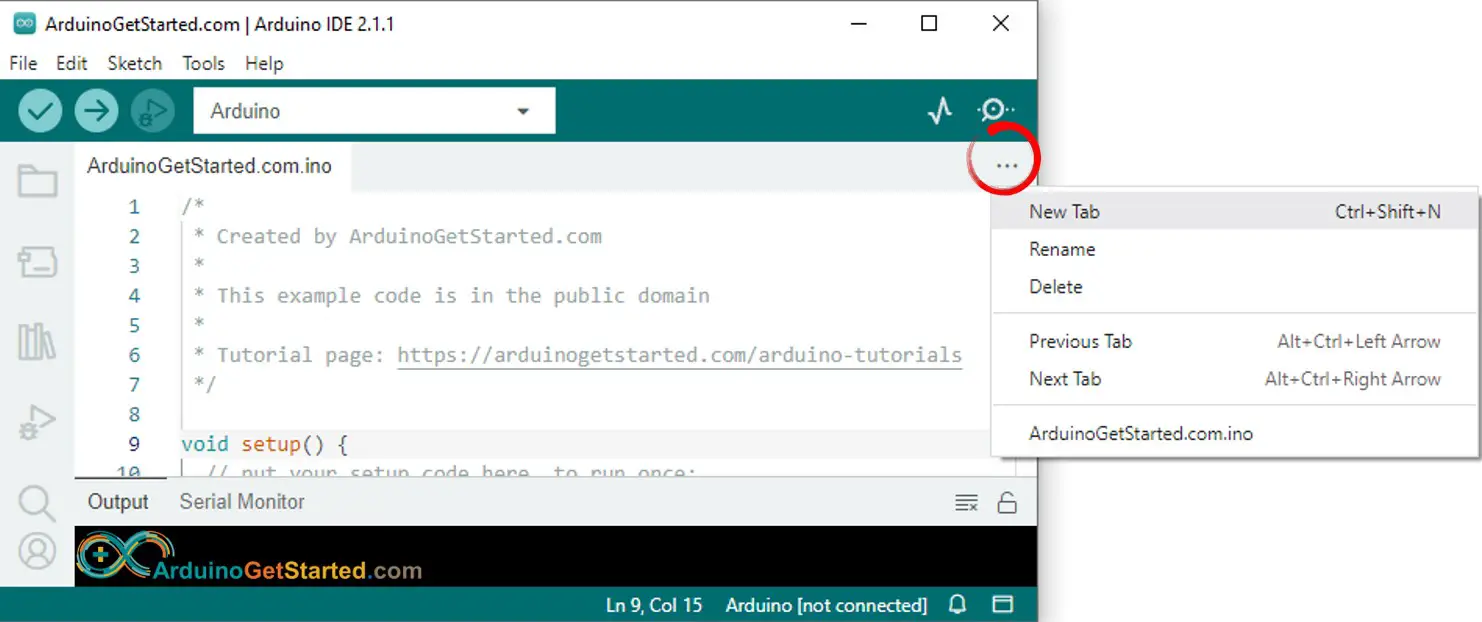
- Either click on the button just below the serial monitor icon and choose New Tab, or use Ctrl+Shift+N keys.
- Give file's name index.h and click OK button
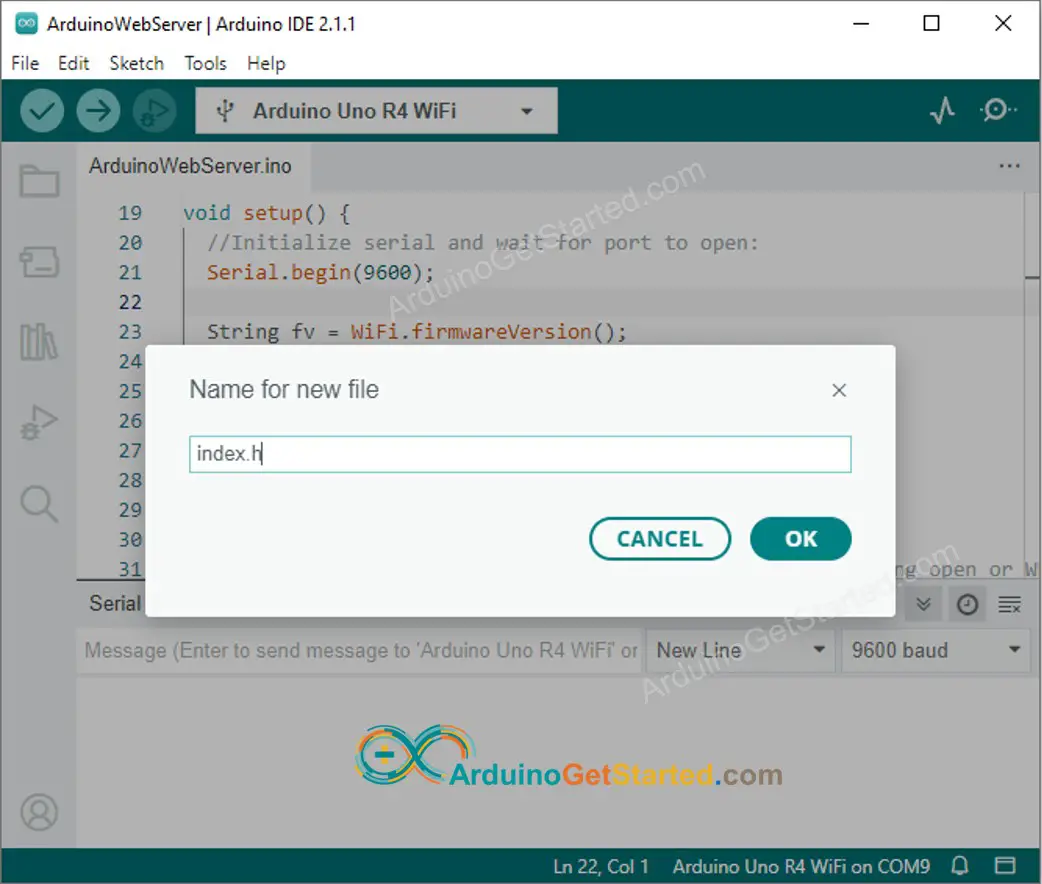
- Copy the below code and paste it to the index.h.
- Replace the line REPLACE_YOUR_HTML_CONTENT_HERE by your HTML content you prepared before. There is no problem with new line character. The below is an example of index.h file:
- Now you have the code in two files: ArduinoGetStarted.com.ino and index.h
- Click Upload button on Arduino IDE to upload code to Arduino
- Access the web page of Arduino board via web browser as before. You will see it as below:
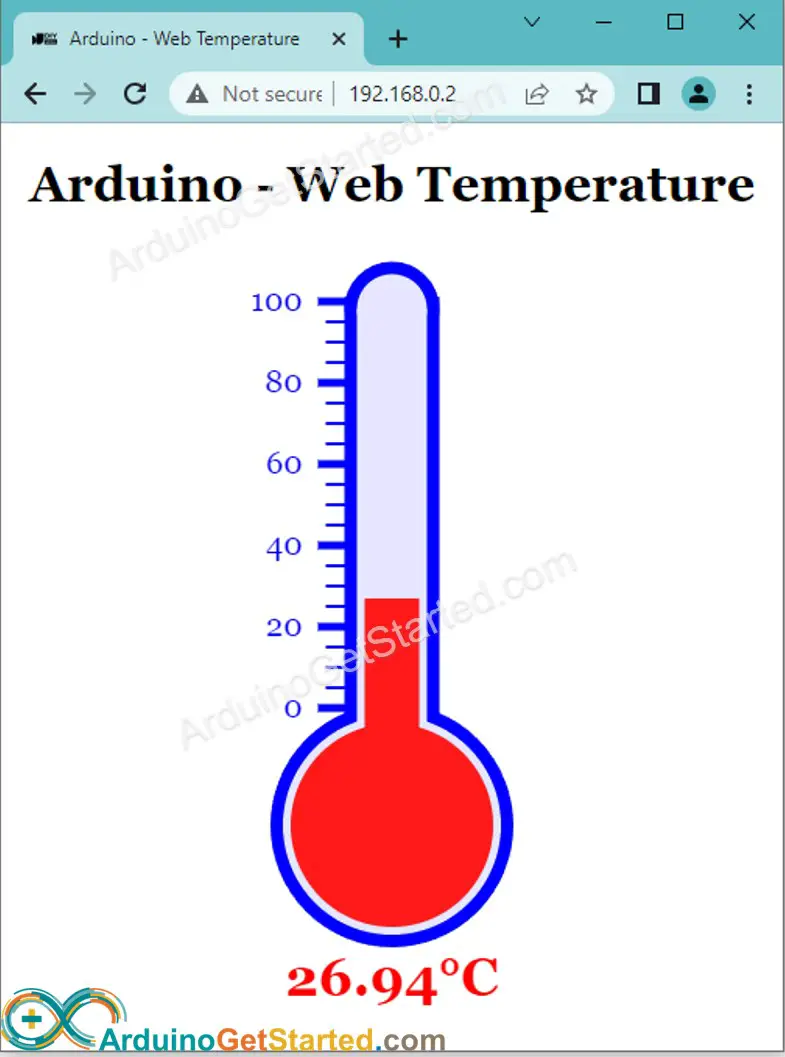
For a more comprehensive and detailed illustration, please refer to the Arduino - DS18B20 Temperature Sensor via Web tutorial
※ NOTE THAT:
- If you make changes to the HTML content within the index.h file but don't modify anything in the ArduinoGetStarted.com.ino file, the Arduino IDE won't refresh or update the HTML content when you compile and upload the code to the ESP32.
- To force the Arduino IDE to update the HTML content in this situation, you need to make a modification in the ArduinoGetStarted.com.ino file. For example, you can add an empty line or insert a comment. This action triggers the IDE to recognize that there have been changes in the project, ensuring that your updated HTML content gets included in the upload.
Arduino Web Server - Multiple Pages
Check out this Arduino - Web Server Multiple Pages tutorial.