Arduino - TM1637 4-Digit 7-Segment Display
A standard 4-digit 7-segment display is needed for clock, timer and counter projects, but it usually requires 12 connections. The TM1637 module makes it easier by only requiring 4 connections: 2 for power and 2 for controlling the segments.
This tutorial will not overload you by deep driving into hardware. Instead, We will learn how to connect the 4-digit 7-segment display to Arduino, how to program it do display what we want.
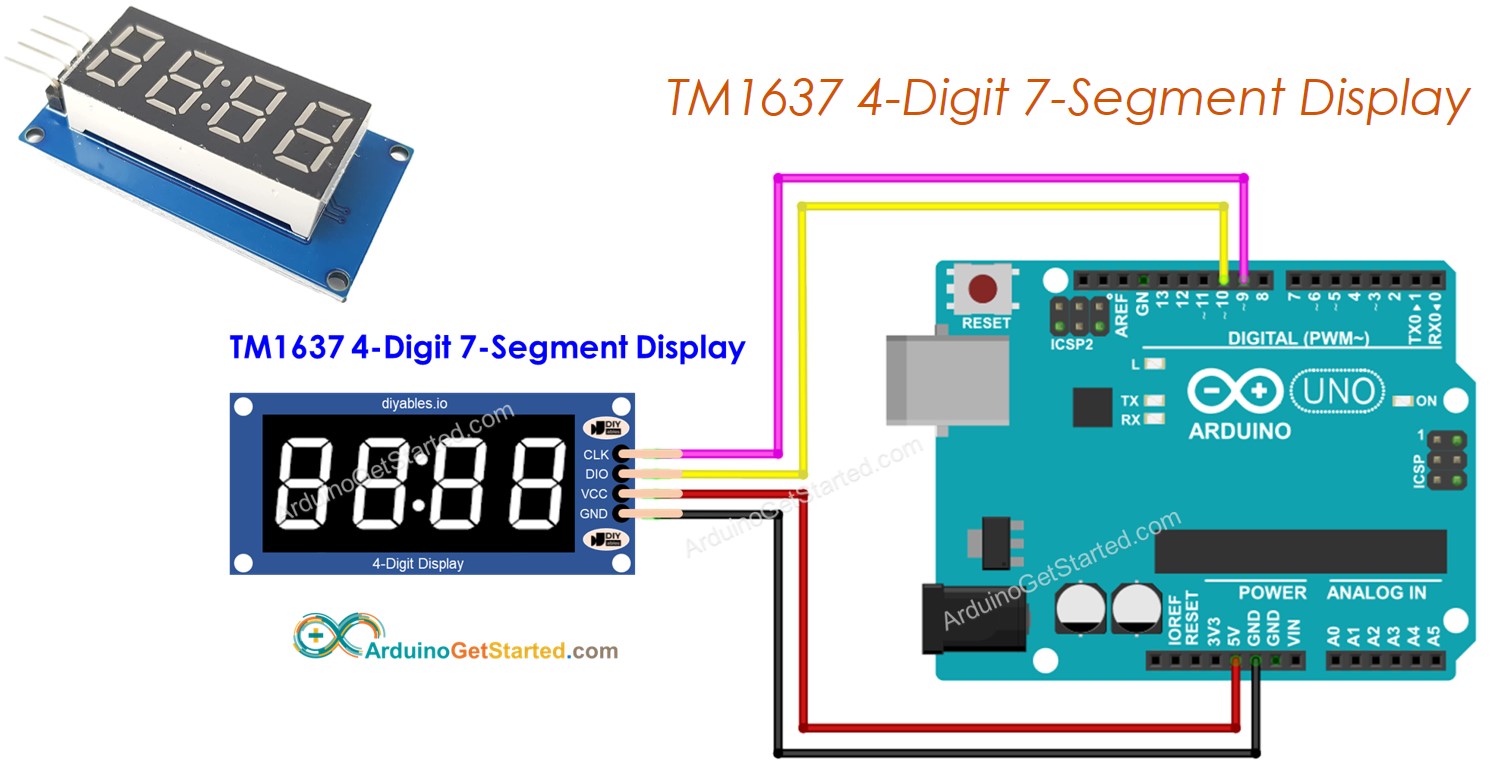
This tutorial are going to use the colon-separated 4-digit 7-segment display module. If you want to display the float numbers, please use the 74HC595 4-digit 7-segment Display Module
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About TM1637 4-digit 7-segment Display
A TM1637 module typically consists of four 7-segment LEDs and a colon-shaped LED in the middle: It is ideal for displaying time in hours and minutes, or minutes and seconds, or scores of two teams.
Pinout
TM1637 4-digit 7-segment display module includes 4 pins:
- CLK pin: is a clock input pin. Connect to any digital pin on Arduino.
- DIO pin: is a Data I/O pin. Connect to any digital pin on Arduino.
- VCC pin: pin supplies power to the module. Connect it to the 3.3V to 5V power supply.
- GND pin: is a ground pin.
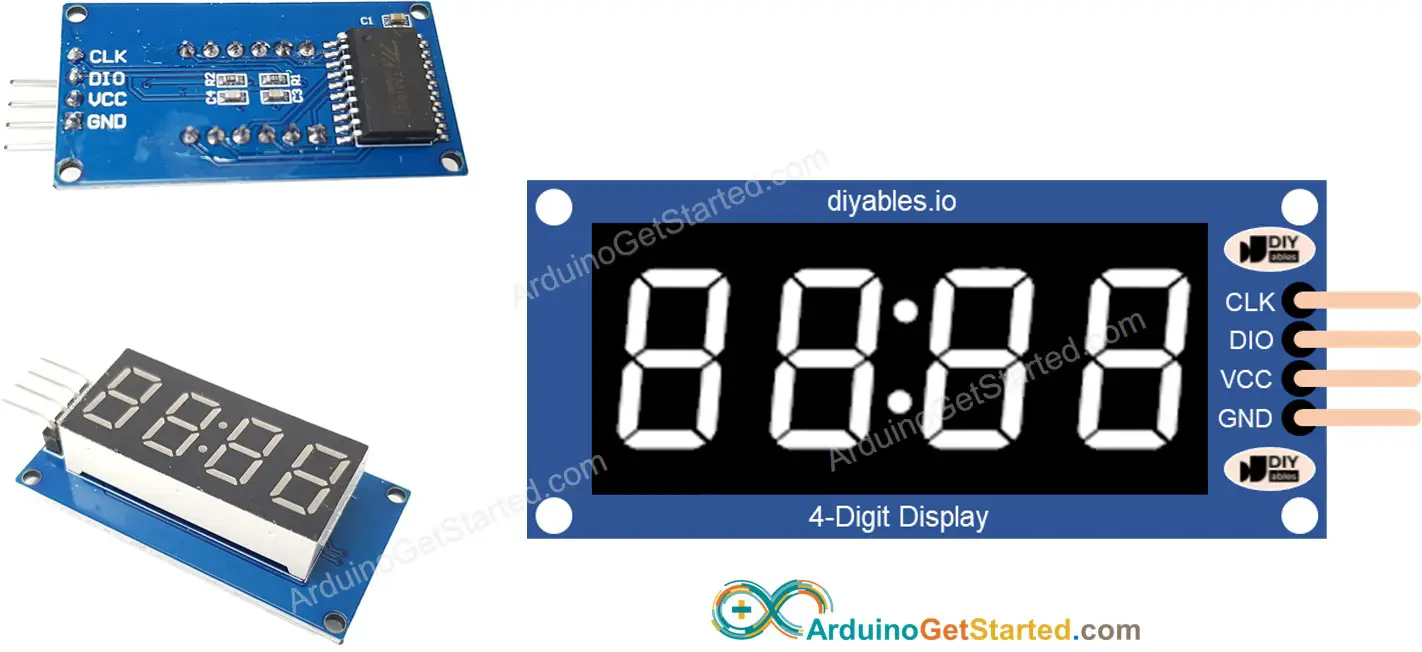
Wiring Diagram
To connect a TM1637 to an Arduino, connect four wires: two for power and two for controlling the display. The module can be powered from the 5-volt output of the Arduino. Connect the CLK and DIO pins to any digital pins of Arduino. For example, 2 and 3 on the Arduino. The pin numbers in the code should be changed if different pins are used.
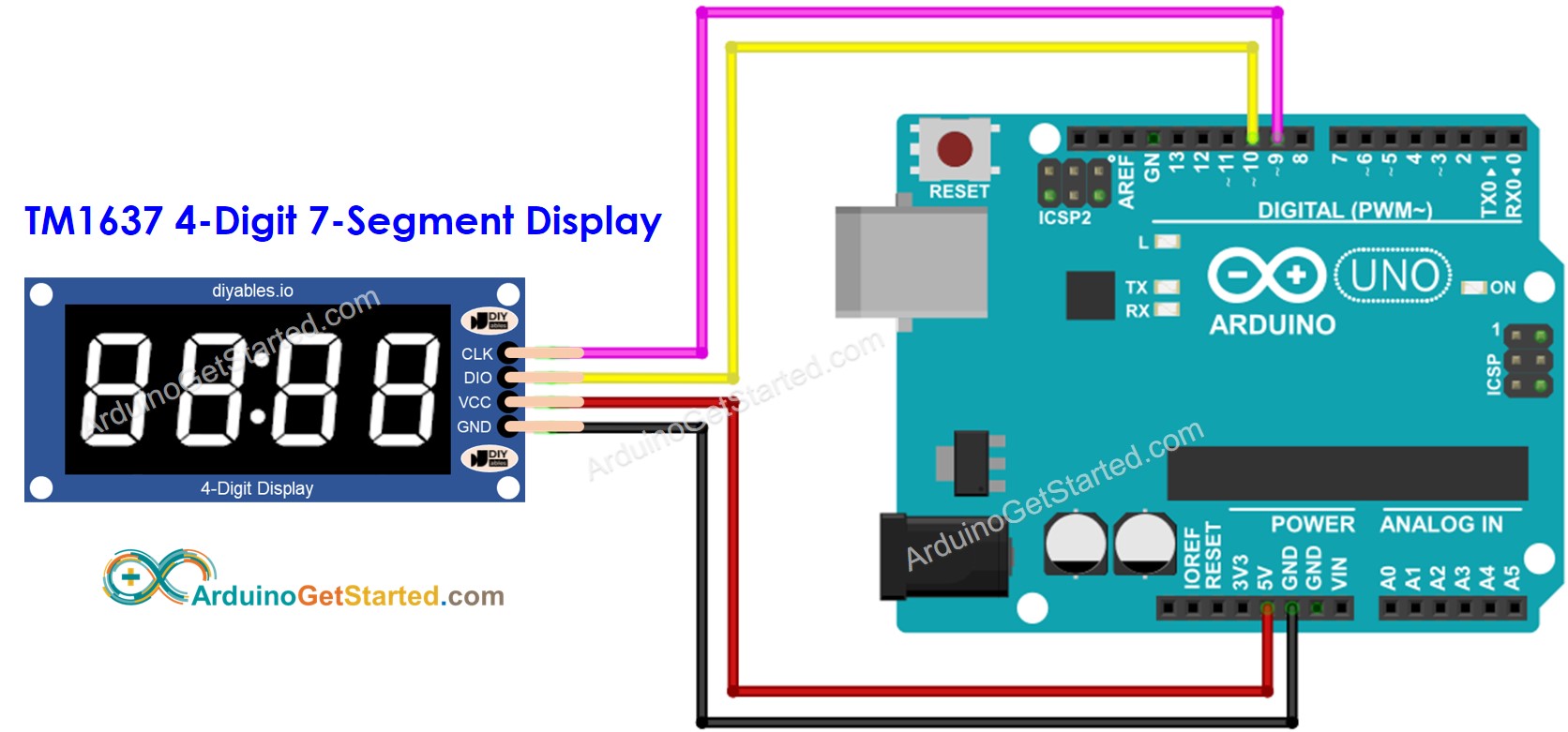
This image is created using Fritzing. Click to enlarge image
Library Installation
To program easily for TM1637 4-digit 7-segment Display, we need to install TM1637Display library by Avishay Orpaz. Follow the below steps to install the library:
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search “TM1637”, then find the TM1637Display library by Avishay Orpaz
- Click Install button.
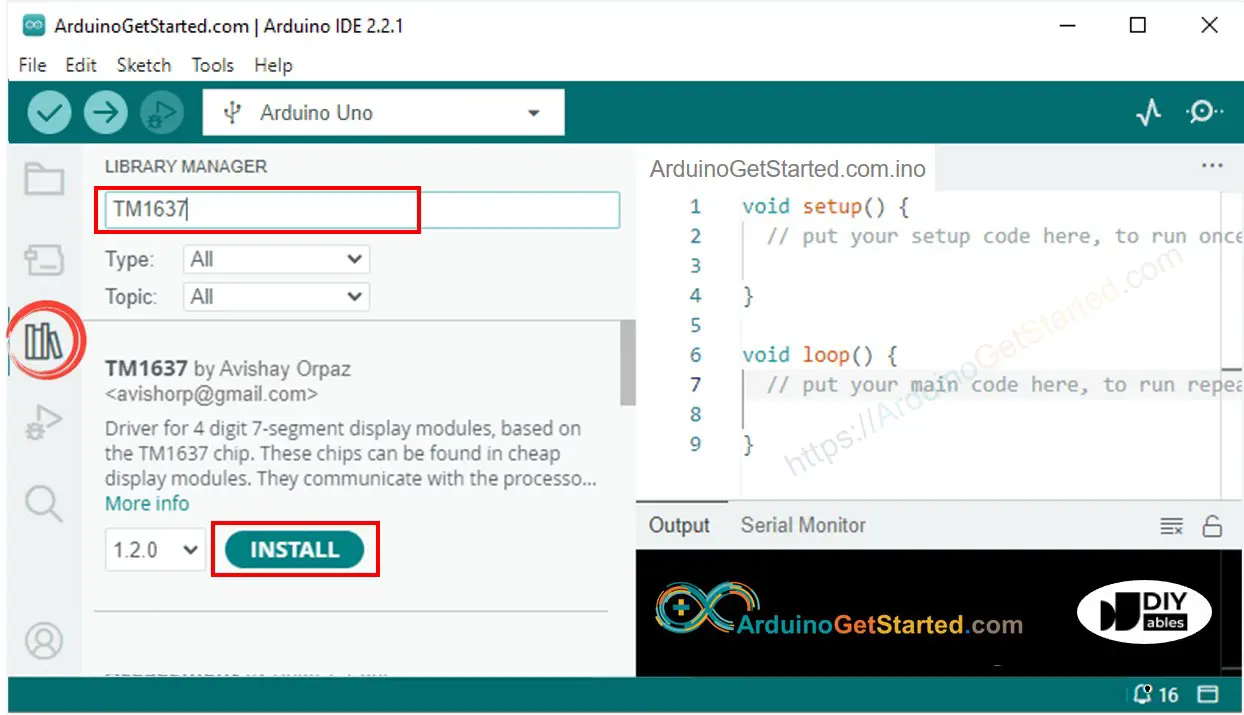
How To Program For TM1637 4-digit 7-segment using Arduino
- Include the library
- Define Arduino's pins that connects to CLK and DIO of the display module. For example, pin D9 and D10
- Create a display object of type TM1637Display
- Then you can display number, number with decimal, number with minus sign, or letter. In the case of leter, you need to define the letter form. Let's see one by one.
- Display number: see below examples, '_' in below description represents for a digit that does not display anything in pratice:
- Display the number with a colon or dot:
You can see more detail in the function references at the end of this tutorial
Arduino Code
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- See the states of the 7-segment display
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.
Function References
The below are references for the following functions:
- display.clear()
- display.showNumberDec()
- display.showNumberDecEx()
- display.setSegments()
- display.setBrightness()
display.clear()
Description
This function clear the display. It turns all LEDs off
display.showNumberDec()
Description
The function is used to display a decimal number on the 7-segment display.
Syntax
Parameter
- num: This is the number to be displayed on the 7-segment display. It should be within the range of -9999 to 9999.
- leading_zero: This is an optional parameter with a default value of false. If it is set to true, leading zeros will be displayed.
- length: This is an optional parameter with a default value of 4. It sets the number of digits to be displayed on the 7-segment display.
- pos: This is an optional parameter with a default value of 0. It sets the position of the most significant digit of the number.
Please note that, if the number is out of range or if the value of length is greater than 4, the function will not display anything.
showNumberDecEx()
Description
The function is used to display a decimal number on the 7-segment display with additional features compared to the showNumberDec() function. It is an advanced version of showNumberDec() that allows you to control the dot or colon segments of each digit individually.
Syntax
Parameter
- num1: This is the number to be displayed on the 7-segment display. It should be within the range of -9999 to 9999.
- dots: This parameter is used to specify which segments of the display should be turned on as dots. Each bit of the value corresponds to a digit on the display: Valid value
- 0b10000000: display the first dot: 0.000
- 0b01000000: display the second dot: 00.00
- 0b00100000: display the third dot: 000.0
- 0b01000000: For displays with just a colon: 00:00
- leading_zero: This is an optional parameter with a default value of false. If it is set to true, leading zeros will be displayed.
- length: This is an optional parameter with a default value of 4. It sets the number of digits to be displayed on the 7-segment display.
- pos: This is an optional parameter with a default value of 0. It sets the position of the most significant digit of the number.
For example, if you call display.showNumberDecEx(1530,0b01000000); it will display the number 15:30 on the 7-segment display.
Please note that, if the number is out of range or if the value of length is greater than 4, the function will not display anything.
setSegments()
Description
The function is used to set the segments of the 7-segment display directly. It can be used to dislay letters, special character, or turn all all LED segment.
Syntax
Parameter
- segments: This parameter sets the segments of the 7-segment display, it's an array of bytes, where each byte represents the segments of each digit. Each segment is represented by a bit in the byte.
- length: This is an optional parameter with a default value of 4. It sets the number of digits to be displayed on the 7-segment display.
- pos: This is an optional parameter with a default value of 0. It sets the position of the most significant digit of the number.
This function is useful when you want to display characters or symbols that are not included in the basic 7-segment display. By setting the segments directly, you can display any pattern you want.
Please note that, if the number is out of range or if the value of length is greater than 4, the function will not display anything.
setBrightness()
Description
The function is used to set the brightness of the 7-segment display.
Syntax
Parameter
- brightness: This parameter sets the brightness level of the 7-segment display. The value should be in the range of 0 to 7. A higher value results in a brighter display.
- on: This is an optional parameter with a default value of true. It's used to turn on or off the display. If it's set to false, the display will be turned off.