Arduino - Water Sensor Relay
In this tutorial, we will learn how to use an Arduino to activate a relay when it detects water. Then you can connect the relay to a pump, or a siren, buzzer to make a water alarm.
Hardware Required
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About Water Sensor and Relay
If you do not know about water sensor and relay (pinout, how it works, how to program ...), learn about them in the following tutorials:
- Arduino - Water Sensor tutorial
- Arduino - Relay tutorial
How It Works
Arduino periodically reads the value from the water sensor. If the value exceeds a pre-defined threshold, the Arduino activates the relay; otherwise, it deactivates the relay.
Wiring Diagram
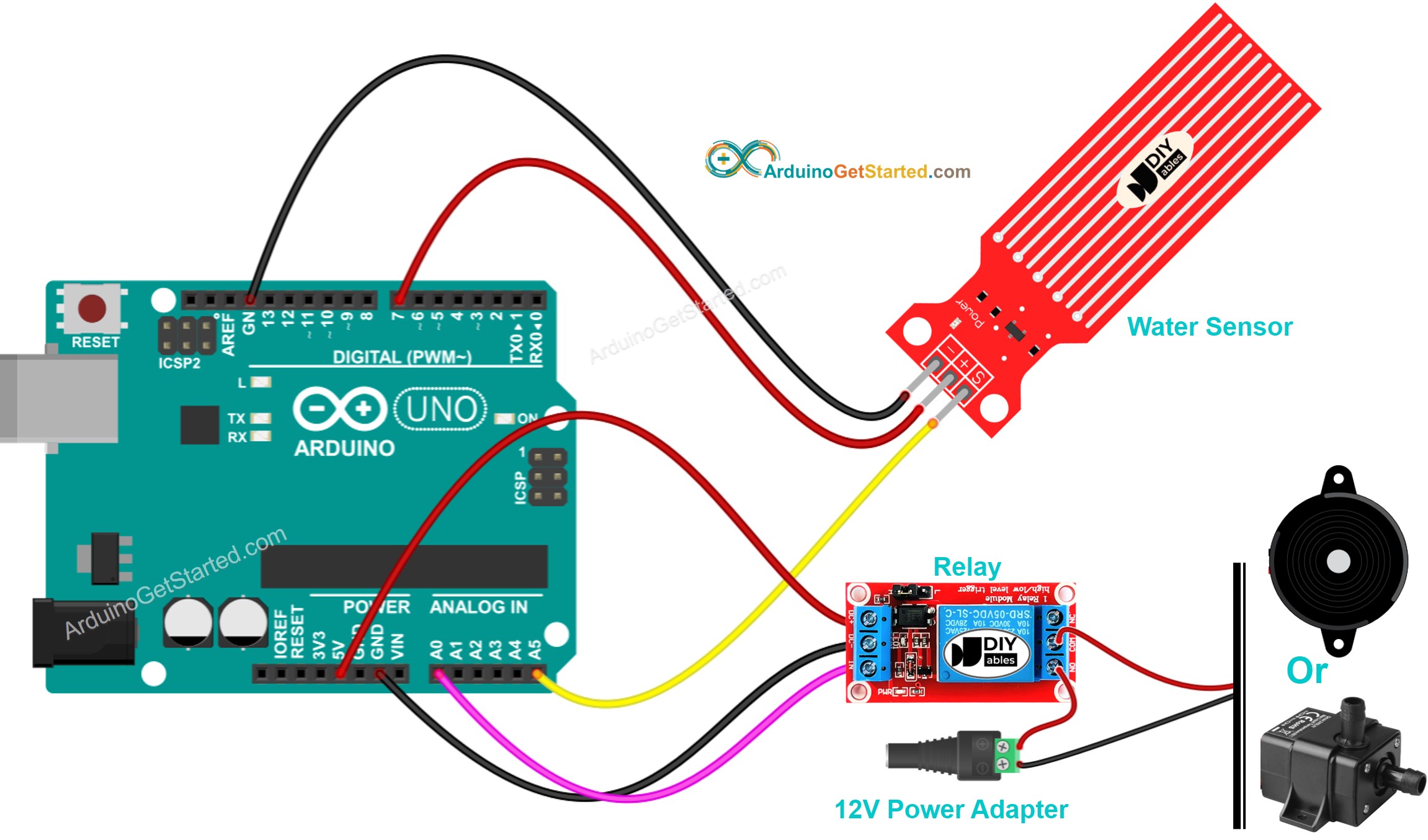
This image is created using Fritzing. Click to enlarge image
Arduino Code
Quick Steps
- Connect Arduino to PC via USB cable
- Open Arduino IDE, select the right board and port
- Copy the above code and open with Arduino IDE
- Update the value of THRESHOLD in the code to a suitable value for your application
- Click Upload button on Arduino IDE to upload code to Arduino
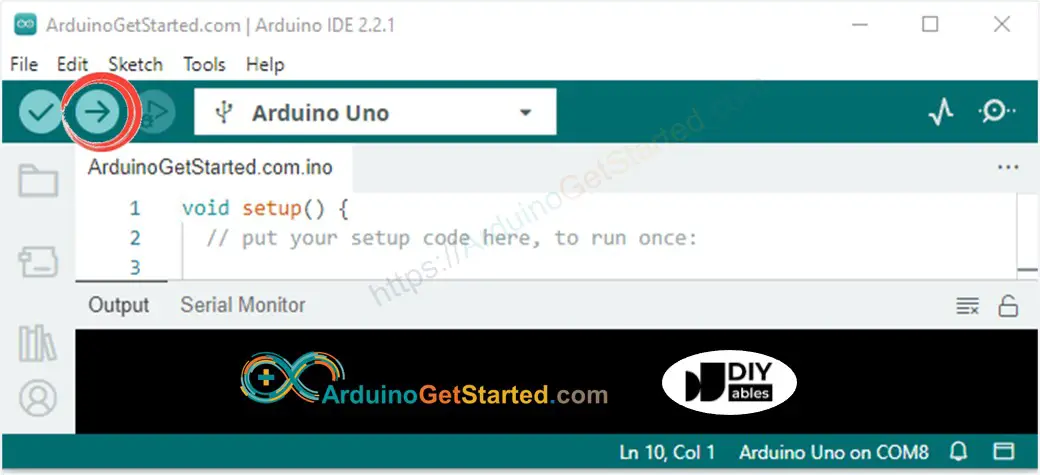
- Embed the water sensor into the water
- See the relay's state
Code Explanation
Read the line-by-line explanation in comment lines of source code!
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.