Arduino - IR Remote Control
You've likely encountered the infrared remote controller, also known as the IR remote controller, while using home electronic devices like TVs and air conditioners... In this tutorial, we are going to learn how to use infrared (IR) remote controller and infrared receiver to control Arduino. In detail, we will learn:
- How to connect an IR receiver to Arduino board
- How to program Arduino to read the command from IR remote controller via IR receiver
Then you can modify the code to control LED, fan, pump, actuator... via IR remote controller.
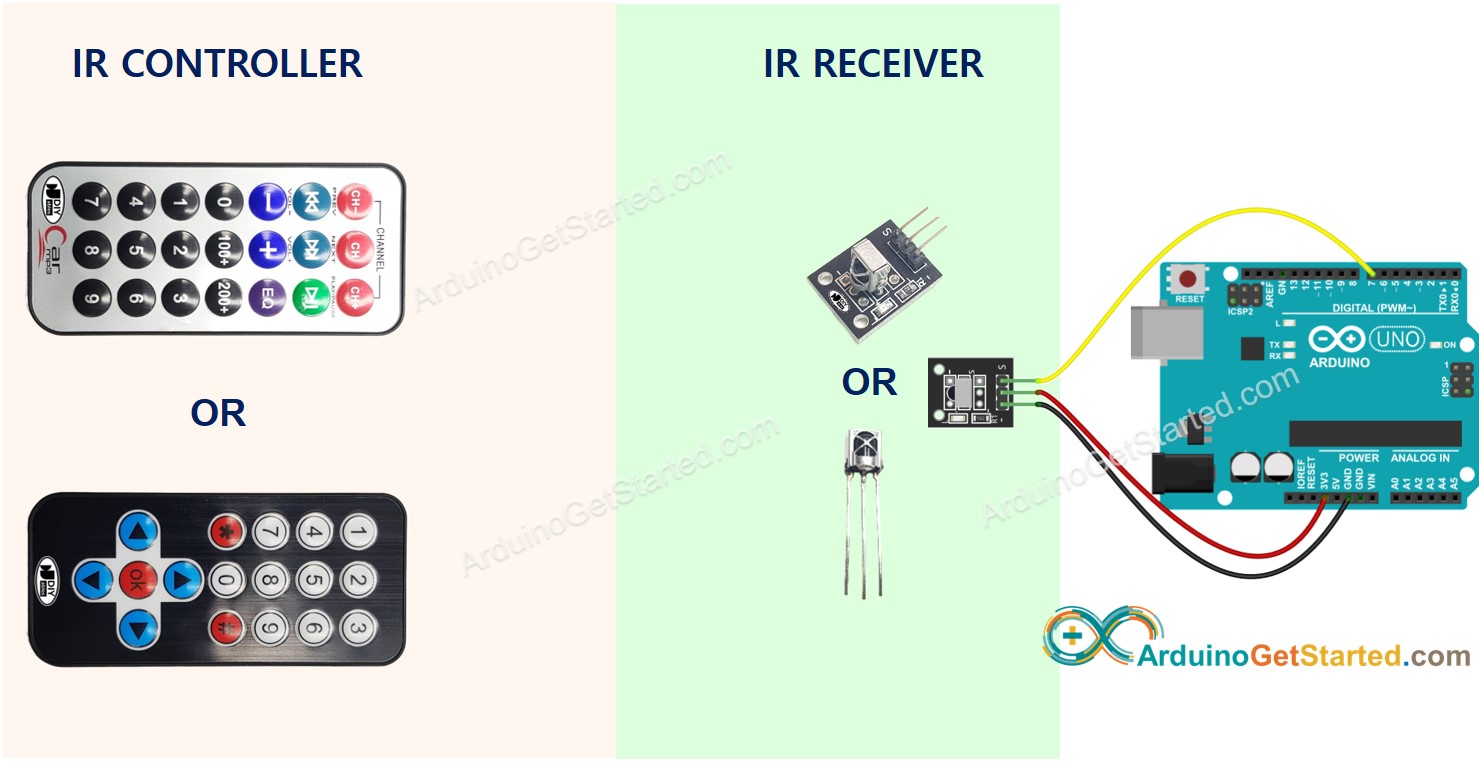
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About IR Remote Control
An IR control system includes two components:
- IR remote controller
- IR receiver
An IR kit usually includes two above components.
IR remote controller
The IR remote controller is a handheld device that emits infrared signals. The IR remote controller consists of a keypad with various buttons:
- Each button on the remote controller corresponds to a specific function or command.
- When a button is pressed, the remote emits an infrared signal that carries a unique code or pattern associated with the pressed button.
- These infrared signals are not visible to the human eye as they are in the infrared spectrum.
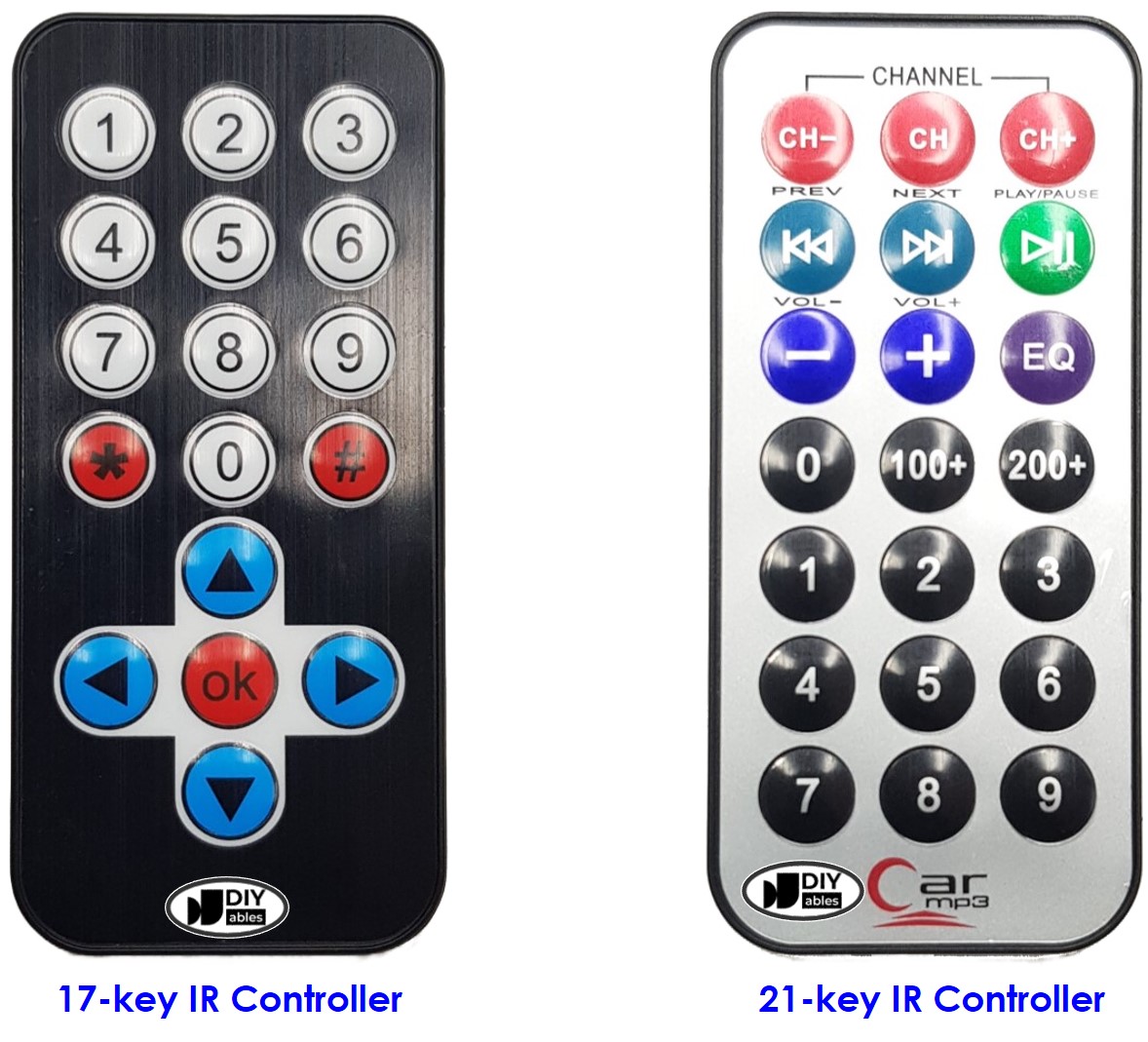
IR Receiver
The IR receiver module is a sensor that detects and receives the infrared signals emitted by the remote controller.
The infrared receiver detects the incoming infrared signals and converts them into the code (command) representing the button pressed on the remote controller.
The IR Receiver can be a sensor or a module. You can use the following choices:
- IR Receiver Module only
- IR Receiver Sensor only
- IR Receiver Sensor + Adapter
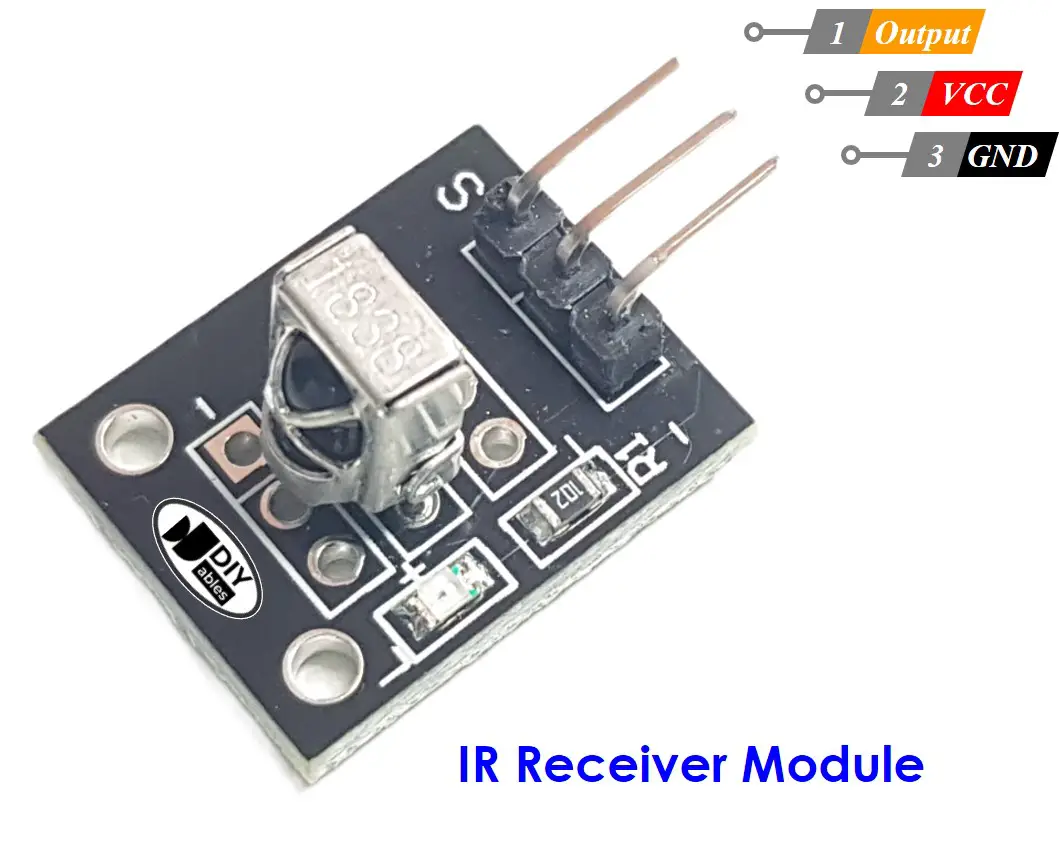
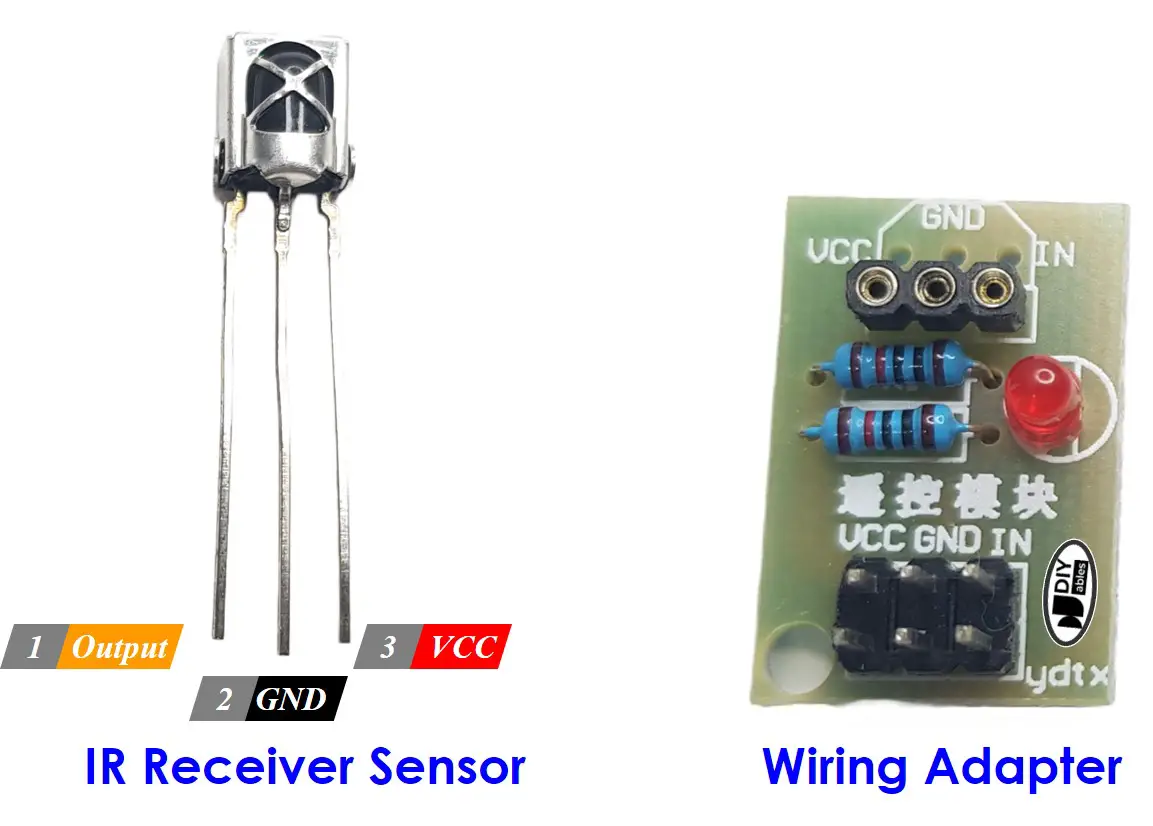
IR Receiver Pinout
IR receiver module or sensor has three pins:
- VCC pin: Connect this pin to the 3.3V or 5V pin of the Arduino or external power source.
- GND pin: Connect this pin to GND pin of the Arduino or external power source..
- OUT (Output) pin: This pin is the output pin of the IR receiver module. Connected to a digital input pin on the Arduino.
How It Works
When user presses a button on the IR remote controller
- The IR remote controller encodes the command corresponding to the button to the infrared signal via a specific protocol
- The IR remote controller emits the encoded infrared signal
- The IR receiver receives the encoded infrared signal
- The IR receiver decoded the encoded infrared signal in to the command
- The Arduino reads the command from the IR receiver
- The Arduino maps the command to the key pressed
It seems to be complicated but don't worry. With the help of DIYables_IRcontroller library, it is a piece of cake.
Wiring Diagram
Wiring diagram between Arduino and IR Receiver Module
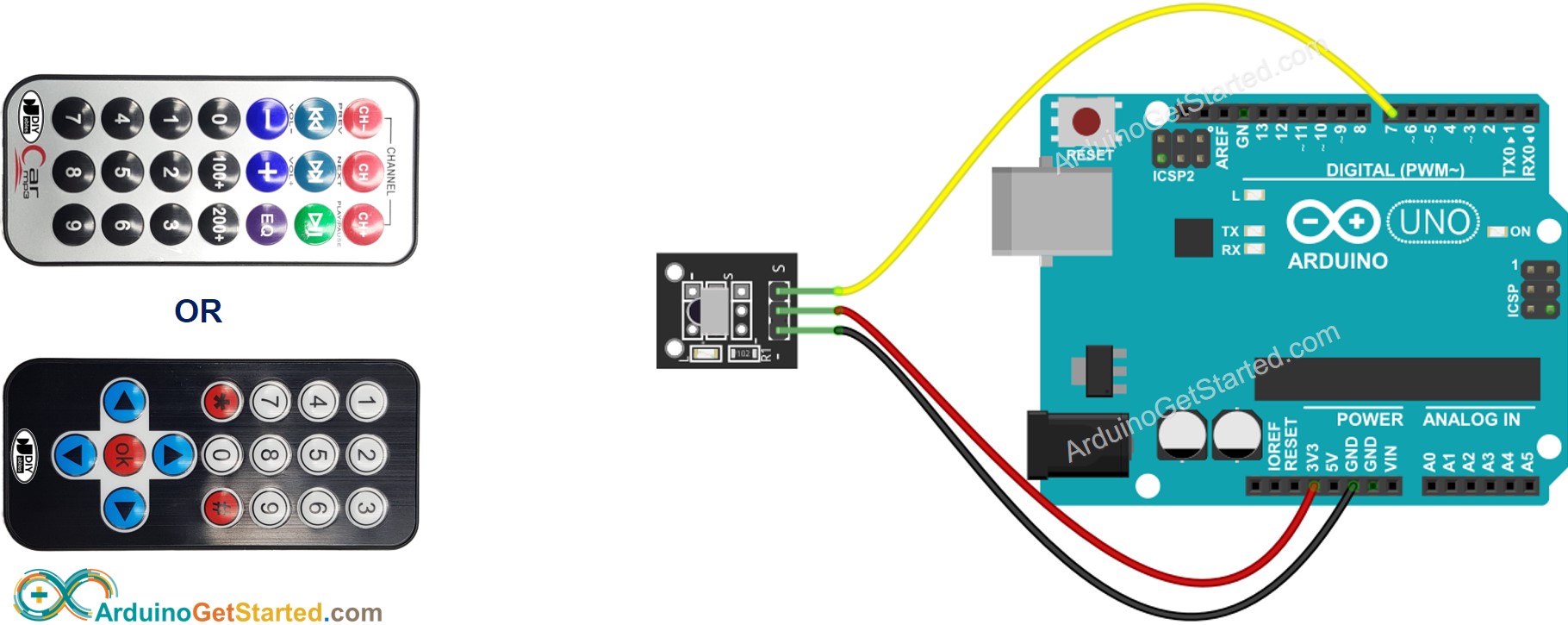
This image is created using Fritzing. Click to enlarge image
The real wiring diagram
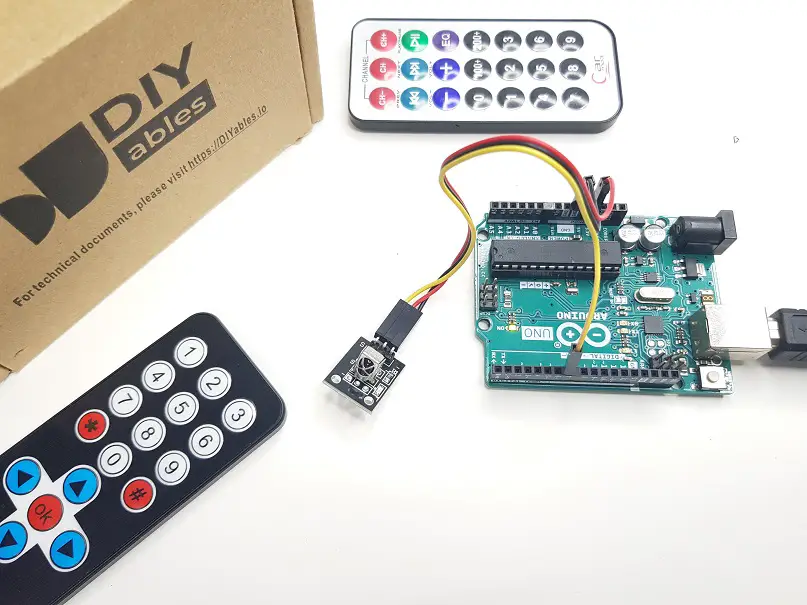
Wiring diagram between Arduino and IR Receiver Sensor
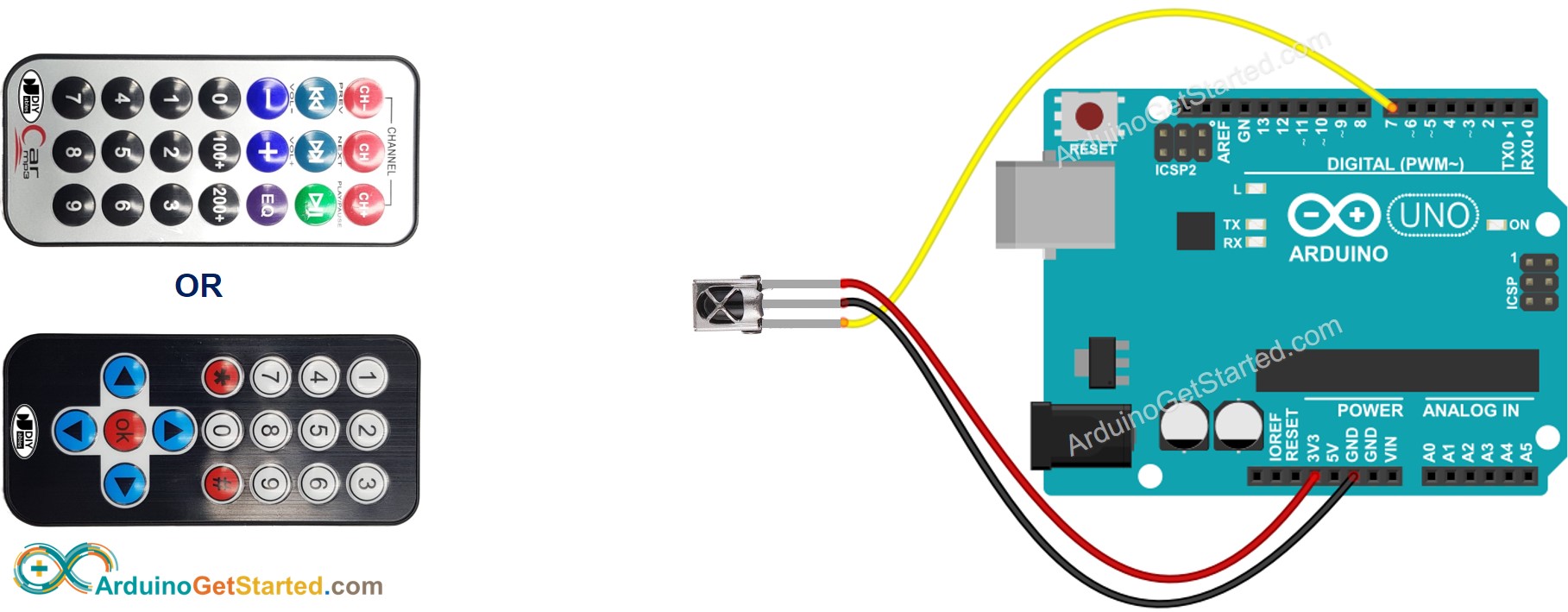
This image is created using Fritzing. Click to enlarge image
The real wiring diagram
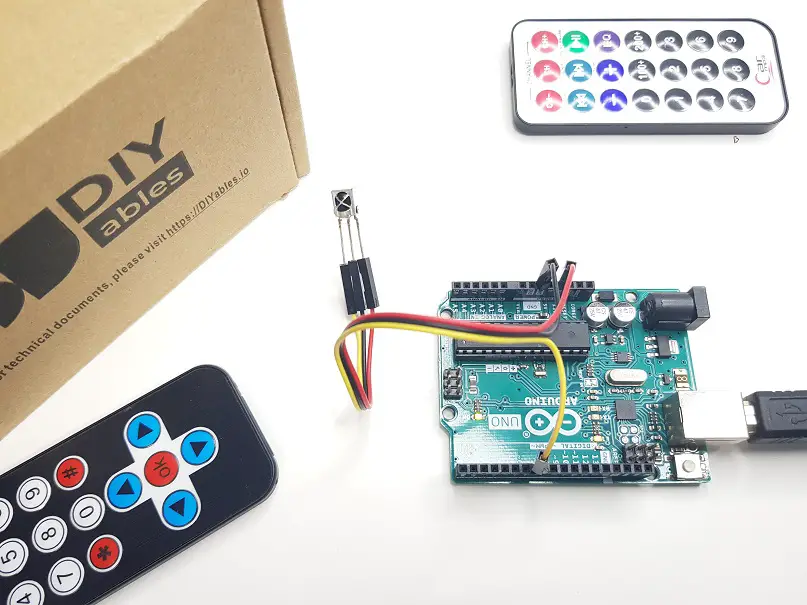
Wiring diagram between Arduino and IR Receiver Sensor and Adapter
You can also connect The IR receiver sensor to the adapter before connecting to the Arduino.
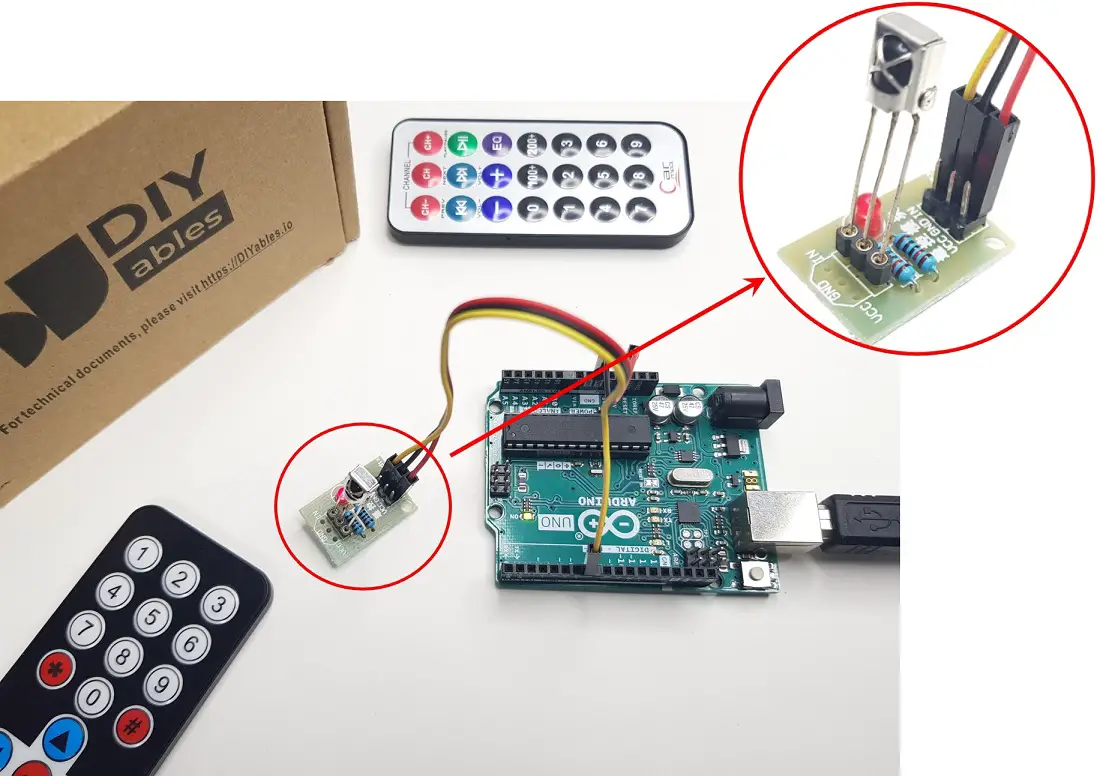
How To Program For IR Remote Controller
- Include the library:
- Declare a DIYables_IRcontroller_17 or DIYables_IRcontroller_21 object corresponds with 17-key or 21-key IR remote controllers:
- Initialize the IR Controller.
- In the loop, check if a key is pressed or not. If yes, get the key
- Once you have detected a key press, you can perform specific actions based on each key.
Arduino Code
- Arduino code for DIYables 17-key IR remote controller
- Arduino code for DIYables 21-key IR remote controller
Quick Steps
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search "DIYables_IRcontroller", then find the DIYables_IRcontroller library by DIYables
- Click Install button to install DIYables_IRcontroller library.
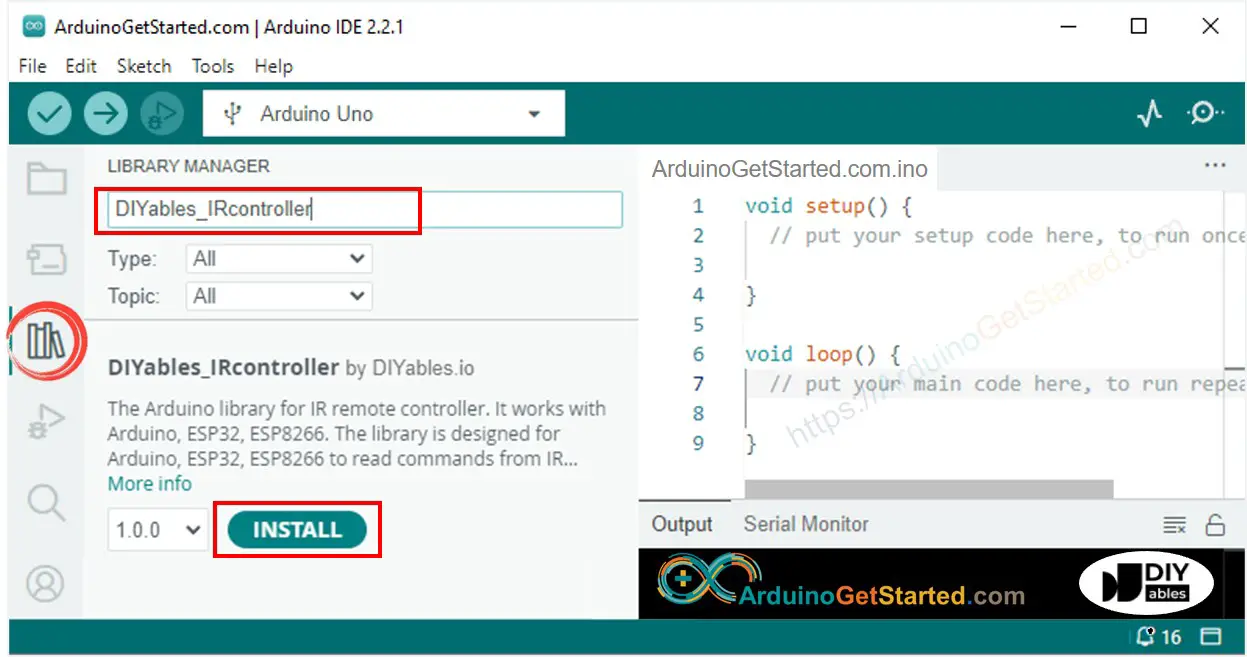
- You will be asked for installing the library dependency as below image:
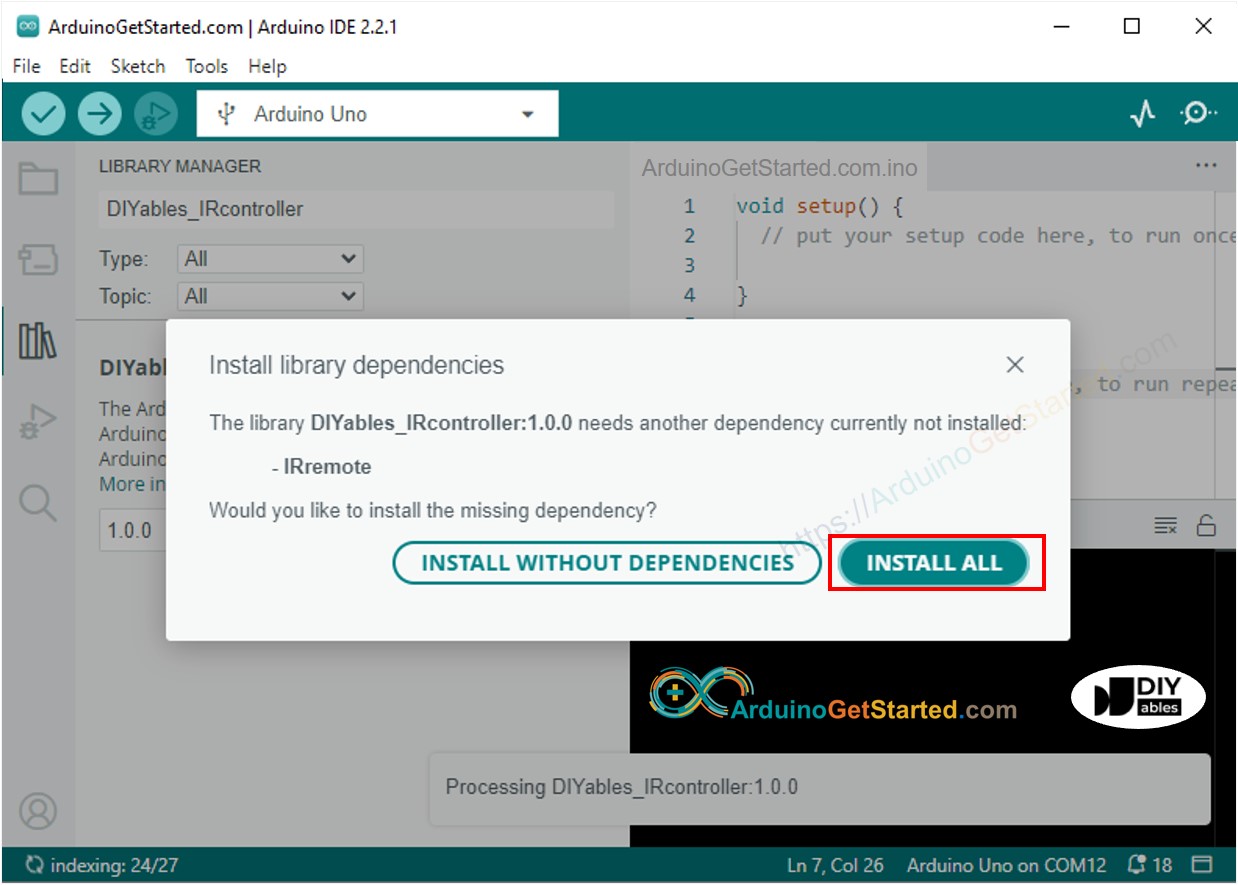
- Click Install all button to install the dependency
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Press keys on the remote controller one by one
- See the result on Serial Monitor.
- The below is the result when you press keys on 21-key IR controller one by one:
Now you can modify the code to control LED, fan, pump, actuator... via IR remote controllers.
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.