Arduino - 4-Channel Relay Module
When we want to control 4 high-voltage devices such as pumps, fans, actuators... We can use multiple relay modules. However, there is a simpler way is to use a 4-channel relay module. A 4-channel relay module is a combination of 4 relays on a single board.
A 4-channel relay module vs 4 x 1-channel relay modules:
- A 4-channel relay module has simpler wiring.
- A 4-channel relay module uses less space.
- A 4-channel relay module is cheaper.
- The programming is the same.
Hardware Required
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About 4-Channel Relay Module
Pinout
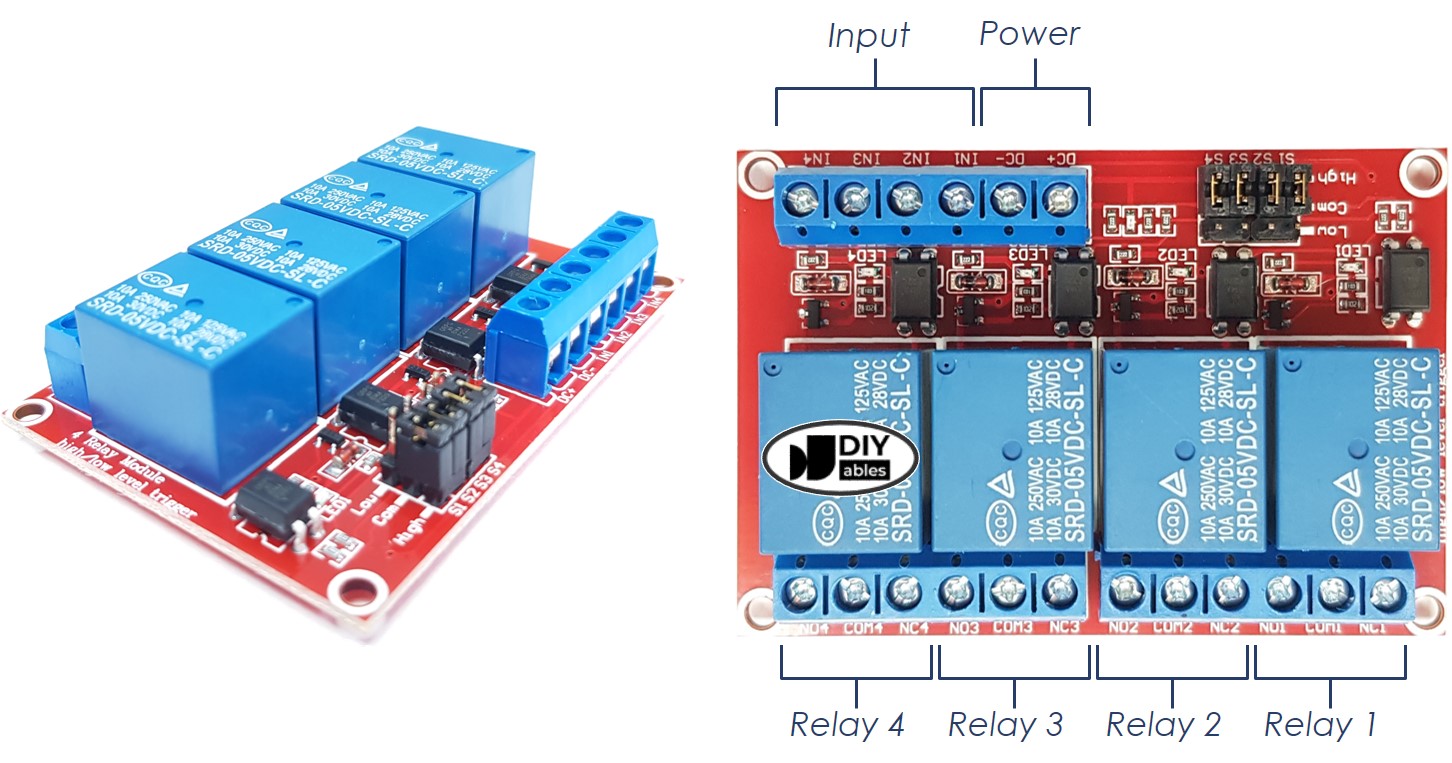
A 4-channel relay module has the following pins:
- Power pins for relay boards
- DC+: connect this pin to 5V pin of power supply
- DC-: connect this pin to the GND pin of the power supply and also to the GND pin of the Arduino
- Signal pins:
- IN1: this pin receives the control signal from Arduino to control relay 1 on the module
- IN2: this pin receives the control signal from Arduino to control relay 2 on the module
- IN3: this pin receives the control signal from Arduino to control relay 3 on the module
- IN4: this pin receives the control signal from Arduino to control relay 4 on the module
- Output pins: NCx (normally closed pin), NOx (normally open pin), COMx (common pin),
- NC1, NO1, COM1: These pins connect to a high-voltage device that is controlled by relay 1
- NC2, NO2, COM2: These pins connect to a high-voltage device that is controlled by relay 2
- NC3, NO3, COM3: These pins connect to a high-voltage device that is controlled by relay 3
- NC4, NO4, COM4: These pins connect to a high-voltage device that is controlled by relay 4
For detail of how to connect relay to high-voltage, what are differences between normally closed and normally open, see Arduino - Relay tutorial
It also has 4 jumpers to select between the low trigger and the high trigger for each relay individually.
Wiring Diagram
4-channel relay module consumes considerable power. Therefore, we should NOT power the module directly from the 5V pin of Arduino. We need to use external 5V power for the module instead.
So, we need to use three power sources:
- A 5V power adapter for Arduino
- A 5V power adapter for the 4-channel relay module
- A higher-voltage power adapter (12VDC, 24VDC, 48VDC, 220AC...) for things that are controlled by the 4-channel relay module
- Wiring diagram with three power sources. Power supply for Arduino (not included in the image) can be via either USB cable or power jack.
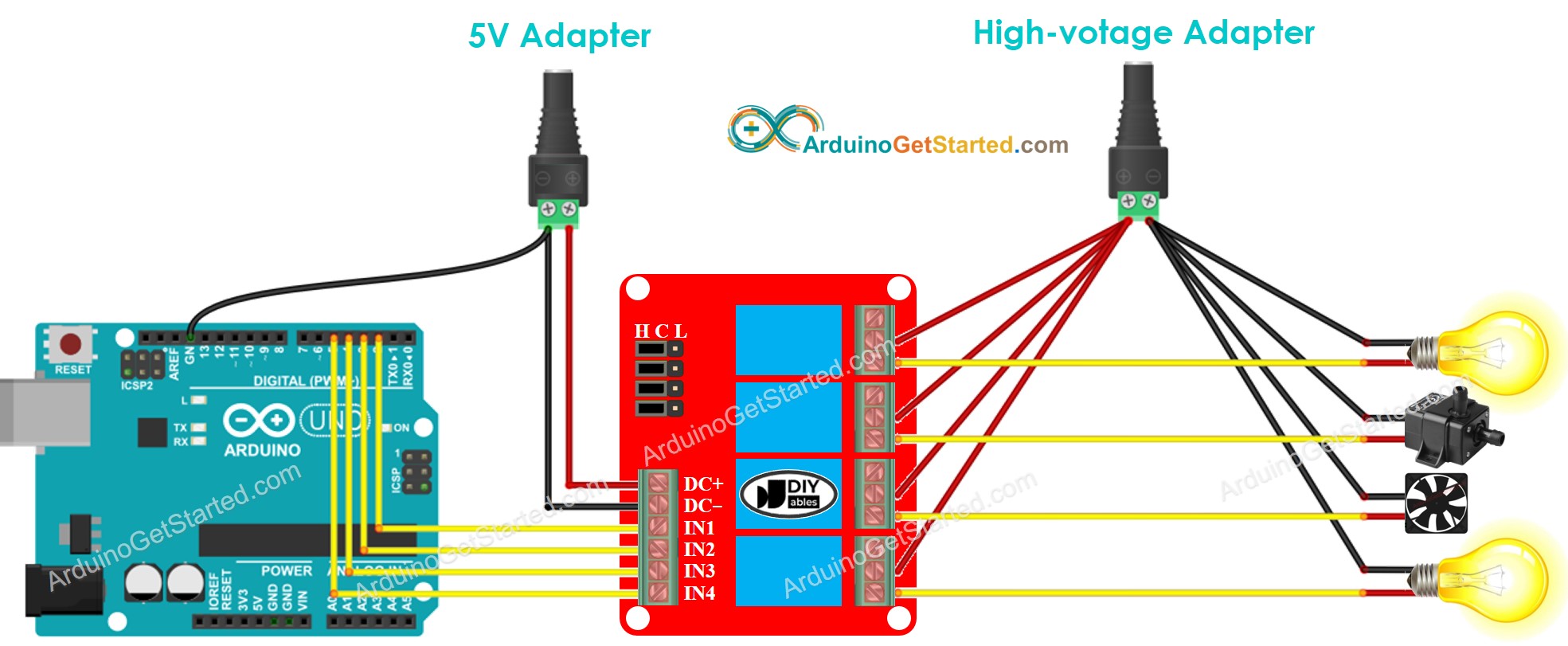
This image is created using Fritzing. Click to enlarge image
- We can reduce the number of power adapters by using a single 5V power source for both Arduino and the 4-channel relay module.
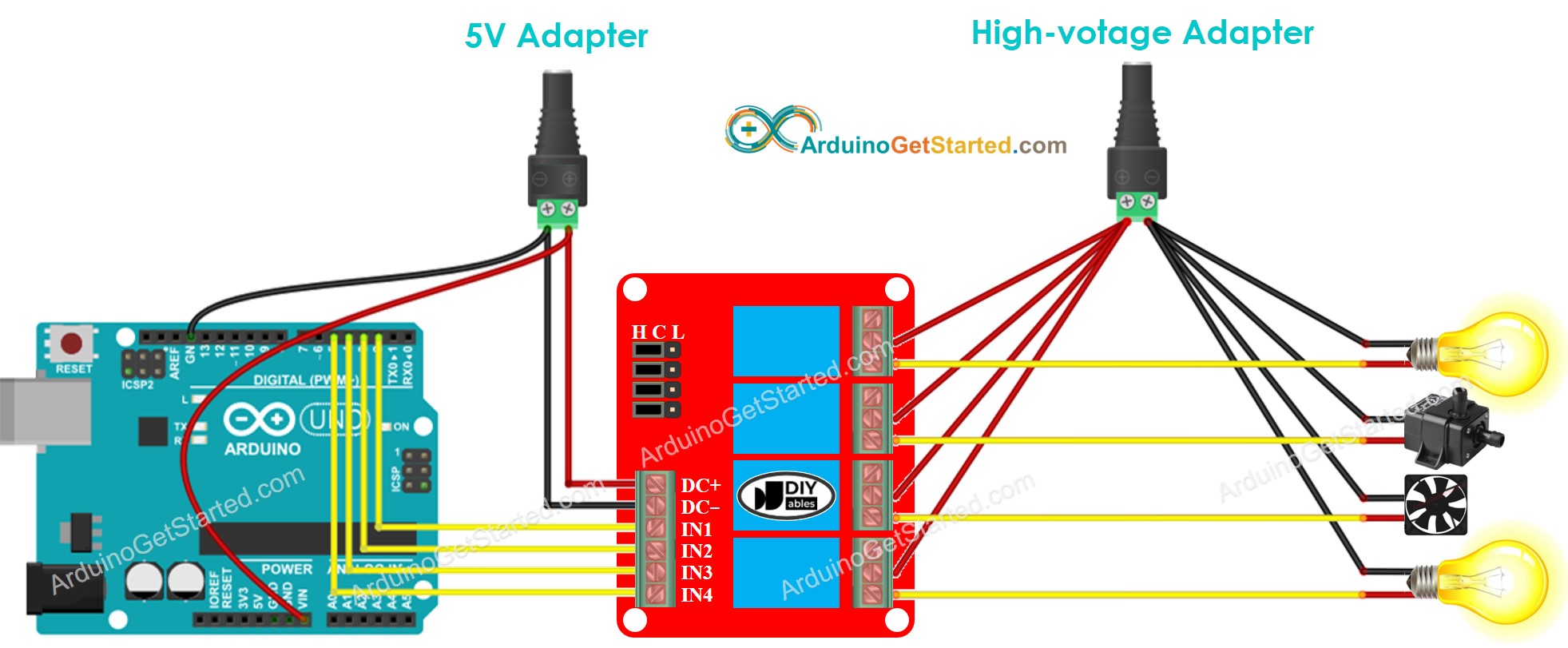
This image is created using Fritzing. Click to enlarge image
※ NOTE THAT:
If 4 devices that are controlled by a 4-channel relay module use the same voltage, we can use a single high-voltage power adapter for all. However, if they use different voltages, we can use different high-voltage power adapters independently.
How To Program For 4-Channel Relay Module
- Initializes the Arduino pin to the digital output mode by using pinMode() function.
- Control the relay's state by using digitalWrite() function.
Arduino Code
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Listen the click sound on relays.
- See the result on Serial Monitor.
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.