Arduino - Touch Sensor - Piezo Buzzer
We are going to learn how to:
- Make sound if the touch sensor is touched.
- Stop making sound if the touch sensor is NOT touched.
- Make melody if the touch sensor is touched.
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables.
About Piezo Buzzer and Touch Sensor
If you do not know about piezo buzzer and touch sensor (pinout, how it works, how to program ...), learn about them in the following tutorials:
Please note that this tutorial use 3-5V buzzer, but you can adapt it for 12v buzzer. you can learn about Arduino - Buzzer tutorial
Wiring Diagram
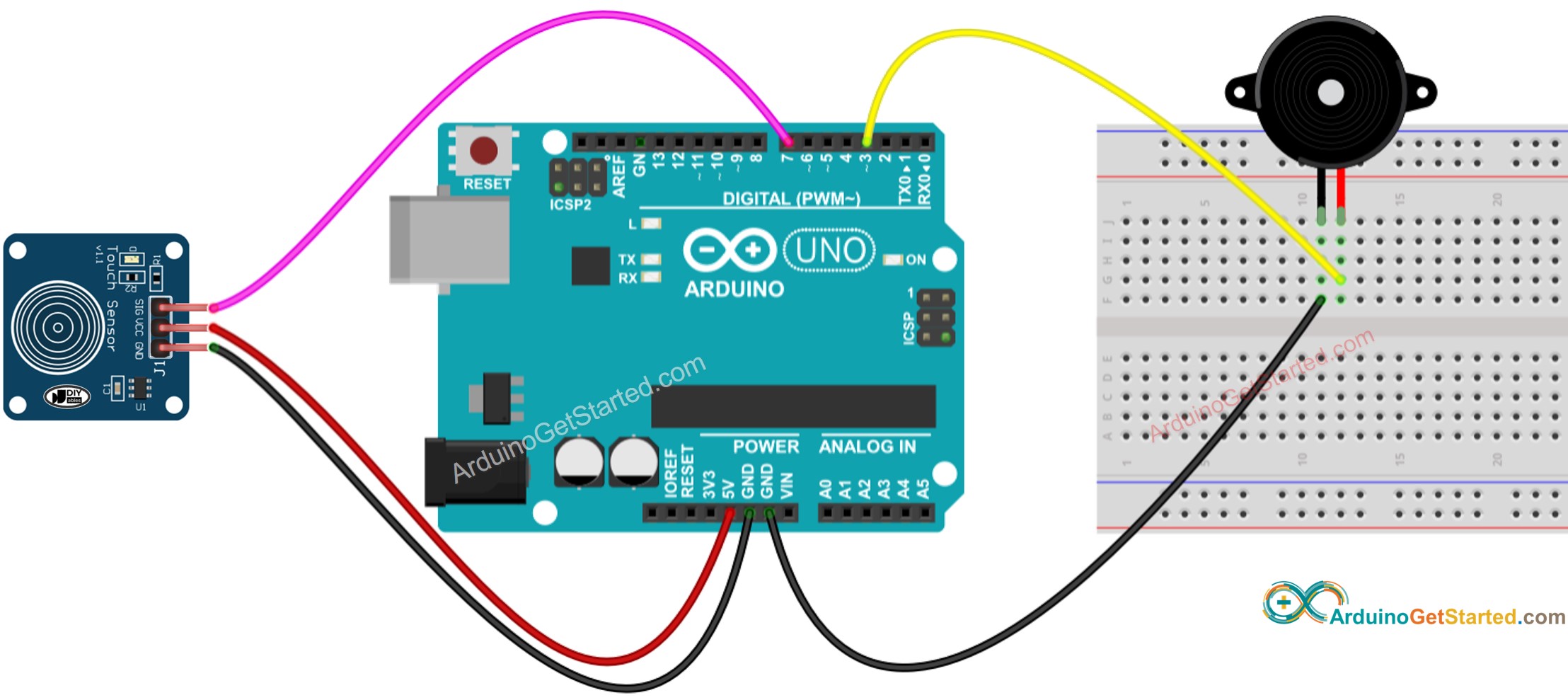
This image is created using Fritzing. Click to enlarge image
Arduino Code - Simple Sound
Quick Steps
- Connect Arduino to PC via USB cable
- Open Arduino IDE, select the right board and port
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Touch and keep touching the touch sensor several seconds
- Listen to piezo buzzer's sound
Code Explanation
Read the line-by-line explanation in comment lines of source code!
Arduino Code - Melody
Quick Steps
- Copy the above code and open with Arduino IDE
- Create the pitches.h file On Arduino IDE by:
- Either click on the button just below the serial monitor icon and choose New Tab, or use Ctrl+Shift+N keys.
- Give file's name pitches.h and click OK button
- Copy the below code and paste it to the created pitches.h file.
- Click Upload button on Arduino IDE to upload code to Arduino
- Touch the touch sensor
- Listen to piezo buzzer's melody
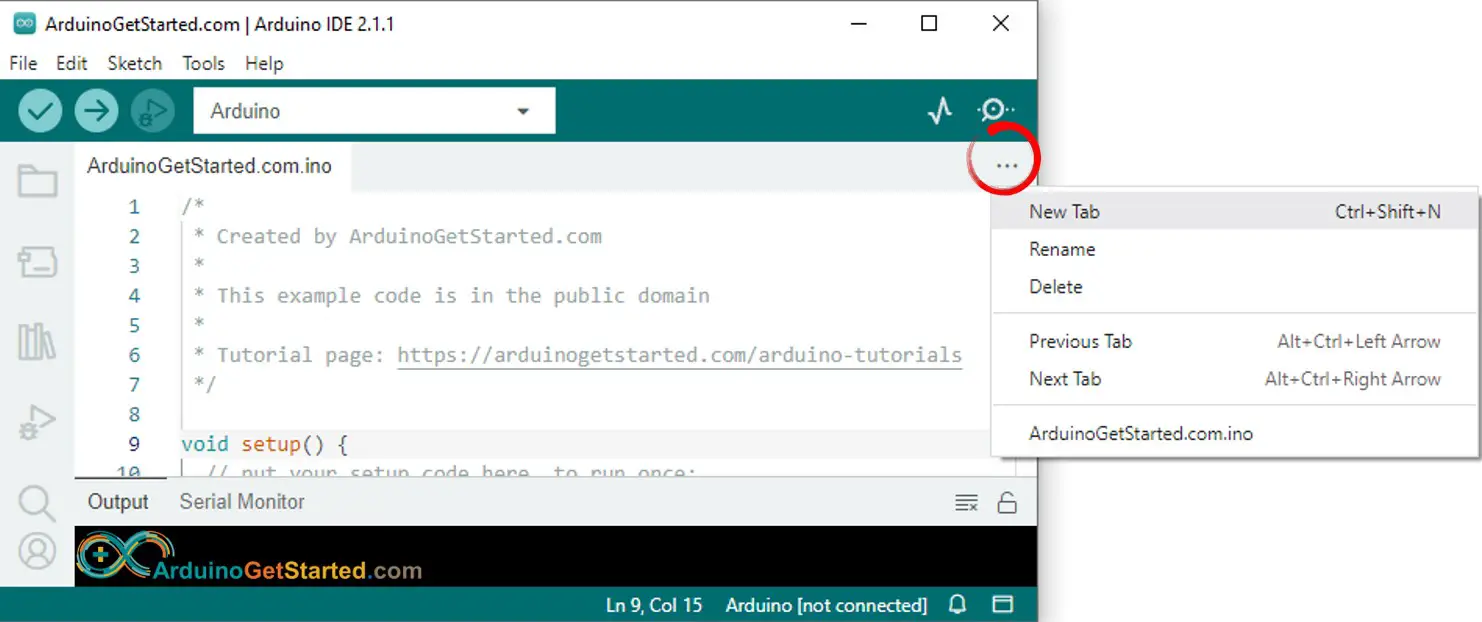
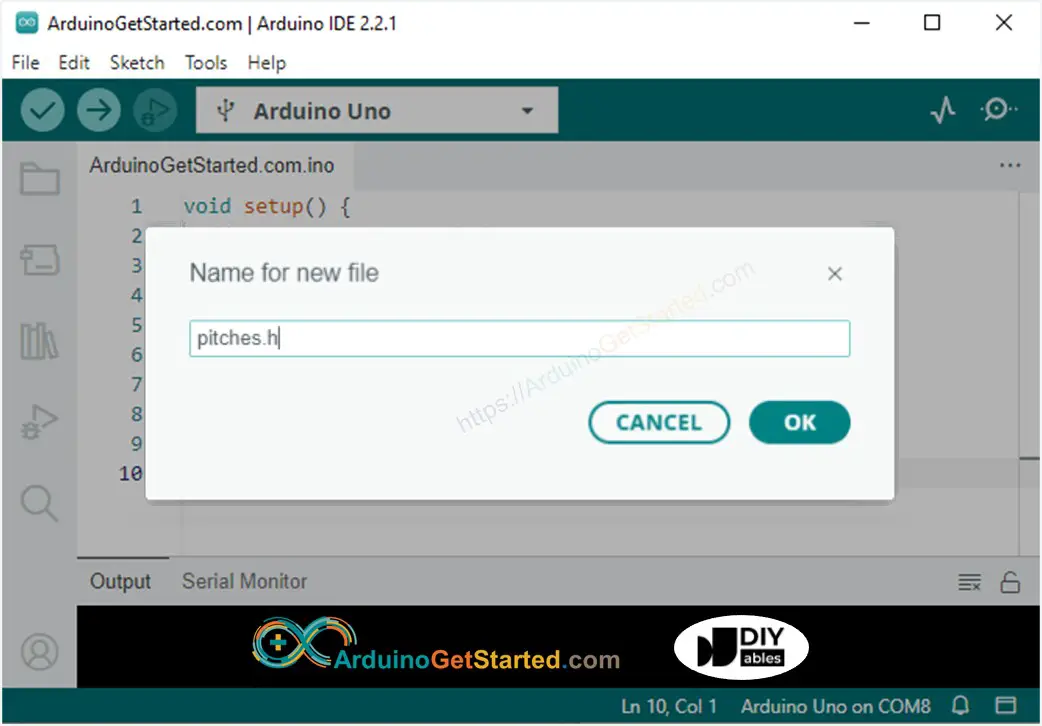
Code Explanation
Read the line-by-line explanation in comment lines of source code!
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.