Arduino - Bluetooth LED Matrix
In this tutorial, we will learn how to control an LED matrix display using a smartphone via Bluetooth or BLE by using Arduino. We will have the option to choose between two different modules, HC-05 for Classic Bluetooth (Bluetooth 2.0) and HM-10 for Bluetooth Low Engery (BLE, Bluetooth 4.0). The tutorial will provide step-by-step instructions for both modules. To send messages from the smartphone to the LED matrix display, we will use the Bluetooth Serial Monitor App, which can be found at Bluetooth Serial Monitor App. Once the message is received by Arduino, it will be displayed on the LED matrix display.
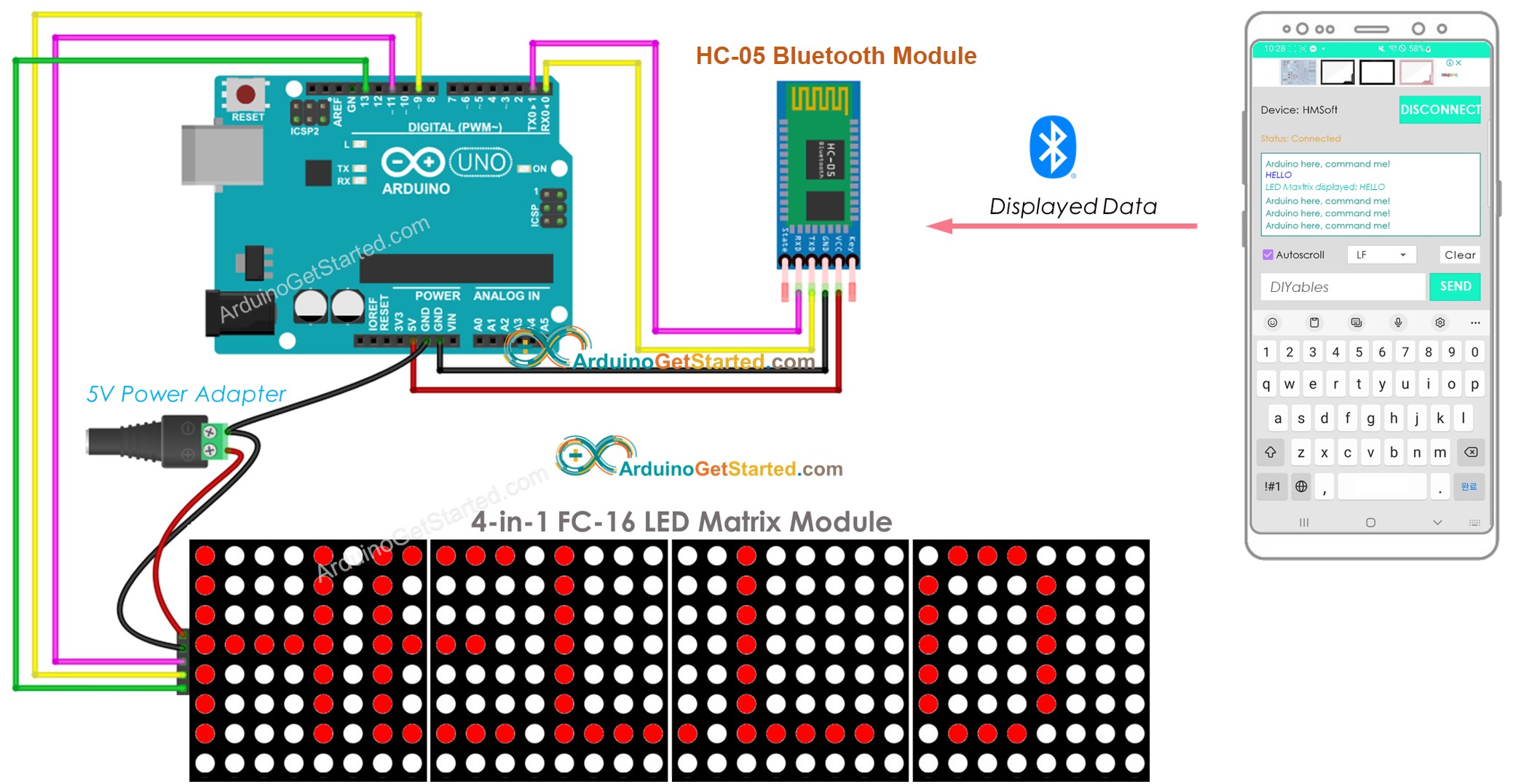
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
About LED matrix display and Bluetooth Module
Before starting this tutorial, it is recommended that you have a basic understanding of LED matrix displays and Bluetooth modules, including their pinouts, how they work, and how to program them. If you are not familiar with these topics, please review the following tutorials for more information:
- Arduino - LED Matrix Display tutorial
- Arduino - Bluetooth tutorial
- Arduino - BLE tutorial
Wiring Diagram
- To control the LED matrix display using Classic Bluetooth, you need to use the HC-05 Bluetooth module and connect it to Arduino according to the below wiring diagram:
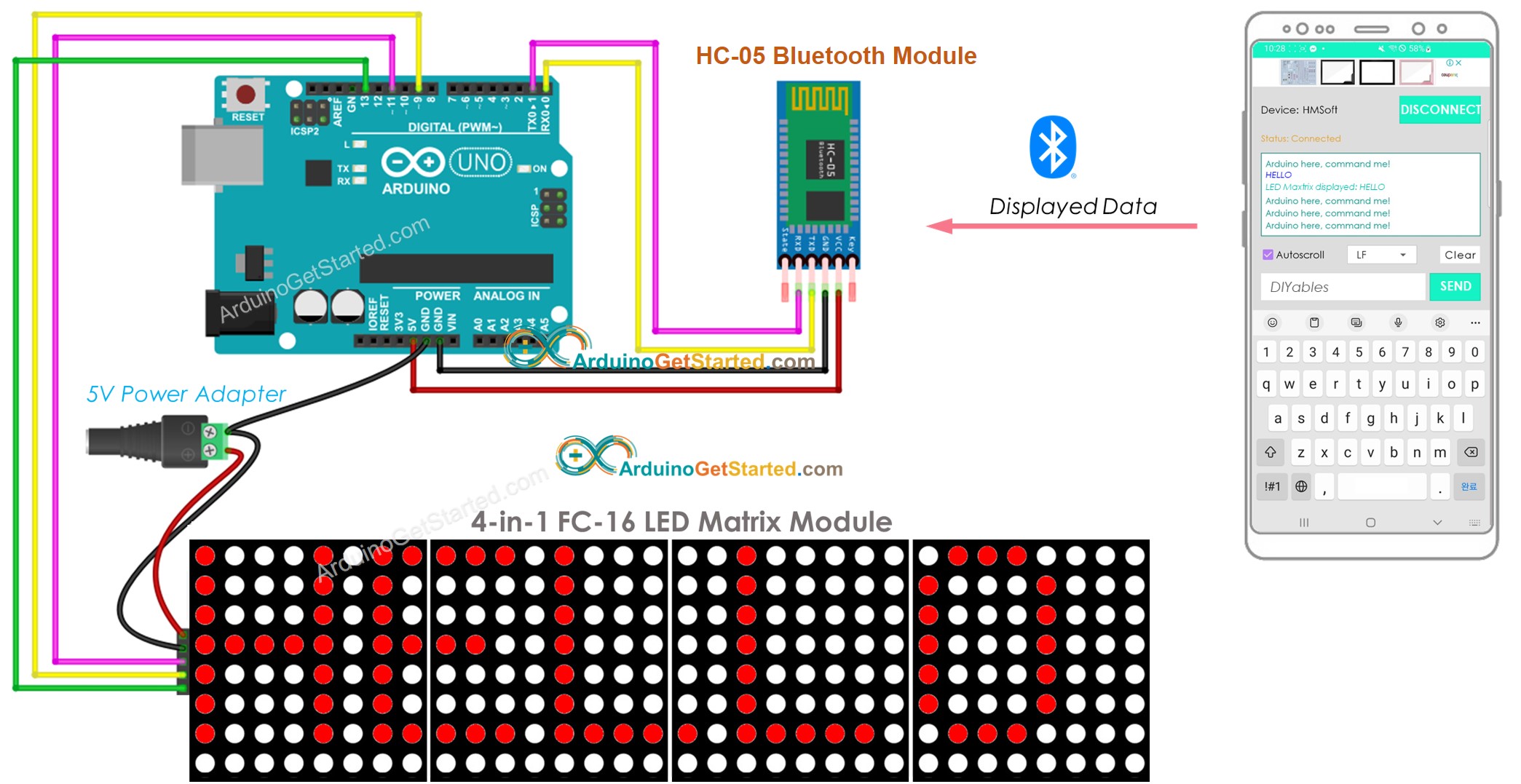
This image is created using Fritzing. Click to enlarge image
- To control the LED matrix display using BLE, you need to use the HM-10 Bluetooth module and connect it to Arduino according to the below wiring diagram:
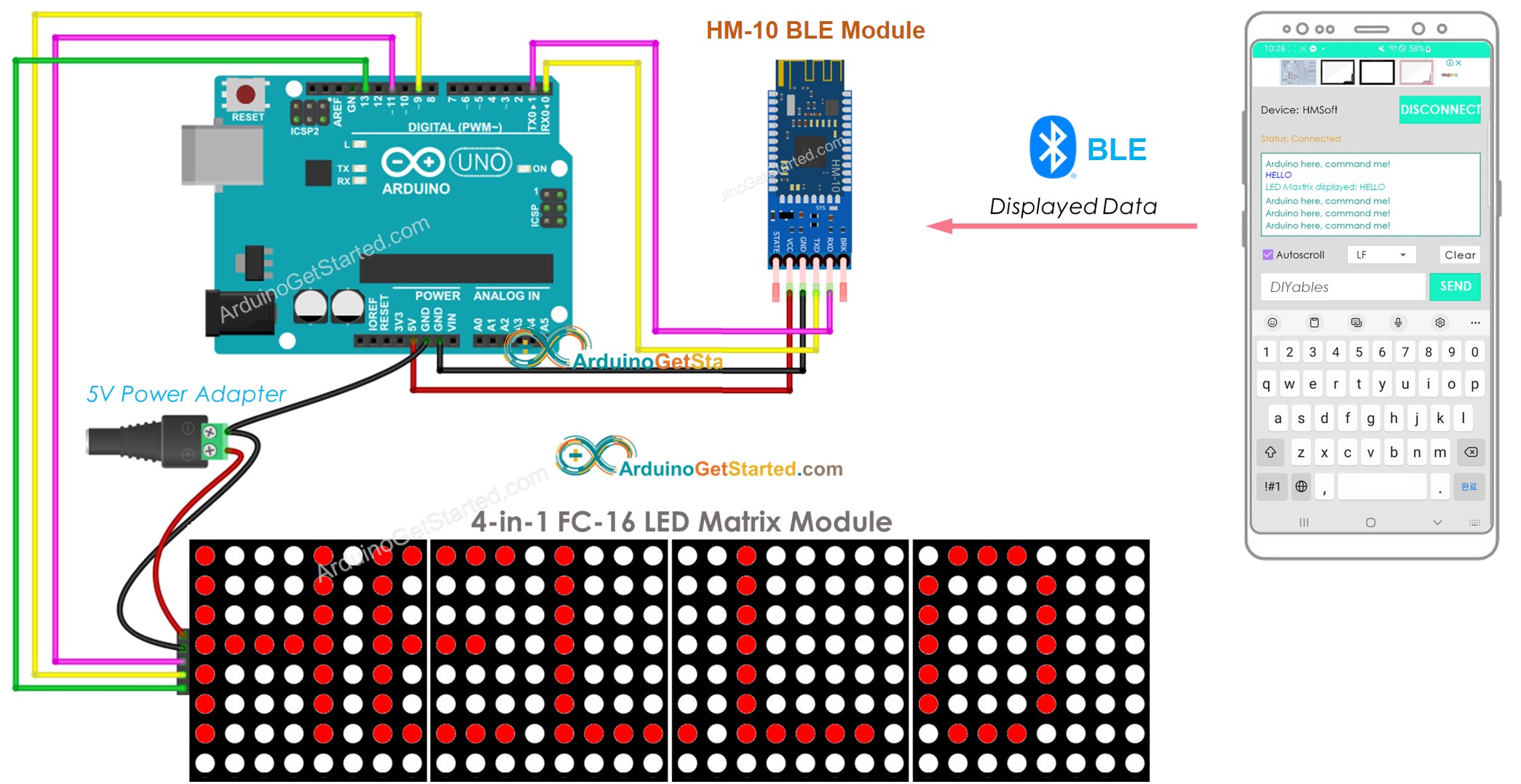
This image is created using Fritzing. Click to enlarge image
Arduino Code - controls LED matrix display via Bluetooth/BLE
The code below will work for both the HC-10 Bluetooth module and the HM-10 BLE module
Quick Steps
To control an LED matrix display using Bluetooth or BLE:
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search “MD_Parola”, then find the MD_Parola library
- Click Install button.
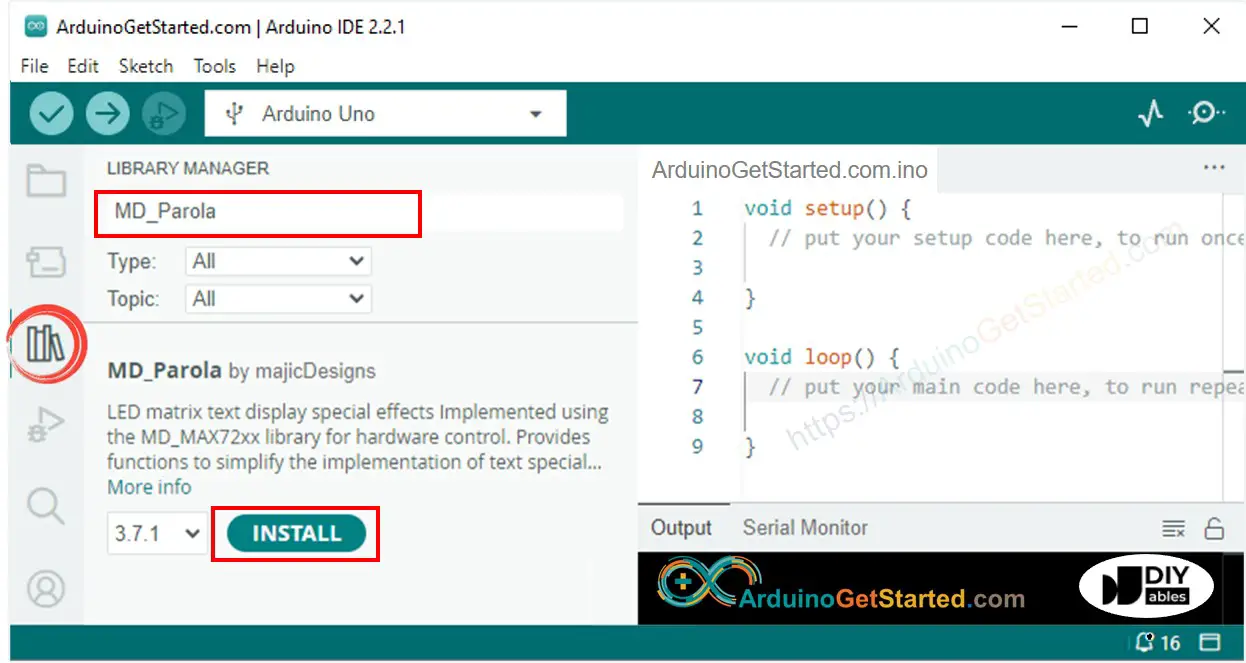
- You will be asked to install the MD_MAX72XX library for dependency. Click Install All button.
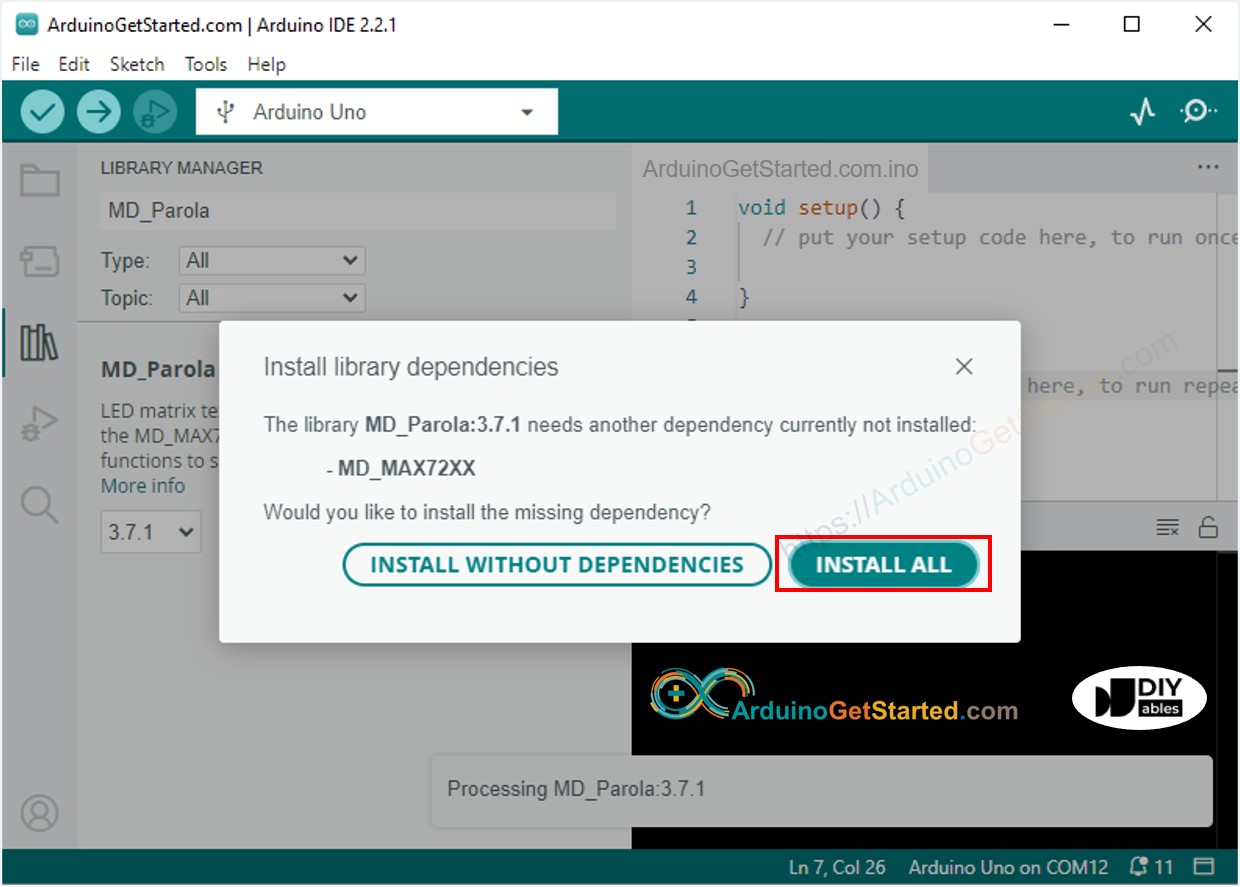
- Install the Bluetooth Serial Monitor App on your smartphone.
- Copy the provided code and open it with the Arduino IDE. Upload the code to your Arduino. If you are unable to upload the code, try disconnecting the TX and RX pins from the Bluetooth module, uploading the code, and then reconnecting the RX/TX pins again.
- Open the Bluetooth Serial Monitor App on your smartphone.
- Select the Classic Bluetooth or BLE option, depending on the module you are using.
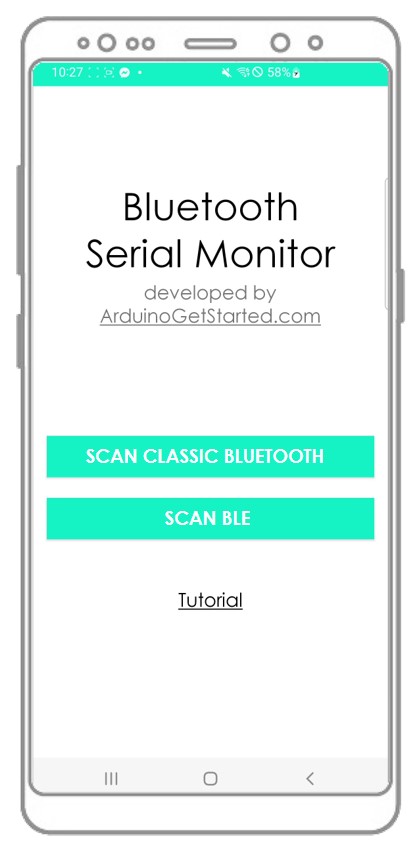
- Pair the Bluetooth App with the HC-05 Bluetooth module or the HM-10 BLE module.
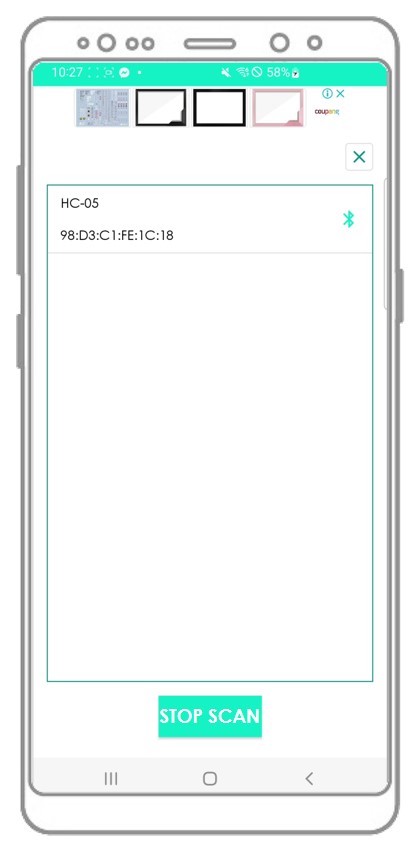
- Type a message, for example “HELLO” and click the Send button to send it to the Arduino.
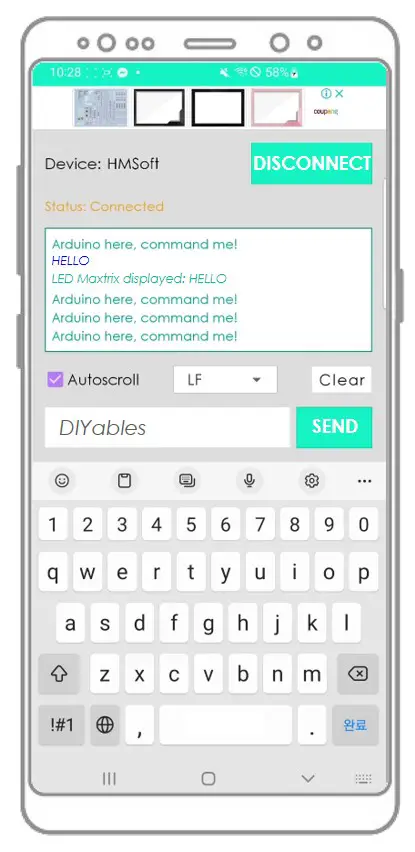
- Observe the message displayed on the LED matrix display and on the Bluetooth App.
- Verify the result on the Android App.
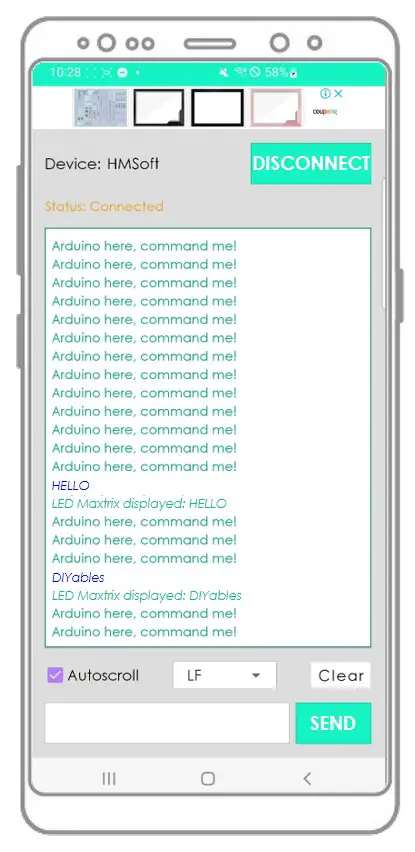
If you found the Bluetooth Serial Monitor app to be helpful, please consider leaving a 5-star rating on the Play Store. Your feedback is greatly appreciated! Thank you!
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.