Arduino - Door Open - Send Email Notification
We are going to learn how to use Arduino to send a notification to you via email when someone opens your door. We will use Arduino, door sensor, and Ethernet Shield. You can also use any other Ethernet or WiFi shield by making a small modification in the Arduino code.
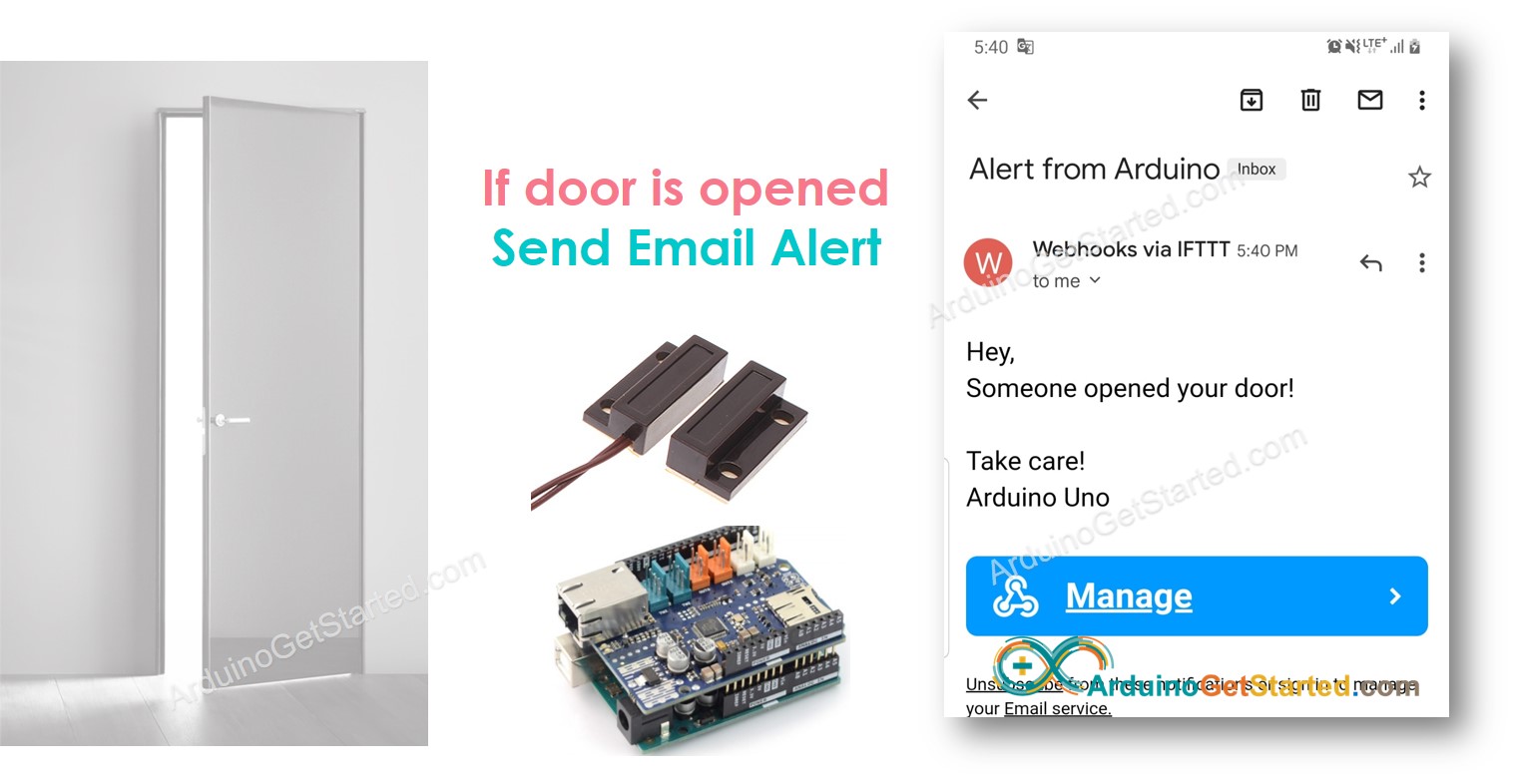
The tutorial provides the Arduino code for two cases:
- Arduino Uno R4 WiFi
- Arduino Uno/Mega with Ethernet Shield
Hardware Required
1 | × | Arduino UNO R4 WiFi | |
1 | × | USB Cable Type-A to Type-C (for USB-A PC) | |
1 | × | USB Cable Type-C to Type-C (for USB-C PC) |
Alternatively if using Ethernet:
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About Door Sensor and Sending Email
If you do not know about the door sensor (pinout, how it works, how to program ...) and how to send email from Arduino, learn about them in the following tutorials:
- Arduino - Door Sensor tutorial
- Arduino - Send Email tutorial
Wiring Diagram
- If using the Ethernet Shield, stack Ethernet Shield on Arduino Uno or Mega
- Connect the door sensor to Arduino as below writing diagram
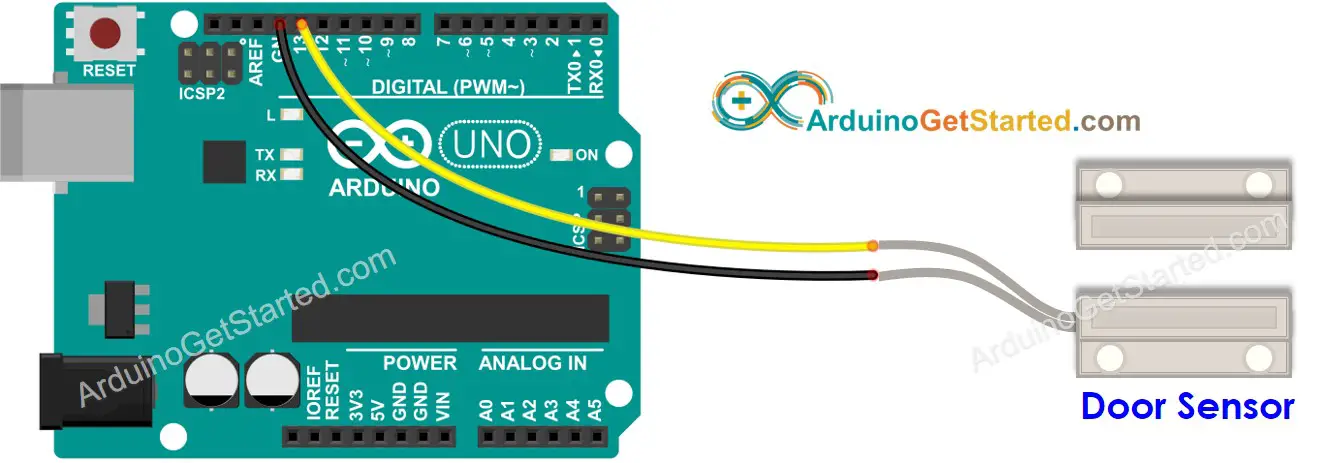
This image is created using Fritzing. Click to enlarge image
What we need to do
We need to do to main tasks:
- Create an Applet on the IFTTT website
- Write Arduino code that makes an HTTP request to the Applet when the door is opened.
How it works
When the door is opened:
- Arduino makes an HTTP request to IFTTT Applet we created,
- The IFTTT Applet will send an email to the email address.
The email address, email subject, and email content are specified when we create the IFTTT Applet.
How To Create an IFTTT Applet
- Create an IFTTT account and Login to IFTTT.
- Go to IFTTT Home page and click the Create button
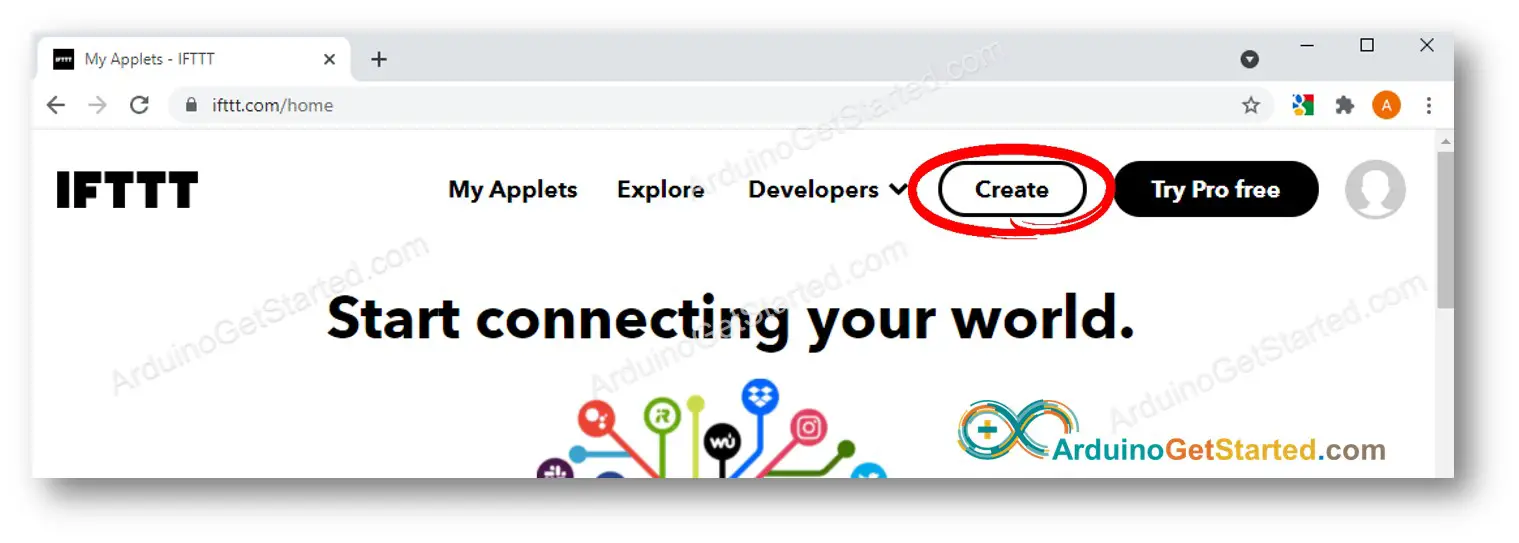
- Click the Add button
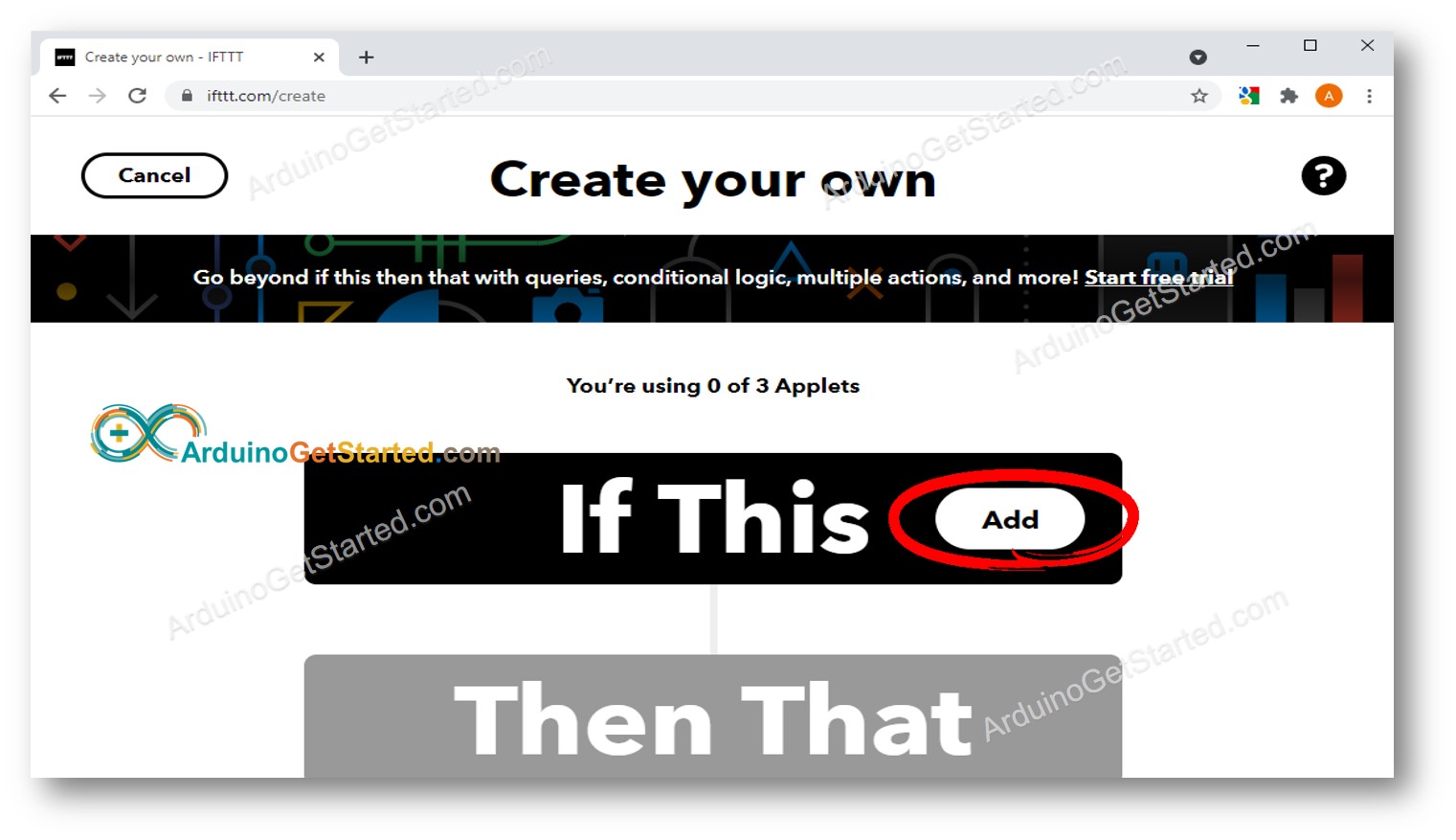
- Search for Webhooks, and then click the Webhooks icon
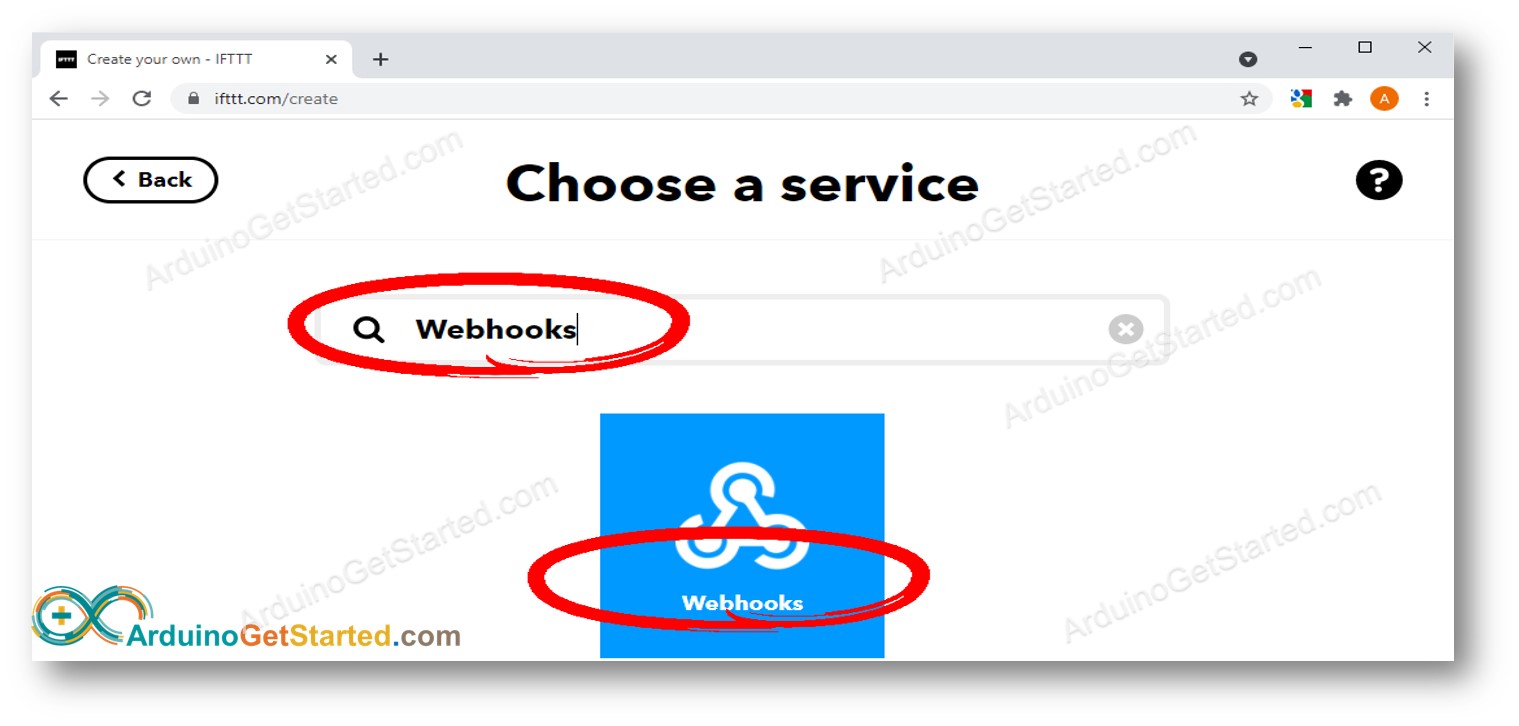
- Click the Receive a web request icon
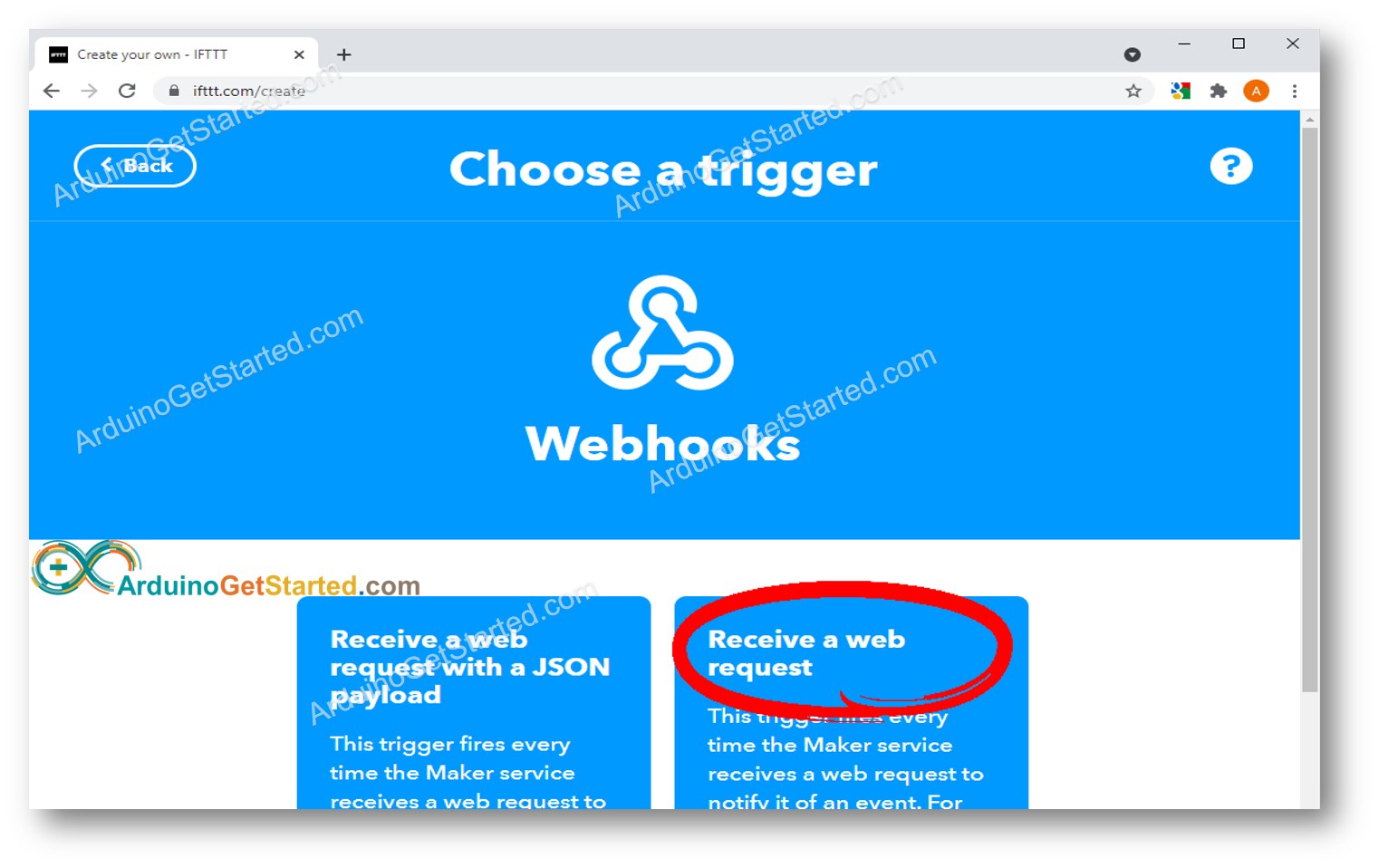
- Click the Connect button
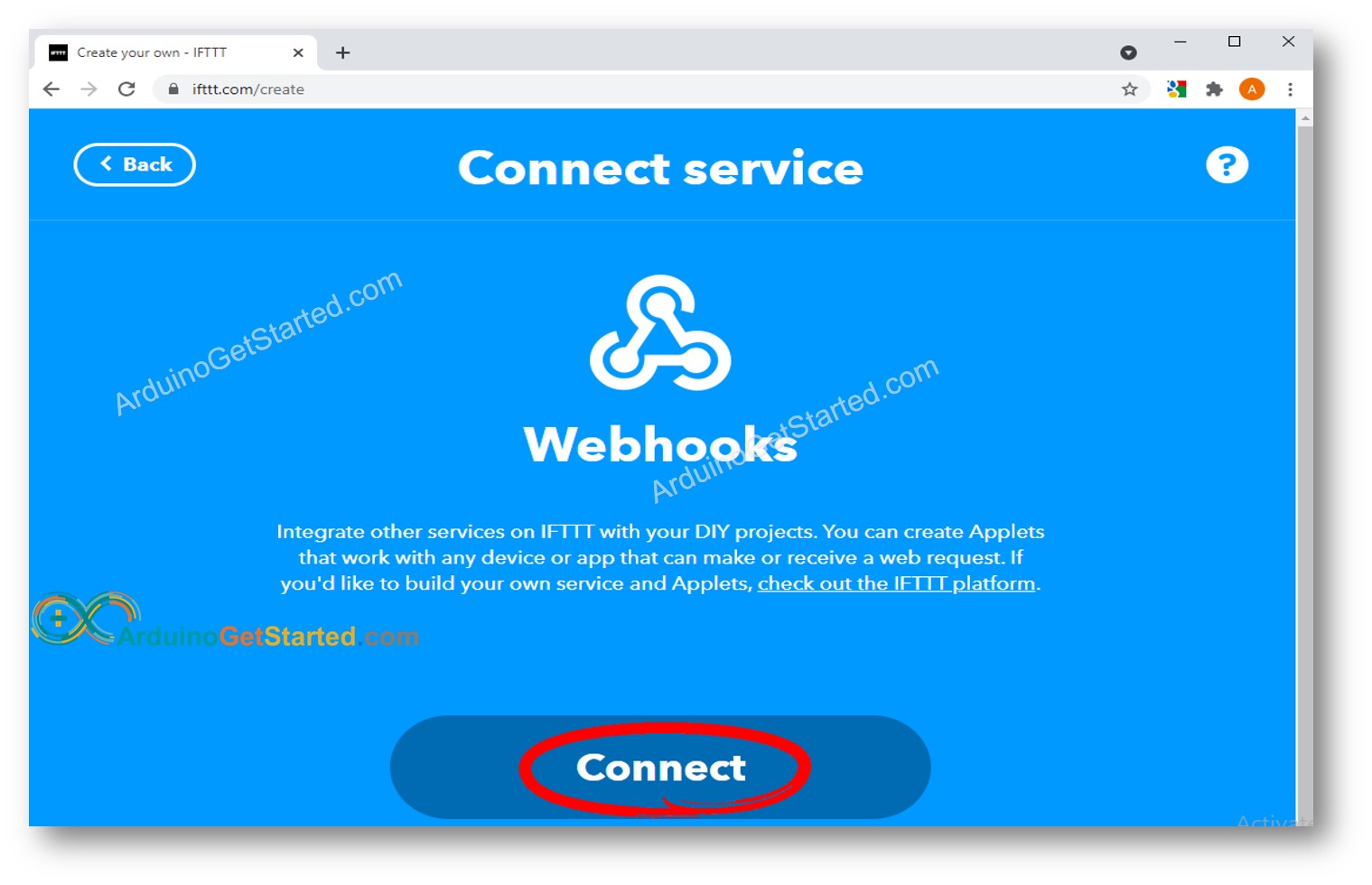
- Type an event name. You can give any name you want and memorize it to use later. The event name is a part of URL that Arduino will make request to. In this tutorial, we use a name send-email. And then click Create trigger button
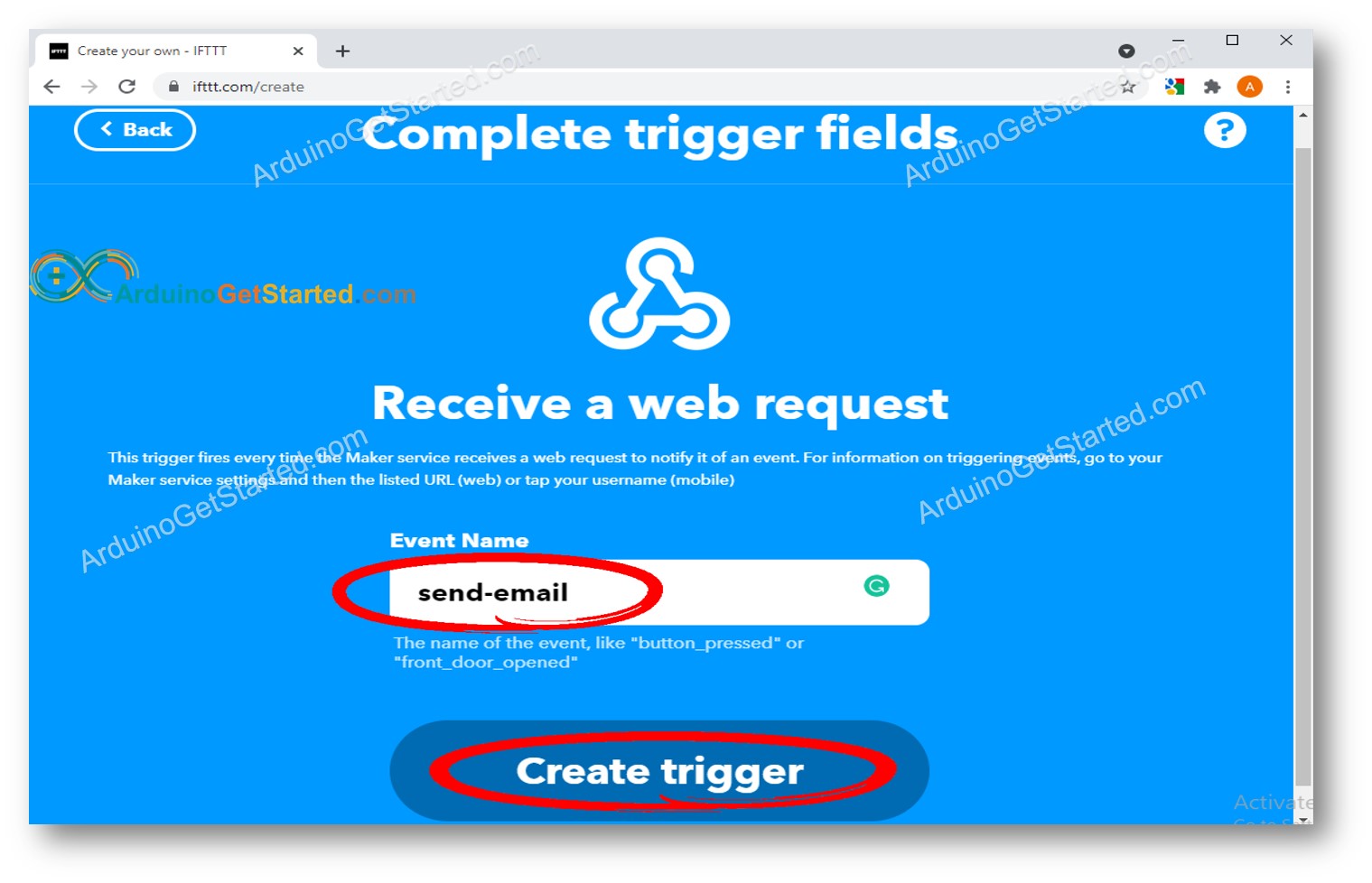
- Click the Add button to add the action
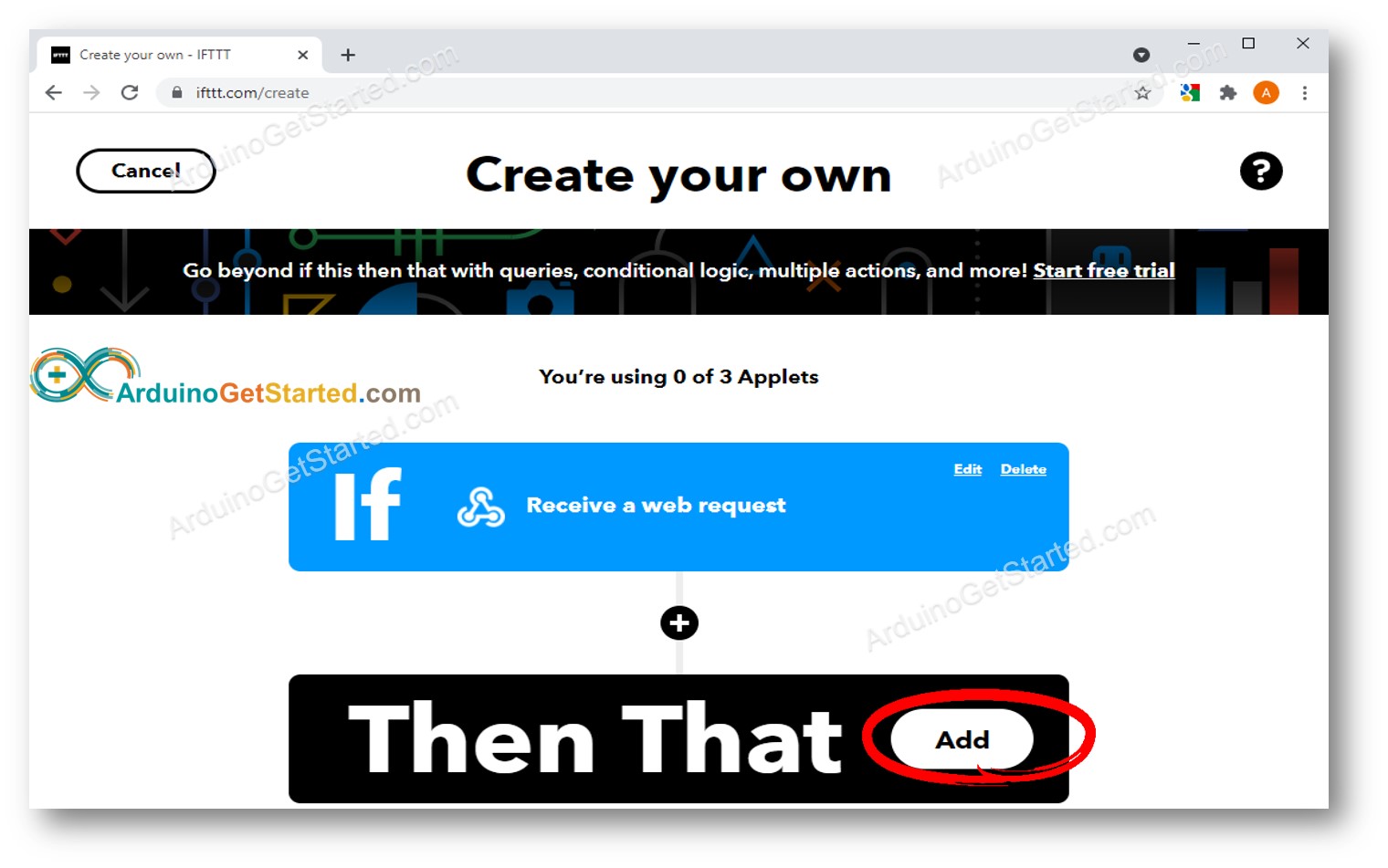
- Search for email, and then click the Email icon
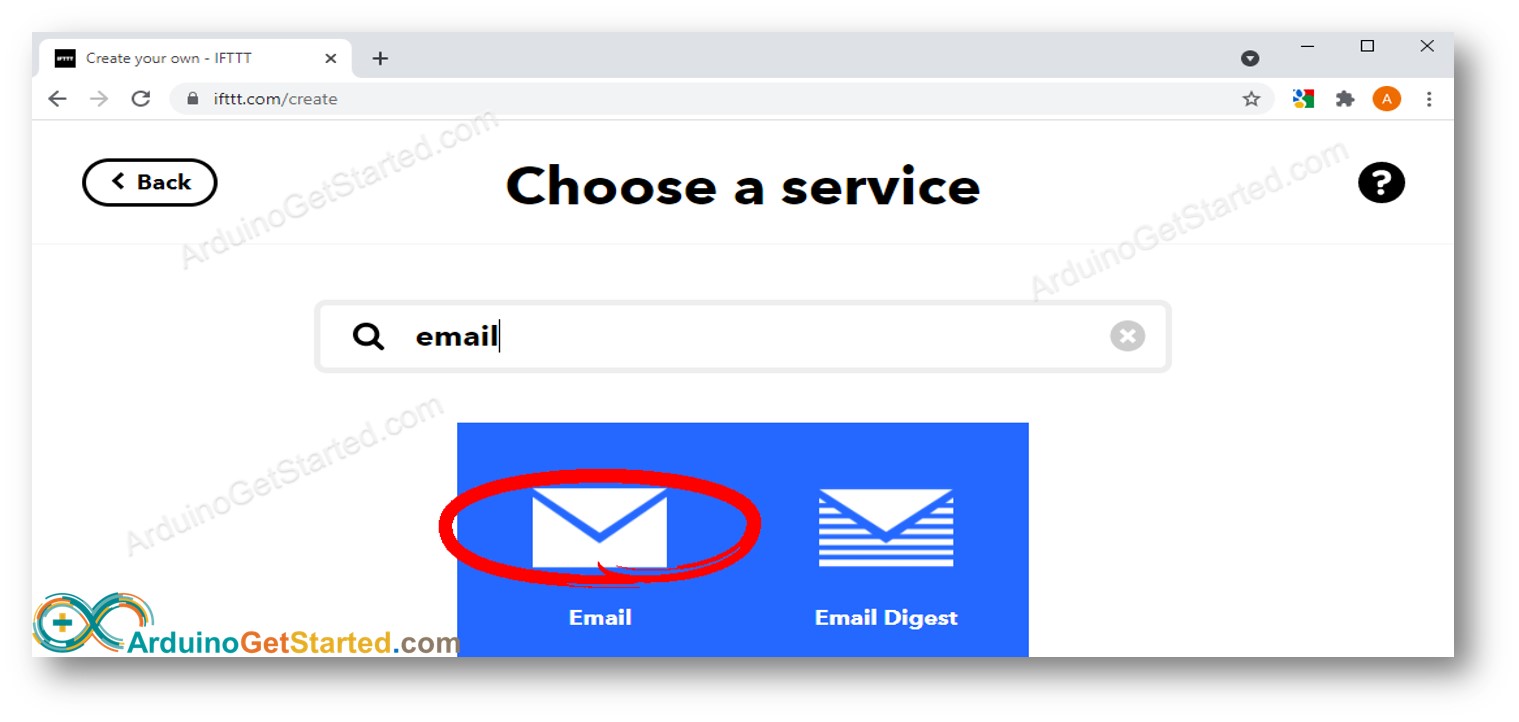
- Click the Send me an email icon
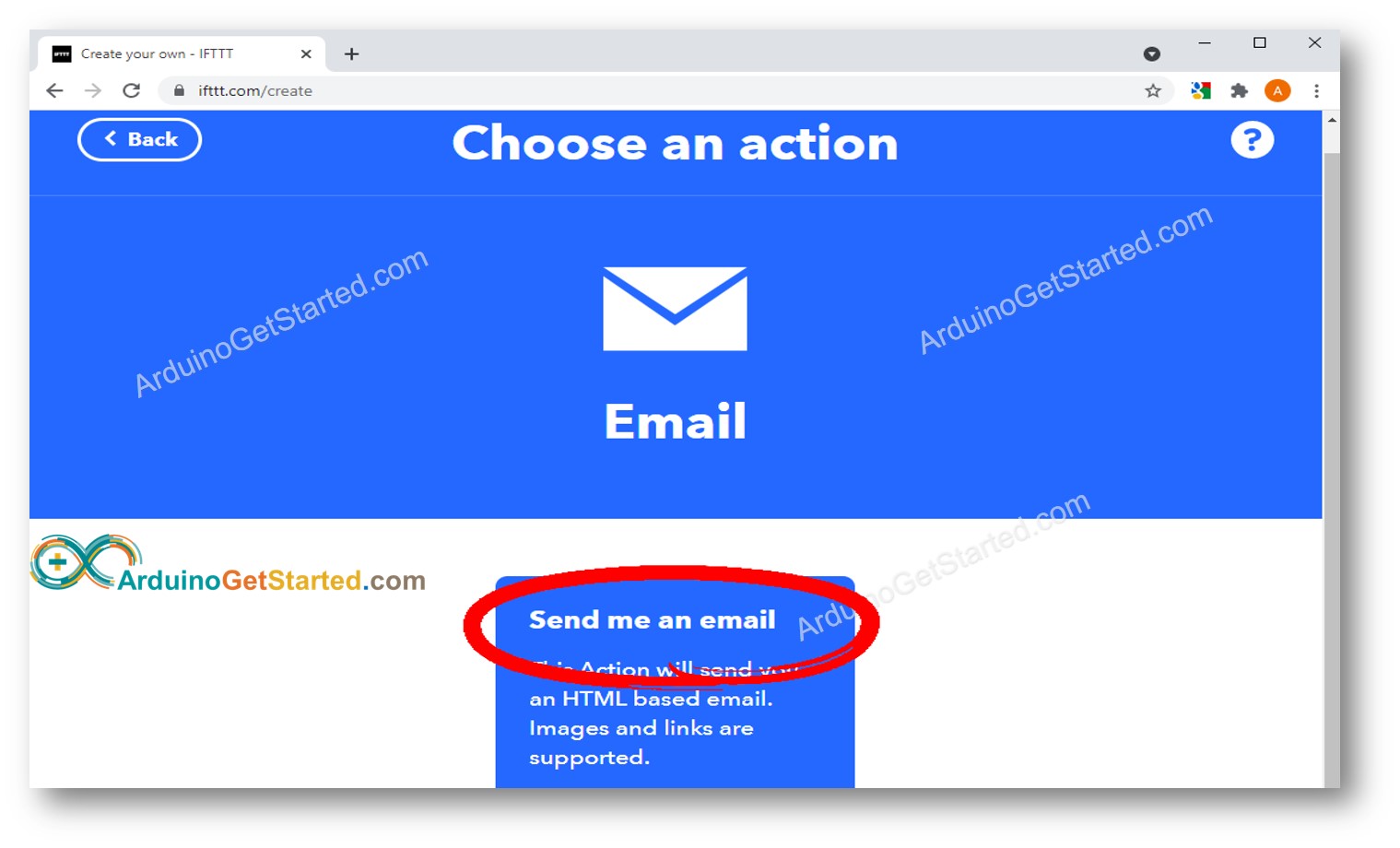
- Click the Connnect button
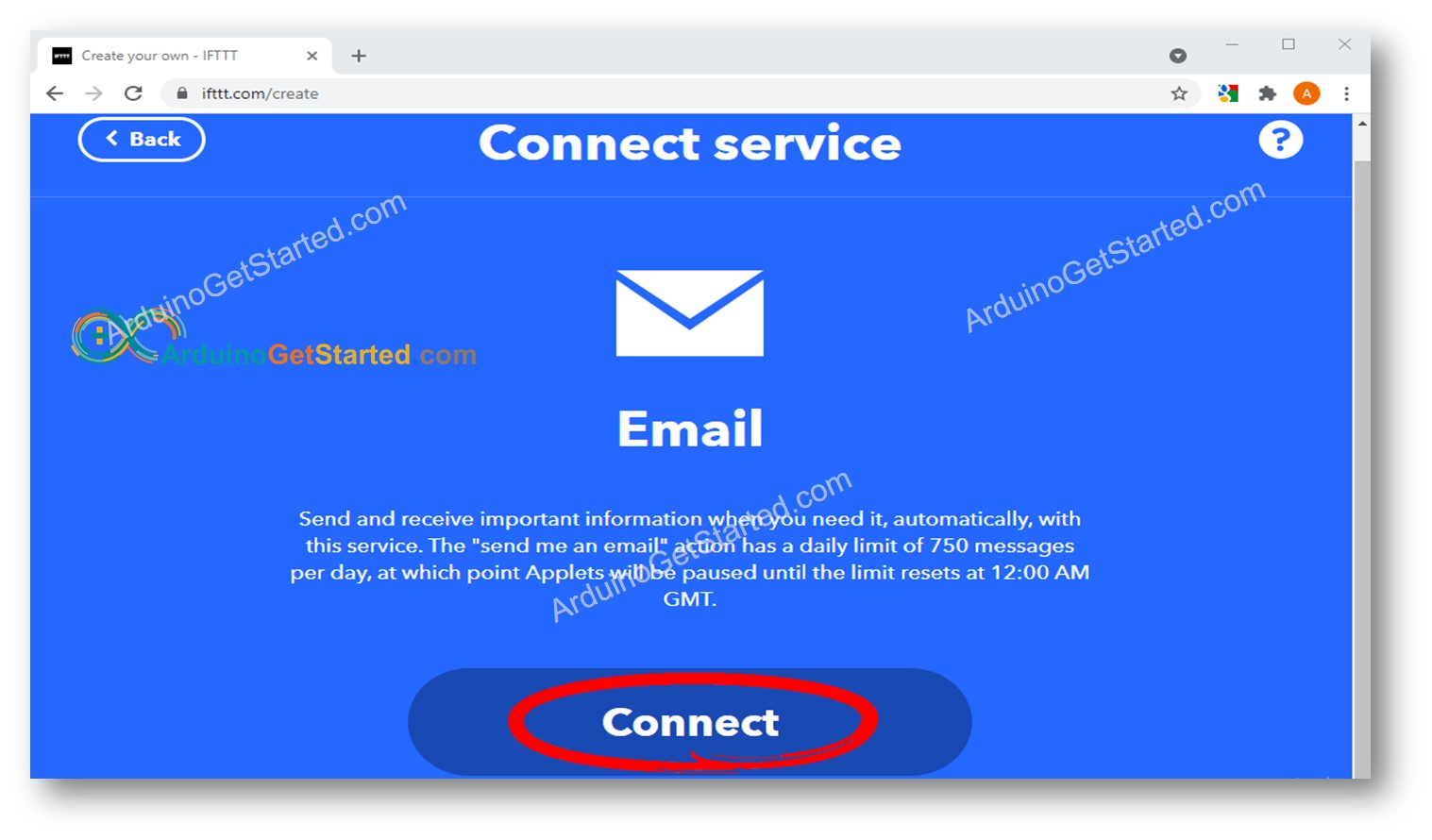
- Input your email address and then click the Semd PIN button
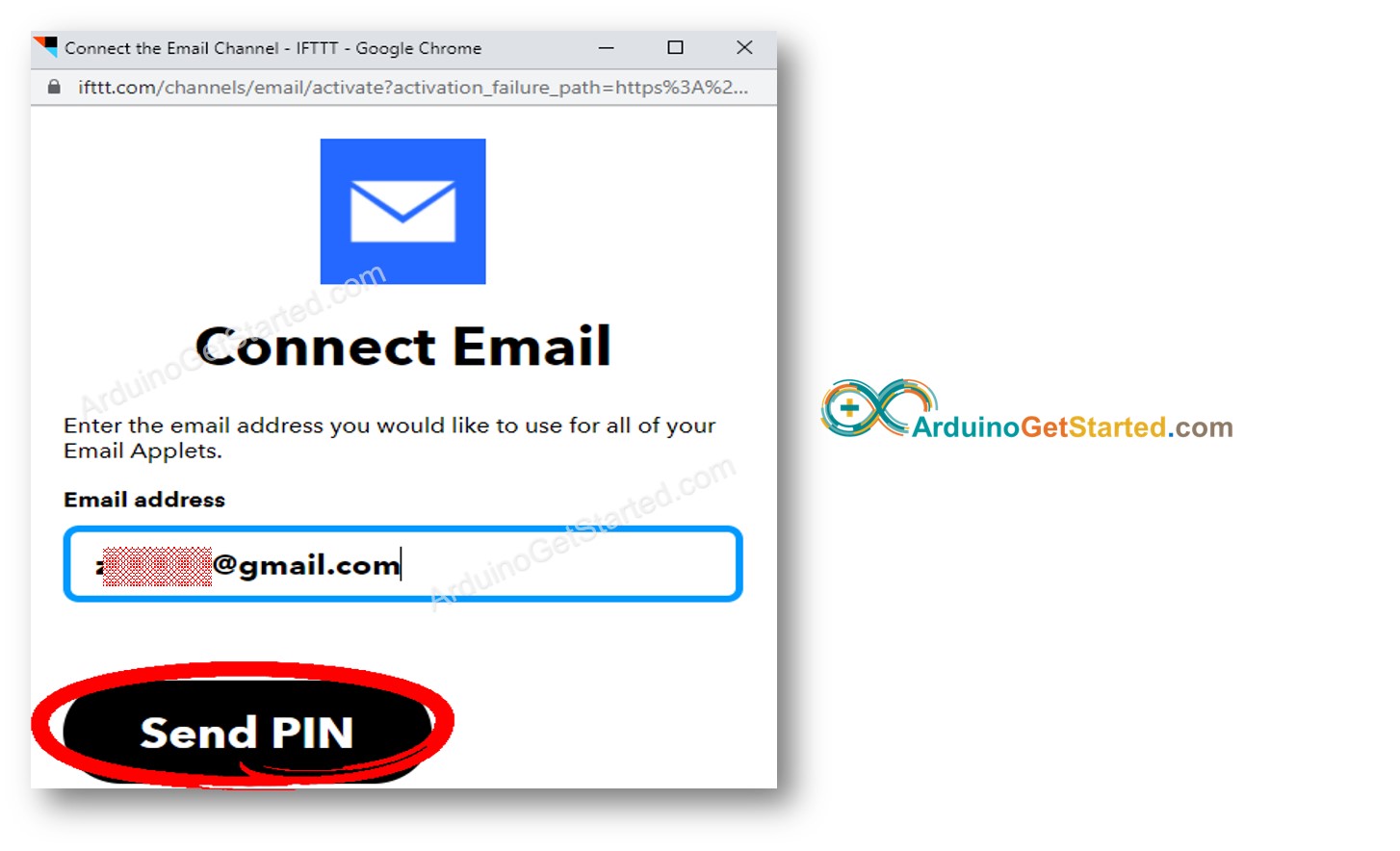
- You will receive an email, get the PIN from email and input to Web UI to do verification
- After that the email subject and content editor appears
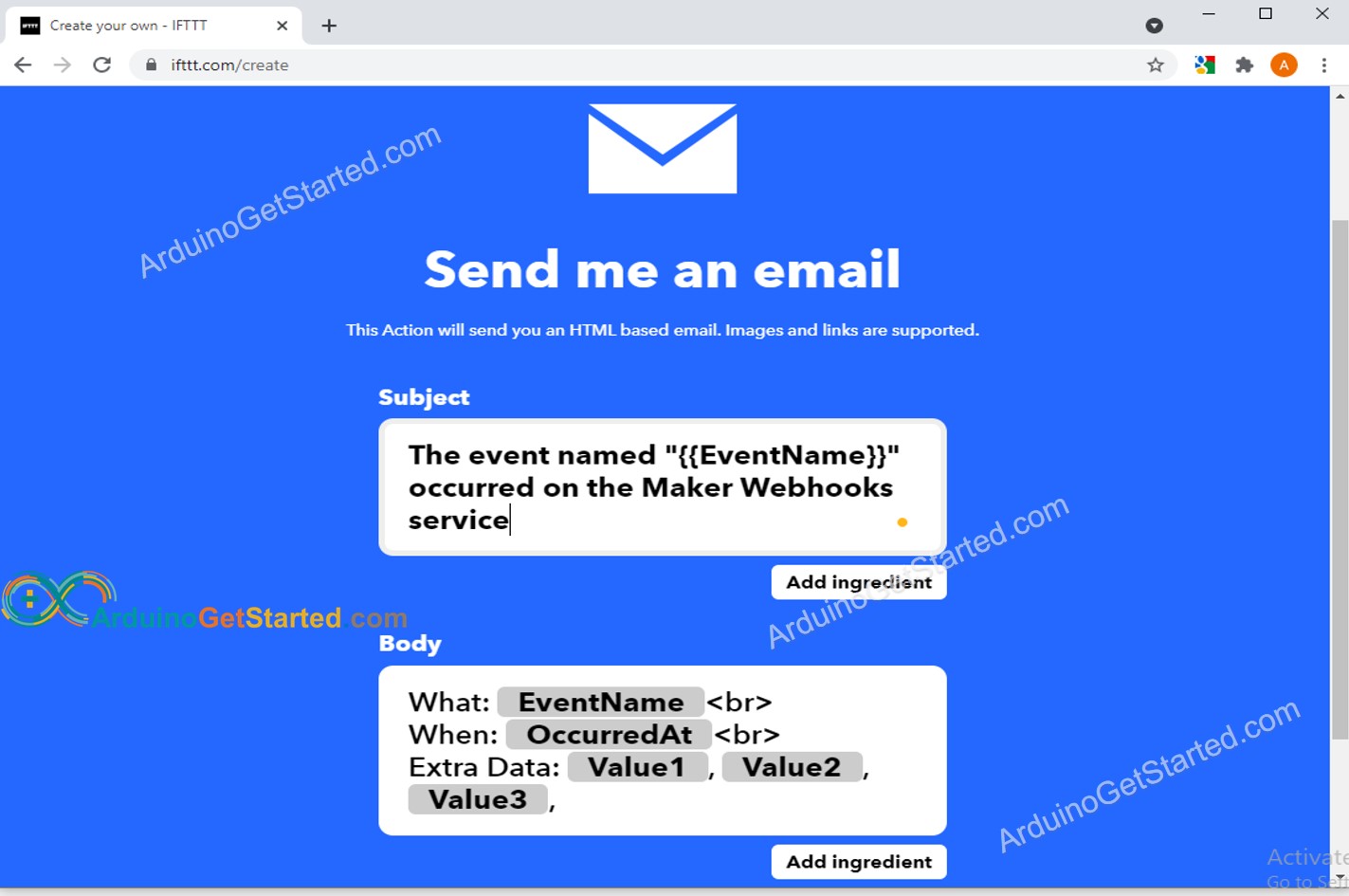
- You can give any subject and content for the email. The below are example
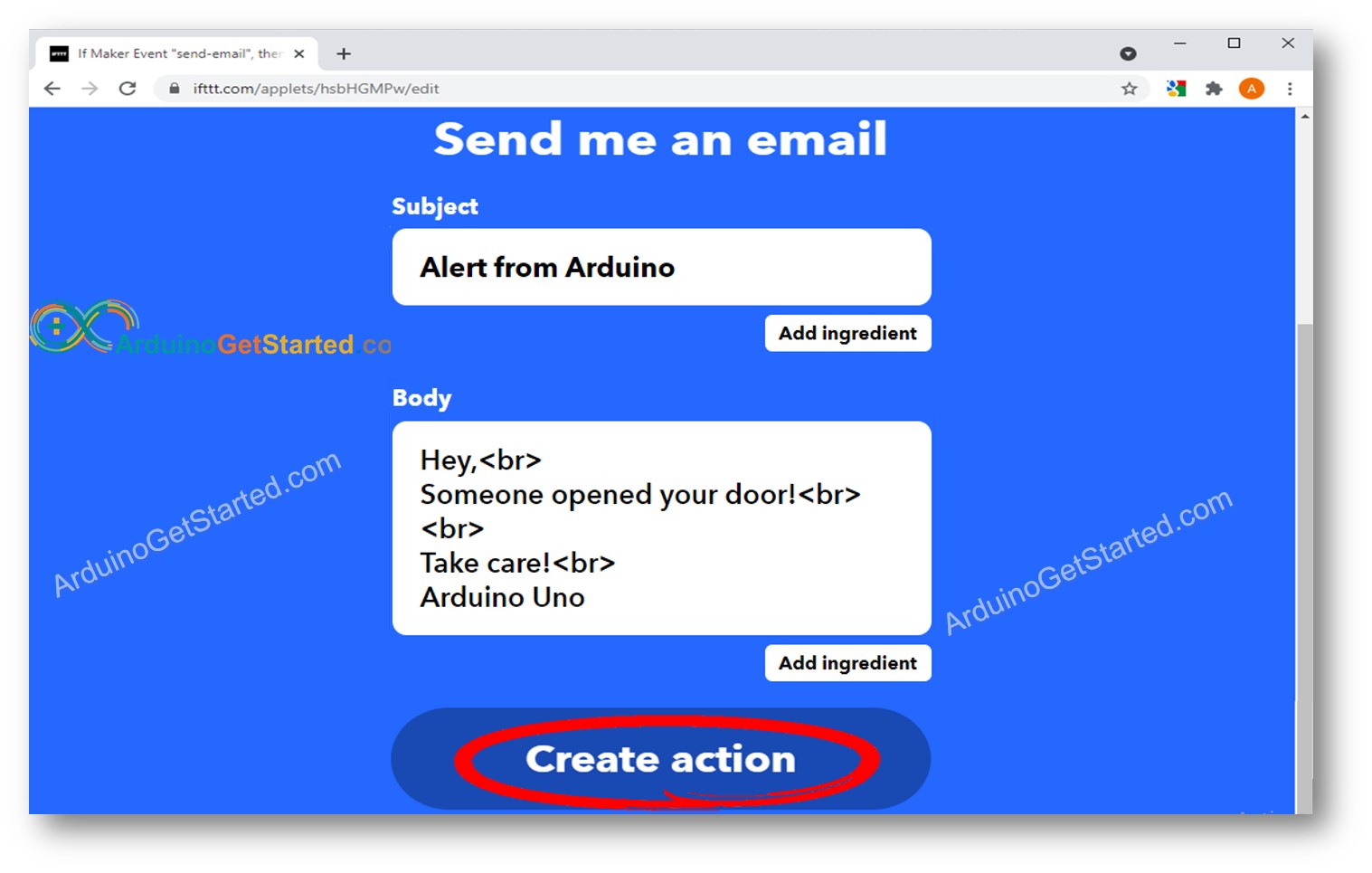
- Subject:
- Content:
Someone opened your door!
Take care!
Arduino Uno
- Click the Create Action button
- Click the Continue button
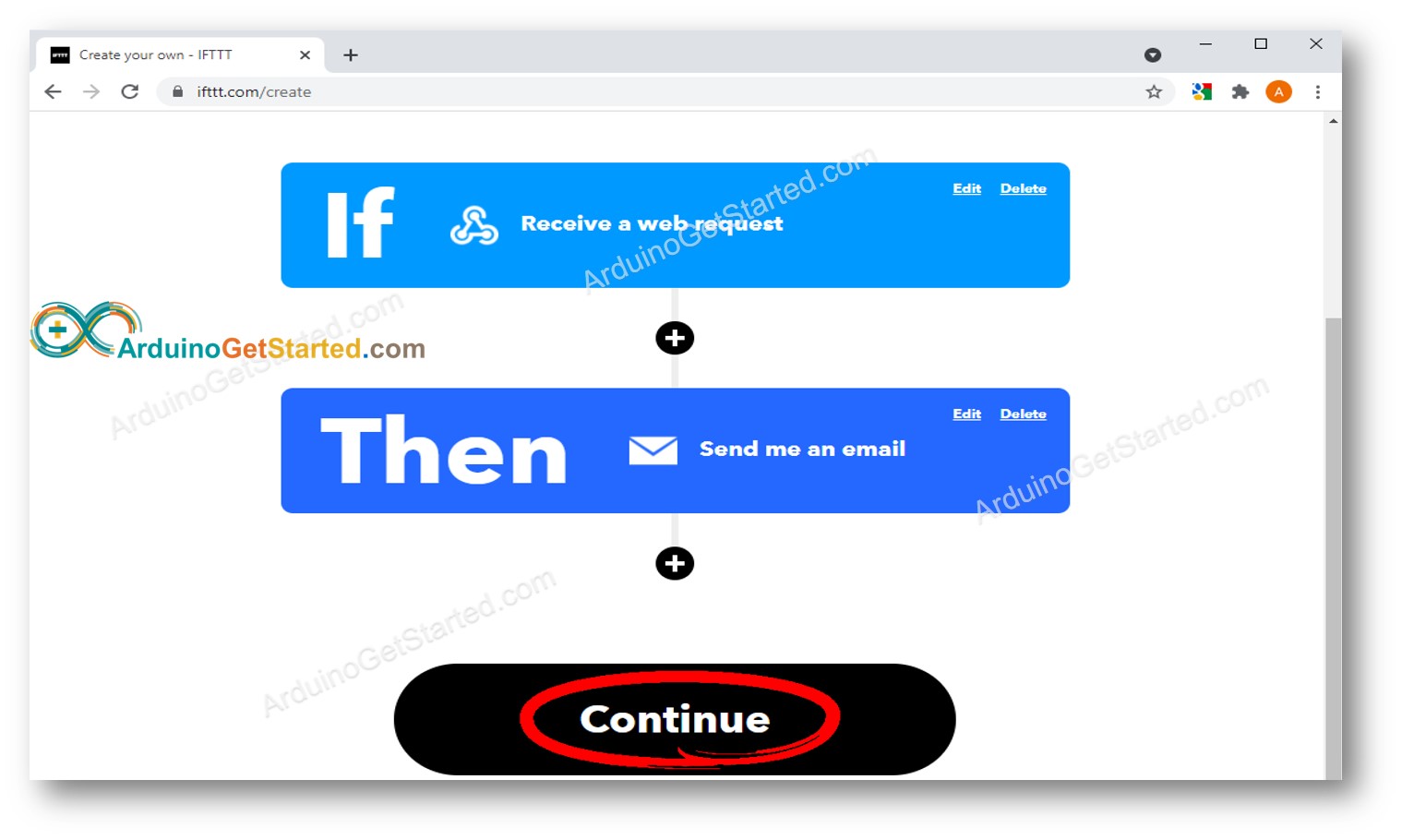
- Click the Finish button
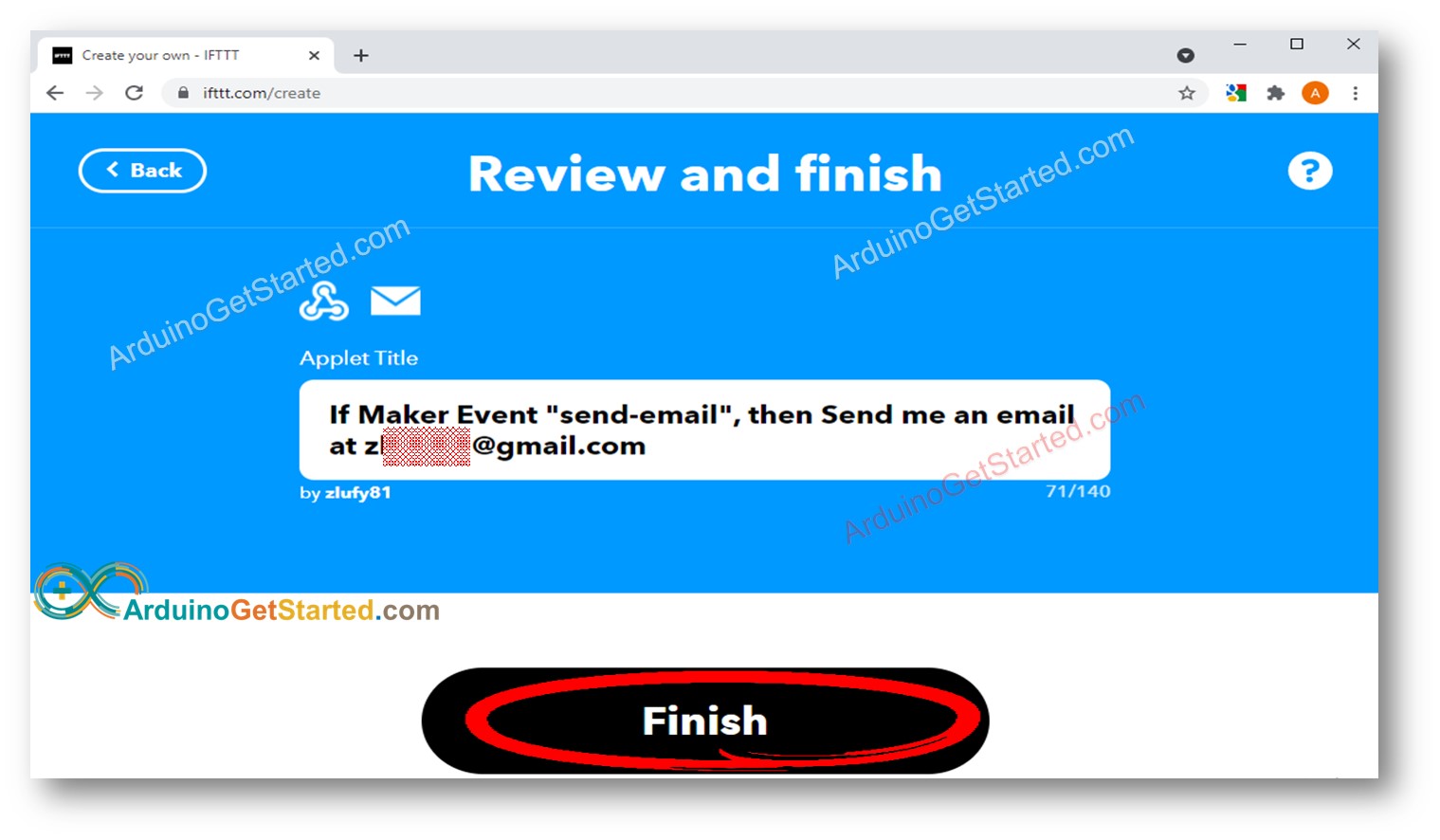
Now you succeeded to create an IFTTT Applet for your Arduino. The next step is to get the IFTTT Webhooks key, which is used to authenticate and identify your Arduino.
- Visit IFTTT Webhooks page
- Click the Documentation button
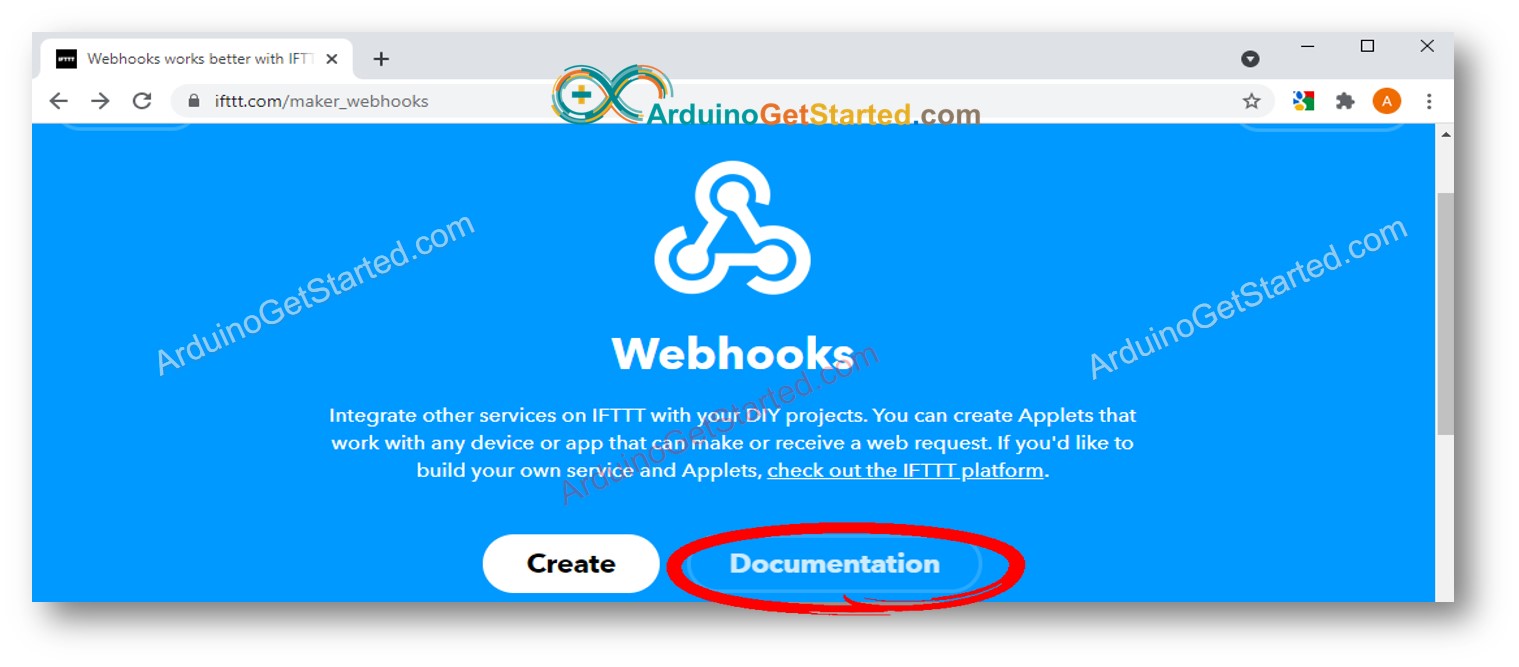
- You will see the Webhooks key as below
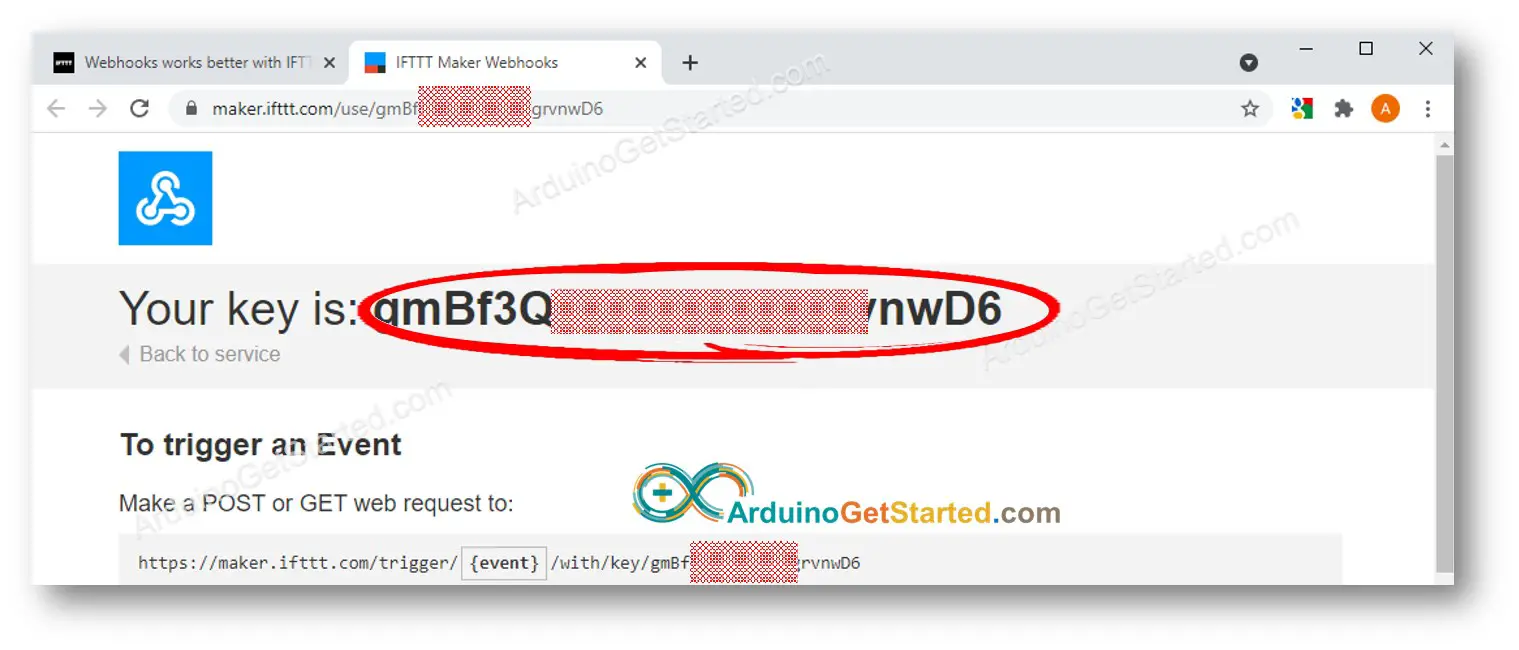
- Copy and wire down your Webhooks to use on Arduino code.
Now, We just need to write Arduino code that makes an HTTP request to the below URL:
※ NOTE THAT:
- In the URL, the send-email is the event name we used:
- If you already used this name for another Applet, you can give it any other name.
- If you use another name, please replace the send-email by your event name
- Webhooks key is generated by IFTTT. Please keep it secret. You can change it by regenerating it on the IFTTT website.
Before writing Arduino code, We can test the IFTTT Applet by doing:
- Open a web browser
- Copy the below link:
- Paste it on the address bar of the web browser
- Replace XXXXXXXXXXXXXXXXXXXXX with your Webhooks key.
- Press the enter key on your keyboard. You will see the browser display the message as below image.
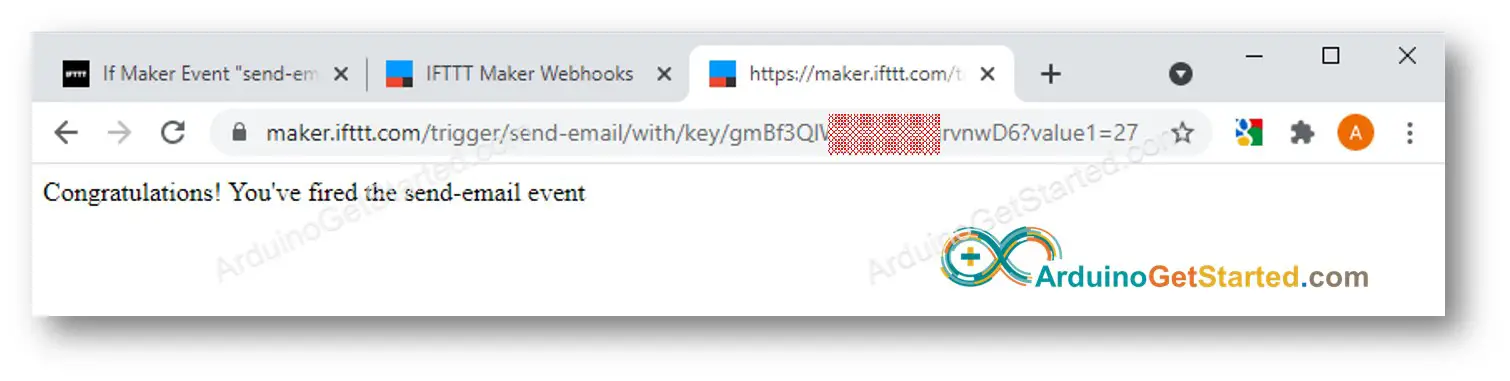
- And you will receive an email.
Arduino Code for Arduino Uno R4 WiFi
Arduino Code for Arduino and Ethernet Shield
Quick Steps
- If using the Ethernet Shield, stack Ethernet Shield on Arduino
- Do wiring as above image
- Install the door sensor on your door.
- Connect Arduino to PC via USB cable
- Open Arduino IDE, select the right board and port
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
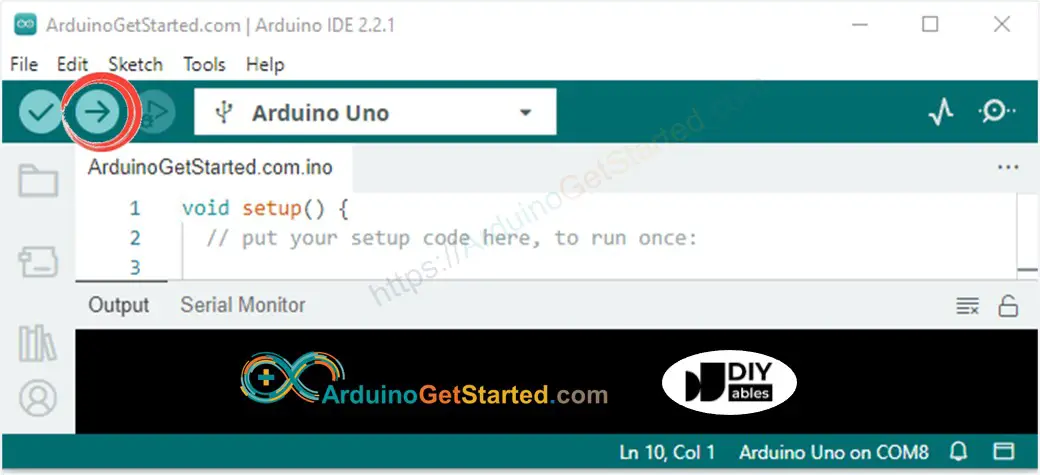
- Close and then open the door
- You will receive an email notification as below
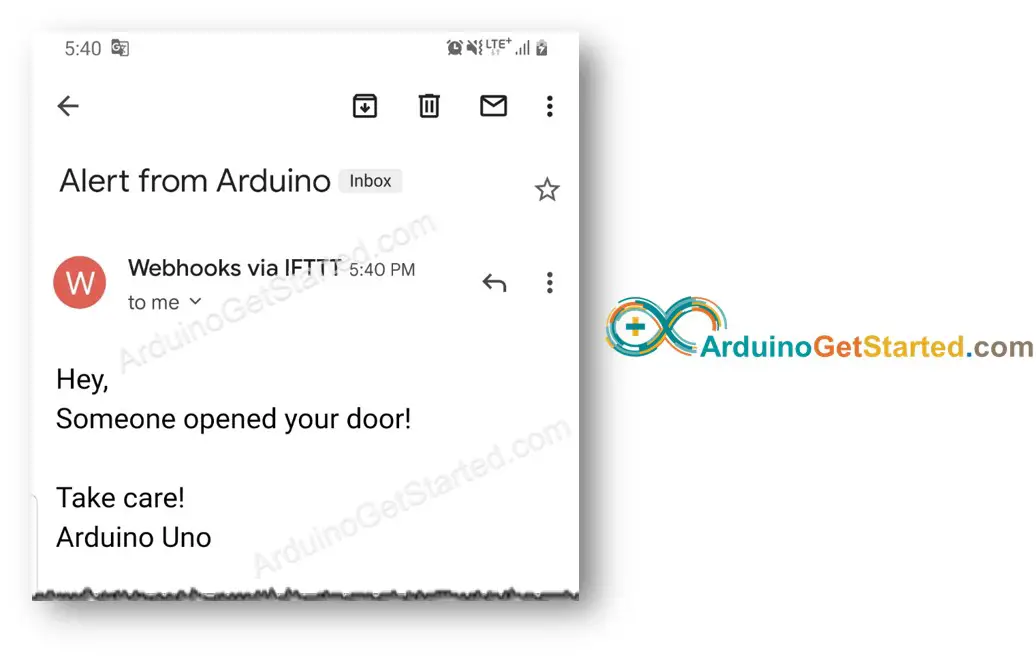
- The log on Serial Monitor as below:
Code Explanation
Read the line-by-line explanation in comment lines of source code!
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.