Arduino - Web Server Password
In this tutorial, we'll learn how to create a secure Arduino web server that requires a username and password for login. Before accessing any web pages hosted on the Arduino, users will be prompted to enter their username and password.
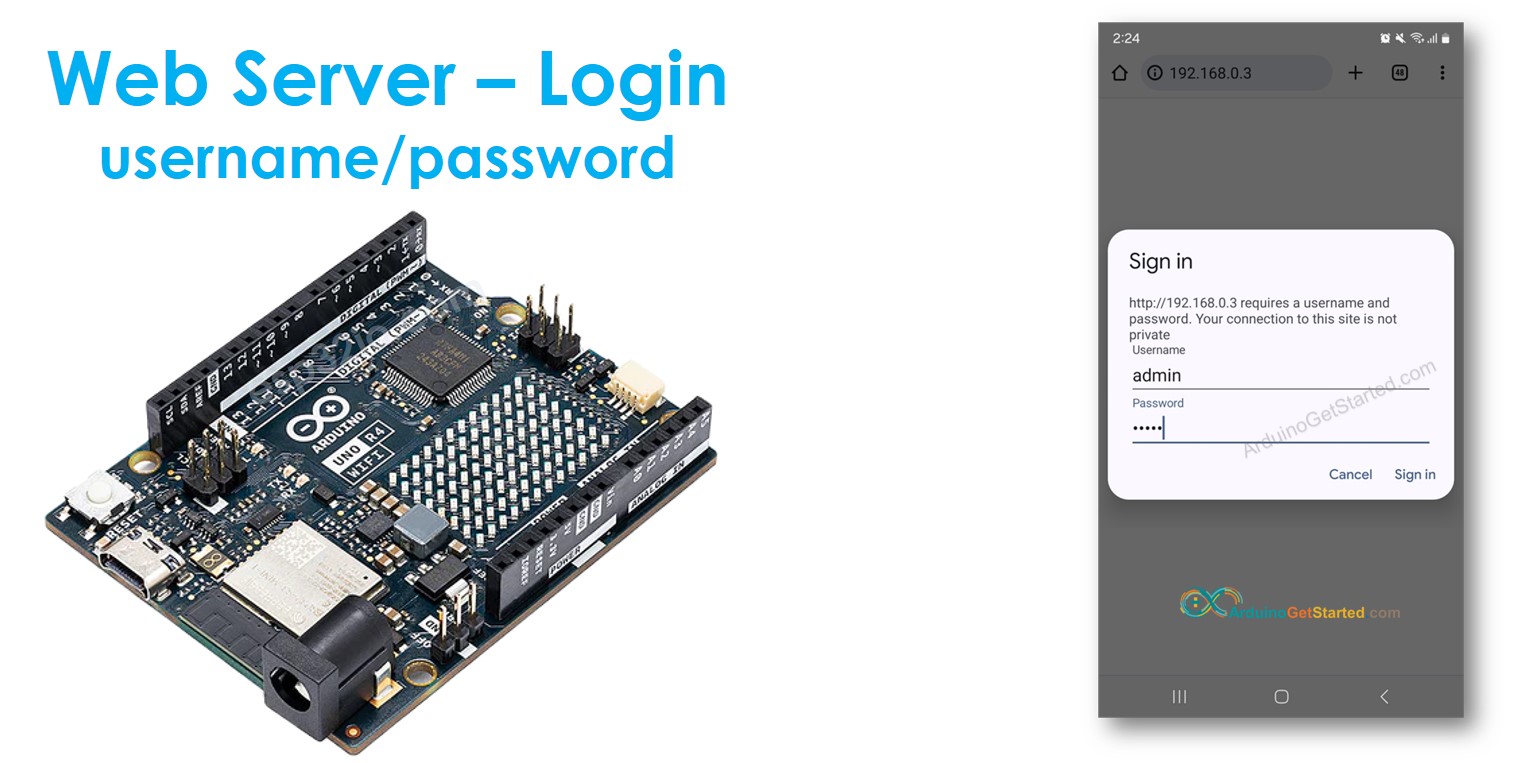
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some links in this section are Amazon affiliate links. If you make a purchase through these links, we may earn a commission at no extra cost to you.
Additionally, some links direct to products from our own brand, DIYables.
Additionally, some links direct to products from our own brand, DIYables.
About Arduino and Web Server
If you're not familiar with Arduino and Web Server (including pinout, how it works, and programming), you can learn about them through the following tutorials:
Arduino Code - Web server Username/Password
/*
* Created by ArduinoGetStarted.com
*
* This example code is in the public domain
*
* Tutorial page: https://arduinogetstarted.com/tutorials/arduino-web-server-password
*/
#include <WiFiS3.h>
const char ssid[] = "YOUR_WIFI_SSID"; // change your network SSID (name)
const char pass[] = "YOUR_WIFI_PASSWORD"; // change your network password (use for WPA, or use as key for WEP)
int status = WL_IDLE_STATUS;
WiFiServer server(80);
const char* www_username = "admin";
const char* www_password = "arduino";
void setup() {
Serial.begin(9600);
delay(1000);
String fv = WiFi.firmwareVersion();
if (fv < WIFI_FIRMWARE_LATEST_VERSION)
Serial.println("Please upgrade the firmware");
// attempt to connect to WiFi network:
while (status != WL_CONNECTED) {
Serial.print("Attempting to connect to SSID: ");
Serial.println(ssid);
// Connect to WPA/WPA2 network. Change this line if using open or WEP network:
status = WiFi.begin(ssid, pass);
// wait 5 seconds for connection:
delay(5000);
}
server.begin();
Serial.print("IP Address: ");
Serial.println(WiFi.localIP());
}
void loop() {
// Check if a client has connected
WiFiClient client = server.available();
if (client) {
Serial.println("Client connected");
boolean authorized = checkAuthorization(client);
if (authorized) {
Serial.println("Client is authorized");
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: text/html");
client.println("Connection: close");
client.println();
// replaced by your HTML code here:
client.println("<!DOCTYPE HTML>");
client.println("<html>");
client.println("<head><title>Arduino Web Server</title></head>");
client.println("<body>");
client.println("<h1>Login Successful!</h1>");
client.println("<p>You are now logged in.</p>");
client.println("</body>");
client.println("</html>");
} else {
Serial.println("Client is unauthorized");
client.println("HTTP/1.1 401 Unauthorized");
client.println("WWW-Authenticate: Basic realm=\"Arduino\"");
client.println("Content-Type: text/html");
client.println("Connection: close");
client.println();
client.println("<!DOCTYPE HTML>");
client.println("<html>");
client.println("<head><title>401 Unauthorized</title></head>");
client.println("<body><h1>401 Unauthorized</h1></body>");
client.println("</html>");
}
// Close the connection
delay(1);
client.stop();
Serial.println("Client disconnected");
}
}
boolean checkAuthorization(WiFiClient client) {
boolean authorized = false;
while (client.connected()) {
String header = client.readStringUntil('\n');
//Serial.println(header);
if (header.startsWith("Authorization:")) {
String encodedCreds = header.substring(21, header.length() - 1);
String decodedCreds = base64_decode(encodedCreds);
String userPass = String(www_username) + ":" + String(www_password);
if (decodedCreds.equals(userPass)) {
authorized = true;
}
} else if (header == "\r") { // Detect empty line to break
break;
}
}
return authorized;
}
String base64_decode(String input) {
String decoded = "";
int padding = 0;
for (int i = 0; i < input.length(); i += 4) {
long value = 0;
for (int j = 0; j < 4; j++) {
value = value << 6;
if (input[i + j] == '=') {
padding++;
} else {
if (input[i + j] >= 'A' && input[i + j] <= 'Z') {
value += input[i + j] - 'A';
} else if (input[i + j] >= 'a' && input[i + j] <= 'z') {
value += input[i + j] - 'a' + 26;
} else if (input[i + j] >= '0' && input[i + j] <= '9') {
value += input[i + j] - '0' + 52;
} else if (input[i + j] == '+') {
value += 62;
} else if (input[i + j] == '/') {
value += 63;
}
}
}
for (int j = 0; j < 3 - padding; j++) {
char c = (value >> ((2 - j) * 8) & 0xFF);
decoded += c;
}
}
return decoded;
}
Quick Steps
- If this is the first time you use Arduino Uno R4, see how to setup environment for Arduino Uno R4 on Arduino IDE.
- Connect the Arduino board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the correct Arduino board and COM port.
- Copy the above code and open with Arduino IDE
- Change the wifi information (SSID and password) in the code to yours
- Click Upload button on Arduino IDE to upload code to Arduino
- Open the Serial Monitor
- Check out the result on Serial Monitor.
COM6
Connecting to WiFi...
Connected to WiFi
Arduino Web Server's IP address: 192.168.0.3
Autoscroll
Clear output
9600 baud
Newline
- You will see an IP address on the Serial Monitor, for example: 192.168.0.3
- Type the IP address on the address bar of a web browser on your smartphone or PC.
- Please note that you need to change the 192.168.0.3 to the IP address you got on Serial Monitor.
- You will see a page that prompt you to input username/password.
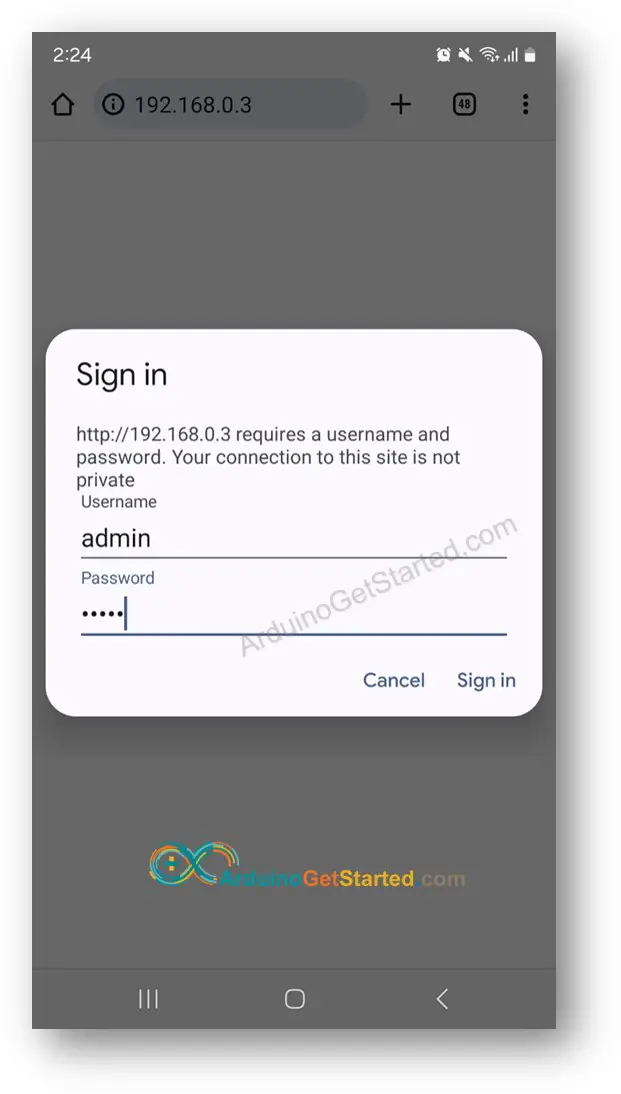
- Type username/password that are in the Arduino code, in this case: admin as username, arduino as password
- If you input the username/password correctly, the web content of Arduino will be shown:
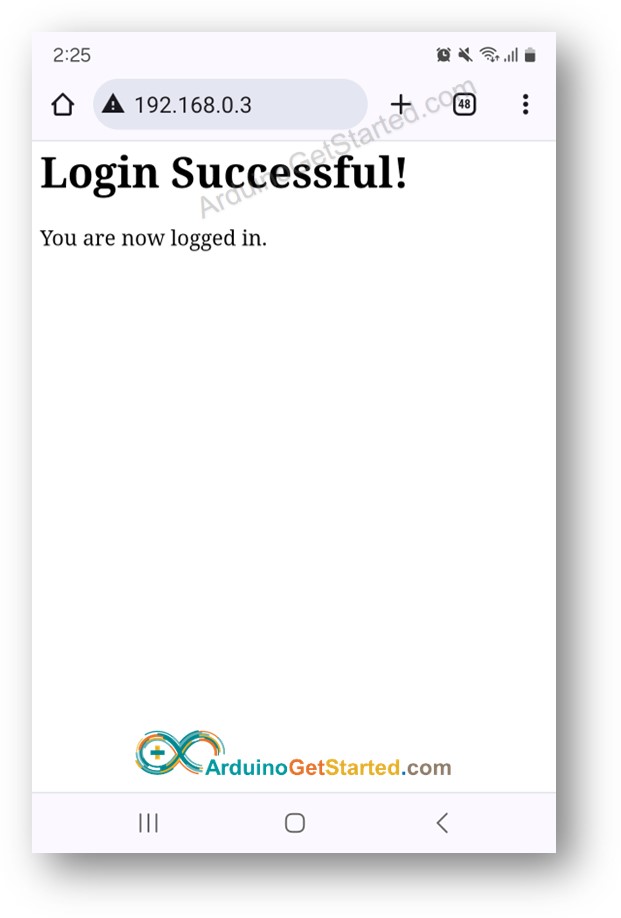
※ NOTE THAT:
- You can adjust the username and password for your website directly in the code by changing the values assigned to two variables: www_username and www_password.
- You have the flexibility to customize this code by adding your own HTML, CSS, and JavaScript to design your webpage according to your preferences.
- It's important to note that within the code itself, there isn't any HTML code specifically for the login form where users input their username and password. Don't be surprised by this! Instead, the login form is generated dynamically by the web browser when needed.
Advanced Knowledge
This section provides in-depth information on how the username/password system operates without HTML for the login form:
- When you initially type in the IP address of the Arduino into a web browser, the browser sends a request to the Arduino via HTTP, but without any username/password details.
- Upon receiving this request, the Arduino's code checks if there are any username/password credentials included. If none are found, the Arduino doesn't respond with the page's content. Instead, it sends back an HTTP message with headers instructing the browser to ask the user for their username/password. Importantly, this response doesn't contain HTML code for the login form.
- When the browser gets this response, it interprets the HTTP headers and understands that the Arduino is asking for a username/password. Consequently, the browser dynamically creates a login form for the user to input their credentials.
- The user then enters their username/password into this form.
- The browser includes the entered username/password in an HTTP request and sends it back to the Arduino.
- The Arduino checks the username/password included in the HTTP request. If they are correct, it provides the content of the requested page. If they are incorrect, the process is repeated, prompting the user to input the correct credentials again.
- Once the user correctly inputs their username/password for the first time, subsequent requests do not require them to re-enter the details. This is because the browser automatically saves the credentials and includes them in subsequent requests.