Arduino - Temperature Humidity Sensor
In this tutorial, we are going to learn:
- The differences between DHT11 and DHT22 temperature and humidity sensor
- How to read temperature and humidity value from DHT11 using Arduino
- How to read temperature and humidity value from DHT22 using Arduino
If you only want to get temperature, we recommend using a waterproof DS18B20 temperature sensor instead. It is an inexpensive and neat sensor. You can put it in hot or cold water.
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
About DHT11 and DHT22 Temperature and Humidity Sensor
The commons between DHT11 and DHT22
- Pinouts are the same
- Wiring to Arduino is the same
- Programming (with a library) is similar (only one line of code is different)
The differences between DHT11 and DHT22
DHT11 | DHT22 | |
---|---|---|
Price | ultra low cost | low cost |
Temperature Range | 0°C to 50°C | -40°C to 80°C |
Temperature Accuracy | ± 2°C | ± 0.5°C |
Humidity Range | 20% to 80% | 0% to 100% |
Humidity Accuracy | 5% | ± 2 to 5% |
Reading Rate | 1Hz (once every second) | 0.5Hz (once every 2 seconds) |
Body size | 15.5mm x 12mm x 5.5mm | 15.1mm x 25mm x 7.7mm |
Operating Voltage | 3 to 5V | 3 to 5V |
As you can see, the DHT22 is a little more accurate, larger range but more expensive than DHT11.
Pinout
DHT11 and DHT22 sensor in original form have four pins:
- GND pin: needs to be connected to GND (0V)
- VCC pin: needs to be connected to VCC (5V, or 3.3V)
- DATA pin: the pin is used to communicate between the sensor and Arduino
- NC pin: Not connected, we can ignore this pin
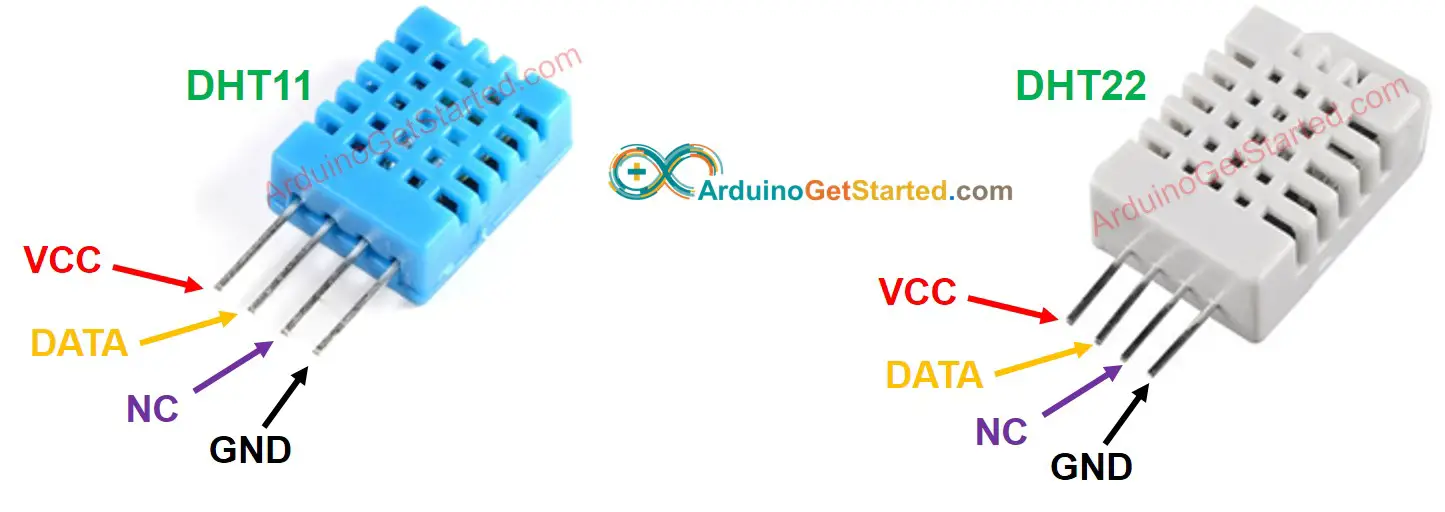
Some manufacturers provide DHT11 and DHT22 sensor in module form with three pins: GND, VCC and DATA pins (or alternatively: -, +, and OUT pins).
※ NOTE THAT:
In module form, the order of the module's pins can vary between manufacturers. ALWAYS use the labels printed on the module. Look closely!
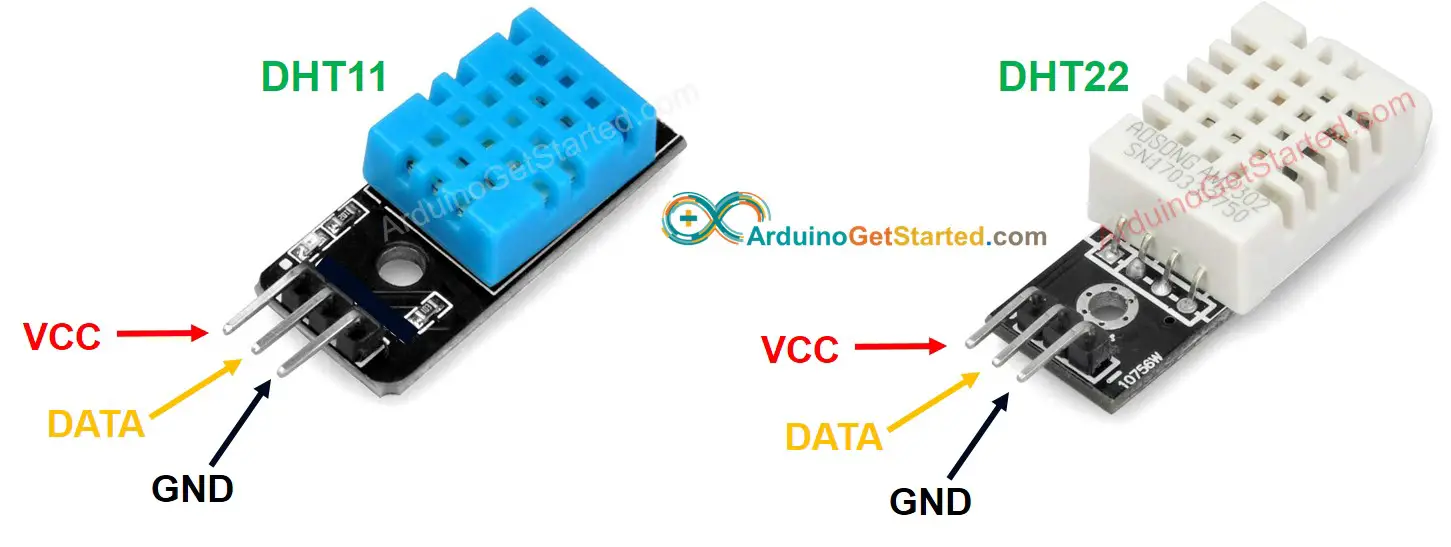
Wiring Diagram
Wiring to Arduino is the same for both sensors. In orginal form, A resistor from 5K to 10K Ohms is required to keep the data line high and in order to enable the communication between the sensor and the Arduino
Arduino - DHT11 Sensor Wiring
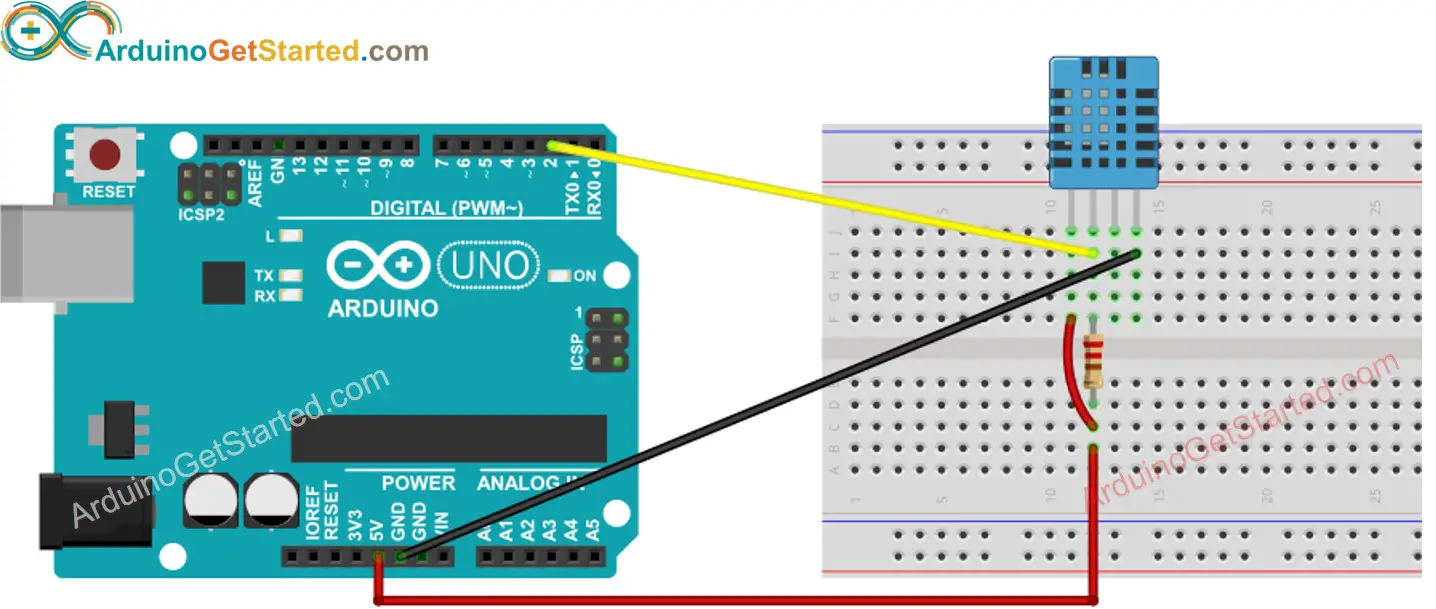
This image is created using Fritzing. Click to enlarge image
Arduino - DHT22 Sensor Wiring
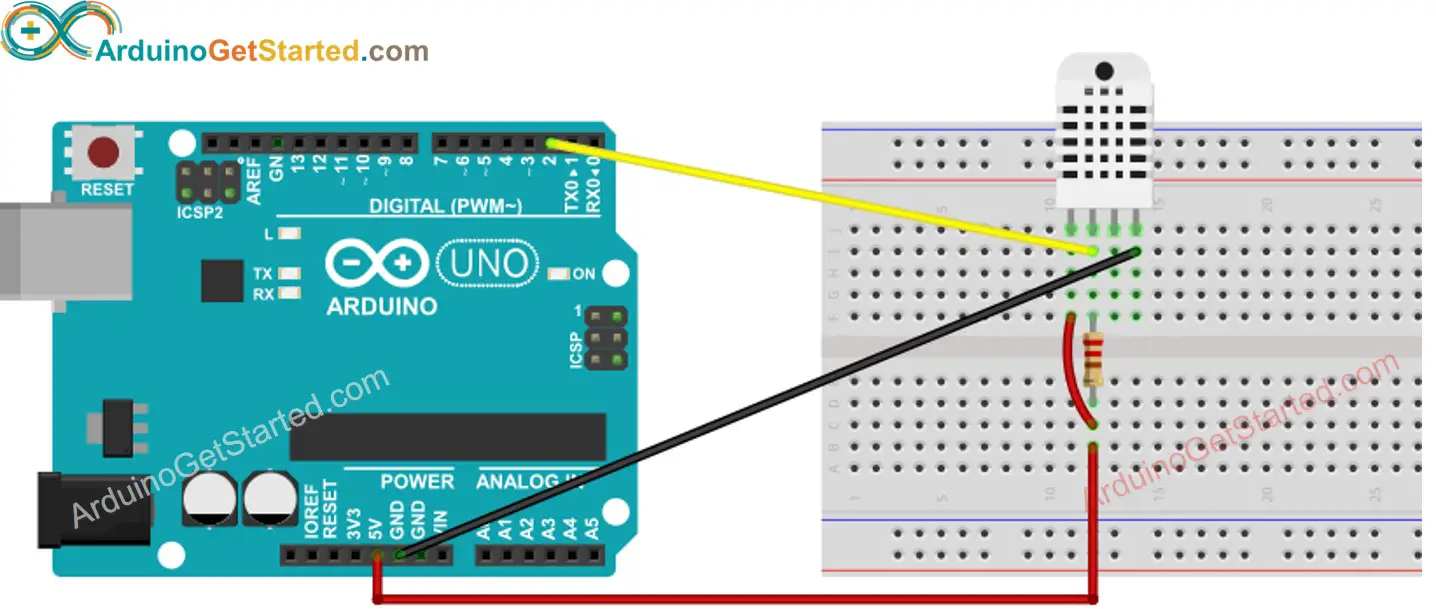
This image is created using Fritzing. Click to enlarge image
Arduino - DHT11 Module Wiring
Most of DHT22 sensor modules have a built-in resistor, so you don't need to add it. it saves us some wiring or soldering works.
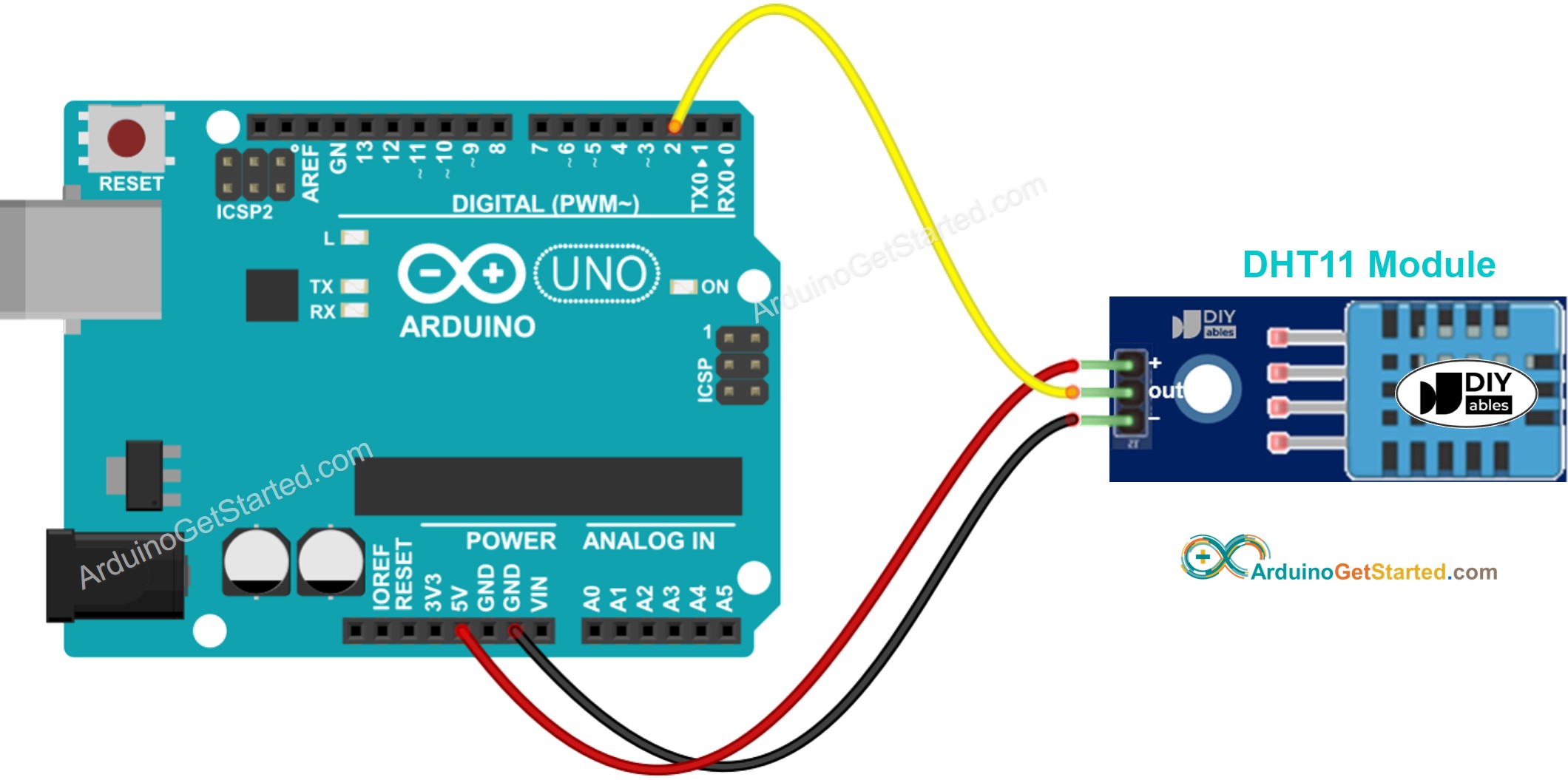
This image is created using Fritzing. Click to enlarge image
Arduino - DHT22 Module Wiring
Most of DHT22 sensor modules have a built-in resistor, so you don't need to add it. it saves us some wiring or soldering works.
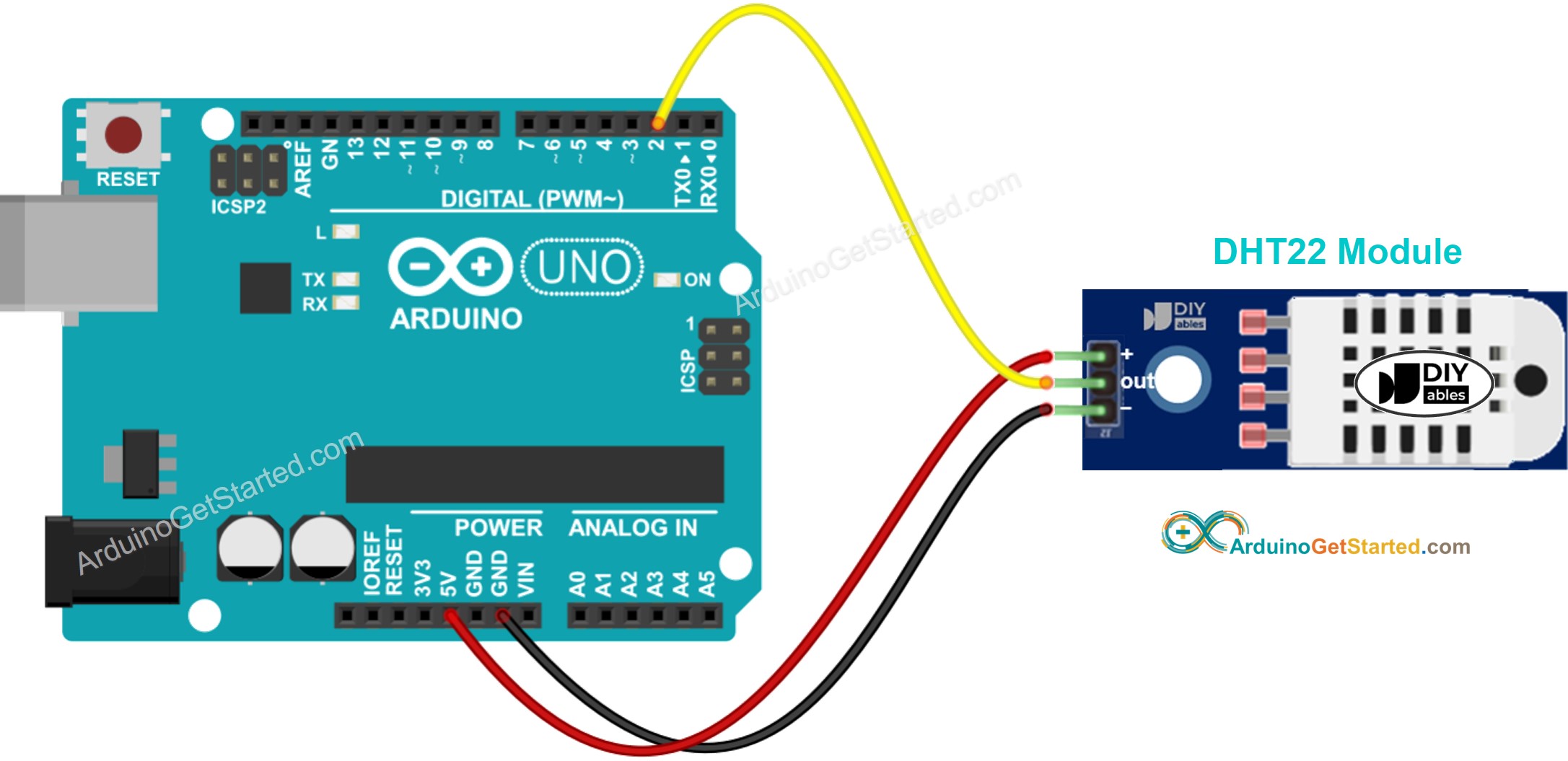
This image is created using Fritzing. Click to enlarge image
How To Program For DHTxx Temperature Sensor
Programming for both sensors is similar. There is only one line of code different.
- Include the library:
- Define the Arduino pin connected to DHT sensor:
- Define the sensor type: DHT11 or DHT22 (This is the different line of code)
or
- Declare DHT object
- Initialize the sensor:
- Read humidity:
- Read temperature in Celsius:
- Read temperature in Fahrenheit:
Arduino Code - DHT11
Arduino Code - DHT22
If you compare two above code, you realize that there is one line different (line 11)
Quick Steps
- Connect Arduino to PC via USB cable
- Open Arduino IDE, select the right board and port
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search “DHT”, then find the DHT sensor library by Adafruit
- Click Install button to install the library.
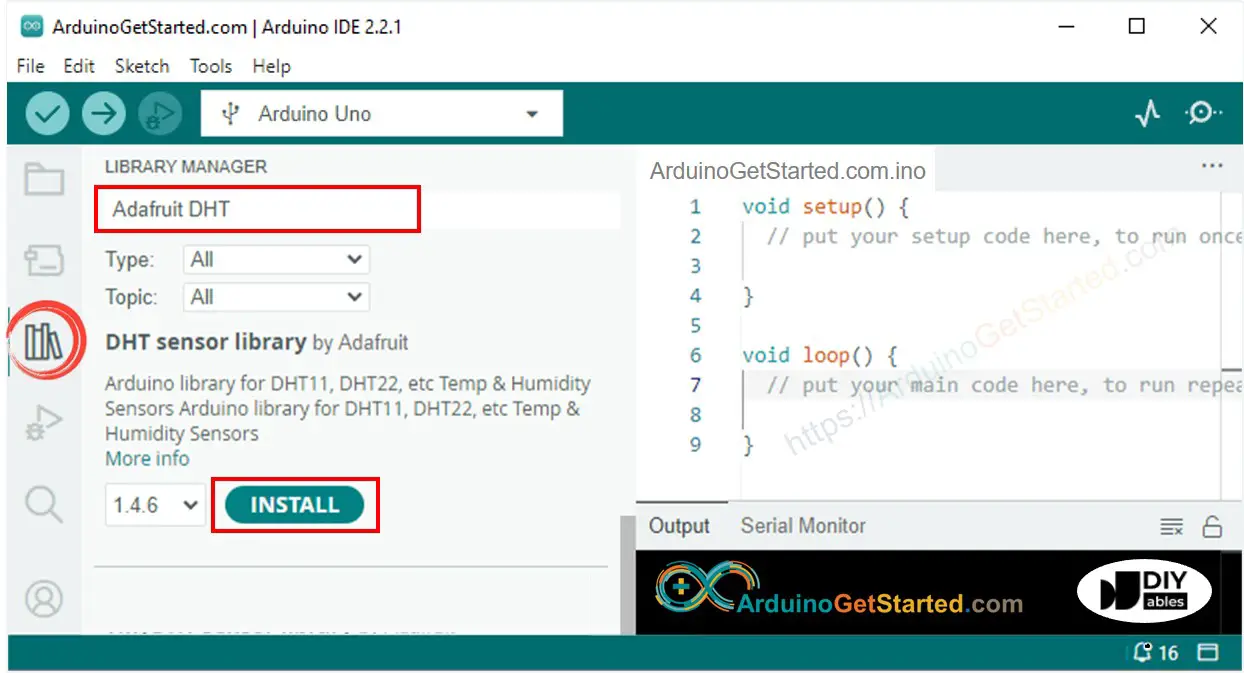
- You will be asked for intalling some other library dependencies
- Click Install All button to install all library dependencies.
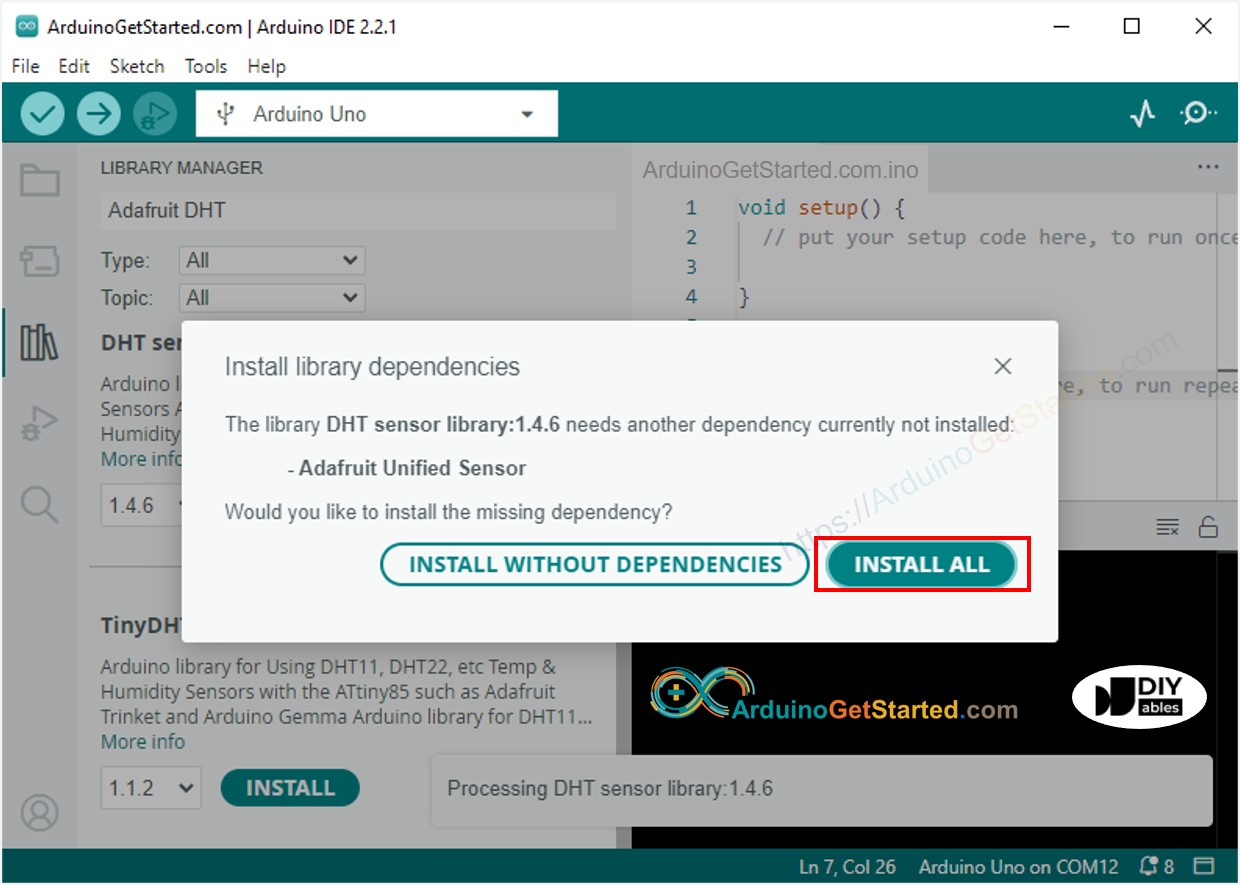
- Copy the above code corresponding to the sensor you have and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Make enviroment around sensor hotter or colder
- See the result on Serial Monitor.
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.